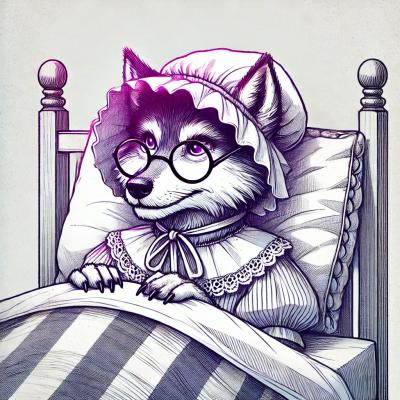
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
cacheable-request
Advanced tools
The cacheable-request npm package is a wrapper for the native HTTP/HTTPS request functionality in Node.js, providing an easy-to-use cache layer for repeat requests. It is designed to cache responses based on their Cache-Control header and supports the caching of GET and HEAD requests.
Caching GET and HEAD requests
This feature allows you to cache GET and HEAD requests. The code sample shows how to create a cacheable request using the cacheable-request package with a custom cache adapter.
const CacheableRequest = require('cacheable-request');
const http = require('http');
const cacheAdapter = require('./some-cache-adapter');
const cacheableRequest = new CacheableRequest(http.request, cacheAdapter);
const req = cacheableRequest('http://example.com', response => {
// Use the response here
});
req.on('request', request => request.end());
Custom cache adapters
This feature allows the use of custom cache adapters to store and retrieve cached responses. The code sample demonstrates how to use a custom cache adapter with the cacheable-request package.
const CacheableRequest = require('cacheable-request');
const http = require('http');
const myCacheAdapter = {
get: (key) => { /* custom get implementation */ },
set: (key, value) => { /* custom set implementation */ }
};
const cacheableRequest = new CacheableRequest(http.request, myCacheAdapter);
HTTP and HTTPS support
The package supports both HTTP and HTTPS protocols. The code sample illustrates how to create a cacheable HTTPS request.
const CacheableRequest = require('cacheable-request');
const https = require('https');
const cacheAdapter = require('./some-cache-adapter');
const cacheableRequest = new CacheableRequest(https.request, cacheAdapter);
const req = cacheableRequest('https://secure.example.com', response => {
// Use the response here
});
req.on('request', request => request.end());
axios-cache-adapter is a cache adapter for the Axios HTTP client. It allows you to easily add caching capabilities to your Axios requests. Unlike cacheable-request, which is a standalone request wrapper, axios-cache-adapter is specifically designed to work with Axios and provides more advanced features like cache invalidation and per-request cache configuration.
node-fetch-cache is a caching layer for node-fetch, a lightweight module that brings window.fetch to Node.js. It provides similar caching functionality to cacheable-request but is tailored for use with node-fetch. It also offers features like cache size limits and custom cache keys.
got is a powerful HTTP request library for Node.js that includes caching as one of its features. It is more feature-rich than cacheable-request, offering a wide range of HTTP capabilities such as retries, streams, and more. got's caching is built-in and does not require a separate adapter, making it a more integrated solution.
Wrap native HTTP requests with RFC compliant cache support
RFC 7234 compliant HTTP caching for native Node.js HTTP/HTTPS requests. Caching works out of the box in memory or is easily pluggable with a wide range of storage adapters.
Note: This is a low level wrapper around the core HTTP modules, it's not a high level request library.
This release contains breaking changes. This is the new way to use this package.
import http from 'http';
import CacheableRequest from 'cacheable-request';
// Then instead of
const req = http.request('http://example.com', cb);
req.end();
// You can do
const cacheableRequest = new CacheableRequest(http.request);
const cacheReq = cacheableRequest('http://example.com', cb);
cacheReq.on('request', req => req.end());
// Future requests to 'example.com' will be returned from cache if still valid
// You pass in any other http.request API compatible method to be wrapped with cache support:
const cacheableRequest = new CacheableRequest(https.request);
const cacheableRequest = new CacheableRequest(electron.net);
import CacheableRequest from 'cacheable-request';
// Now You can do
const cacheableRequest = new CacheableRequest(http.request).request();
const cacheReq = cacheableRequest('http://example.com', cb);
cacheReq.on('request', req => req.end());
// Future requests to 'example.com' will be returned from cache if still valid
// You pass in any other http.request API compatible method to be wrapped with cache support:
const cacheableRequest = new CacheableRequest(https.request).request();
const cacheableRequest = new CacheableRequest(electron.net).request();
The biggest change is that when you do a new
CacheableRequest you now want to call request
method will give you the instance to use.
- const cacheableRequest = new CacheableRequest(http.request);
+ const cacheableRequest = new CacheableRequest(http.request).request();
We are now using pure esm support in our package. If you need to use commonjs you can use v8 or lower. To learn more about using ESM please read this from sindresorhus
: https://gist.github.com/sindresorhus/a39789f98801d908bbc7ff3ecc99d99c
If-None-Match
/If-Modified-Since
headersAge
header on cached responsesnpm install cacheable-request
import http from 'http';
import CacheableRequest from 'cacheable-request';
// Then instead of
const req = http.request('http://example.com', cb);
req.end();
// You can do
const cacheableRequest = new CacheableRequest(http.request).createCacheableRequest();
const cacheReq = cacheableRequest('http://example.com', cb);
cacheReq.on('request', req => req.end());
// Future requests to 'example.com' will be returned from cache if still valid
// You pass in any other http.request API compatible method to be wrapped with cache support:
const cacheableRequest = new CacheableRequest(https.request).createCacheableRequest();
const cacheableRequest = new CacheableRequest(electron.net).createCacheableRequest();
cacheable-request
uses Keyv to support a wide range of storage adapters.
For example, to use Redis as a cache backend, you just need to install the official Redis Keyv storage adapter:
npm install @keyv/redis
And then you can pass CacheableRequest
your connection string:
const cacheableRequest = new CacheableRequest(http.request, 'redis://user:pass@localhost:6379').createCacheableRequest();
View all official Keyv storage adapters.
Keyv also supports anything that follows the Map API so it's easy to write your own storage adapter or use a third-party solution.
e.g The following are all valid storage adapters
const storageAdapter = new Map();
// or
const storageAdapter = require('./my-storage-adapter');
// or
const QuickLRU = require('quick-lru');
const storageAdapter = new QuickLRU({ maxSize: 1000 });
const cacheableRequest = new CacheableRequest(http.request, storageAdapter).createCacheableRequest();
View the Keyv docs for more information on how to use storage adapters.
Returns the provided request function wrapped with cache support.
Type: function
Request function to wrap with cache support. Should be http.request
or a similar API compatible request function.
Type: Keyv storage adapter
Default: new Map()
A Keyv storage adapter instance, or connection string if using with an official Keyv storage adapter.
Returns an event emitter.
Type: object
, string
http-cache-semantics
options.Type: boolean
Default: true
If the cache should be used. Setting this to false will completely bypass the cache for the current request.
Type: boolean
Default: false
If set to true
once a cached resource has expired it is deleted and will have to be re-requested.
If set to false
(default), after a cached resource's TTL expires it is kept in the cache and will be revalidated on the next request with If-None-Match
/If-Modified-Since
headers.
Type: number
Default: undefined
Limits TTL. The number
represents milliseconds.
Type: boolean
Default: false
When set to true
, if the DB connection fails we will automatically fallback to a network request. DB errors will still be emitted to notify you of the problem even though the request callback may succeed.
Type: boolean
Default: false
Forces refreshing the cache. If the response could be retrieved from the cache, it will perform a new request and override the cache instead.
Type: function
The callback function which will receive the response as an argument.
The response can be either a Node.js HTTP response stream or a responselike object. The response will also have a fromCache
property set with a boolean value.
request
event to get the request object of the request.
Note: This event will only fire if an HTTP request is actually made, not when a response is retrieved from cache. However, you should always handle the request
event to end the request and handle any potential request errors.
response
event to get the response object from the HTTP request or cache.
error
event emitted in case of an error with the cache.
Errors emitted here will be an instance of CacheableRequest.RequestError
or CacheableRequest.CacheError
. You will only ever receive a RequestError
if the request function throws (normally caused by invalid user input). Normal request errors should be handled inside the request
event.
To properly handle all error scenarios you should use the following pattern:
cacheableRequest('example.com', cb)
.on('error', err => {
if (err instanceof CacheableRequest.CacheError) {
handleCacheError(err); // Cache error
} else if (err instanceof CacheableRequest.RequestError) {
handleRequestError(err); // Request function thrown
}
})
.on('request', req => {
req.on('error', handleRequestError); // Request error emitted
req.end();
});
Note: Database connection errors are emitted here, however cacheable-request
will attempt to re-request the resource and bypass the cache on a connection error. Therefore a database connection error doesn't necessarily mean the request won't be fulfilled.
Hooks have been implemented since version v9
and are very useful to modify response before saving it in cache. You can use hooks to modify response before saving it in cache.
The hook will pre compute response right before saving it in cache. You can include many hooks and it will run in order you add hook on response object.
import http from 'http';
import CacheableRequest from 'cacheable-request';
const cacheableRequest = new CacheableRequest(request, cache).request();
// adding a hook to decompress response
cacheableRequest.addHook('onResponse', async (value: CacheValue, response: any) => {
const buffer = await pm(gunzip)(value.body);
value.body = buffer.toString();
return value;
});
here is also an example of how to add in the remote address
import CacheableRequest, {CacheValue} from 'cacheable-request';
const cacheableRequest = new CacheableRequest(request, cache).request();
cacheableRequest.addHook('onResponse', (value: CacheValue, response: any) => {
if (response.connection) {
value.remoteAddress = response.connection.remoteAddress;
}
return value;
});
You can also remove hook by using below
CacheableRequest.removeHook('onResponse');
Cacheable-Request is an open source package and community driven that is maintained regularly. In addtion we have a couple of other guidelines for review:
To post an issue, navigate to the "Issues" tab in the main repository, and then select "New Issue." Enter a clear title describing the issue, as well as a description containing additional relevant information. Also select the label that best describes your issue type. For a bug report, for example, create an issue with the label "bug." In the description field, Be sure to include replication steps, as well as any relevant error messages.
If you're reporting a security violation, be sure to check out the project's security policy.
Please also refer to our Code of Conduct for more information on how to report issues.
To ask a question, create an issue with the label "question." In the issue description, include the related code and any context that can help us answer your question.
This project is under the MIT license.
MIT © Luke Childs 2017-2021 and Jared Wray 2022+
FAQs
Wrap native HTTP requests with RFC compliant cache support
The npm package cacheable-request receives a total of 7,047,299 weekly downloads. As such, cacheable-request popularity was classified as popular.
We found that cacheable-request demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.