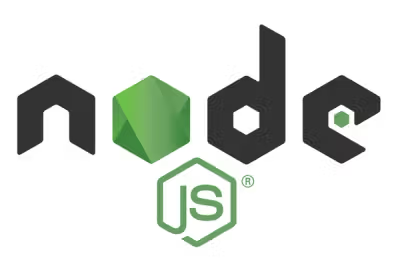
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Dynamic bytes buffer for nodejs/iojs, a bit like the bytearray
in Python.
+------- cap -------+
+------- size --+ | => buf (uint8 array)
|UNIT|UNIT|UNIT|UNIT|
Reasons to start this project:
Buffer.concat
, s + s
etc.var Buf = require('bbuf').Buf;
var buf = new Buf(4); // created with buf unit <bbuf [0]>
buf.put('abc'); // 3 buf => <bbuf [3] 61 62 63>
buf.put('123'); // 3 buf => <bbuf [6] 61 62 63 31 32 33>
buf.pop(4); // 4 buf => <bbuf [2] 61 62>
buf.length // 2
buf.cap // 8
buf.toString() // 'ab'
buf.clear() // 2 buf => <bbuf [0]>
buf.cap // 0
Create a buf instance by buffer unit size.
Test if an object is a Buf instance.
Put string/buffer/buf/byte/bytes-array object to buf, return bytes put. O(k)
buf.put('abcd'); // 4
buf.put(buf);
buf.put(new Buffer('abcd'));
buf.put(97);
buf.put([98, 99, 100]);
// buf.toString() => 'abcdabcdabcdabcd'
Pop buf on the right end, return bytes poped. O(1)
Get/Set buf size.
buf.put('abcd'); // buf => <bbuf [4] 61 62 63 64>
buf.length // 4
buf.length = 5; // buf => <bbuf [5] 61 62 63 64 20> , appended a ' '
buf.length = 2; // buf => <bbuf [2] 61 62> // truncate
Get buf capacity.
Grow buf capacity to given size.
(We dont need to grow size manually to put data onto buf, but this method can reduce the memory allocation times if the result's bytes size is known to us).
Return string from buf.
buf.put('abcd');
buf.toString(); // 'abcd'
Clear buf. O(!)
buf.put('abcd');
buf.clear();
buf.length // 0
buf.cap // 0
Copy buf into a new buf instance. O(n)
buf.put('abcd'); // buf => <bbuf [4] 61 62 63 64>
buf.copy(); // <bbuf [4] 61 62 63 64>
Index accessors for a buf instance. all O(1)
buf.put('abced');
buf[0]; // 97
buf[0] = 'c'; // 'c'
buf[2] = 97; // 97
buf.toString(); // 'cbaed'
buf.charAt(0); // 'c'
Slice buf into a new buf instance. O(k)
buf.put('abcd'); // 4. buf => <bbuf [4] 61 62 63 64>
buf.slice(0) // <bbuf [4] 61 62 63 64>
buf.slice(-1) // <bbuf [1] 64>
buf.slice(1, 3) // <bbuf [2] 62 63>
Don't use String.prototype.slice.apply(buf, [begin, end])
,
because we are playing with bytes
but not chars
:
buf.put('你好');
String.prototype.slice.apply(buf, [0, 1]); // '你'
buf.charAt(0) !== '你'; // true
But you can use Array.prototype.slice
to slice bytes
from buf
:
buf.slice(0, 1).bytes(); // [ 228 ]
[].slice.apply(buf, [0, 1]); // [ 228 ]
Get bytes array. O(n)
buf.put('abcd'); // 4
buf.bytes(); // [ 97, 98, 99, 100 ]
Compare string/buffer/buf with this buf, similar to C's strcmp
. O(min(m, n))
buf.put('cde');
buf.cmp('abc'); // 2
buf.cmp('cde'); // 0
buf.cmp('mnp'); // -10
buf.equals('cde'); // true
buf.equals(buf.copy()); // true
Find the first index of string/buffer/buf in this buf. (Boyer-Moore algorithm)
buf.put('abcde');
buf.indexOf('ab'); // 0
buf.indexOf('what'); // -1
buf.indexOf('d', 1); // 3
buf.indexOf(98); // 1
Don't use String.prototype.indexOf
for buf
, if you are trying to find the byte index
instead of the char index
(e.g. to parse bytes from a socket):
buf.put('你好');
buf.indexOf('好'); // 3
String.prototype.indexOf.call(buf, '好'); // 1
But you can use Array.prototype.indexOf
to search a single byte:
[].indexOf.apply(buf, [228]) // 0
Test if the buf is only maked up of spaces . (' \t\n\r\v\f'
) O(n)
Test if the buf starts/ends with string/buffer/buf
. (Note that we are talking about bytes, not chars). O(min(n, k))
buf.put('abcde');
buf.startsWith('ab'); // true
buf.endsWith('de'); // true
Simple benchmark between v8 string + operator
, v8 array join
,
node buffer.write
and bbuf.put
:
v8 string + operator: 1000000 op in 202 ms => 4950495ops heapUsed: 76034848
v8 array join: 1000000 op in 379 ms => 2638522.4ops heapUsed: 71710880
node buf fixed size: 1000000 op in 355 ms => 2816901.4ops heapUsed: 14669800
bbuf dynamic size: 1000000 op in 371 ms => 2695417.8ops heapUsed: 36397128
MIT. (c) Chao Wang hit9@icloud.com
FAQs
Dynamic bytes buffer for nodejs/iojs.
We found that bbuf demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.