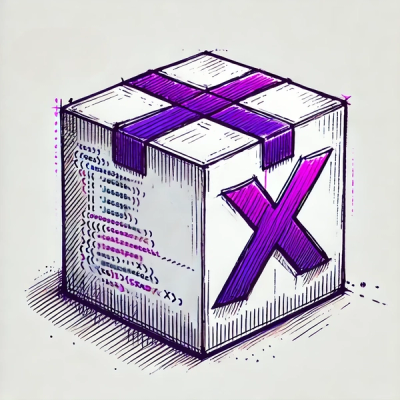
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Burro is a useful creature that auto-packages objects in length-prefixed JSON byte streams.
Burro is made up of 4 streams that makes sending/receiving objects a breeze
Sender streams:
Receiver streams:
var burro = require("burro"),
stream = require("stream");
// dummy network stream
var network = new stream.PassThrough();
// wrap! auto encode/decode json frames
var socket = burro.wrap(network);
// send data
socket.write({message: "どもうありがとう!", from: "japan", to: "usa"});
socket.write({message: "thank you!", from: "usa", to: "japan"});
// dummy parser; extracts message from payload
var parser = new stream.Transform({objectMode: true});
parser._transform = function _transform (obj, encoding, done) {
this.push(obj.from + " says: " + obj.message + "\n");
done();
};
// cross the streams!
socket.pipe(parser).pipe(process.stdout);
Output
japan says: どもうありがとう!
usa says: thank you!
Available via npm:
$ npm install burro
Or via git:
$ git clone git://github.com/naomik/burro.git node_modules/burro
wrap
This is burro's easy mode. It will automatically construct the entire burro chain around your existing duplex stream. If you want to configure the burro chain manually, please see lib/burro.js
var socket = burro.wrap(duplexStream);
Arguments
stream.Duplex
API. It must have
the following functions defined: _read
, _write
, pipe
, unpipe
.Burro is a pretty versatile beast and can even handle very large payloads. It is also tested against network packet fragmenting and buffering, thanks to hiccup. See the tests for more details.
Development dependencies:
$ npm install mocha
$ npm install hiccup
FAQs
auto-packaged, length-prefixed JSON byte streams
The npm package burro receives a total of 24 weekly downloads. As such, burro popularity was classified as not popular.
We found that burro demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.