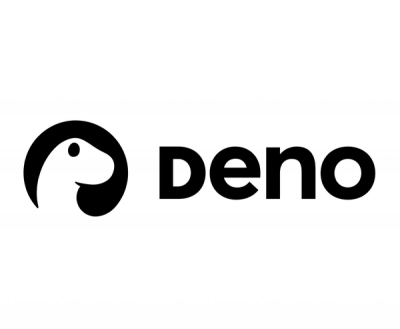
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
A tiny javascript library for obfuscating and revealing text in DOM elements.
// Select elements and start.
let b = baffle('.someSelector').start();
// Do something else.
someAsyncFunction(result => {
// Change the text and reveal over 1500ms.
b.text(text => result.text).reveal(1500);
});
Download the latest release or install with npm.
npm install --save baffle
If you linked baffle directly in your HTML, you can use window.baffle
. If you're using a module bundler, you'll need to import baffle.
// CommonJS
let baffle = require('baffle');
// ES6
import baffle from 'baffle';
To initialize baffle, all you need to do is call it with some elements. You can pass a NodeList, Node, or CSS selector.
// With a selector.
let b = baffle('.baffle');
// With a NodeList
let b = baffle(document.querySelectorAll('.baffle'));
// With a Node
let b = baffle(document.querySelector('.baffle'));
Once you have a baffle instance, you have access to all of the baffle methods. Usually, you'll want to b.start()
and, eventually, b.reveal()
.
// Start obfuscating...
b.start();
// Or stop obfuscating...
b.stop();
// Obfuscate once...
b.once();
// You can set options after intializing...
b.set({...options});
// Or change the text at any time...
b.text(text => 'Hi Mom!');
// Eventually, you'll want to reveal your text...
b.reveal(1000);
// And they're all chainable...
b.start()
.set({ speed: 100 })
.text(text => 'Hi dad!')
.reveal(1000);
You can set options on baffle during initialization or anytime afterward with baffle.set()
.
// During initialize
baffle('.baffle', {
characters: '+#-•=~*',
speed: 75
});
// Any time with set()
b.set({
characters: ' ¯\_(ツ)_/¯',
speed: 25
});
###
options.characters
The characters baffle uses to obfuscate your text. It can be a string or an array of characters.Default:
'AaBbCcDdEeFfGgHhIiJjKkLlMmNnOoPpQqRrSsTtUuVvWwXxYyZz~!@#$%^&*()-+=[]{}|;:,./<>?'
options.speed
This is the frequency (in milliseconds) at which baffle updates your text when running.
Default:
50
An instance of baffle has six methods, all of which are chainable.
###
baffle.once()
Obfuscates each element once, usingoptions.characters
.
###
baffle.start()
Starts obfuscating your elements, updating everyoptions.speed
milliseconds.
###
baffle.stop()
Stops obfuscating your elements. This won't reveal your text. It will only stop updating it. To reveal it, usereveal()
.
###
baffle.reveal([duration], [delay])
Reveals your text overduration
milliseconds (default:0
), with the option to delay bydelay
milliseconds.
###
baffle.set([options])
Updates instance options using the passedoptions
object. You can set any number of keys, even while running.
###
baffle.text(fn)
Updates the text in each element of your instance using functionfn
, which receives the current text as it's only parameter. The value returned fromfn
will be used as the new text.
FAQs
A tiny javascript library for obfuscating and revealing text in DOM elements.
The npm package baffle receives a total of 152 weekly downloads. As such, baffle popularity was classified as not popular.
We found that baffle demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.