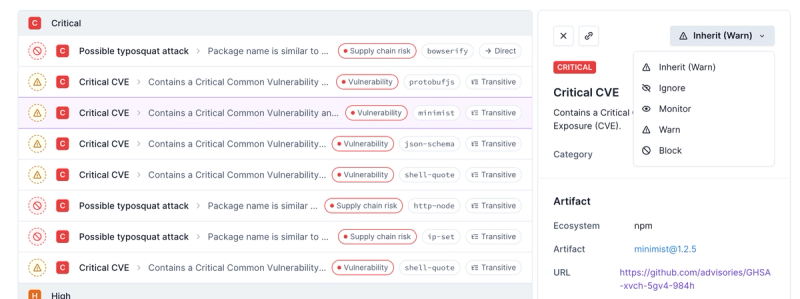
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
bent
Advanced tools
Readme
Functional HTTP client for Node.js w/ async/await.
const bent = require('bent')
const getJSON = bent('json')
const getBuffer = bent('buffer')
let obj = await getJSON('http://site.com/json.api')
let buffer = await getBuffer('http//site.com/image.png')
As you can see, bent is a function that returns an async function.
Bent takes options which constrain what is accepted by the client. Any response that falls outside the constraints will generate an error.
If you don't set a response encoding ('json'
, 'string'
or 'buffer'
)
then the response stream will be returned after the statusCode check.
const bent = require('bent')
const getStream = bent('http://site.com')
let stream = await getStream('/json.api')
The following options are available.
'GET'
, 'PUT'
, and any ALLCAPS string will be
used to set the HTTP method. Defaults to 'GET'
'string'
, 'buffer'
, and
'json'
. If no encoding is set, which is the default, the response
object/stream will be returned instead of a decoded response.200
will be the only acceptable status code, but
if status codes are provided 200
must be added explicitely.The returned async function is used for subsequent requests.
async request(url[, body=null])
const bent = require('bent')
const put = bent('PUT', 201)
await put('http://site.com/upload', Buffer.from('test'))
FAQs
Functional HTTP client for Node.js w/ async/await.
The npm package bent receives a total of 215,656 weekly downloads. As such, bent popularity was classified as popular.
We found that bent demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.