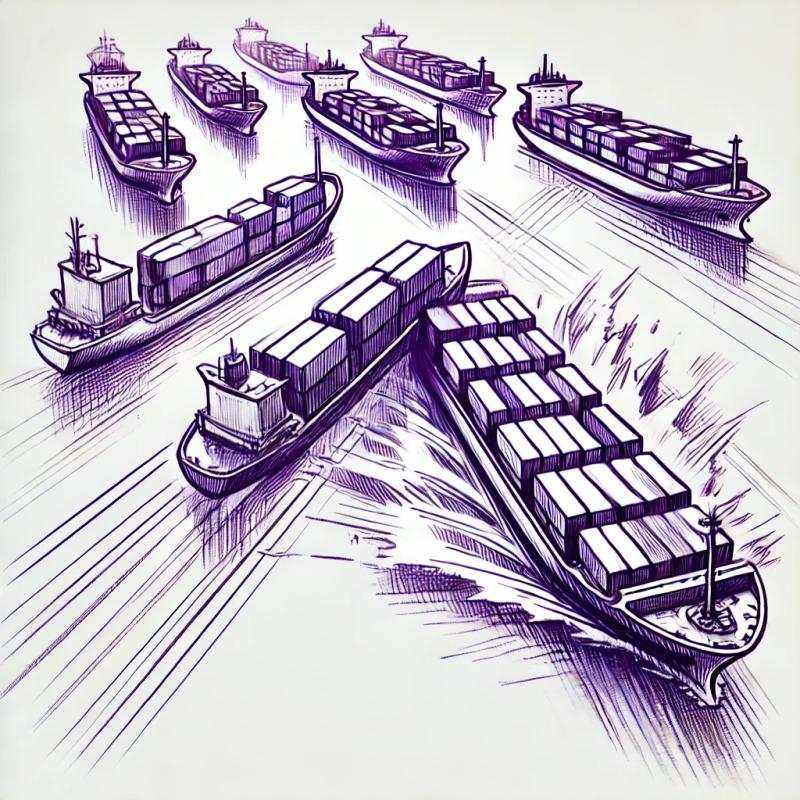
Security News
Namecheap Takes Down Polyfill.io Service Following Supply Chain Attack
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
capnp-ts
Advanced tools
Changelog
0.7.0 (2021-08-19)
capnpc-js
is being retired in favor of compiling
directly to js from capnpc-ts
instead. This makes the one compiler
serve both purposes; js-only users who are annoyed by the extra d.ts
files may simply delete them. This was done to work around bugs in
source-map-support that prevent importing capnp.js files when a
capnp.ts file is also present.Readme
A strongly typed Cap'n Proto implementation for the browser and Node.js using TypeScript.
Here's a quick usage example:
import * as capnp from 'capnp-ts';
import {MyStruct} from './myschema.capnp';
export function loadMessage(buffer: ArrayBuffer): MyStruct {
const message = capnp.Message.fromArrayBuffer(buffer);
return message.getRoot(MyStruct);
}
An extended readme is available on the project site: https://github.com/jdiaz5513/capnp-ts.
FAQs
Strongly typed Cap'n Proto implementation for the browser and Node.js using TypeScript
We found that capnp-ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.
Security News
A JavaScript library maintainer is under fire after merging a controversial PR to support legacy versions of Node.js.