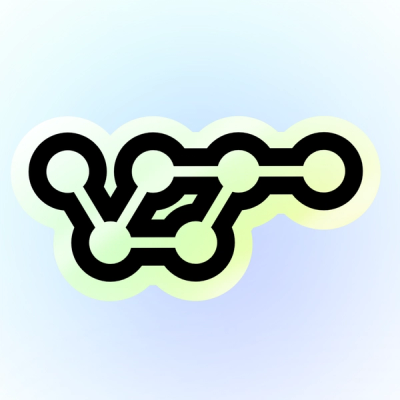
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
The bfj (Big Friendly JSON) npm package is designed to handle large JSON files efficiently. It provides functions to parse and stringify JSON asynchronously, which helps in managing large datasets without blocking the Node.js event loop. This is particularly useful for server-side applications where performance and non-blocking operations are critical.
Parse JSON asynchronously
This feature allows asynchronous parsing of JSON files. The 'read' method returns a promise that resolves with the parsed JSON data, making it suitable for large files that could block the event loop if parsed synchronously.
const bfj = require('bfj');
bfj.read('./large-file.json').then(data => {
console.log(data);
}).catch(err => {
console.error('Error parsing JSON!', err);
});
Stringify JSON asynchronously
This feature allows for asynchronous JSON stringification. The 'write' method writes JSON data to a file asynchronously and returns a promise, which is useful for handling large amounts of data without freezing the server.
const bfj = require('bfj');
const data = { large: 'data' };
bfj.write('./output.json', data).then(() => {
console.log('Data has been written successfully');
}).catch(err => {
console.error('Error writing JSON!', err);
});
Stream JSON data
This feature supports streaming JSON data using 'walk', which processes a stream of JSON data and returns a promise. It is ideal for processing data that is too large to fit into memory all at once.
const bfj = require('bfj');
const fs = require('fs');
const stream = fs.createReadStream('large-input.json');
bfj.walk(stream).then(data => {
console.log('Streamed JSON data:', data);
}).catch(err => {
console.error('Error streaming JSON!', err);
});
jsonstream is a package that provides similar functionalities to bfj, focusing on streaming JSON objects. Unlike bfj, which offers both parsing and stringifying, jsonstream primarily deals with transforming streams of JSON data.
stream-json offers tools for parsing and streaming JSON files in a memory-efficient manner. It is similar to bfj but provides more granular control over the streaming process, such as filtering and mapping streams of JSON objects.
Big-friendly JSON. Asynchronous streaming functions for large JSON data sets.
If you need to parse large JSON strings or stringify large JavaScript data sets, it makes sense to do so asynchronously rather than monopolising the event loop. BFJ implements those asynchronous functions.
Seven functions are exported.
Three are concerned with parsing, or turning JSON strings into JavaScript data:
walk
asynchronously walks
a stream,
emitting events
as it encounters
JSON tokens.
Analagous to a
SAX parser.
parse
asynchronously parses
a stream of JSON.
read
asynchronously parses
a JSON file from disk.
The four remaining functions handle the reverse transformations; serialising JavaScript data to JSON:
eventify
asynchronously traverses
a data structure
depth-first,
emitting events
as it encounters items.
By default
it coerces
promises, buffers, dates and iterables
to JSON-friendly values.
streamify
asynchronously serialises data
to a stream of JSON.
stringify
asynchronously serialises data
to a JSON string.
write
asynchronously serialises data
to a JSON file on disk.
If you're using npm:
npm install bfj --save
Or if you just want the git repo:
git clone git@github.com:philbooth/bfj.git
Import the library
using require
:
var bfj = require('bfj');
Seven functions
are exported:
walk
,
parse
,
read
,
eventify
,
streamify
,
stringify
and
write
.
walk
returns an event emitter
and asynchronously walks
a stream of JSON data,
emitting events
as it encounters
tokens.
It takes two arguments; a readable stream from which the JSON will be read and an options object that supports the following property.
options.discard
:
The number of characters
to process before
discarding them
to save memory.
The default value
is 16384
.The emitted events
are defined
as public properties
of an object,
bfj.events
:
bfj.events.array
indicates that
an array context
has been entered
by encountering
the [
character.
bfj.events.endArray
indicates that
an array context
has been left
by encountering
the ]
character.
bfj.events.object
indicates that
an object context
has been entered
by encountering
the {
character.
bfj.events.endObject
indicates that
an object context
has been left
by encountering
the }
character.
bfj.events.property
indicates that
a property
has been encountered
in an object.
The listener
will be passed
the name of the property
as its argument
and the next event
to be emitted
will represent
the property's value.
bfj.events.string
indicates that
a string
has been encountered.
The listener
will be passed
the value
as its argument.
bfj.events.number
indicates that
a number
has been encountered.
The listener
will be passed
the value
as its argument.
bfj.events.literal
indicates that
a JSON literal
(either true
, false
or null
)
has been encountered.
The listener
will be passed
the value
as its argument.
bfj.events.error
indicates that
a syntax error
has occurred.
The listener
will be passed
the Error
instance
as its argument.
bfj.events.end
indicates that
the end of the input
has been reached
and the stream is closed.
var emitter = bfj.walk(fs.createReadStream(path));
emitter.on(bfj.events.array, array);
emitter.on(bfj.events.object, object);
emitter.on(bfj.events.property, property);
emitter.on(bfj.events.string, value);
emitter.on(bfj.events.number, value);
emitter.on(bfj.events.literal, value);
emitter.on(bfj.events.endArray, endScope);
emitter.on(bfj.events.endObject, endScope);
emitter.on(bfj.events.error, error);
emitter.on(bfj.events.end, end);
parse
returns a promise
and asynchronously parses
a stream of JSON data.
It takes two arguments; a readable stream from which the JSON will be parsed and an options object that supports the following properties.
options.reviver
:
Transformation function,
invoked depth-first
against the parsed
data structure.
This option
is analagous to the
reviver parameter for JSON.parse.
options.discard
:
The number of characters
to process before
discarding them
to save memory.
The default value
is 16384
.
If there are no syntax errors, the returned promise is resolved with the parsed data. If syntax errors occur, the promise is rejected with the first error.
bfj.parse(fs.createReadStream(path)).
then(function (data) {
// :)
}).
catch(function (error) {
// :(
});
read
returns a promise and
asynchronously parses
a JSON file
read from disk.
It takes two arguments; the path to the JSON file and an options object that supports the following properties.
options.reviver
:
Transformation function,
invoked depth-first
against the parsed
data structure.
This option
is analagous to the
reviver parameter for JSON.parse.
options.discard
:
The number of characters
to process before
discarding them
to save memory.
The default value
is 16384
.
If there are no syntax errors, the returned promise is resolved with the parsed data. If syntax errors occur, the promise is rejected with the first error.
bfj.read(path).
then(function (data) {
// :)
}).
catch(function (error) {
// :(
});
eventify
returns an event emitter
and asynchronously traverses
a data structure depth-first,
emitting events as it
encounters items.
By default it coerces
promises, buffers, dates and iterables
to JSON-friendly values.
It takes two arguments; the data structure to traverse and an options object that supports the following properties.
options.promises
:
By default,
promises are coerced
to their resolved value.
Set this property
to 'ignore'
if you'd prefer
to ignore promises
in the data.
options.buffers
:
By default,
buffers are coerced
using their toString
method.
Set this property
to 'ignore'
if you'd prefer
to ignore buffers
in the data.
options.dates
:
By default,
dates are coerced
using their toJSON
method.
Set this property
to 'ignore'
if you'd prefer
to ignore dates
in the data.
options.maps
:
By default,
maps are coerced
to plain objects.
Set this property
to 'ignore'
if you'd prefer
to ignore maps
in the data.
options.iterables
:
By default,
other iterables
(i.e. not arrays, strings or maps)
are coerced
to arrays.
Set this property
to 'ignore'
if you'd prefer
to ignore other iterables
in the data.
options.circular
:
By default,
circular references
will emit an error.
Set this property
to 'ignore'
if you'd prefer
to silently skip past
circular references
in the data.
The emitted events
are defined
as public properties
of an object,
bfj.events
:
bfj.events.array
indicates that
an array
has been encountered.
bfj.events.endArray
indicates that
the end of an array
has been encountered.
bfj.events.object
indicates that
an object
has been encountered.
bfj.events.endObject
indicates that
the end of an object
has been encountered.
bfj.events.property
indicates that
a property
has been encountered
in an object.
The listener
will be passed
the name of the property
as its argument
and the next event
to be emitted
will represent
the property's value.
bfj.events.string
indicates that
a string
has been encountered.
The listener
will be passed
the value
as its argument.
bfj.events.number
indicates that
a number
has been encountered.
The listener
will be passed
the value
as its argument.
bfj.events.literal
indicates that
a JSON literal
(either true
, false
or null
)
has been encountered.
The listener
will be passed
the value
as its argument.
bfj.events.end
indicates that
the end of the data
has been reached and
no further events
will be emitted.
var emitter = bfj.eventify(data);
emitter.on(bfj.events.array, array);
emitter.on(bfj.events.object, object);
emitter.on(bfj.events.property, property);
emitter.on(bfj.events.string, string);
emitter.on(bfj.events.number, value);
emitter.on(bfj.events.literal, value);
emitter.on(bfj.events.endArray, endArray);
emitter.on(bfj.events.endObject, endObject);
emitter.on(bfj.events.end, end);
streamify
returns a readable stream
and asynchronously serialises
a data structure to JSON,
pushing the result
to the returned stream.
It takes two arguments; the data structure to serialise and an options object that supports the following properties.
options.space
:
Indentation string
or the number of spaces
to indent
each nested level by.
This option
is analagous to the
space parameter for JSON.stringify.
options.promises
:
By default,
promises are coerced
to their resolved value.
Set this property
to 'ignore'
if you'd prefer
to ignore promises
in the data.
options.buffers
:
By default,
buffers are coerced
using their toString
method.
Set this property
to 'ignore'
if you'd prefer
to ignore buffers
in the data.
options.dates
:
By default,
dates are coerced
using their toJSON
method.
Set this property
to 'ignore'
if you'd prefer
to ignore dates
in the data.
options.maps
:
By default,
maps are coerced
to plain objects.
Set this property
to 'ignore'
if you'd prefer
to ignore maps
in the data.
options.iterables
:
By default,
other iterables
(i.e. not arrays, strings or maps)
are coerced
to arrays.
Set this property
to 'ignore'
if you'd prefer
to ignore other iterables
in the data.
options.circular
:
By default,
circular references
will emit
a 'dataError' event
on the returned stream.
Set this property
to 'ignore'
if you'd prefer
to silently skip past
circular references
in the data.
var stream = bfj.streamify(data);
stream.on('data', read);
stream.on('end', end);
stream.on('dataError', error);
stringify
returns a promise and
asynchronously serialises a data structure
to a JSON string.
The promise is resolved
to the JSON string
when serialisation is complete.
It takes two arguments; the data structure to serialise and an options object that supports the following properties.
options.space
:
Indentation string
or the number of spaces
to indent
each nested level by.
This option
is analagous to the
space parameter for JSON.stringify.
options.promises
:
By default,
promises are coerced
to their resolved value.
Set this property
to 'ignore'
if you'd prefer
to ignore promises
in the data.
options.buffers
:
By default,
buffers are coerced
using their toString
method.
Set this property
to 'ignore'
if you'd prefer
to ignore buffers
in the data.
options.dates
:
By default,
dates are coerced
using their toJSON
method.
Set this property
to 'ignore'
if you'd prefer
to ignore dates
in the data.
options.maps
:
By default,
maps are coerced
to plain objects.
Set this property
to 'ignore'
if you'd prefer
to ignore maps
in the data.
options.iterables
:
By default,
other iterables
(i.e. not arrays, strings or maps)
are coerced
to arrays.
Set this property
to 'ignore'
if you'd prefer
to ignore other iterables
in the data.
options.circular
:
By default,
circular references
will reject
the returned promise.
Set this property
to 'ignore'
if you'd prefer
to silently skip past
circular references
in the data.
bfj.stringify(data).
then(function (json) {
// :)
})
.catch(function (error) {
// :(
});
write
returns a promise
and asynchronously serialises a data structure
to a JSON file on disk.
The promise is resolved
when the file has been written,
or rejected with the error
if writing failed.
It takes three arguments; the path to the JSON file, the data structure to serialise and an options object that supports the following properties.
options.space
:
Indentation string
or the number of spaces
to indent
each nested level by.
This option
is analagous to the
space parameter for JSON.stringify.
options.promises
:
By default,
promises are coerced
to their resolved value.
Set this property
to 'ignore'
if you'd prefer
to ignore promises
in the data.
options.buffers
:
By default,
buffers are coerced
using their toString
method.
Set this property
to 'ignore'
if you'd prefer
to ignore buffers
in the data.
options.dates
:
By default,
dates are coerced
using their toJSON
method.
Set this property
to 'ignore'
if you'd prefer
to ignore dates
in the data.
options.maps
:
By default,
maps are coerced
to plain objects.
Set this property
to 'ignore'
if you'd prefer
to ignore maps
in the data.
options.iterables
:
By default,
other iterables
(i.e. not arrays, strings or maps)
are coerced
to arrays.
Set this property
to 'ignore'
if you'd prefer
to ignore other iterables
in the data.
options.circular
:
By default,
circular references
will cause the write
to fail.
Set this property
to 'ignore'
if you'd prefer
to silently skip past
circular references
in the data.
bfj.write(path, data).
then(function () {
// :)
}).
catch(function (error) {
// :(
});
Yes.
The development environment
relies on node.js,
JSHint,
Mocha,
Chai,
Mockery and
Spooks.
Assuming that
you already have
node and NPM
set up,
you just need
to run
npm install
to install
all of the dependencies
as listed in package.json
.
You can
lint the code
with the command
npm run lint
.
You can
run the tests
with the command
npm test
.
0.12 and the latest stable io.js.
MIT.
FAQs
Big-friendly JSON. Asynchronous streaming functions for large JSON data sets.
The npm package bfj receives a total of 2,906,019 weekly downloads. As such, bfj popularity was classified as popular.
We found that bfj demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.