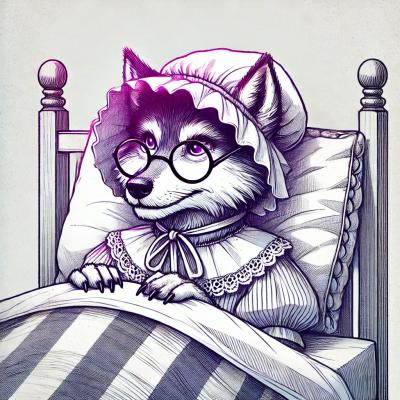
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Encode and parse data in the Concise Binary Object Representation (CBOR) data format (RFC8949).
Encode and parse data in the Concise Binary Object Representation (CBOR) data format (RFC8949).
This package supersedes node-cbor, with the following goals:
This project now only supports versions of Node that the Node team is
currently supporting.
Ava's support
statement
is what we will be using as well. Since the first release will not be soon,
that means Node 18
+ is required.
npm install --save cbor2
See the full API documentation.
Example:
import {decode, diagnose, encode} from 'cbor';
const encoded = encode(true); // Returns Uint8Array(1) [ 245 ]
decode(encoded); // Returns true
// Use integers as keys:
const m = new Map();
m.set(1, 25);
encode(m); // Returns Uint8Array(3) [ 161, 1, 24, 25 ]
diagnose(encode(m)); // {1: 25_0}
If you load cbor2
using import {decode, encode} from 'cbor2';
, all of the
type converters will be loaded automatically. If you import only pieces of
the API and you want the type converters loaded, please also do
import 'cbor2/types';
.
The following types are supported for encoding:
Decoding supports the above types, including the following CBOR tag numbers:
Tag | Generated Type |
---|---|
0 | Date |
1 | Date |
2 | BigInt |
3 | BigInt |
24 | any |
32 | URL |
33 | Tagged |
34 | Tagged |
35 | RegExp |
64 | Uint8Array |
65 | Uint16Array |
66 | Uint32Array |
67 | BigUint64Array |
68 | Uint8ClampedArray |
69 | Uint16Array |
70 | Uint32Array |
71 | BigUint64Array |
72 | Int8Array |
73 | Int16Array |
74 | Int32Array |
75 | BigInt64Array |
77 | Int16Array |
78 | Int32Array |
79 | BigInt64Array |
81 | Float32Array |
82 | Float64Array |
85 | Float32Array |
86 | Float64Array |
258 | Set |
262 | any |
279 | RegExp |
55799 | any |
0xffff | Error |
0xffffffff | Error |
0xffffffffffffffff | Error |
There are several ways to add a new encoder:
toCBOR
methodThis is the easiest approach, if you can modify the class being encoded. Add
a toCBOR()
method to your class, which should return a two-element array
containing the tag number and data item that encodes your class. If the tag
number is undefined
, no tag will be written.
For example:
class Foo {
constructor(one, two) {
this.one = one;
this.two = two;
}
toCBOR() {
return [64000, [this.one, this.two]];
}
}
You can also modify an existing type by monkey-patching a toCBOR
function
onto its prototype, but this isn't recommended.
toJSON()
methodIf your object does not have a toCBOR()
method, but does have a toJSON()
method, the value returned from toJSON()
will be used to serialize the
object.
registerEncoder
Sometimes, you want to support an existing type without modification to that
type. In this case, call registerEncoder(type, encodeFunction)
. The
encodeFunction
takes an object instance and returns the same type as
toCBOR
above:
import {registerEncoder} from 'cbor2/encoder';
class Bar {
constructor() {
this.three = 3;
}
}
registerEncoder(Bar, b => [undefined, b.three]);
Most of the time, you will want to add support for decoding a new tag type. If
the Decoder class encounters a tag it doesn't support, it will generate a Tag
instance that you can handle or ignore as needed. To have a specific type
generated instead, call Tag.registerDecoder()
with the tag number and a function that will convert the tags value to the appropriate type. For the Foo
example above, this might look like:
import {Tag} from 'cbor2/tag';
Tag.registerDecoder(64000, tag => new Foo(tag.contents[0], tag.contents[1]));
You can also replace the default decoders by passing in an appropriate tag function. For example:
// Tag 0 is Date/Time as an ISO-8601 string
import 'cbor2/types';
import {Tag} from 'cbor2/tag';
Tag.registerDecoder(0, ({contents}) => Temporal.Instant.from(contents));
The tests for this package use a set of test vectors from RFC 8949 appendix A
by importing a machine readable version of them from
https://github.com/cbor/test-vectors. For these tests to work, you will need
to use the command git submodule update --init
after cloning or pulling this
code. See the git docs
for more information.
FAQs
Encode and parse data in the Concise Binary Object Representation (CBOR) data format (RFC8949).
The npm package cbor2 receives a total of 251 weekly downloads. As such, cbor2 popularity was classified as not popular.
We found that cbor2 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.