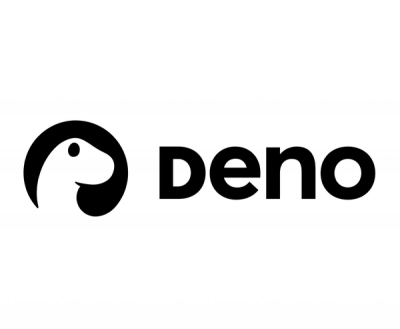
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
bionode-vcf
Advanced tools
a vcf parser in javascript
You need to install the latest Node.JS first, please check nodejs.org or do the following:
# Ubuntu
sudo apt-get install npm
# Mac
brew install node
# Both
npm install -g n
n stable
To use bionode-vcf
as a command line tool, you can install it globally with -g
.
npm install bionode-vcf -g
Or, if you want to use it as a JavaScript library, you need to install it in your local project folder inside the node_modules
directory by doing the same command without -g
.
npm i bionode-vcf # 'i' can be used as shortcut to 'install'
vcf.read
takes params: path
vcf
, zip
and gz
.var vcf = require('bionode-vcf');
vcf.read("/path/sample.vcf");
vcf.on('data', function(feature){
console.log(feature);
})
vcf.on('end', function(){
console.log('end of file')
})
vcf.on('error', function(err){
console.error('it\'s not a vcf', err)
})
vcf.readStream
takes params: stream
and extension
vcf
, zip
and gz
.var vcf = require('bionode-vcf');
var fileStream = s3.getObject({
Bucket: [BUCKETNAME],
Key: [FILENAME]
}).createReadStream(); // or stream data from any other source
vcf.read(filestream, 'zip'); // default value is `vcf`
vcf.on('data', function(feature){
console.log(feature);
})
vcf.on('end', function(){
console.log('end of file')
})
vcf.on('error', function(err){
console.error('it\'s not a vcf', err)
})
VCF format specifications and more information about the fileds can be found at 1000 genomes webpage and samtools github page
We welcome all kinds of contributions at all levels of experience, please read the CONTRIBUTING.md to get started!
FAQs
a vcf parser in javascript
The npm package bionode-vcf receives a total of 3 weekly downloads. As such, bionode-vcf popularity was classified as not popular.
We found that bionode-vcf demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.