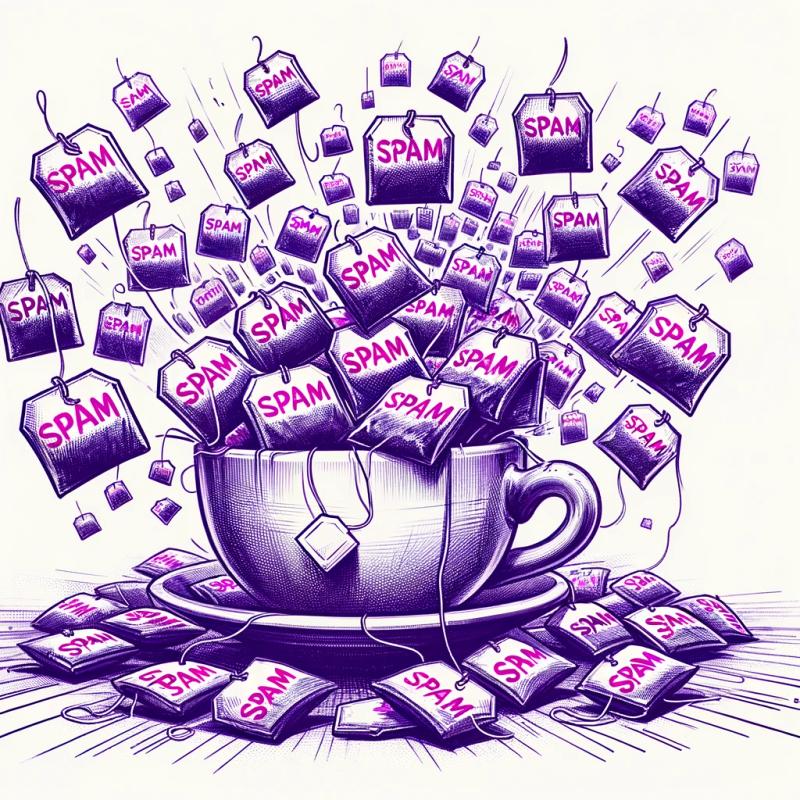
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
binary-extract
Advanced tools
Readme
Extract one or more values from a buffer of json without parsing the whole thing.
var extract = require('binary-extract');
var buf = new Buffer(JSON.stringify({
foo: 'bar',
bar: 'baz',
nested: {
bar: 'nope'
}
}));
var value = extract(buf, 'bar');
// => 'baz'
var values = extract(buf, ['foo', 'nested'])
// => ["bar", {"bar":"nope"}]
With the object from bench.js
, extract()
is ~2-4x faster than
JSON.parse(buf.toString())
. It is also way more memory efficient as the
blob stays out of the V8 heap.
The big perf gain comes mainly from not parsing everything and not converting the buffer to a string.
$ npm install binary-extract
Extract the value of keys
in the json buf
.
The value can be any valid JSON structure.
If keys
is a String, returns a value. If keys
is an Array of
keys, returns an array of values.
MIT
FAQs
Extract values from a binary json blob
The npm package binary-extract receives a total of 15 weekly downloads. As such, binary-extract popularity was classified as not popular.
We found that binary-extract demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.