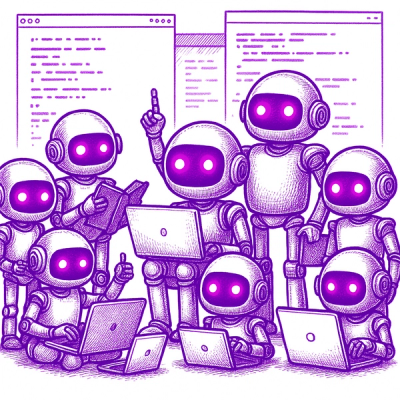
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
calc-image-stats
Advanced tools
Calculate Band Statistics for an Image
🦺 memory-safe: uses iterators to avoid copying pixel value arrays
🚀 fast: uses calc-stats, which avoids intermediary calculations
♦️ dynamic: works on numerical image data in any layout (by using xdim)
🧭 precise: support for super precise calculations (by using preciso)
⭐ type-safe: supports TypeScript
npm install calc-image-stats
import calcImageStats from "calc-image-stats";
// array of RGBA values of 10x10 image
const data = [
52, 70, 42, 255, 56, 72, 53, 255, 45, 60, 45, 255,
37, 54, 30, 255, 62, 85, 48, 255, 70, 88, 53, 255,
// ... 376 more items
];
const stats = calcImageStats(data, { height: 10, width: 10 });
stats will be the following object:
{
depth: 4, // number of bands
height: 10,
width: 10,
bands: [
{
// red band
count: 100,
valid: 100,
invalid: 0,
median: 87,
min: 9,
max: 220,
sum: 10489,
range: 211,
mean: 104.89,
std: 53.908792418305936,
modes: [51, 69, 87, 190],
mode: 99.25
},
{ ... }, // green band
{ ... }, // blue band
{
// alpha band
count: 100,
valid: 100,
invalid: 0,
median: 255,
min: 255,
max: 255,
sum: 25500,
range: 0,
mean: 255,
std: 0,
modes: [255],
mode: 255
}
]
};
const stats = calcImageStats(data, {
height: 123456,
precise: true, // calculate using super precise numerical strings
stats: ["variance"], // choose which stats to calculate
width: 123456
});
{
depth: 1, // only 1 band of data
height: 123456,
width: 123456,
bands: [
{
// super precise result because we set precise to true
variance: "1321.41725347154236125321387514273412736"
}
]
}
FAQs
Calculate Band Statistics for an Image
We found that calc-image-stats demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.