Browser-FS-Access
This module allows you to easily use the
File System Access API on supporting browsers,
with a transparent fallback to the <input type="file">
and <a download>
legacy methods.
This library is a ponyfill.
Read more on the background of this module in my post
Progressive Enhancement In the Age of Fugu APIs.
Live Demo
See the library in action: https://browser-fs-access.glitch.me/.
Installation
You can install the module with npm.
npm install --save browser-fs-access
Usage Example
The module feature-detects support for the File System Access API and
only loads the actually relevant code.
import {
fileOpen,
directoryOpen,
fileSave,
} from 'https://unpkg.com/browser-fs-access';
(async () => {
const blob = await fileOpen({
mimeTypes: ['image/*'],
});
const blobs = await fileOpen({
mimeTypes: ['image/*'],
multiple: true,
});
const blobsInDirectory = await directoryOpen({
recursive: true,
});
await fileSave(blob, {
fileName: 'Untitled.png',
extensions: ['.png'],
});
})();
API Documentation
Opening files:
const options = {
mimeTypes: ['image/*'],
extensions: ['.png', '.jpg', '.jpeg', '.webp'],
multiple: true,
description: 'Image files',
};
const blobs = await fileOpen(options);
Opening directories:
const options = {
recursive: true,
};
const blobs = await directoryOpen(options);
The module also polyfills a webkitRelativePath
property on returned files in a consistent way, regardless of the underlying implementation.
Saving files:
const options = {
fileName: 'Untitled.txt',
extensions: ['.txt'],
};
const handle = previouslyOpenedBlob.handle;
await fileSave(someBlob, options, handle);
Browser-FS-Access in Action
You can see the module in action in the Excalidraw drawing app.
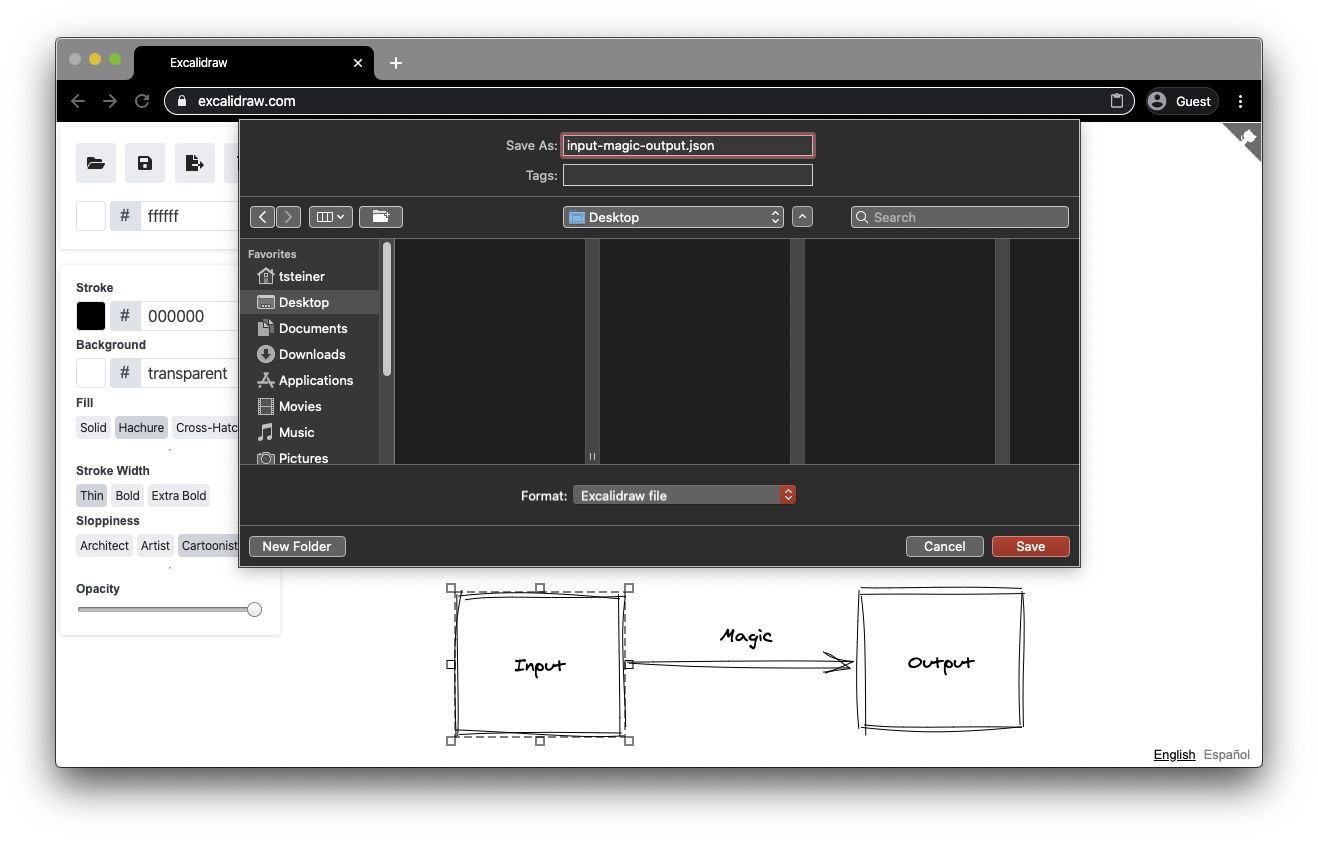
Alternatives
A similar, but more extensive library called
native-file-system-adapter
is provided by @jimmywarting.
Acknowledgements
Thanks to @developit
for improving the dynamic module loading
and @dwelle for the helpful feedback,
issue reports, and the Windows build fix.
Directory operations were made consistent regarding webkitRelativePath
and parallelized and sped up significantly by
@RReverser.
The TypeScript type annotations were provided by
@nanaian.
License and Note
Apache 2.0.
This is not an official Google product.