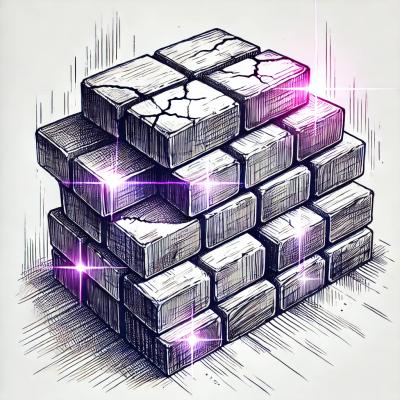
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Create pdf documents from liquid templates.
This library uses https://liquidjs.com/, https://symbology.dev/, https://www.npmjs.com/package/bz-date, https://www.npmjs.com/package/btrz-formatter and https://pdfmake.github.io/docs/0.1/ and link them togethers while adding some helpers to easily generate PDFs from templates.
npm i btrz-pdf
The documents generated only use Helvetica fonts.
Async method using a cb to return a buffer, best used with Express to return the PDF in the response.
Parameters
name | definition |
---|---|
liquidTemplate | object representing a pdfmake document with valid liquidSyntax |
data | Object with data needed by the liquid template |
cb | A callback with the signature (err, buffer) |
Async method that returns a promise with a PDF document
Parameters
name | definition |
---|---|
liquidTemplate | object representing a pdfmake document with valid liquidSyntax |
data | Object with data needed by the liquid template |
Returns an empty PDFmake document definition with some default styles
Async method that returns a PDFmake document defintion after processing the template and the data
Parameters
name | definition |
---|---|
liquidTemplate | object representing a pdfmake document with valid liquidSyntax |
data | Object with data needed by the liquid template |
Response
{
"defaultStyle": {
"font": "Helvetica",
"fontSize": 10,
"lineHeight": 1.3
},
"styles": {
"header": {
"fontSize": 16,
"bold": true,
"margin": [0, 0, 0, 10]
},
"subheader": {
"fontSize": 14,
"bold": true,
"margin": [0, 20, 0, 0]
},
"innerheader": {
"fontSize": 12,
"bold": true
},
"tableHeader": {
"fontSize": 8,
"bold": true,
"margin": [0, 4, 0, 0]
},
"table": {
"fontSize": 8,
"margin": [0, 8, 0, 0]
},
"attachedTable": {
"fontSize": 8
},
"cell": {
"margin": [0, 4, 0, 0]
},
"cellError": {
"margin": [0, 4, 0, 0],
"color": "#FF0000"
},
"cellMoney": {
"margin": [0, 4, 0, 0],
"alignment": "right"
},
"cellMoneyError": {
"margin": [0, 4, 0, 0],
"color": "#FF0000",
"alignment": "right"
},
"footer": {
"fontSize": 8
}
},
"content": []
}
const btrzPdf = require("btrz-pdf");
//Returns a PDF as data with Express
async previewPdf(req, res) {
try {
PDF.returnPdfBinary(template, data, (error, binary) => {
if (error) {
res.status(500).send(error);
} else {
res.contentType("application/pdf");
res.send(binary);
}
});
return;
} catch (err) {
res.status(500).send(error);
}
}
const btrzPdf = require("btrz-pdf");
//Returns a PDFmake document defintion from the template and the data
async getLiquid(req, res) {
try {
const document = await PDF.toDocumentDefinition(template, data);
res.send(document);
} catch (err) {
res.status(500).send(error.message);
}
}
Generates a barcode based on some data
Parameters
name | definition | required | default |
---|---|---|---|
data | The string to use to generate the barcode, it can be hardcoded or some of the 'data' given to the template | Y | |
type | The barcode type | N | code128 |
height | The barcode height | N | 30 |
width | The barcode width | N | 200 |
It will use the value of ticket.code to generate the barcode with all the defaults
{% barcode ticket.code %}
It will use the value given and use generate a 'code11' barcode with a height of 50 and a width of 300
{% barcode 1234 code11 50 300 %}
It supports all types supported by https://symbology.dev/. While symbology supports QRCODE PDFMake also had support for QR natively and we recommend it.
Returns a date formatted by the given format from a property of an object given to the liquid template data.
{%- dateTime ticket createdAt %} //"12/21/2021 11:38 AM"
{%- dateTime ticket createdAt mm/dd/yyyy hh:MM:ss %} //"12/21/2021 11:38:00"
Parameters
name | definition | required | default |
---|---|---|---|
item | An object in the data given to the liquid template | N | ticket |
propName | The name of the property of the item (it should be a BzDate object) | N | createdAt |
format | A format object | N | providerPreferences defaults (see prereqs) |
Prerequisites
There are some prerequisites to use any of the Date helpers. The data given to the liquid template requires the following object to be present
{
"providerPreferences": {
"preferences": {
"dateFormat": "mm/dd/yyyy", //any date format string will do
"timeFormat": "h:MM TT", //any time format string will do
"timeZone": {
"name": "(UTC-5:00) New York (United States), Toronto (Canada)",
"daylight": true,
"tz": "America/Toronto"
} //any timezone as defined in BzDate will do
}
}
}
Convenience method that will default format to providerPreferenes.preferences.dateFormat
Parameters
name | definition | required | default |
---|---|---|---|
item | An object in the data given to the liquid template | N | ticket |
propName | The name of the property of the item (it should be a BzDate object) | N | createdAt |
{%- dateF ticket createdAt %} //"12/21/2021"
Convenience method that will default format to providerPreferenes.preferences.timeFormat
Parameters
name | definition | required | default |
---|---|---|---|
item | An object in the data given to the liquid template | N | ticket |
propName | The name of the property of the item (it should be a BzDate object) | N | createdAt |
{%- timeF ticket createdAt %} //"11:38 AM"
Convenience method that will default format based on the humanDate
property given to the template as part of the data object. humanDate
can be either 'mm' or 'dd'
(humanDate === "mm") ? "ddd mmm dd, yyyy" : "ddd dd mmm, yyyy"
Parameters
name | definition | required | default |
---|---|---|---|
item | An object in the data given to the liquid template | N | ticket |
propName | The name of the property of the item (it should be a BzDate object) | N | createdAt |
{%- humanDate ticket createdAt %} //"Tue Dec 21, 2021"
Convenience method that will default format based on the humanDate
property given to the template as part of the data object. humanDate
can be either 'mm' or 'dd' plus the providerPreferenes.preferences.timeFormat
(humanDate === "mm") ? "ddd mmm dd, yyyy" : "ddd dd mmm, yyyy"
Parameters
name | definition | required | default |
---|---|---|---|
item | An object in the data given to the liquid template | N | ticket |
propName | The name of the property of the item (it should be a BzDate object) | N | createdAt |
{%- humanDateTime ticket createdAt %} //"Tue Dec 21, 2021 11:38 AM"
This tag will parse "some" HTML code and try to generate compatible PDFmake text objects.
Parameters
name | definition | required | default |
---|---|---|---|
property | this will be "evaluated" from the data provided to the liquid template | Y |
{% h ticket.lexiconKeys.terms %} //If will get the string in the property and parse it
h1 - will generate a text node with "style": "header" h2 - will generate a text node with "style": "subheader" h3 - will generate a text node with "style": "innerheader" b - will generate a text node with "bold": true i - will generate a text node with "italics'": true
This tag generates an horizontal line
Parameters
name | definition | required | default |
---|---|---|---|
width | the width of the line | N | 500 |
weight | the weight of the line | N | 1 |
rgb | the colour of the line | N | 0,0,0 |
{%- hline 475 2 255,112,0 -%} //Generates an svg line with the width, weight and colour given
{
"svg": "<svg height='2' width='100'><line x1='0' y1='0' x2='1000' y2='0' style='stroke:rgb(255,112,0);stroke-width:2' /></svg>",
"width": 475
}
Returns a translation based on a key, it can use a string or variables from the data given to the liquid template
Prerequisites
There are some prerequisites to use the t tag. The data given to the liquid template requires a localizer
object with a .get(key)
method that can return the translation. The implementation is up to you.
Parameters
name | definition | required | default |
---|---|---|---|
property | the key for the lexicon | Y |
{% t 'issued' %} //Will return the value on the lexicon with the 'issued' key
{% t fare.lexiconKeys.description %} //Will return the value on the lexicon with the key stored in the `data.fare.lexiconKeys.description` property
Returns a money value
Parameters
name | definition | required | default |
---|---|---|---|
item | An object in the data given to the liquid template | N | ticket |
propName | The name of the property of the item | N | total |
"{%- money ticket total -%}" //Given that ticket.total is 2800000 returns "28.00"
Adds the values of a property in objects in a collection and returns the total as a money value
Parameters
name | definition | required | default |
---|---|---|---|
item | An object in the data given to the liquid template | N | ticket |
propName | The name of the property of the item that is a collection of objects | N | taxes |
innerPropName | The name of the property in the objects in the collection | N | calculated |
"{%- money ticket fees subTotal -%}"
Returns the currency symbol based on the currency used to paid the item or the default currency given in the providerPreferences.preferences.supportedCurrencies[0]
Parameters
name | definition | required | default |
---|---|---|---|
item | An object in the data given to the liquid template | N | ticket |
{%- curcySymbol ticket -%}",
Returns the currency ISO code based on the currency used to paid the item or the default currency given in the providerPreferences.preferences.supportedCurrencies[0]
Parameters
name | definition | required | default |
---|---|---|---|
item | An object in the data given to the liquid template | N | ticket |
{%- curcyIso ticket -%}
FAQs
Generates pdf documents based on a liquid template
The npm package btrz-pdf receives a total of 201 weekly downloads. As such, btrz-pdf popularity was classified as not popular.
We found that btrz-pdf demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.