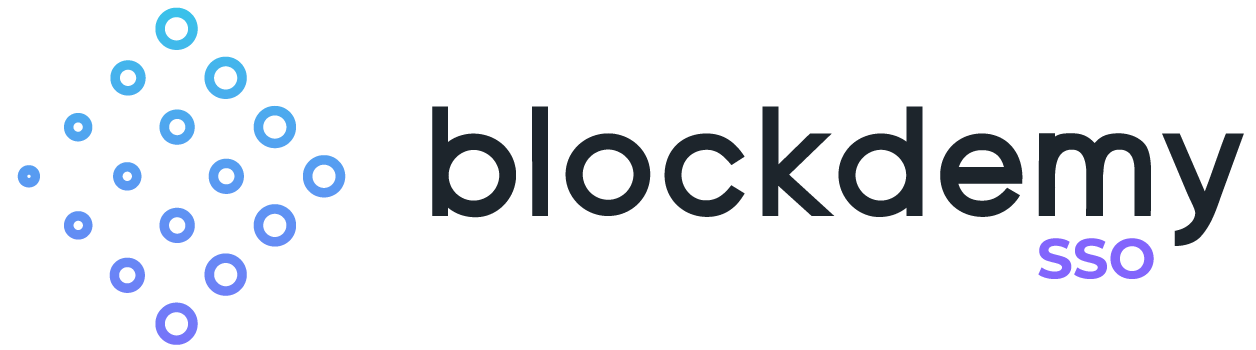
Blockdemy SSO
Official Blockdemy's Single Sign On Client
Blockdemy SSO is a client that allows to communicate and get user data from our Single Sign On Service
Documentation
Getting started
Run the following command to install Blockdemy SSO client
$ yarn add blockdemy-sso
Blockdemy SSO Client
In order to start using the sso client, it is needed to be instantiated using the following code
import BlockdemySSO from 'blockdemy-sso'
const SSO_URL = 'https://api.id.blockdemy.com'
const API_KEY = '<API_KEY>'
const SingleSignOn = new BlockdemySSO(API_KEY, SSO_URL);
const start = async () => {
const user = await SingleSignOn.getUserById();
}
Using the Mongoose Plugin
In order to use the mongoose plugin to autopopulate users using their ssoId, you just need to add the following into your user schema:
import SingleSignOn from 'path/to/your/blockdemy-sso/instance';
const UserSchema = new Schema({
ssoId: { type: Schema.Types.ObjectId }
});
UserSchema.plugin(SingleSignOn.populateUsers);
Query user data methods
Every method corresponds with the GraphQL API query endpoint with the same name
They can be checked at our Blockdemy SSO Playground
Method | params | returns | Description |
---|
usersById(ids) | ids: Array[String] | Array[Object] | Recover the list of users given valid ids |
user(id) | id: String | Object | Recover user given a valid id |
userFromToken(token) | token: String | Object | Recover user given a jwt token |
userByUsername(username) | username: String | Object | Recover user given a valid username |
userByAddress(address) | address: String | Object | Retrieve the user that owns an ethereum address |
usernameExists(username) | username: String | Boolean | Indicates if an username has been taken |
userEmailExists(email) | email: String | Boolean | Indicates if an email has been taken and verified |
userHasEthAddress(userId, address) | userId: String, address: String | Boolean | Indicates if an specific user owns an ethereum address |
userEthAddressExists(address) | address: String | Boolean | Indicates if an ethereum address has been taken before |
userSearch(query, filters, params) | query: Object, filters: Object, params: Object | Array[Object] | Recover a list of users given valid filters and parameters for search |
organization(id) | id: String | Object | Recover organization given a valid id |
organizationByIdentifier(identifier) | identifier: String | Object | Recover organization given a valid identifier |
organizationsByIds(ids) | ids: Array[String] | Array[Object] | Recover the list of organizations given valid ids |
Mutate user data methods
Every method corresponds with the GraphQL API mutation endpoint with the same name
They can be checked at our
Blockdemy SSO Playground
Method | params | returns | Description |
---|
userEdit(userId, user) | userId: String, user: Object | Object | Edit user data and retrieves the new user |
userAddEthAddress(userId, user) | userId: String, address: String, signature: String | Object | Add ethereum address validated with a signature to user |
userEditPassword(userId, newPassword, oldPassword) | userId: String, newPassword: String, oldPassword: String | Object | Change password tu user using its last password to validate |
Contributing
Blockdemy SSO is open for contributions in order to make it easy to make our technology more open for the community.
Authors
- Ernesto García - Tech Leader @blockdemy
License
This project is licensed under the MIT License