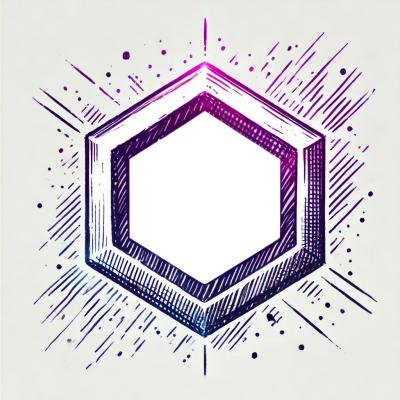
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
blockbid-message
Advanced tools
This library makes it easy to send messages in a distributed network transparent way via various brokers but initially via RabbitMQ.
This library makes it easy to send messages in a distributed network transparent way via various brokers but initially via RabbitMQ.
At a later point we should have plugins to make it work with various messaging paradigms:
blockbid-messages/amqp
requires node. blockbid-messages/socketio-browser
may require browser objects. (YTBI)blockbid-tools
and ensure it supports versioningYou can install by referencing a version tag directly off the github repo.
yarn add blockbid/blockbid-message#<semverish>
import { createConsumer, createProducer } from 'blockbid-message';
import { filter } from 'rxjs/opeerators';
// createProducer accepts a list of middleware
// the message passes top down
// It returns an RxJS Observer that sends messages
const producer = createProducer(
transformMessageSomehow,
broadCastsMessagesSomewhere
);
// createConsumer also accepts a list of middleware
// the message also passes top down
// It returns an RxJS Observable that will receive the message
const consumer = createConsumer(
receivesMessagesFromSomewhere,
logOrTransformMessage,
doSomeMoreTransformation
);
// Use RxJS's Observable#next() method to send a message
producer.next({
content: 'Hello World!',
route: 'hello'
});
// Note that because consumer is simply an RxJS observable
// you can apply filtering and throttling or do whatever you want to it
const sub = consumer
.pipe(filter(msg => msg.content.toLowerCase().includes('world')))
.subscribe(msg => {
console.log(`Received: ${msg.content}`);
});
Generic message objects look like this:
// Generic message
export interface IMessage {
content: any;
route?: any;
}
You might use a message by sending it to the next()
method of a producer.
producer.next({
content: 'Hi there!',
route: 'some-queue'
});
Middleware are effectively functions designed to decorate RxJS streams and looks like this:
// Generic Middleware decorates a stream
export type Middleware<T extends IMessage> = (
a: Observable<T>
) => Observable<T>;
You might use a middleware by passing it as one of the arguments to the createProducer()
or createConsumer()
functions
import {tap} from 'rxjs/operators';
function logger(stream: Observable<IMessage>) {
return stream
.pipe(tap(
(msg:IMessage) => console.log(`Stream logged: ${msg.content}`
));
}
// Pass the middleware in order to the consumer or producer
const consumer = createConsumer(someReceiver, logger);
AMQP Middleware is designed to work in Node environments only due to limitations with the amqplib package it is based on.
import { createConsumer, createProducer } from 'blockbid-message';
import { createAmqpConnector } from 'blockbid-message/amqp';
const { sender, receiver } = createAmqpConnector({
declarations: {
// This declares the queue you want to use
queues: [
{
durable: false,
name: 'hello'
}
]
},
uri: 'amqp://user:password@somerabbitserver.io/user'
});
// Here is an RxJS Observer that sends the message
const producer = createProducer(sender());
producer.next({
content: 'Hello World!',
route: 'hello'
});
// Here is an RxJS Observable that will receive the message
const consumer = createConsumer(
receiver({
noAck: true,
queue: 'hello'
})
);
const sub = consumer.subscribe(msg => {
console.log(`Received: ${msg.content}`);
});
sub.unsubscribe();
For usage and examples please look at the basic tests thrown together here
blockbid-message
uses RxJS v6.0 so you need to pipe all your operators:
import { filter } from 'rxjs/operators';
// ...
consumer.pipe(filter(forUserEvents(userId))).subscribe(
msg => {
dealWithMessage(msg.content);
},
() => {}
);
FAQs
This library makes it easy to send messages in a distributed network transparent way via various brokers but initially via RabbitMQ.
The npm package blockbid-message receives a total of 0 weekly downloads. As such, blockbid-message popularity was classified as not popular.
We found that blockbid-message demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.