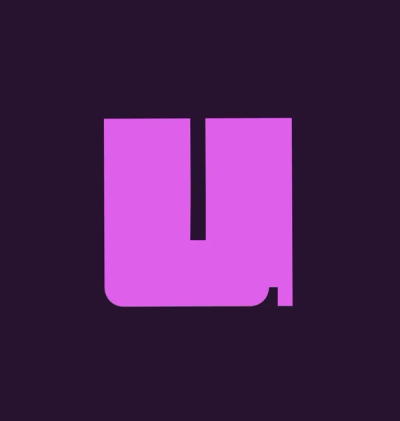
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
boar-tasks-server
Advanced tools
This repository contains Gulp-based tasks to make server-side applications easier. It can be used with any server side framework.
These tasks are helpers, you have to define your build tasks in your project's gulpfile.js
. You can find examples in our existing services or workshop material.
Usually we create a tasks.config.js
file which is for override the default task settings.
// tasks.config.js
module.exports = {
server: {
environmentVariables: {
DEBUG: 'suite-sdk,suiterequest',
PORT: 9100,
BASE_URL: 'http://localhost:9100',
NODE_ENV: 'development'
}
}
};
// gulpfile.js
let gulp = require('gulp');
let runSequence = require('run-sequence');
let config = require('./tasks.config');
let tasks = require('boar-tasks-server').getTasks(gulp, config);
gulp.task('start', function(cb) {
runSequence(['server', 'server-watch'], cb);
});
gulp.task('test', tasks.server.test);
gulp.task('server', tasks.server.start);
gulp.task('server-jshint', function() { return tasks.server.jshint(); });
gulp.task('server-watch', function() { gulp.watch(tasks.config.server.filePattern) });
Run a server with Nodemon for development purposes. It automatically restarts the server if any file changed and notifies the developer about it.
Default configuration
const path = require('path');
const appRootPath = path.join(process.cwd(), 'server', 'processes', 'web');
Config.server = {
path: 'server/',
runnable: path.join(appRootPath, 'index.js'),
filePattern: ['server/**/!(*.spec).{pug,js}', 'package.json'],
watchPattern: 'server/**/*.js',
environmentVariables: {
NODE_ENV: process.env.NODE_ENV || 'development',
APP_ROOT_PATH: appRootPath,
IP: process.env.IP || undefined,
PORT: process.env.PORT || 9100,
BASE_URL: process.env.BASE_URL || 'http://localhost:9100'
}
};
Usage
gulp.task('server', tasks.server.start);
Docker
If you'd like to run your server in Docker and restart on file changes you have to set the NODEMON_LEGACY_WATCH
environment variable to true
. It forces Nodemon to use legacy change detection mode which is the only way to support Docker.
Run all the tests found (all *.spec.js
files) in the codebase.
Default configuration
Config.server = {
path: 'server/',
test: {
requires: ['co-mocha'],
flags: ['reporter dot', 'colors'],
environmentVariables: {
NODE_ENV: process.env.NODE_ENV || 'test',
APP_ROOT_PATH: process.cwd() + '/server/'
}
}
};
Usage
gulp.task('test', tasks.server.test);
Run the specified command by spawning a child process. It sets the server environment variables from the configuration also for the child process.
Default configuration
Config.server = {
environmentVariables: {
PORT: process.env.PORT || 9100,
BASE_URL: process.env.BASE_URL || 'http://localhost:9100'
}
};
Usage
// Creating task for a job-runner
gulp.task('job-runner', function (cb) { return tasks.server.runCommand('server/processes/job-runner', cb) });
Run the specified command by spawning a child process. It sets the test environment variables from the configuration also for the child process.
Default configuration
Config.server = {
test: {
environmentVariables: {
NODE_ENV: process.env.NODE_ENV || 'test',
APP_ROOT_PATH: process.cwd() + '/server/processes/web/'
}
},
};
Usage
// Creating task for a job-runner
gulp.task('job-runner', function (cb) { return tasks.server.runTestCommand('server/processes/job-runner', cb) });
Run the specified command by spawning a child process. It sets the given environment variables also for the child process.
Usage
// Creating task for a job-runner
gulp.task('job-runner', function (cb) { return tasks.server.runEnvironmentCommand('server/processes/job-runner', { NODE_ENV: 'integration' }, cb) });
Check code style using ESLint on the selected JavaScript files.
Default configuration
Config.server = {
codeStylePattern: 'server/**/*.js'
}
Usage
gulp.task('server-codestyle', tasks.server.codeStyle);
Check code style on the selected template files using pug-lint.
Default configuration
Config.server = {
app: {
templateCodeStylePattern: 'server/app/**/*.pug'
}
}
Code style rules
Install pug-lint-config-emarsys
to your project and create a file in your project's root called .pug-lintrc
with the following content:
{
"extends": "emarsys"
}
Usage
gulp.task('server-template-code-style', tasks.server.templateCodeStyle);
FAQs
Boar Tasks for server side
The npm package boar-tasks-server receives a total of 15 weekly downloads. As such, boar-tasks-server popularity was classified as not popular.
We found that boar-tasks-server demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.