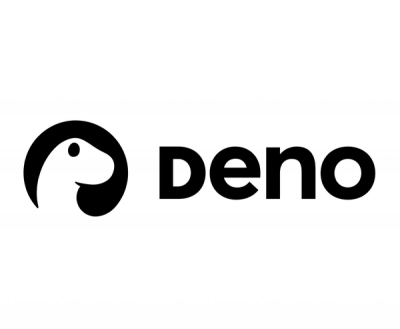
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
a high-priority asynchronous task queue.
(adapted from kriskowal/asap)
assuming we have access to a high-priority async method (like MutationObserver
, MessageChannel
, or setImmediate
):
setTimeout(function() {
console.log('this will print third');
});
briskit(function() {
console.log('this will print second');
});
(function() {
console.log('this will print first');
}());
briskit
will use setTimeout
as the async provider if nothing better is available.
multiple briskit
instances can be created with the fork
method. each instance will independently execute its own stack.
var $briskit = briskit.fork();
execution of a briskit
instance's stack can be stopped and started with the defer
and flush
methods, respectively.
var $briskit = briskit.fork();
$briskit.defer();
$briskit(function(){ console.log( "I'm deferred!" ) });
briskit(function(){ console.log( "I'm not!" ) }); // -> I'm not!
$briskit.flush(); // -> I'm deferred!
briskit
will use the best possible async provider for its environment, but if for whatever reason you would like to override that choice:
briskit.use( 'name' );
Name | Native Method |
---|---|
nextTic | setImmediate |
observer | MutationObserver |
worker | MessageChannel |
timeout | setTimeout |
use
will also accept a function that returns a custom provider. a custom, synchronous provider might look something like:
briskit.use(function() {
return function( cb ) {
cb();
};
});
the briskit stack can be used as a standalone class. all parameters are optional:
Parameter | Type | Default | Description |
---|---|---|---|
autoflush | boolean | false | When true, the stack will attempt to flush as soon as a callback is enqueued. |
provider | function | function( cb ){ cb() } | The function used for flush calls. Synchronous by default. |
prealloc | number | 1024 | The preallocated stack size. |
// commonjs
var stack = briskit.stack( true );
// ES6 (requires compilation)
import Stack from 'briskit/src/stack';
var stack = new Stack( true );
// usage
stack.defer();
stack.enqueue(function() {
// ...
});
stack.flush();
FAQs
High-priority asynchronous task queue
The npm package briskit receives a total of 7 weekly downloads. As such, briskit popularity was classified as not popular.
We found that briskit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.