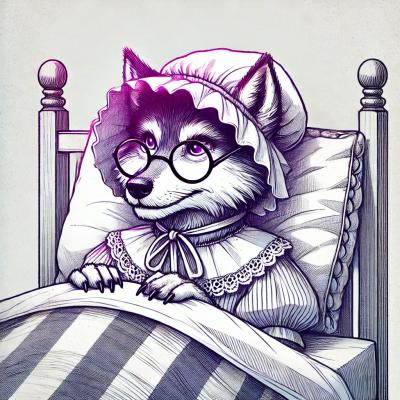
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
[](https://travis-ci.org/bitcoinjs/bip174) [](https://www.npmjs.org/package/bip174)
The bip174 npm package is a library for handling Partially Signed Bitcoin Transactions (PSBT) as defined by BIP 174. It provides tools to create, modify, and finalize PSBTs, which are useful for multi-party transaction signing and other advanced Bitcoin transaction workflows.
Create a PSBT
This feature allows you to create a new PSBT by adding inputs and outputs. The code sample demonstrates how to initialize a PSBT, add an input with a transaction hash and index, and add an output with a recipient address and value.
const { Psbt } = require('bip174');
const psbt = new Psbt();
psbt.addInput({
hash: 'transactionHash',
index: 0,
nonWitnessUtxo: Buffer.from('hexString', 'hex')
});
psbt.addOutput({
address: 'recipientAddress',
value: 1000
});
console.log(psbt.toBase64());
Sign a PSBT
This feature allows you to sign a PSBT. The code sample demonstrates how to load a PSBT from a base64 string, create a key pair, and sign the first input of the PSBT.
const { Psbt } = require('bip174');
const psbt = Psbt.fromBase64('base64PsbtString');
const keyPair = { publicKey: Buffer.from('publicKeyHex', 'hex'), privateKey: Buffer.from('privateKeyHex', 'hex') };
psbt.signInput(0, keyPair);
console.log(psbt.toBase64());
Finalize a PSBT
This feature allows you to finalize a PSBT and extract the final transaction. The code sample demonstrates how to load a PSBT from a base64 string, finalize all inputs, and extract the final transaction in hexadecimal format.
const { Psbt } = require('bip174');
const psbt = Psbt.fromBase64('base64PsbtString');
psbt.finalizeAllInputs();
const tx = psbt.extractTransaction();
console.log(tx.toHex());
The bitcoinjs-lib package is a comprehensive library for Bitcoin-related operations, including transaction creation, signing, and verification. It provides more general functionality compared to bip174, which is specifically focused on PSBT handling.
The bcoin package is a full Bitcoin node implementation written in JavaScript. It includes extensive functionality for transaction creation, signing, and network communication. While it supports PSBT, it is a more heavyweight solution compared to the focused bip174 package.
The bitcore-lib package is a library for Bitcoin and blockchain-related operations. It provides tools for creating and signing transactions, as well as interacting with the Bitcoin network. It offers broader functionality than bip174, which is specialized for PSBT.
A BIP174 compatible partial Transaction encoding library.
TODO: Increase coverage. Squish bugs.
WARNING: Until v1.0.0 release, this library will be in flux and have many bugs. Be warned.
combine
has highest priority.In order to keep this library as separate from Bitcoin logic as possible, This library will not implement the following responsibilities. But rather, down the road bitcoinjs-lib will adopt this class and extend it internally to allow for the following:
const { Psbt } = require('bip174')
const psbt = new Psbt()
psbt.addInput({
hash: '865dce988413971fd812d0e81a3395ed916a87ea533e1a16c0f4e15df96fa7d4',
index: 3,
})
psbt.addInput({
hash: 'ff5dce988413971fd812d0e81a3395ed916a87ea533e1a16c0f4e15df96fa7d4',
index: 1,
})
psbt.addOutput({
script: Buffer.from(
'a914e18870f2c297fbfca54c5c6f645c7745a5b66eda87',
'hex',
),
value: 1234567890,
})
psbt.addOutput({
script: Buffer.from(
'a914e18870f2c297fbfca54c5c6f645c7745a5b66eda87',
'hex',
),
value: 987654321,
})
psbt.addRedeemScriptToInput(0, Buffer.from(
'00208c2353173743b595dfb4a07b72ba8e42e3797da74e87fe7d9d7497e3b2028903',
'hex',
))
psbt.addWitnessScriptToInput(0, Buffer.from(
'522103089dc10c7ac6db54f91329af617333db388cead0c231f723379d1b9903' +
'0b02dc21023add904f3d6dcf59ddb906b0dee23529b7ffb9ed50e5e861519268' +
'60221f0e7352ae',
'hex',
))
psbt.addBip32DerivationToInput(0, {
masterFingerprint: Buffer.from('d90c6a4f', 'hex'),
pubkey: Buffer.from(
'023add904f3d6dcf59ddb906b0dee23529b7ffb9ed50e5e86151926860221f0e73',
'hex',
),
path: "m/0'/0'/3'",
})
psbt.addBip32DerivationToInput(0, {
masterFingerprint: Buffer.from('d90c6a4f', 'hex'),
pubkey: Buffer.from(
'03089dc10c7ac6db54f91329af617333db388cead0c231f723379d1b99030b02dc',
'hex',
),
path: "m/0'/0'/2'",
})
const b64 = psbt.toBase64();
FAQs
[](https://github.com/bitcoinjs/bip174/actions/workflows/main_ci.yml) [](https://www.npmjs.org/package/bip174)
The npm package bip174 receives a total of 90,743 weekly downloads. As such, bip174 popularity was classified as popular.
We found that bip174 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.