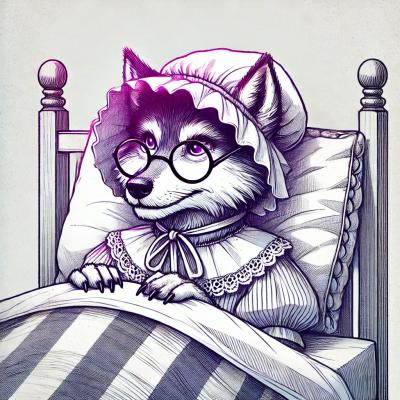
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The catharsis npm package is a JavaScript library for parsing and stringifying JSDoc type expressions. It allows developers to convert JSDoc type expressions into JavaScript objects and vice versa, making it easier to work with type annotations in code documentation.
Parsing JSDoc Type Expressions
This feature allows you to parse JSDoc type expressions into JavaScript objects. The example code parses the JSDoc type expression 'Array.<string>' and converts it into a JavaScript object.
const catharsis = require('catharsis');
const parsedType = catharsis.parse('Array.<string>');
console.log(parsedType);
Stringifying JSDoc Type Expressions
This feature allows you to convert JavaScript objects back into JSDoc type expressions. The example code takes a JavaScript object representing a type and converts it into a JSDoc type expression string.
const catharsis = require('catharsis');
const typeObj = { type: 'NameExpression', name: 'string' };
const typeString = catharsis.stringify(typeObj);
console.log(typeString);
Doctrine is a popular library for parsing JSDoc comments, including type expressions. It provides more comprehensive support for JSDoc comments, including tags and descriptions, making it a more feature-rich alternative to catharsis.
jsdoctypeparser is another library for parsing JSDoc type expressions. It focuses specifically on type expressions, similar to catharsis, but offers a different API and may have different performance characteristics.
A JavaScript parser for Google Closure Compiler and JSDoc type expressions.
Catharsis is designed to be:
var catharsis = require('catharsis');
// Google Closure Compiler parsing
var type = '!Object';
var parsedType;
try {
parsedType = catharsis.parse(type); // {"type":"NameExpression,"name":"Object","nullable":false}
} catch(e) {
console.error('unable to parse %s: %s', type, e);
}
// JSDoc-style type expressions enabled
var jsdocType = 'string[]'; // Closure Compiler expects Array.<string>
var parsedJsdocType;
try {
parsedJsdocType = catharsis.parse(jsdocType, {jsdoc: true});
} catch (e) {
console.error('unable to parse %s: %s', jsdocType, e);
}
// Converting parse results back to type expressions
catharsis.stringify(parsedType); // !Object
catharsis.stringify(parsedJsdocType); // string[]
catharsis.stringify(parsedJsdocType, {restringify: true}); // Array.<string>
// Converting parse results to descriptions of the type expression
catharsis.describe(parsedType).simple; // non-null Object
catharsis.describe(parsedJsdocType).simple; // Array of string
See the test/specs directory for more examples of Catharsis' parse results.
Parse a type expression, and return the parse results. Throws an error if the type expression cannot be parsed.
When called without options, Catharsis attempts to parse type expressions in the same way as
Closure Compiler. When the jsdoc
option is enabled, Catharsis can also parse several kinds of
type expressions that are permitted in JSDoc:
function
is treated as a function type with no parameters.Array.<string>
and
Array<string>
will be parsed in the same way.[]
to a name expression (for example, string[]
) to interpret it as a type
application with the expression Array
(for example, Array.<string>
).#
, ~
, :
, and /
.MyClass(foo, bar)
).type
: A string containing a Closure Compiler type expression.options
: Options for parsing the type expression.
options.jsdoc
: Specifies whether to enable parsing of JSDoc-style type expressions. Defaults
to false
.options.useCache
: Specifies whether to use the cache of parsed types. Defaults to true
.An object containing the parse results. See the test/specs directory for examples of the parse results for different type expressions.
The object also includes two non-enumerable properties:
jsdoc
: A boolean indicating whether the type expression was parsed with JSDoc support enabled.typeExpression
: A string containing the type expression that was parsed.Stringify parsedType
, and return the type expression. If validation is enabled, throws an error if
the stringified type expression cannot be parsed.
parsedType
: An object containing a parsed Closure Compiler type expression.options
: Options for stringifying the parse results.
options.cssClass
: Synonym for options.linkClass
. Deprecated in version 0.8.0; will be
removed in a future version.options.htmlSafe
: Specifies whether to return an HTML-safe string that replaces left angle
brackets (<
) with the corresponding entity (<
). Note: Characters in name expressions
are not escaped.options.linkClass
: A CSS class to add to HTML links. Used only if options.links
is
provided. By default, no CSS class is added.options.links
: An object whose keys are name expressions and whose values are URIs. If a
name expression matches a key in options.links
, the name expression will be wrapped in an
HTML <a>
tag that links to the URI. If options.linkClass
is specified, the <a>
tag will
include a class
attribute. Note: When using this option, parsed types are always
restringified, and the resulting string is not cached.options.restringify
: Forces Catharsis to restringify the parsed type. If this option is not
specified, and the parsed type object includes a typeExpression
property, Catharsis will
return the typeExpression
property without modification when possible. Defaults to false
.options.useCache
: Specifies whether to use the cache of stringified type expressions.
Defaults to true
.options.validate
: Specifies whether to validate the stringified parse results by attempting
to parse them as a type expression. If the stringified results are not parsable by default, you
must also provide the appropriate options to pass to the parse()
method. Defaults to false
.A string containing the type expression.
Convert a parsed type to a description of the type expression. This method is especially useful if your users are not familiar with the syntax for type expressions.
The describe()
method returns the description in two formats:
For example, if you call describe('?function(new:MyObject, string)=')
, it returns the following
object:
{
simple: 'optional nullable function(constructs MyObject, string)',
extended: {
description: 'function(string)',
modifiers: {
functionNew: 'Returns MyObject when called with new.',
functionThis: '',
optional: 'Optional.',
nullable: 'May be null.',
repeatable: ''
},
returns: ''
}
}
parsedType
: An object containing a parsed Closure Compiler type expression.options
: Options for creating the description.
options.codeClass
: A CSS class to add to the tag that is wrapped around type names. Used
only if options.codeTag
is provided. By default, no CSS class is added.options.codeTag
: The name of an HTML tag (for example, code
) to wrap around type names.
For example, if this option is set to code
, the type expression Array.<string>
would have
the simple description <code>Array</code> of <code>string</code>
.options.language
: A string identifying the language in which to generate the description.
The identifier should be an
ISO 639-1 language code (for example,
en
). It can optionally be followed by a hyphen and an
ISO 3166-1 alpha-2 country code (for example,
en-US
). If you use values other than en
, you must provide translation resources in
options.resources
. Defaults to en
.options.linkClass
: A CSS class to add to HTML links. Used only if options.links
is
provided. By default, no CSS class is added.options.links
: An object whose keys are name expressions and whose values are URIs. If a
name expression matches a key in options.links
, the name expression will be wrapped in an
HTML <a>
tag that links to the URI. If options.linkClass
is specified, the <a>
tag will
include a class
attribute. Note: When using this option, the description is not cached.options.resources
: An object that specifies how to describe type expressions for a given
language. The object's property names should use the same format as options.language
. Each
property should contain an object in the same format as the translation resources in
res/en.json. If you specify a value for options.resources.en
, it will override
the defaults in res/en.json.options.useCache
: Specifies whether to use the cache of descriptions. Defaults to true
.An object with the following properties:
simple
: A string that provides a complete description of the type expression.extended
: An object containing details about the outermost type expression.
extended.description
: A string that provides a basic description of the type expression,
excluding the information contained in other properties.extended.modifiers
: Information about modifiers that apply to the type expression.
extended.modifiers.functionNew
: A string describing what a function returns when called
with new
. Used only for function types.extended.modifiers.functionThis
: A string describing what the keyword this
refers to
within a function. Used only for function types.extended.modifiers.nullable
: A string indicating whether the type is nullable or
non-nullable.extended.modifiers.optional
: A string indicating whether the type is optional.extended.modifiers.repeatable
: A string indicating whether the type can be providedextended.returns
: A string describing the function's return value. Used only for function
types.With npm:
npm install catharsis
Or by cloning the git repo:
git clone git://github.com/hegemonic/catharsis.git
cd catharsis
npm install
Take a look at the issue tracker to see what's in store for Catharsis.
Bug reports, feature requests, and pull requests are always welcome! If you're working on a large pull request, please contact me in advance so I can help things go smoothly.
Note: The parse tree's format should not be considered final until Catharsis reaches version 1.0. I'll do my best to provide release notes for any changes.
@
sign (for example,
module:@prefix/mymodule~myCallback
) are now supported.Promise.<string>
).{0: string}
) are now
parsed correctly.{foo:function()}
), are now parsed correctly.foo?=
).number[][]
) are now parsed
correctly when JSDoc-style type expressions are enabled..
) as a separator, regardless
of whether JSDoc-style type expressions are enabled....
)
modifier when JSDoc-style type expressions are enabled.!string|!number
).describe()
method, which converts a parsed type to a description of the type.linkClass
option to the stringify()
method, and deprecated the existing cssClass
option. The cssClass
option will be removed in a future release.README
.undefinedHTML
) are now parsed correctly when JSDoc-style type expressions are enabled....function()
) are now
parsed and stringified correctly....!number
) are now parsed and stringified correctly.constructor
).function[]
when JSDoc-style type
expressions are enabled.?
(nullable) and !
(non-nullable) modifiers. For example, ?string
and string?
are now
treated as equivalent.Foo."bar"
is now parsed correctly....*
) are now parsed correctly.MyClass(2)
).parse()
method now correctly parses name expressions that contain
hyphens.parse()
method now correctly parses function types when JSDoc-style type
expressions are enabled.parse()
method's lenient
option has been renamed to jsdoc
. Note: This change is
not backwards-compatible with previous versions.stringify()
method now accepts cssClass
and links
options, which you can use to
add HTML links to a type expression.stringify()
method no longer caches HTML-safe type expressions as if they were normal
type expressions.stringify()
method's options parameter may now include an options.restringify
property, and the behavior of the options.useCache
property has changed.:
and /
.[]
(for example, string[]
)
will be interpreted as a type application with the expression Array
(for example,
Array.<string>
).parse()
and stringify()
methods now honor all of the specified options.lenient
: A boolean indicating whether the type expression was parsed in lenient mode.typeExpression
: A string containing the original type expression.stringify()
method now honors the useCache
option. If a parsed type includes a
typeExpression
property, and useCache
is not set to false
, the stringified type will be
identical to the original type expression.integer
, are now
parsed correctly.parse()
and stringify()
methods are now synchronous, and the parseSync()
and
stringifySync()
methods have been removed. Note: This change is not backwards-compatible
with previous versions.reservedWord: true
property.new
or this
properties unless the properties are defined
in the type expression. In addition, the new
and this
properties can now use any type
expression.*
), are now parsed and stringified
correctly.null
and undefined
literals with additional properties, such as repeatable
, are now
stringified correctly.stringify()
and stringifySync()
methods, which convert a parsed type to a type
expression.opts
argument to parse()
and parseSync()
methods. Note: The
change to parse()
is not backwards-compatible with previous versions.FAQs
A JavaScript parser for Google Closure Compiler and JSDoc type expressions.
We found that catharsis demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.