Comparing version 0.0.15 to 0.1.0
{ | ||
"name": "bleat", | ||
"description": "Abstraction library for hiding differences in JavaScript BLE APIs", | ||
"description": "Abstraction library following Web Bluetooth specification for hiding differences in JavaScript BLE APIs", | ||
"homepage": "https://github.com/thegecko/bleat", | ||
@@ -12,2 +12,3 @@ "authors": "Rob Moran <rob@thegecko.org>", | ||
"keywords": [ | ||
"web-bluetooth", | ||
"bleat", | ||
@@ -14,0 +15,0 @@ "ble", |
var gulp = require('gulp'); | ||
var jshint = require('gulp-jshint'); | ||
var concat = require('gulp-concat'); | ||
var uglify = require('gulp-uglifyjs'); | ||
var apis = ['classic', 'web-bluetooth']; | ||
var adapters = ['noble', 'evothings']; | ||
gulp.task('lint', function() { | ||
return gulp.src(['dist/*.js', '!dist/*.min.js', '!dist/bleat.js']) | ||
return gulp.src(['dist/*.js', '!dist/*.min.js']) | ||
.pipe(jshint()) | ||
@@ -13,6 +15,16 @@ .pipe(jshint.reporter('default')); | ||
gulp.task('release', function() { | ||
return gulp.src(['dist/bleat.core.js', 'dist/bleat.*.js', '!dist/*.min.js']) | ||
.pipe(concat('bleat.js')) | ||
.pipe(gulp.dest('dist')) | ||
.pipe(uglify('bleat.min.js', { | ||
apis.forEach(api => { | ||
adapters.forEach(adapter => { | ||
minify(api, adapter); | ||
}); | ||
}); | ||
}); | ||
function minify(api, adapter) { | ||
return gulp.src([ | ||
'dist/bluetooth.helpers.js', | ||
'dist/api.' + api + '.js', | ||
'dist/adapter.' + adapter + '.js' | ||
]) | ||
.pipe(uglify(api + '.' + adapter + '.min.js', { | ||
output: { | ||
@@ -23,4 +35,4 @@ comments: /@license/ | ||
.pipe(gulp.dest('dist')); | ||
}); | ||
} | ||
gulp.task('default', ['lint', 'release']); |
19
index.js
@@ -1,6 +0,13 @@ | ||
var bleat = require('./dist/bleat.core'); | ||
require('./dist/bleat.chromeos'); | ||
require('./dist/bleat.evothings'); | ||
require('./dist/bleat.noble'); | ||
module.exports = bleat; | ||
module.exports = { | ||
helpers: require('./dist/bluetooth.helpers'), | ||
get classic() { | ||
var bluetooth = require('./dist/api.classic'); | ||
require('./dist/adapter.noble')(bluetooth); | ||
return bluetooth; | ||
}, | ||
get webbluetooth() { | ||
var bluetooth = require('./dist/api.web-bluetooth'); | ||
require('./dist/adapter.noble')(bluetooth); | ||
return bluetooth; | ||
} | ||
}; |
{ | ||
"name": "bleat", | ||
"version": "0.0.15", | ||
"description": "Abstraction library for hiding differences in JavaScript BLE APIs", | ||
"version": "0.1.0", | ||
"description": "Abstraction library following Web Bluetooth specification for hiding differences in JavaScript BLE APIs", | ||
"homepage": "https://github.com/thegecko/bleat", | ||
@@ -14,2 +14,3 @@ "author": "Rob Moran <rob@thegecko.org>", | ||
"keywords": [ | ||
"web-bluetooth", | ||
"bleat", | ||
@@ -20,11 +21,13 @@ "ble", | ||
], | ||
"engines": { | ||
"node": ">=4.0.0" | ||
}, | ||
"optionalDependencies": { | ||
"noble": "^1.1.0" | ||
"noble": "^1.3.0" | ||
}, | ||
"devDependencies": { | ||
"gulp": "^3.8.7", | ||
"gulp": "^3.9.1", | ||
"gulp-jshint": "^1.9.0", | ||
"gulp-concat": "^2.6.0", | ||
"gulp-uglifyjs": "^0.5.0" | ||
} | ||
} |
291
README.md
@@ -1,2 +0,3 @@ | ||
# bleat | ||
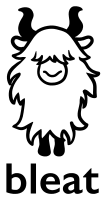 | ||
___ | ||
@@ -8,274 +9,92 @@ [](https://circleci.com/gh/thegecko/bleat) | ||
Bleat (Blutooth Low Energy Abstraction Tool) provides a simplified BLE layer which uses adapters to abstract the usage of BLE in JavaScript on different platforms. | ||
Bleat (Bluetooth Low Energy Abstraction Tool) provides a simplified BLE layer which uses adapters to abstract the usage of BLE in JavaScript on different platforms. | ||
## Implemented | ||
## APIs | ||
* Central Mode | ||
* Device Scan | ||
* Advertised Service UUIDs | ||
* Connect/Disconnect | ||
* List Services/Characteristics/Descriptors | ||
* Read/Write/Notify Characteristics | ||
* Read/Write Descriptors | ||
Bleat has 2 APIs: | ||
* Central Adapters | ||
* Android/iOS (using Evothings/Cordova/PhoneGap) https://github.com/evothings/cordova-ble/blob/master/ble.js | ||
* Mac/Linux (using noble on Node.js) https://github.com/sandeepmistry/noble | ||
* ChromeOS https://developer.chrome.com/apps/bluetoothLowEnergy | ||
* The [Classic API](api_classic.md), originally developed for this project | ||
* The [Extended Web Bluetooth API](api_web-bluetooth.md) specification developed for use in browsers | ||
## Roadmap (to be implemented) | ||
* Central Adapters | ||
* Web (following Web Bluetooth) https://www.w3.org/community/web-bluetooth | ||
* Peripheral Mode | ||
* Advertising | ||
* Peripheral Adapters | ||
* Mac/Linux (using bleno on Node.js) https://github.com/sandeepmistry/bleno | ||
* Tessel https://tessel.io/modules#module-ble | ||
* Espruino http://www.espruino.com/Bluetooth | ||
## Installation | ||
``` | ||
npm install bleat | ||
-or- | ||
bower install bleat | ||
``` | ||
To install the library, either clone or copy the files to your project or use a package manager: | ||
## Usage | ||
The main `bleat.core.js` file offers the BLE layer while each `bleat.<platform>.js` file represents an adapter. | ||
The `bleat.js` file contains the BLE layer and all adapters concatenated together (not minified). | ||
The `bleat.min.js` file is a minified version of the BLE layer and all adapters. | ||
Files follow the UMD (https://github.com/umdjs/umd), so should work with AMD (requirejs), CommonJS (node) and plain JavaScript projects. | ||
Refer to the `example_<adapter>` files for simple examples. | ||
### Plain JavaScript (globals) | ||
Include (or require) the `bleat.core.js` file before the adapter you wish to use or include the minified file offering all adapters. | ||
e.g. | ||
``` | ||
<script src="path/to/bleat.core.js"></script> | ||
<script src="path/to/bleat.<adapter>.js"></script> | ||
-or- | ||
<script src="path/to/bleat.min.js"></script> | ||
$ npm install bleat | ||
``` | ||
### Node.js | ||
Simply require it up! | ||
-or- | ||
``` | ||
var bleat = require('bleat'); | ||
$ bower install bleat | ||
``` | ||
## API | ||
## Usage | ||
### bleat | ||
The ```bluetooth.helpers.js``` file contains general helper objects and functions. | ||
#### bleat.init | ||
The ```api.<api>.js``` files each offer a BLE API. | ||
Initialise bleat. | ||
The ```adapter.<platform>.js``` files each represent an adapter targetting a specific BLE engine, the ```adapter-template.js``` file is an empty template to ease creation of a new adapter. | ||
``` | ||
void bleat.init([readyFn], [errorFn], [adapterName]); | ||
``` | ||
The ```<api>.<adapter>.min.js``` files are minified versions of the BLE APIs including the helpers and a single adapter. | ||
function readyFn(): callback function once init has completed | ||
Files follow the [UMDJS](https://github.com/umdjs/umd) specification, so should work with CommonJS (such as [node.js](https://nodejs.org/)), AMD (such as [RequireJS](http://requirejs.org/)) and plain JavaScript projects. | ||
function errorFn(message): callback function for all errors while bleating | ||
Refer to the ```example_<adapter>_<api>``` files and folders for simple examples of how to use each combination. | ||
string adapterName: when multiple adapters available, specify which to use | ||
### CommonJS (node.js) | ||
#### bleat.startScan | ||
Simply require it up, specifying the api you wish to use: | ||
Start scanning for devices. | ||
```node | ||
var bleat = require('bleat').classic; | ||
``` | ||
void bleat.startScan(foundFn); | ||
-or- | ||
```node | ||
var bleat = require('bleat').webbluetooth; | ||
``` | ||
function foundFn(device): callback function for each device discovered. | ||
### AMD (RequireJS) | ||
#### bleat.stopScan | ||
To use bleat with [RequireJS](http://requirejs.org/), set the ```requirejs.config``` to load your API of choice as ```bleat``` and add a dependency on your adapter of choice: | ||
Stop scanning for devices. | ||
```js | ||
requirejs.config({ | ||
baseUrl: 'path/to/bleat', | ||
paths: { | ||
bleat: 'api.classic', | ||
adapter: 'adapter.evothings' | ||
}, | ||
shim: { | ||
bleat: { | ||
deps: ['adapter'] | ||
} | ||
} | ||
}); | ||
``` | ||
void bleat.stopScan(); | ||
``` | ||
### device | ||
You can then use bleat as follows: | ||
string device.address: uuid of device | ||
string device.name: name of device | ||
bool device.connected: whether device is connected | ||
string array device.serviceUUIDs: array of advertised services | ||
object services: map of device services keyed on service uuid (available once connected) | ||
#### device.hasService | ||
Returns whether a device has the specified service. | ||
```js | ||
require(['bleat'], function(bleat) { | ||
// Use bleat here | ||
}); | ||
``` | ||
bool device.hasService(serviceUUID); | ||
``` | ||
string serviceUUID: service to search for | ||
### Plain JavaScript (globals) | ||
#### device.connect | ||
Include the ```bluetooth.helpers.js``` file, then the bleat api file you wish to use and finally the adapter file you wish to use: | ||
Connect to the device. | ||
```html | ||
<script src="path/to/bluetooth.helpers.js"></script> | ||
<script src="path/to/api.classic.js"></script> | ||
<script src="path/to/adapter.evothings.js"></script> | ||
``` | ||
void device.connect(connectFn, [disconnectFn], [suppressDiscovery]); | ||
``` | ||
function connectFn(): callback once connected | ||
Alternatively, you can just include the minified file which contains the API and adapter you wish to use: | ||
function disconnectFn(): callback when disconnected | ||
bool suppressDiscovery: don't undertake automatic discovery of services, characteristics and descriptors | ||
#### device.disconnect | ||
Disconnect from device. | ||
```html | ||
<script src="path/to/web-bluetooth.evothings.min.js"></script> | ||
``` | ||
void device.disconnect(); | ||
``` | ||
#### device.discoverServices | ||
Discover all services for this device | ||
``` | ||
void device.discoverServices(callbackFn) | ||
``` | ||
function callbackFn(): callback once discovery complete | ||
#### device.discoverAll | ||
Discover all services, characteristics and descriptors for this device | ||
``` | ||
void device.discoverAll(callbackFn) | ||
``` | ||
function callbackFn(): callback once discovery complete | ||
### Service | ||
string uuid: uuid of service | ||
bool primary: whether service is primary or not | ||
object characteristics: map of service characteristics keyed on characteristic uuid | ||
#### service.discoverCharacteristics | ||
Discover all characteristics for this service | ||
``` | ||
void service.discoverCharacteristics(callbackFn) | ||
``` | ||
function callbackFn(): callback once discovery complete | ||
### Characteristic | ||
string uuid: uuid of characteristic | ||
string array properties: characteristic properties | ||
object descriptors: map of characteristic descriptors keyed on descriptor uuid | ||
#### characteristic.read | ||
Read value of characteristic. | ||
``` | ||
void characteristic.read(completeFn); | ||
``` | ||
function completeFn(ArrayBuffer): callback function containing value | ||
#### characteristic.write | ||
Write value to characteristic. | ||
``` | ||
void characteristic.write(bufferView, completeFn); | ||
``` | ||
DataView bufferView: value to write | ||
function completeFn(): callback function once completed | ||
#### characteristic.enableNotify | ||
Enable notifications when characteristic value changes. | ||
``` | ||
void characteristic.enableNotify(notifyFn, completeFn); | ||
``` | ||
function notifyFn(ArrayBuffer): callback function containing value when changes | ||
function completeFn(): callback function once completed | ||
#### characteristic.disableNotify | ||
Disable characteristic notifications. | ||
``` | ||
void characteristic.disableNotify(completeFn); | ||
``` | ||
function completeFn(): callback function once completed | ||
#### characteristic.discoverDescriptors | ||
Discover all descriptors for this characteristic | ||
``` | ||
void characteristic.discoverDescriptors(callbackFn) | ||
``` | ||
function callbackFn(): callback once discovery complete | ||
### Descriptor | ||
string uuid: uuid of descriptor | ||
#### descriptor.read | ||
Read value of descriptor. | ||
``` | ||
void descriptor.read(completeFn); | ||
``` | ||
function completeFn(ArrayBuffer): callback function containing value | ||
#### descriptor.write | ||
Write value to descriptor. | ||
``` | ||
void descriptor.write(bufferView, completeFn); | ||
``` | ||
DataView bufferView: value to write | ||
function completeFn(): callback function once completed | ||
A global object ```root.bleat``` will then be available to use. |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Dynamic require
Supply chain riskDynamic require can indicate the package is performing dangerous or unsafe dynamic code execution.
Found 1 instance in 1 package
Minified code
QualityThis package contains minified code. This may be harmless in some cases where minified code is included in packaged libraries, however packages on npm should not minify code.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Dynamic require
Supply chain riskDynamic require can indicate the package is performing dangerous or unsafe dynamic code execution.
Found 1 instance in 1 package
Minified code
QualityThis package contains minified code. This may be harmless in some cases where minified code is included in packaged libraries, however packages on npm should not minify code.
Found 1 instance in 1 package
225073
3
29
3113
6
100
2