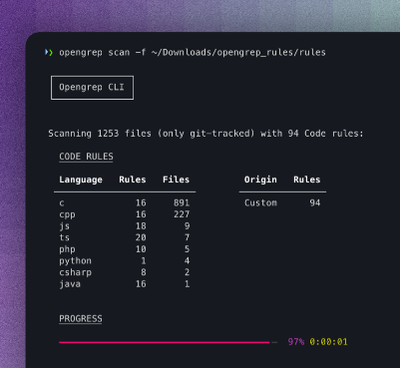
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
backbone-session
Advanced tools
Flexible and simple session management for Backbone apps
// Using CommonJS
var Session = require('backbone-session');
// or AMD
define(['backbone-session'], function (Session) {
// ...
})
// Extend from Session to implement your API's behaviour
var Account = Session.extend({
signIn: function () {},
signOut: function () {},
getAuthStatus: function () {}
});
// Using the custom Account implementation
var session = new Account();
session.fetch()
.then(session.getAuthStatus)
.then(function () {
console.log('Logged in as %s', session.get('name'));
})
.fail(function () {
console.log('Not yet logged in!');
});
Using a simple facade that feels more Backboney helps avoid third party SDKs and APIs leaking into your app code. Your app will be less locked-in to your authentication provider.
Backbone Session uses the localStorage
API to cache tokens and user profile information.
Backbone Session is merely a facade, or interface. It's up to you to implement its methods to do what your API requires.
Many Backbone apps will have a singleton app
object that tracks state. That's a good place to keep your Backbone Session instance.
Backbone Session implementations can be synchronous or asynchronous. For the sake of consistency, it is recommended to use Promises even with a syncronous implementation.
Backbone Session inherits all of Backbone Models's methods and properties.
It overrides and extends the following interfaces:
Default: Backbone.Session
Either a Function or a String that represents the key used to access localStorage
. If a Function, its return value will be the key.
Returns: jQuery Promise
Example:
session.signIn({
username: 'alice@example.com',
password: 'hunter2'
}).then(function () {
// Do stuff after logging in ...
}).fail(function () {
// Handle the failed log in attempt ...
});
Returns: jQuery Promise
Example:
session.signOut().then(function () {
// Now the user is logged out ...
});
Returns: jQuery Promise
Example:
session.getAuthStatus()
.then(function () {
// The user is already logged in ...
})
.fail(function () {
// The user is not yet logged in ...
});
A basic Backbone Model and Collection to extend and inherit from. Session implementations can replace them with a patched Model or Collection to seamlessly handle network authentication, error handling, logging, etc. Session consumers should extend their models from this base.
Example:
// Session implementation
var MyAPI = Backbone.Session.extend({
Model: Backbone.Model.extend({
sync: function () {
console.log('Syncing...');
return Backbone.Model.sync.apply(this, arguments);
}
})
});
// Session consumer
var session = new MyAPI();
var MyModel = session.prototype.Model.extend({
url: '/foo/bar'
});
var item = new MyModel();
item.fetch(); // prints: Syncing...
bower install backbone-session
npm install backbone-session
or
package.json
"dependencies": {
"backbone-session": ""
}
<script src="backbone-session.js"></script>
FAQs
Flexible and simple session management for Backbone apps
The npm package backbone-session receives a total of 5 weekly downloads. As such, backbone-session popularity was classified as not popular.
We found that backbone-session demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.