Browser Capture Screenshot

Browser Capture Screenshot utilises Element Capture API or Region Capture API to capture screenshot of a given Element from the page.
npm install browser-capture-screenshot
Demo
See demo at https://amoshydra.github.io/browser-capture-screenshot
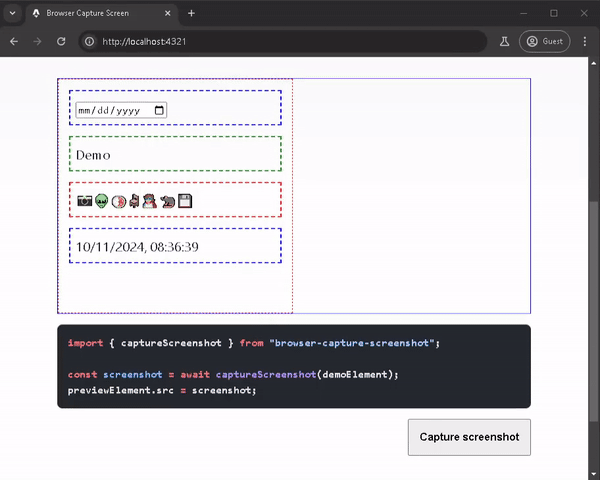
Usage
import { capture } from "browser-capture-screenshot";
const screenshot = await capture(demoElement);
previewElement.src = screenshot;
ScreenshotSession
provides more granular controls:
import { ScreenshotSession } from "browser-capture-screenshot";
const session = new ScreenshotSession();
await session.start();
setTimeout(async () => {
const screenshot = await session.capture(demoElement);
previewElement.src = screenshot;
await session.stop();
}, 1000);
API
capture
A simplified API for capturing screenshot. This method uses ScreenshotSession
internally.
export function capture(element: HTMLElement, options?: CaptureOptions): Promise<string>
return
A base64 encoded string representing the captured image wrapped in a Promise. e.g.: data:image/png;base64,iVBORw0K...
.
param element
The element HTMLElement
to take screenshot of.
param options
optional.
param options.api
optional. Specify how the screenshot should be captured.
{ api: "element" }
- uses Element Capture API{ api: "region" }
- uses Region Capture API
Default to using Element Capture API when not specified.
ScreenshotSession
export class ScreenshotSession {
static isSupported(): boolean;
start(): Promise<void>
stop()
capture(element: HTMLElement, options?: CaptureOptions): Promise<string>
}
Compatibility
This implementation requires the browser to support the following experimental API:
Notes:
- When using Element Capture API:
- The capture region need to be visible in the viewport
- System UI (such as context menu) or User Agent widget (i.e. Date Picker, Select option list) will not be captured
References
References
Similar projects