Comparing version 3.0.7 to 3.0.8
@@ -1,2 +0,10 @@ | ||
export declare class Config { | ||
export interface ConfigInterface { | ||
getString(key: string): string; | ||
getStrings(key: string): string[]; | ||
getBool(key: string): boolean; | ||
getInt(key: string): number; | ||
getFloat(key: string): number; | ||
set(key: string, value: string): void; | ||
} | ||
export declare class Config implements ConfigInterface { | ||
private static DEFAULT_SECTION; | ||
@@ -3,0 +11,0 @@ private static DEFAULT_COMMENT; |
import * as rbac from '../rbac'; | ||
import { ConfigInterface } from '../config'; | ||
import { Assertion } from './assertion'; | ||
export declare const sectionNameMap: { | ||
[index: string]: string; | ||
}; | ||
export declare const requiredSections: string[]; | ||
export declare class Model { | ||
@@ -15,2 +20,4 @@ model: Map<string, Map<string, Assertion>>; | ||
loadModelFromText(text: string): void; | ||
loadModelFromConfig(cfg: ConfigInterface): void; | ||
private hasSection; | ||
printModel(): void; | ||
@@ -32,1 +39,9 @@ buildRoleLinks(rm: rbac.RoleManager): Promise<void>; | ||
export declare function newModel(...text: string[]): Model; | ||
/** | ||
* newModelFromFile creates a model from a .CONF file. | ||
*/ | ||
export declare function newModelFromFile(path: string): Model; | ||
/** | ||
* newModelFromString creates a model from a string which contains model text. | ||
*/ | ||
export declare function newModelFromString(text: string): Model; |
@@ -30,3 +30,3 @@ "use strict"; | ||
const log_1 = require("../log"); | ||
const sectionNameMap = { | ||
exports.sectionNameMap = { | ||
r: 'request_definition', | ||
@@ -38,2 +38,3 @@ p: 'policy_definition', | ||
}; | ||
exports.requiredSections = ['r', 'p', 'e', 'm']; | ||
class Model { | ||
@@ -47,4 +48,3 @@ /** | ||
loadAssertion(cfg, sec, key) { | ||
// console.log('loadAssertion: ', sec, key); | ||
const secName = sectionNameMap[sec]; | ||
const secName = exports.sectionNameMap[sec]; | ||
const value = cfg.getString(`${secName}::${key}`); | ||
@@ -60,3 +60,2 @@ return this.addDef(sec, key, value); | ||
loadSection(cfg, sec) { | ||
// console.log('loadSection: ', sec); | ||
let i = 1; | ||
@@ -74,3 +73,2 @@ for (;;) { | ||
addDef(sec, key, value) { | ||
// console.log('addDef: ', sec, key. value); | ||
if (value === '') { | ||
@@ -116,9 +114,4 @@ return false; | ||
loadModel(path) { | ||
// console.log('loadModel: ', path); | ||
const cfg = config_1.Config.newConfig(path); | ||
this.loadSection(cfg, 'r'); | ||
this.loadSection(cfg, 'p'); | ||
this.loadSection(cfg, 'e'); | ||
this.loadSection(cfg, 'm'); | ||
this.loadSection(cfg, 'g'); | ||
this.loadModelFromConfig(cfg); | ||
} | ||
@@ -128,8 +121,21 @@ // loadModelFromText loads the model from the text. | ||
const cfg = config_1.Config.newConfigFromText(text); | ||
this.loadSection(cfg, 'r'); | ||
this.loadSection(cfg, 'p'); | ||
this.loadSection(cfg, 'e'); | ||
this.loadSection(cfg, 'm'); | ||
this.loadSection(cfg, 'g'); | ||
this.loadModelFromConfig(cfg); | ||
} | ||
loadModelFromConfig(cfg) { | ||
for (const s in exports.sectionNameMap) { | ||
this.loadSection(cfg, s); | ||
} | ||
const ms = []; | ||
exports.requiredSections.forEach(n => { | ||
if (!this.hasSection(n)) { | ||
ms.push(exports.sectionNameMap[n]); | ||
} | ||
}); | ||
if (ms.length > 0) { | ||
throw new Error(`missing required sections: ${ms.join(',')}`); | ||
} | ||
} | ||
hasSection(sec) { | ||
return this.model.get(sec) !== undefined; | ||
} | ||
// printModel prints the model to the log. | ||
@@ -304,1 +310,19 @@ printModel() { | ||
exports.newModel = newModel; | ||
/** | ||
* newModelFromFile creates a model from a .CONF file. | ||
*/ | ||
function newModelFromFile(path) { | ||
const m = new Model(); | ||
m.loadModel(path); | ||
return m; | ||
} | ||
exports.newModelFromFile = newModelFromFile; | ||
/** | ||
* newModelFromString creates a model from a string which contains model text. | ||
*/ | ||
function newModelFromString(text) { | ||
const m = new Model(); | ||
m.loadModelFromText(text); | ||
return m; | ||
} | ||
exports.newModelFromString = newModelFromString; |
{ | ||
"name": "casbin", | ||
"version": "3.0.7", | ||
"version": "3.0.8", | ||
"description": "An authorization library that supports access control models like ACL, RBAC, ABAC in Node.JS", | ||
@@ -5,0 +5,0 @@ "main": "lib/index.js", |
@@ -30,5 +30,5 @@ # node-Casbin | ||
[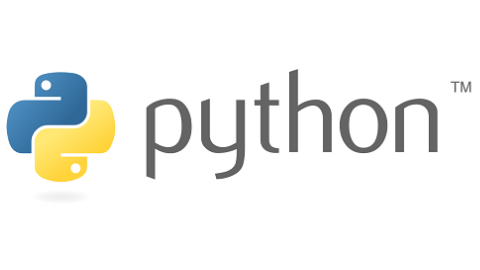](https://github.com/casbin/pycasbin) | [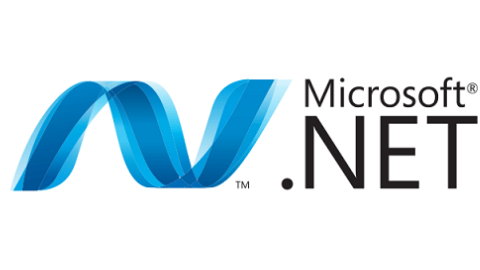](https://github.com/casbin-net/Casbin.NET) | [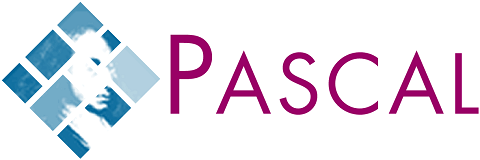](https://github.com/casbin4d/Casbin4D) | [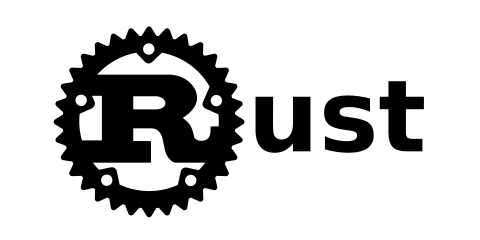](https://github.com/Devolutions/casbin-rs) | ||
[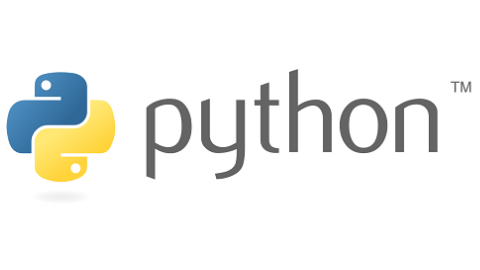](https://github.com/casbin/pycasbin) | [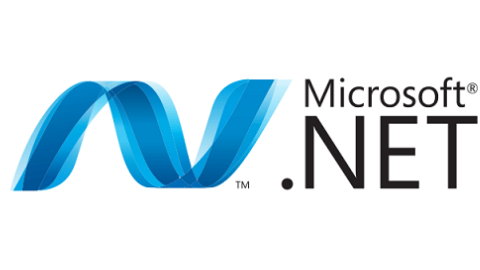](https://github.com/casbin-net/Casbin.NET) | [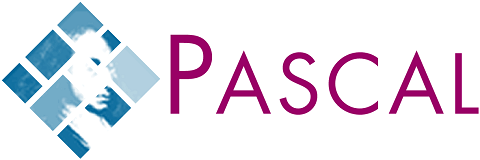](https://github.com/casbin4d/Casbin4D) | [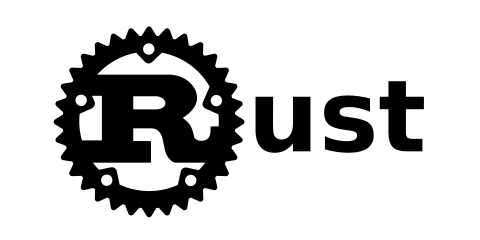](https://github.com/casbin/casbin-rs) | ||
----|----|----|---- | ||
[PyCasbin](https://github.com/casbin/pycasbin) | [Casbin.NET](https://github.com/casbin-net/Casbin.NET) | [Casbin4D](https://github.com/casbin4d/Casbin4D) | [Casbin-RS](https://github.com/Devolutions/casbin-rs) | ||
[PyCasbin](https://github.com/casbin/pycasbin) | [Casbin.NET](https://github.com/casbin-net/Casbin.NET) | [Casbin4D](https://github.com/casbin4d/Casbin4D) | [Casbin-RS](https://github.com/casbin/casbin-rs) | ||
production-ready | production-ready | experimental | WIP | ||
@@ -35,0 +35,0 @@ |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
180922
4118