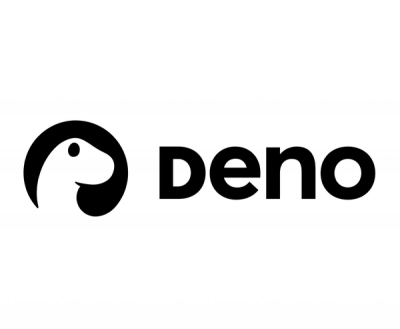
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
capacitance
Advanced tools
Collapse stream to a promise.
We are often write ugly code such as below:
let buffers = [];
stream.on('data', buffer => buffers.push(buffer));
stream.on('end', () => {
console.log(Buffer.concat(buffers));
});
Use the Capacitance, just write:
stream.pipe(new Capacitance).then(data => {
console.log(data);
});
#!/usr/bin/env babel-node --stage 0
import Capacitance from 'capacitance';
import http from 'http';
http.createServer(async (req, res) => {
switch(req.method) {
case 'GET':
res.writeHead(200, { 'Content-Type': 'text/html' });
res.write(`
<form method="post">
<input name="test" value="xxx" />
<input type="submit" value="Submit" />
</form>
`);
return res.end();
case 'POST':
// Convert stream to a promise and await it as a synchronous data
let data = await req.pipe(new Capacitance());
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.write(data);
return res.end();
}
}).listen(8000);
FAQs
Collapse stream to a promise.
The npm package capacitance receives a total of 6 weekly downloads. As such, capacitance popularity was classified as not popular.
We found that capacitance demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.