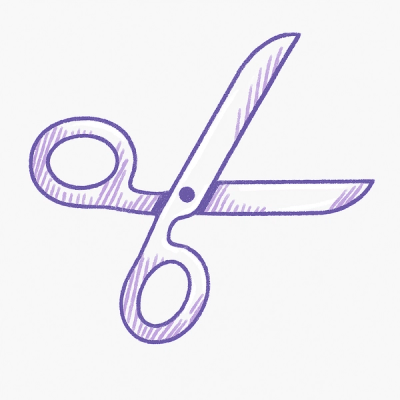
Security News
Knip Hits 500 Releases with v5.62.0, Improving TypeScript Config Detection and Plugin Integrations
Knip hits 500 releases with v5.62.0, refining TypeScript config detection and updating plugins as monthly npm downloads approach 12M.
General reactive JavaScript programming using the idea of React Hooks.
Even though React Hooks are actually a constrained solution for using state and managing side effects in functional stateless components, they have proven to be very elegant in their design. I wanted to use this kind of reactive programming in areas other than React development, so I wrote Batis.
Batis essentially revolves around the concept of a Hook and its host. Running a functional stateless Hook requires a host that manages the state and effects and reports asynchronous state changes that should result in a new run.
npm install batis
import {Host, useEffect, useLayoutEffect, useMemo, useState} from 'batis';
function useGreeting(salutation) {
const [name, setName] = useState(`John`);
useLayoutEffect(() => {
setName(`Jane`);
}, []);
useEffect(() => {
// Unlike React, Batis always applies all state changes, whether
// synchronous or asynchronous, in batches. Therefore, Janie is not
// greeted individually.
setName(`Janie`);
setName((prevName) => `${prevName} and Johnny`);
const handle = setTimeout(() => setName(`World`), 0);
return () => clearTimeout(handle);
}, []);
return useMemo(() => `${salutation} ${name}!`, [salutation, name]);
}
const greeting = new Host(useGreeting);
console.log(greeting.run(`Hi`));
console.log(greeting.rerun());
greeting.reset();
console.log(greeting.run(`Bye`));
console.log(greeting.rerun());
await greeting.nextAsyncStateChange;
console.log(greeting.run(`Hello`));
[ 'Hi Jane!', 'Hi John!' ]
[ 'Hi Janie and Johnny!' ]
[ 'Bye Jane!', 'Bye John!' ]
[ 'Bye Janie and Johnny!' ]
[ 'Hello World!' ]
The React Hooks API reference also applies to this library and should be consulted.
Below you can see the subset of React Hooks implemented by Batis:
React Hook | Status |
---|---|
useState | ✅Implemented |
useEffect | ✅Implemented |
useLayoutEffect | ✅Implemented |
useMemo | ✅Implemented |
useCallback | ✅Implemented |
useRef | ✅Implemented |
useReducer | ✅Implemented |
useContext | ❌Not implemented |
useImperativeHandle | ❌Not implemented |
useDebugValue | ❌Not implemented |
FAQs
General reactive JavaScript programming using the idea of React Hooks.
The npm package batis receives a total of 21 weekly downloads. As such, batis popularity was classified as not popular.
We found that batis demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Knip hits 500 releases with v5.62.0, refining TypeScript config detection and updating plugins as monthly npm downloads approach 12M.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.