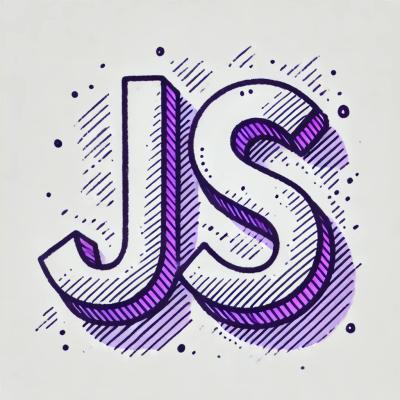
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
babel-template
Advanced tools
The babel-template npm package is a utility that allows developers to create reusable AST (Abstract Syntax Tree) templates based on a string input. It simplifies the process of generating AST nodes programmatically, which is particularly useful when working with Babel plugins that manipulate code.
AST Template Creation
This feature allows the creation of AST nodes from a template string. The example shows how to create a variable declaration that requires a module, with placeholders for the module name and source.
const template = require('babel-template');
const buildRequire = template(`
var IMPORT_NAME = require(SOURCE);
`);
const ast = buildRequire({
IMPORT_NAME: 'myModule',
SOURCE: '"my-module"'
});
This package is an official part of the Babel ecosystem and serves a similar purpose to babel-template, providing API improvements and better integration with the latest Babel versions. It offers enhanced usability and security features.
Recast is another tool for manipulating ASTs. It focuses on preserving the original formatting as much as possible, which is different from babel-template's approach of creating new AST nodes from templates. Recast is better suited for applications where code formatting needs to be maintained.
Generate an AST from a string template.
In computer science, this is known as an implementation of quasiquotes.
npm install --save-dev babel-template
import template from "babel-template";
import generate from "babel-generator";
import * as t from "babel-types";
const buildRequire = template(`
var IMPORT_NAME = require(SOURCE);
`);
const ast = buildRequire({
IMPORT_NAME: t.identifier("myModule"),
SOURCE: t.stringLiteral("my-module")
});
console.log(generate(ast).code);
const myModule = require("my-module");
template(code, [opts])
Type: string
babel-template
accepts all of the options from babylon, and specifies
some defaults of its own:
allowReturnOutsideFunction
is set to true
by default.allowSuperOutsideMethod
is set to true
by default.Type: boolean
Default: false
Set this to true
to preserve any comments from the code
parameter.
babel-template
returns a function
which is invoked with an optional object
of replacements. See the usage section for an example.
FAQs
Generate an AST from a string template.
The npm package babel-template receives a total of 2,188,360 weekly downloads. As such, babel-template popularity was classified as popular.
We found that babel-template demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.