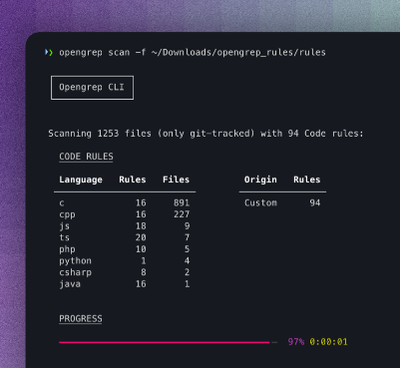
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
cache-manager-redis-store
Advanced tools
The cache-manager-redis-store npm package is a Redis store for the cache-manager module. It allows you to use Redis as a caching backend for your Node.js applications, providing a simple and efficient way to cache data and improve performance.
Basic Setup
This code demonstrates how to set up a basic cache using cache-manager-redis-store. It initializes a cache with Redis as the backend, specifying the host, port, and time-to-live (TTL) for cached items.
const cacheManager = require('cache-manager');
const redisStore = require('cache-manager-redis-store');
const cache = cacheManager.caching({
store: redisStore,
host: 'localhost', // default value
port: 6379, // default value
ttl: 600 // time to live in seconds
});
Set and Get Cache
This code demonstrates how to set a value in the cache and then retrieve it. The value 'bar' is stored with the key 'foo' and a TTL of 10 seconds. The value is then retrieved and logged to the console.
cache.set('foo', 'bar', { ttl: 10 }, (err) => {
if (err) throw err;
cache.get('foo', (err, result) => {
if (err) throw err;
console.log(result); // 'bar'
});
});
Wrap Function
This code demonstrates how to use the wrap function to cache the result of a long-running operation. The wrap function checks if the value is already in the cache; if not, it executes the provided function, caches the result, and returns it.
cache.wrap('foo', (cb) => {
// Simulate a long-running operation
setTimeout(() => {
cb(null, 'bar');
}, 2000);
}, { ttl: 10 }, (err, result) => {
if (err) throw err;
console.log(result); // 'bar'
});
Del Cache
This code demonstrates how to delete a value from the cache. The value associated with the key 'foo' is removed from the cache.
cache.del('foo', (err) => {
if (err) throw err;
console.log('Cache for key foo deleted');
});
The 'redis' package is a low-level Redis client for Node.js. It provides a comprehensive set of features for interacting with a Redis server, but it does not include caching functionalities out of the box. It is more flexible and can be used for a wider range of Redis operations compared to cache-manager-redis-store.
The 'ioredis' package is another popular Redis client for Node.js. It supports advanced Redis features like clustering and sentinel. Similar to the 'redis' package, it does not provide built-in caching functionalities but offers a robust and feature-rich interface for interacting with Redis.
The 'node-cache' package is an in-memory caching solution for Node.js. It provides a simple API for caching data in memory, but it does not support Redis or other external storage backends. It is suitable for applications that require a lightweight, in-memory cache without the need for a distributed cache like Redis.
Redis cache store for node-cache-manager.
node-cache-manager-redis
?This is a completely different version than the earlier node-cache-manager-redis. This package does not use redis-pool
which is unnecessary and not actively maintained.
This package aims to provide the most simple wrapper possible by just passing the configuration to the underlying node_redis
package.
npm install cache-manager-redis-store --save
or
yarn add cache-manager-redis-store
See examples below on how to implement the Redis cache store.
var cacheManager = require('cache-manager');
var redisStore = require('cache-manager-redis-store');
var redisCache = cacheManager.caching({
store: redisStore,
host: 'localhost', // default value
port: 6379, // default value
auth_pass: 'XXXXX',
db: 0,
ttl: 600
});
// listen for redis connection error event
var redisClient = redisCache.store.getClient();
redisClient.on('error', (error) => {
// handle error here
console.log(error);
});
var ttl = 5;
redisCache.set('foo', 'bar', { ttl: ttl }, (err) => {
if (err) {
throw err;
}
redisCache.get('foo', (err, result) => {
console.log(result);
// >> 'bar'
redisCache.del('foo', (err) => {
});
});
});
function getUser(id, cb) {
setTimeout(() => {
console.log("Returning user from slow database.");
cb(null, { id: id, name: 'Bob' });
}, 100);
}
var userId = 123;
var key = `user_${userId}`;
// Note: ttl is optional in wrap()
redisCache.wrap(key, (cb) => {
getUser(userId, cb);
}, { ttl: ttl }, (err, user) => {
console.log(user);
// Second time fetches user from redisCache
redisCache
.wrap(key, () => getUser(userId))
.then(console.log)
.catch(err => {
// handle error
});
});
var cacheManager = require('cache-manager');
var redisStore = require('cache-manager-redis-store');
var redisCache = cacheManager.caching({ store: redisStore, db: 0, ttl: 600 });
var memoryCache = cacheManager.caching({ store: 'memory', max: 100, ttl: 60 });
var multiCache = cacheManager.multiCaching([memoryCache, redisCache]);
var userId2 = 456;
var key2 = `user_${userId2}`;
// Set value in all caches
multiCache.set('foo2', 'bar2', { ttl: ttl }, (err) => {
if (err) {
throw err;
}
// Fetches from highest priority cache that has the key
multiCache.get('foo2', (err, result) => {
console.log(result);
// Delete from all caches
multiCache.del('foo2');
});
});
// Note: ttl is optional in wrap
multiCache.wrap(key2, (cb) => {
getUser(userId2, cb);
}, (err, user) => {
console.log(user);
// Second time fetches user from memoryCache, since it's highest priority.
// If the data expires in the memory cache, the next fetch would pull it from
// the 'someOtherCache', and set the data in memory again.
multiCache.wrap(key2, (cb) => {
getUser(userId2, cb);
}, (err, user) => {
console.log(user);
});
});
Want to help improve this package? We take pull requests.
The node-cache-manager-redis-store
is licensed under the MIT license.
v3.0.1 - 17 Oct, 2022
Fixed bug where the redisStore.del
would no longer accept an options object, which broke the multiCaching interface.
FAQs
Redis store for node-cache-manager
We found that cache-manager-redis-store demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.