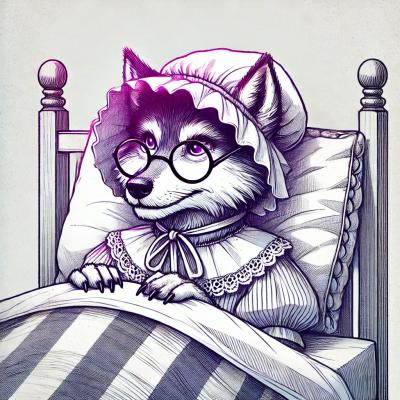
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Inspired by three.js and ammo.js, and driven by the fact that the web lacks a physics engine, here comes cannon.js. The rigid body physics engine includes simple collision detection, various body shapes, contacts, friction and constraints.
Demos - Documentation - Rendering hints - NPM package - CDN
Just include cannon.js or cannon.min.js in your html and you're done:
<script src="cannon.min.js"></script>
Install the cannon package via NPM:
npm install --save cannon
Alternatively, point to the Github repo directly to get the very latest version:
npm install --save schteppe/cannon.js
The sample code below creates a sphere on a plane, steps the simulation, and prints the sphere simulation to the console. Note that Cannon.js uses SI units (metre, kilogram, second, etc.).
// Setup our world
var world = new CANNON.World({
gravity: new CANNON.Vec3(0, 0, -9.82) // m/s²
});
// Create a sphere
var radius = 1; // m
var sphereBody = new CANNON.Body({
mass: 5, // kg
position: new CANNON.Vec3(0, 0, 10), // m
shape: new CANNON.Sphere(radius)
});
world.addBody(sphereBody);
// Create a plane
var groundBody = new CANNON.Body({
mass: 0 // mass == 0 makes the body static
});
var groundShape = new CANNON.Plane();
groundBody.addShape(groundShape);
world.addBody(groundBody);
var fixedTimeStep = 1.0 / 60.0; // seconds
var maxSubSteps = 3;
// Start the simulation loop
var lastTime;
(function simloop(time){
requestAnimationFrame(simloop);
if(lastTime !== undefined){
var dt = (time - lastTime) / 1000;
world.step(fixedTimeStep, dt, maxSubSteps);
}
console.log("Sphere z position: " + sphereBody.position.z);
lastTime = time;
})();
If you want to know how to use cannon.js with a rendering engine, for example Three.js, see the Examples.
Sphere | Plane | Box | Convex | Particle | Heightfield | Trimesh | |
---|---|---|---|---|---|---|---|
Sphere | Yes | Yes | Yes | Yes | Yes | Yes | Yes |
Plane | - | - | Yes | Yes | Yes | - | Yes |
Box | - | - | Yes | Yes | Yes | Yes | (todo) |
Cylinder | - | - | Yes | Yes | Yes | Yes | (todo) |
Convex | - | - | - | Yes | Yes | Yes | (todo) |
Particle | - | - | - | - | - | (todo) | (todo) |
Heightfield | - | - | - | - | - | - | (todo) |
Trimesh | - | - | - | - | - | - | - |
The simpler todos are marked with @todo
in the code. Github Issues can and should also be used for todos.
Create an issue if you need help.
FAQs
A lightweight 3D physics engine written in JavaScript.
The npm package cannon receives a total of 1,718 weekly downloads. As such, cannon popularity was classified as popular.
We found that cannon demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.