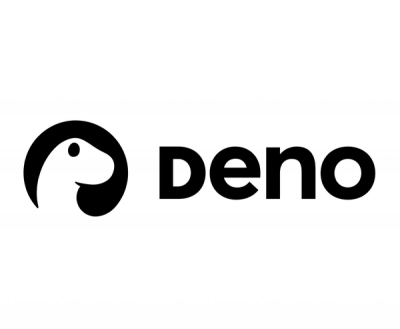
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
cdk-github
Advanced tools
AWS CDK v2 L3 constructs for GitHub.
This project aims to make GitHub's API accessible through CDK with various helper constructs to create resources in GitHub. The target is to replicate most of the functionality of the Terraform GitHub Provider.
Internally AWS CloudFormation custom resources will be used to track GitHub resources (such as Secrets).
JavaScript/TypeScript:
npm install wtfjoke/cdk-github
This library provides the following constructs:
Currently the constructs only support authentication via a GitHub Personal Access Token. The token needs to be a stored in a AWS SecretsManager Secret and passed to the construct as parameter.
import { ActionSecret } from 'cdk-github';
export class ActionSecretStack extends Stack {
constructor(scope: Construct, id: string, props?: StackProps) {
super(scope, id, props);
const sourceSecret = Secret.fromSecretNameV2(this, 'secretToStoreInGitHub', 'testcdkgithub');
const githubTokenSecret = Secret.fromSecretNameV2(this, 'ghSecret', 'GITHUB_TOKEN');
new ActionSecret(this, 'GitHubActionSecret', {
githubTokenSecret,
repositoryName: 'cdk-github',
repositoryOwner: 'wtfjoke',
repositorySecretName: 'aRandomGitHubSecret',
sourceSecret,
});
}
}
See full example in ActionSecretStack
import { ActionEnvironmentSecret } from 'cdk-github';
export class ActionEnvironmentSecretStack extends Stack {
constructor(scope: Construct, id: string, props?: StackProps) {
super(scope, id, props);
const sourceSecret = Secret.fromSecretNameV2(this, 'secretToStoreInGitHub', 'testcdkgithub');
const githubTokenSecret = Secret.fromSecretNameV2(this, 'ghSecret', 'GITHUB_TOKEN');
new ActionEnvironmentSecret(this, 'GitHubActionEnvironmentSecret', {
githubTokenSecret,
environment: 'dev',
repositoryName: 'cdk-github',
repositoryOwner: 'wtfjoke',
repositorySecretName: 'aRandomGitHubSecret',
sourceSecret,
});
}
}
See full example in ActionEnvironmentSecretStack
FAQs
AWS CDK Construct Library to interact with GitHub's API.
We found that cdk-github demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.