caravaggio
Advanced tools
Comparing version 1.3.6 to 2.0.0
const path = require('path'); | ||
const fs = require('fs'); | ||
let image; | ||
const getImage = () => (image | ||
? Promise.resolve(image) | ||
: new Promise((resolve, reject) => { | ||
fs.readFile(path.join(__dirname, '..', 'test', 'fixtures', 'caravaggio.jpg'), (err, data) => { | ||
if (err) return reject(err); | ||
image = data; | ||
return resolve(data); | ||
}); | ||
})); | ||
const getImage = (name = 'caravaggio.jpg') => new Promise((resolve, reject) => { | ||
fs.readFile(path.join(__dirname, '..', 'test', 'fixtures', name), (err, data) => { | ||
if (err) return reject(err); | ||
return resolve(data); | ||
}); | ||
}); | ||
@@ -15,0 +11,0 @@ module.exports = (/* url */) => getImage() |
# Changelog | ||
## 2.0.0 | ||
- ✨ Ready to play hard! | ||
- Resize methods implemented, a lot, check the documentation | ||
- Extract method implemented | ||
- Ready for production!! | ||
## 1.3.6 | ||
@@ -4,0 +11,0 @@ |
{ | ||
"name": "caravaggio", | ||
"version": "1.3.6", | ||
"version": "2.0.0", | ||
"description": "A blazing fast image processor service", | ||
@@ -10,3 +10,3 @@ "main": "index.js", | ||
"now-start": "bin/caravaggio --cache memory", | ||
"dev": "NODE_ENV=development micro-dev src/ -s | pino", | ||
"dev": "NODE_ENV=development micro-dev -p 3001 src/ -s | pino", | ||
"test": "npm run lint && npm run test-only -- --coverage --colors=false", | ||
@@ -38,3 +38,2 @@ "test-only": "NODE_ENV=test ALLOW_CONFIG_MUTATIONS=true jest", | ||
"config": "^1.29.2", | ||
"configuration": "^0.1.1", | ||
"fs-extra": "^5.0.0", | ||
@@ -73,4 +72,9 @@ "md5": "^2.2.1", | ||
"<rootDir>/node_modules/" | ||
], | ||
"moduleDirectories": [ | ||
"node_modules", | ||
"src", | ||
"test" | ||
] | ||
} | ||
} |
@@ -12,9 +12,14 @@ [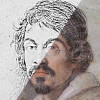](https://res.cloudinary.com/ramiel/image/upload/c_scale,r_0,w_100/v1517679412/caravaggio-logo_xdwpin.jpg) | ||
This is an early release of this software! This is **not** yet suitable for production. | ||
The docker image is not yet production ready. | ||
The api can change without any warning. | ||
Feel free to contribute to make this an amazing project! | ||
From version `2.x` this project is ready for production. If you have still version 1.x, upgrade. There is no migration guide since | ||
the first version was intended for test only | ||
## Installation | ||
### NPM | ||
``` | ||
npm i -g caravaggio | ||
caravaggio | ||
``` | ||
### Docker | ||
@@ -28,9 +33,3 @@ | ||
### Node | ||
``` | ||
npm i -g caravaggio | ||
caravaggio | ||
``` | ||
### Git | ||
@@ -62,2 +61,3 @@ | ||
- [micro](https://github.com/zeit/micro) - A fast microservice library | ||
- A lot of other great developers | ||
- A lot of other great developers | ||
- The amazing photographers on [pexels.com](https://www.pexels.com/) |
const fetch = require('node-fetch'); | ||
const sharp = require('sharp'); | ||
const config = require('config'); | ||
const logger = require('./logger'); | ||
@@ -8,4 +9,7 @@ sharp.cache(config.get('sharp.cache')); | ||
const Image = { | ||
get: url => fetch(url) | ||
.then(body => body.buffer()), | ||
get: (url) => { | ||
logger.debug(`Download image ${url}`); | ||
return fetch(url) | ||
.then(body => body.buffer()); | ||
}, | ||
@@ -12,0 +16,0 @@ getImageHandler: url => Image.get(url) |
@@ -0,1 +1,2 @@ | ||
const { buildDocumentationLink } = require('../utils'); | ||
const blurNormalizer = require('./blur'); | ||
@@ -7,2 +8,3 @@ const cropNormalizer = require('./crop'); | ||
const resizeNormalizer = require('./resize'); | ||
const extractNormalizer = require('./extract'); | ||
const rotateNormalizer = require('./rotate'); | ||
@@ -17,3 +19,6 @@ const progressiveNormalizer = require('./progressive'); | ||
q: qNormalizer, | ||
rs: resizeNormalizer, | ||
resize: resizeNormalizer, | ||
ex: extractNormalizer, | ||
extract: extractNormalizer, | ||
rotate: rotateNormalizer, | ||
@@ -23,3 +28,12 @@ progressive: progressiveNormalizer, | ||
class UnknownOperation extends Error { | ||
constructor(operation) { | ||
super(`Unknown operation "${operation}" | ||
See documentation at ${buildDocumentationLink('')} | ||
`); | ||
this.statusCode = 400; | ||
} | ||
} | ||
module.exports = (config) => { | ||
@@ -45,28 +59,34 @@ const defaultTransformations = config.get('defaultTransformations'); | ||
return (options) => { | ||
const result = addDefaultsTransformations(options.operations).reduce((acc, [name, params]) => { | ||
const normalized = normalizers[name] | ||
? normalizers[name](params) | ||
: {}; | ||
return { | ||
...acc, | ||
...normalized, | ||
input: [ | ||
...acc.input, | ||
...(normalized.input || []), | ||
], | ||
transformations: [ | ||
...acc.transformations, | ||
...(normalized.transformations || []), | ||
], | ||
output: [ | ||
...acc.output, | ||
...(normalized.output || []), | ||
], | ||
}; | ||
}, { | ||
...options, input: [], transformations: [], output: [], | ||
}); | ||
// console.log(JSON.stringify(result, null, ' ')); | ||
const result = addDefaultsTransformations(options.operations).reduce( | ||
(acc, [name, ...params]) => { | ||
if (!normalizers[name]) { | ||
throw new UnknownOperation(name); | ||
} | ||
const normalized = normalizers[name](...params); | ||
// const normalized = normalizers[name] | ||
// ? normalizers[name](...params) | ||
// : {}; | ||
return { | ||
...acc, | ||
...normalized, | ||
input: [ | ||
...acc.input, | ||
...(normalized.input || []), | ||
], | ||
transformations: [ | ||
...acc.transformations, | ||
...(normalized.transformations || []), | ||
], | ||
output: [ | ||
...acc.output, | ||
...(normalized.output || []), | ||
], | ||
}; | ||
}, | ||
{ | ||
...options, input: [], transformations: [], output: [], | ||
}, | ||
); | ||
return result; | ||
}; | ||
}; |
@@ -18,3 +18,3 @@ const logger = require('../logger'); | ||
} | ||
logger.debug(`Applying output operation "${name} -> ${operation}" with parameters: ${JSON.stringify(params, null, '')}`); | ||
logger.debug(`Applying output operation "${name}:${operation}" with parameters: ${JSON.stringify(params, null, '')}`); | ||
return pipeline.applyOperation(operation, ...params); | ||
@@ -21,0 +21,0 @@ }); |
@@ -11,3 +11,3 @@ const logger = require('../logger'); | ||
} | ||
logger.debug(`Applying transformation "${operation}" with parameters: ${JSON.stringify(params, null, '')}`); | ||
logger.debug(`Applying transformation "${name}:${operation}" with parameters: ${JSON.stringify(params, null, '')}`); | ||
return pipeline.applyOperation(operation, ...params); | ||
@@ -14,0 +14,0 @@ }); |
@@ -11,3 +11,6 @@ module.exports = { | ||
buildDocumentationLink: doc => `https://ramiel.gitlab.io/caravaggio/docs/${doc}`, | ||
isPercentage: percentage => `${percentage}`.indexOf('.') !== -1, | ||
percentageToPixel: (percentage, size) => Math.round(percentage * size), | ||
}; | ||
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Deprecated
MaintenanceThe maintainer of the package marked it as deprecated. This could indicate that a single version should not be used, or that the package is no longer maintained and any new vulnerabilities will not be fixed.
Found 1 instance in 1 package
51279
10
56
1322
0
- Removedconfiguration@^0.1.1
- Removedconfiguration@0.1.1(transitive)
- Removedunderscore@1.3.3(transitive)