Comparing version 0.0.5 to 0.0.7
85
bows.js
@@ -1,75 +0,29 @@ | ||
// Generated by CoffeeScript 1.6.3 | ||
(function() { | ||
var bows, colors, id, logger, nColors, numKeys, prefixes, updateArray, updateArrays, | ||
__slice = [].slice; | ||
var logger = require('andlog'), | ||
goldenRatio = 0.618033988749895, | ||
hue = 0, | ||
padLength = 15, | ||
yieldColor, | ||
bows; | ||
logger = require('andlog'); | ||
nColors = function(n) { | ||
var i, r, x, _i; | ||
i = 360 / n; | ||
r = []; | ||
for (x = _i = 0; 0 <= n ? _i <= n : _i >= n; x = 0 <= n ? ++_i : --_i) { | ||
r.push(i * x); | ||
} | ||
return r; | ||
yieldColor = function() { | ||
hue += goldenRatio; | ||
hue = hue % 1; | ||
return hue * 360; | ||
}; | ||
colors = []; | ||
bows = function(str) { | ||
var msg; | ||
msg = "%c" + (str.slice(0, padLength)); | ||
msg += Array(padLength + 3 - msg.length).join(' ') + '|'; | ||
id = 0; | ||
return logger.log.bind(logger, msg, "color: hsl(" + (yieldColor()) + ",99%,40%); font-weight: bold"); | ||
}; | ||
numKeys = 0; | ||
prefixes = {}; | ||
updateArrays = function() { | ||
var array, key, lengths, maxLength, _results; | ||
colors = nColors(numKeys); | ||
lengths = Object.keys(prefixes).map(function(p) { | ||
return p.length; | ||
}); | ||
maxLength = Math.max.apply({}, lengths); | ||
_results = []; | ||
for (key in prefixes) { | ||
array = prefixes[key]; | ||
_results.push(updateArray(key, maxLength)); | ||
bows.config = function(config) { | ||
if (config.padLength) { | ||
return padLength = config.padLength; | ||
} | ||
return _results; | ||
}; | ||
updateArray = function(fullkey, padlength) { | ||
var key, msg, _ref; | ||
_ref = fullkey.split('-'), key = _ref[0], id = _ref[1]; | ||
msg = "%c" + key; | ||
msg += Array(padlength + 2 - msg.length).join(' ') + '|'; | ||
prefixes[fullkey][0] = msg; | ||
return prefixes[fullkey][1] = "color: hsl(" + colors[id] + ",50%,50%); font-weight: bold"; | ||
}; | ||
bows = function(str) { | ||
var fullkey, log; | ||
numKeys += 1; | ||
fullkey = "" + str + "-" + id; | ||
prefixes[fullkey] = ['', '']; | ||
updateArrays(); | ||
id++; | ||
log = logger.log.apply.bind(logger.log, logger); | ||
log.wrap = function() { | ||
var args; | ||
args = 1 <= arguments.length ? __slice.call(arguments, 0) : []; | ||
if (bows.color) { | ||
return prefixes[fullkey].concat(args); | ||
} else { | ||
return args; | ||
} | ||
}; | ||
log.w = log.wrap; | ||
return log; | ||
}; | ||
bows.color = true; | ||
bows.log = logger.log.apply.bind(logger.log, logger); | ||
if (typeof module !== 'undefined') { | ||
@@ -80,3 +34,2 @@ module.exports = bows; | ||
} | ||
}).call(this); |
{ | ||
"name": "bows", | ||
"version": "0.0.5", | ||
"version": "0.0.7", | ||
"description": "Rainbowed console logs for chrome in development", | ||
@@ -14,7 +14,7 @@ "main": "bows.js", | ||
"browserify": "", | ||
"coffeeify": "" | ||
"uglify-js": "~2.3.6" | ||
}, | ||
"repository": "", | ||
"repository": "http://", | ||
"author": "Philip Roberts", | ||
"license": "MIT" | ||
} |
103
README.md
@@ -1,26 +0,35 @@ | ||
# Colorize your logs (WIP) | ||
# Bows | ||
 | ||
Safe, production happy, colourful logging for chrome - makes reading your logs _much_ easier. | ||
- Safely wraps console.log for development mode | ||
- Optional colorizing on a module by module basis | ||
- Colors are automatically assigned and updated, to be as far from each other as possible | ||
 | ||
(Rain)bows makes logging debug messages in your apps much nicer. | ||
- It allows you to create custom loggers for each module in your app, that prefix all log messages with the name of the app, so that you can scan the messages more easily. | ||
- It colors the prefix differently and distinctly for each logger/module so that it's even easier to read. | ||
- It can be safely used in production, where logging will be disabled by default, so that you can leave log messages in your code. | ||
- Loggers safely wrap console.log, to maintain the line number from where they are called in the console output. | ||
## Usage | ||
- Works great in browserify | ||
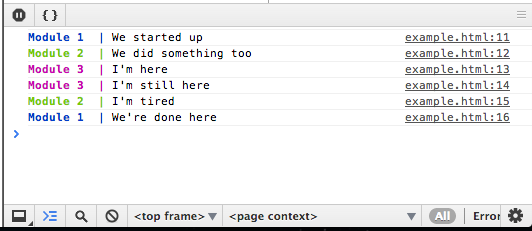 | ||
### Browserify | ||
```javascript | ||
//In mymodule.js | ||
var log = require('bows')('MyModule'); | ||
... | ||
## Installation. | ||
log(log.wrap('My log string')) //=> colorized "MyModule | My log string" | ||
If you are using browserify, you'll want something like: | ||
``` | ||
npm install bows --save | ||
``` | ||
/In another module.js | ||
var log = require('bows')('Another Module'); | ||
If you aren't using browserify, download either (bows.js)[https://raw.github.com/latentflip/bows/master/bows.js] or (bows.min.js)[https://raw.github.com/latentflip/bows/master/bows.min.js]. | ||
log(log.wrap('My log')) //=> colorized "Another Module | My log" | ||
``` | ||
## Usage | ||
- Works great in browserify and the browser. | ||
- Creating a new logger: | ||
- Browserify: `var log = require('bows')("My Module Name")` | ||
- Browser: `var log = bows("My Module Name")` | ||
- Then using it is easy: | ||
- `log("Module loaded") //-> "My Module Name | Module Loaded" | ||
- `log("Did something") //-> "My Module Name | Did something" | ||
- Typically each seperate module/view/etc in your app would create it's own logger. It will be assigned it's own color to make it easy to spot logs from different modules. | ||
- Logging is disabled by default. To enable logging, set `localStorage.debug = true` in your console and refresh the page. | ||
- You can leave the code in in production, and log() will just safely no-op unless localStorage.debug is set. | ||
@@ -30,41 +39,41 @@ ## Example | ||
```javascript | ||
var firstModule = (function() { | ||
var logwrap = bows('firstModule'); | ||
//Should be set in your console to see messages | ||
localStorage.debug = true | ||
//Configure the max length of module names | ||
bows.config({ padLength: 10 }) | ||
return function() { | ||
var a = 1 + 1; | ||
bows.log(logwrap('The result is', a)) | ||
return a | ||
} | ||
})(); | ||
var logger1 = bows('Module 1') | ||
var logger2 = bows('Module 2') | ||
var logger3 = bows('Module 3') | ||
var anotherModule = (function() { | ||
var logwrap = bows('this is another module'); | ||
logger1("We started up") | ||
logger2("We did something too") | ||
logger3("I'm here") | ||
logger3("I'm still here") | ||
logger2("I'm tired") | ||
logger1("We're done here") | ||
``` | ||
return function() { | ||
var b = 1 + 1; | ||
bows.log(logwrap('The result is', b)); | ||
return b; | ||
} | ||
})(); | ||
Result: | ||
var moreModule = (function() { | ||
var logwrap = bows('this is another module'); | ||
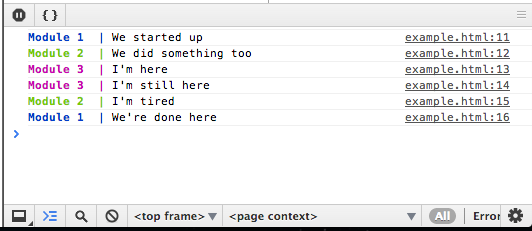 | ||
return function(val) { | ||
bows.log(logwrap('I got a ', val)); | ||
} | ||
})(); | ||
## License | ||
firstModule(); | ||
anotherModule(); | ||
moreModule(5); | ||
``` | ||
MIT | ||
Result: | ||
Copyright Philip Roberts [latentflip.com](http://latentflip.com) | ||
 | ||
## License | ||
## Contributing | ||
MIT | ||
Please feel free to raise issues, or make contributions: | ||
```bash | ||
git clone https://github.com/latentflip/bows.git | ||
cd bows | ||
npm install #install dependencies | ||
#edit bows.js | ||
node build.js #build dist/bows.js and dist/bows.min.js | ||
``` | ||
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Minified code
QualityThis package contains minified code. This may be harmless in some cases where minified code is included in packaged libraries, however packages on npm should not minify code.
Found 1 instance in 1 package
No repository
Supply chain riskPackage does not have a linked source code repository. Without this field, a package will have no reference to the location of the source code use to generate the package.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Environment variable access
Supply chain riskPackage accesses environment variables, which may be a sign of credential stuffing or data theft.
Found 1 instance in 1 package
No repository
Supply chain riskPackage does not have a linked source code repository. Without this field, a package will have no reference to the location of the source code use to generate the package.
Found 1 instance in 1 package
45350
79
105
3
6
1