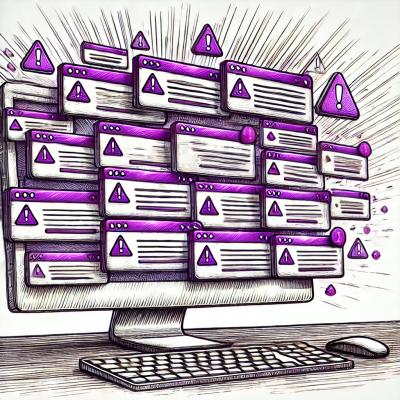
Security News
Combatting Alert Fatigue by Prioritizing Malicious Intent
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
A modern JavaScript utility library delivering modularity, performance, and extras.
Note: A modern JavaScript utility library delivering modularity, performance, and extras.
<script src="https://cdn.jsdelivr.net/npm/celia/celia.min.js"></script>
<script>
// window.celia
celia.each
celia.isArrayLike
celia.isAsyncFunction
celia.isDate
celia.isFalsy
celia.looseEqual
celia.sleep
// ...
// ...
</script>
npm install celia --save
// es6
import { assign, each, getUid, hasOwn, isArrayLike, isUndefined, isWindow, looseEqual, map, noop, sleep, toString, type } from 'celia');
// modularity
import each from 'celia/each';
import isArrayLike from 'celia/isArrayLike';
import isAsyncFunction from 'celia/isAsyncFunction';
import isDate from 'celia/isDate';
import isFalsy from 'celia/isFalsy';
import looseEqual from 'celia/looseEqual';
import sleep from 'celia/sleep';
// ...
// ...
// node
const { assign, each, getUid, hasOwn, isArrayLike, isUndefined, isWindow, looseEqual, map, noop, sleep, toString, type } = require('celia');
// or
const each = require('celia/each');
const isArrayLike = require('celia/isArrayLike');
const isAsyncFunction = require('celia/isAsyncFunction');
const isDate = require('celia/isDate');
const isFalsy = require('celia/isFalsy');
const looseEqual = require('celia/looseEqual');
const sleep = require('celia/sleep');
// ...
// ...
assert(condition, msg)
condition
<Boolean>
msg
<String>
error messageThrows
<Error>
throws Error if condition
is false
assert(1 !== 1, 'assertion error');
// => throw Error: assertion error
value
<Array|ArrayLike|String|Number|Object>
callback
<Function>
context
<any>
optionaleach([1, 2], (val, index)=> {
})
each({ a: 1, b: 2 }, (val, key)=> {
})
each(5, (index, index)=> {
})
each('hello', (c, index)=> {
})
easyHash(value)
value
<any>
Returns
<String>
returns an easy hash valueeasyHash('abc')
// => 'sabc'
easyHash(1)
// => 'n1'
easyHash({})
// => 'o1'
hasOwn(obj, key)
obj
<Object>
key
<any>
Returns
<Boolean>
const obj = { aaa: 111 };
hasOwn(obj, 'test')
// => false
hasOwn(obj, 'aaa')
// => true
looseClone(value)
value
<Object>
Returns
<Object>
const a = { a: 1 };
const b = looseClone(a);
// => a !== b
looseEqual(a, b)
a
<any>
b
<any>
,用于跟 a 比较Returns
<Boolean>
looseEqual(null, undefined)
// => false
looseEqual(null, null)
// => true
looseEqual({ a: 1, b: 2 }, { a: 1, b: 2 })
// => true
looseEqual([1, 2], [1, 2])
// => true
looseEqual(/\d+/, /\d+/)
// => true
looseEqual(new Date(2019, 0, 1, 9, 9, 9), new Date(2019, 0, 1, 9, 9, 9))
// => true
noop(value)
Returns
<Function>
noop()
// => function() {}
sleep(value)
value
<Number>
millisecondsReturns
<Promise>
async function foo() {
await sleep(3000);
}
toString(value)
value
<any>
Returns
<String>
toString({})
// => '[object Object]'
toString([])
// => '[object Array]'
isAbsoluteURL(url)
url
<String>
a request urlReturns
<Boolean>
returns true if the url
is a absolute request urlisAbsoluteURL('/src/isAbsoluteURL.js'))
// => false
isAbsoluteURL('https://github.com'))
// => true
isArrayLike(value)
value
<any>
Returns
<Boolean>
isArrayLike('123')
// => true
isArrayLike(() => { })
// => false
isArrayLike([])
// => true
isArrayLike([1, 2, 3])
// => true
isArrayLike({ 0: 1, length: 1 })
// => true
isAsyncFunction(value)
value
<any>
Returns
<Boolean>
isAsyncFunction(async () => { })
// => true
isAsyncFunction(() => { })
// => false
isBoolean(value)
value
<any>
Returns
<Boolean>
isBoolean(() => { })
// => false
isBoolean(true)
// => true
isDate(value)
value
<any>
Returns
<Boolean>
isDate(new Date())
// => true
isDate({})
// => false
isFalsy(value)
value
<any>
Returns
<Boolean>
isFalsy(false)
// => true
isFalsy(null)
// => true
isFalsy(undefined)
// => true
isFalsy(0)
// => true
isFalsy(NaN)
// => true
isFalsy('')
// => true
isFalsy({})
// => false
isFunction(value)
value
<any>
Returns
<Boolean>
isFunction(async () => { })
// => true
isFunction(() => { })
// => true
isFunction({})
// => false
isInteger(value)
value
<any>
Returns
<Boolean>
isInteger(2)
// => true
isInteger(-2)
// => true
isInteger(1.23)
// => false
isInteger(-1.23)
// => false
isInteger(null)
// => false
isInteger(undefined)
// => false
isInteger('2'));
// => false
isInteger(Infinity)
// => false
isLeapYear(value)
value
<any>
Returns
<Boolean>
isLeapYear(1997)
// => false
isNil(value)
value
<any>
Returns
<Boolean>
isNil(null)
// => true
isNil(undefined)
// => true
isNil({})
// => false
isNumber(value)
value
<any>
Returns
<Boolean>
isNumber(1)
// => true
isNumber(undefined)
// => false
isNumber({})
// => false
isObject(value)
value
<any>
Returns
<Boolean>
isObject(1)
// => false
isObject(undefined)
// => false
isObject({})
// => true
isPlainObject(value)
value
<any>
Returns
<Boolean>
isPlainObject(new Date())
// => false
isPlainObject({})
// => true
isPromiseLike(value)
value
<any>
Returns
<Boolean>
isPromiseLike(null)
// => false
isPromiseLike(undefined)
// => false
isPromiseLike({})
// => false
isPromiseLike(new Promise(() => { }))
// => true
isPromiseLike({ then: () => { }, catch: () => { } })
// => true
isRegExp(value)
value
<any>
Returns
<Boolean>
isRegExp(null)
// => false
isRegExp(undefined)
// => false
isRegExp({})
// => false
isRegExp(Object.create(null))
// => false
isRegExp(/\d+/)
// => true
isString(value)
value
<any>
Returns
<Boolean>
isString(null)
// => false
isString({})
// => false
isString(1)
// => false
isString(true)
// => false
isString('')
// => true
isUndefined(value)
value
<any>
Returns
<Boolean>
isUndefined(null)
// => false
isUndefined(undefined)
// => true
isValidDate(value)
value
<any>
Returns
<Boolean>
isValidDate(null)
// => false
isValidDate(undefined)
// => false
isValidDate(new Date(NaN))
// => false
isValidDate(new Date())
// => true
isWindow(value)
value
<any>
Returns
<Boolean>
isWindow(null)
// => false
isWindow(undefined)
// => false
isWindow(window)
// => true
map(value, callback[, context])
value
<Array|ArrayLike|String|Number|Object>
callback
<Function>
context
<any>
optionalReturns
<Array>
map([1, 2, 3, 4, 5], n => n + 1)
// => [2, 3, 4, 5, 6]
map([1, null, 2, undefined, 3, 4, 5], n => n && (n + 1))
// => [2, 3, 4, 5, 6]
map({ a: 1, b: 2 }, n => n && (n + 1))
// => [2, 3]
transform(value, iterater, accumulator)
value
<any>
iterater
<Function>
accumulator
<any>
initial valueReturns
<any>
const obj = { a: 1, b: 2 };
const newObj = transform(obj, (newObj, value, key) => {
newObj[key] = value + 1;
}, {});
// => { a: 2, b: 3 }
const arr = [1, 2];
const newArr = transform(arr, (newArr, value, key) => {
newArr[key] = value + 1;
}, []);
// => [2, 3]
uid(obj)
obj
<Object>
Returns
<Number>
uid({})
// => 1
uid({})
// => 2
obj
<Object>
mappings
alias({ a: 1, b: 2 }, {
a: 'c',
b: ['e', 'f']
});
// => { a: 1, b: 2, c: 1, e: 2, f: 2 }
assign(value, value2[, ...args])
value
<Object>
value2
<Object>
args
<...Object>
Returns
<Object>
const a = { a: 1 };
const b = { b: 2 };
const c = { c: 3 };
assign(a, b, c);
// => { a: 1, b: 2, c: 3 }
deepAssign(value, value2[, ...args])
value
<Object>
value2
<Object>
args
<...Object>
Returns
<Object>
const a = { a: 1 };
const b = { b: {bb: 11} };
deepAssign(a, b);
// => a.b !== b.b
value
<Object>
callback
<Function>
context
<any>
optionalforIn({ a: 1, b: 2 }, (val, key) => {
});
value
<Object>
callback
<Function>
context
<any>
optionalforOwn({ a: 1, b: 2 }, (val, key) => {
});
get(object, path[, defaultValue])
object
<Object>
path
<String>
defaultValue
<any>
optionalReturns
<any>
const a = { a: [{ b: { c: 3 } }], key: 'value' };
get(null, 'a[0].b.c', 3)
// => 3
get(a, 'a[0].b.c')
// => 3
get(a, 'a["0"].b.c')
// => 3
get(a, 'a.b.c')
// => undefined
get(a, 'a.b.c', 'default')
// => 'default'
get(a, 'key')
// => 'value'
get({ key: null }, 'key')
// => null
object
<Object>
path
<String>
value
<any>
const a = { a: [{ b: { c: 3 } }], key: 'value' };
set(a, 'a[0].b.c', 1);
// => { a: [{ b: { c: 1 } }], key: 'value' }
deepFlat(arr)
arr
<Array>
Returns
<Array>
const arr1 = [1, [2], [], 3, 4, 5];
deepFlat(arr1)
// => [1, 2, 3, 4, 5]
const arr2 = [1, [2, [1, 2, [2, 3]], 3], [], 3, [[1, 2], [[1, 2, 3], 3], [1, 2]], 4, 5];
deepFlat(arr2)
// => [1, 2, 1, 2, 2, 3, 3, 3, 1, 2, 1, 2, 3, 3, 1, 2, 4, 5]
flat(arr[, depth])
arr
<Array>
depth
<Number>
Default: 1flat
<Array>
const arr1 = [1, [2], [], 3, 4, 5];
flat(arr1)
// => [1, 2, 3, 4, 5]
const arr2 = [1, 2, 3, [2, 3, 4], [[1, 2, 3], [3, 4, 5]], 1, 3, 4];
flat(arr2)
// => [1, 2, 3, 2, 3, 4, [1, 2, 3], [3, 4, 5], 1, 3, 4]
forEach(arr, callback[, context])
arr
<Array>
callback
<Function>
context
<any>
OptionalforEach(arr, start, callback[, context])
arr
<Array>
start
<Number>
callback
<Function>
context
<any>
OptionalforEach(arr, start, end, callback[, context])
arr
<Array>
start
<Number>
end
<Number>
callback
<Function>
context
<any>
Optionallet i = 0;
forEach([1, 2, 3], function(num) {
if (num === 1) {
return false;
}
i++;
});
// i === 0
i = 0;
forEach([1, 2, 3, 4, 5], 1, function(num) {
if (num === 3) {
return false;
}
i++;
});
// i === 1
i = 0;
forEach([1, 2, 3, 4, 5], 1, -1, function(num) {
i++;
});
// i === 3
remove(arr, value)
arr
<Array>
value
<any>
Returns
<any>
returns something being removedconst arr = [1, 2, 3, 4, 5];
remove(arr, 2)
// => 2
remove(arr, 9)
// => null
removeAt(arr, index)
arr
<Array>
index
<Number>
Returns
<any>
returns something being removedconst arr = [1, 2, 3, 4, 5];
removeAt(arr, 2)
// => 3
removeAt(arr, 9)
// => null
camelize(value)
value
<String>
Returns
<String>
camelize('-value')
// => 'Value'
camelize('data-value')
// => 'dataValue'
camelize('data-------value')
// => 'dataValue'
camelize('data-attr-value')
// => 'dataAttrValue'
camelize('data--attr--value')
// => 'dataAttrValue'
camelize('data_value')
// => 'dataValue'
camelize('data_______value')
// => 'dataValue'
camelize('data_attr_value')
// => 'dataAttrValue'
camelize('data__attr__value')
// => 'dataAttrValue'
camelize('data value')
// => 'dataValue'
camelize('data value')
// => 'dataValue'
camelize('data attr value')
// => 'dataAttrValue'
camelize('data attr value')
// => 'dataAttrValue'
camelize('data.value')
// => 'dataValue'
camelize('data.......value')
// => 'dataValue'
camelize('data.attr.value')
// => 'dataAttrValue'
camelize('data..attr..value')
// => 'dataAttrValue'
capitalize(value)
value
<String>
Returns
<String>
capitalize('value')
// => 'Value'
base
<String>
arg
<String|Number|Boolean>
pathJoin('https://www.baidu.com', 'path1')
// => 'https://www.baidu.com/path1'
pathJoin('https://www.baidu.com/', 'path1')
// => 'https://www.baidu.com/path1'
pathJoin('https://www.baidu.com', 'path1', 'path2)
// => 'https://www.baidu.com/path1/path2'
val
<String>
obj
<Object>
stringFormat('共{ page }条记录', {})
// => '共{ page }条记录'
stringFormat('共{ page }条记录', { page: 2 })
// => '共2条记录'
obj
<Object>
methodName
<String>
after
<Function>
const obj = {
counter: 2,
increment() {
this.counter++;
}
};
afterCall(obj, 'increment', function (args, ret) {
this.counter = 1;
});
obj.increment();
// 1
obj
<Object>
methodName
<String>
after
<Function>
const obj = {
counter: 2,
increment() {
this.counter++;
}
};
aroundCall(obj, 'increment', function (args, fn) {
this.counter = 2;
fn.apply(this, args);
this.counter = 2;
});
obj.increment();
// 2
obj
<Object>
methodName
<String>
after
<Function>
const obj = {
counter: 2,
increment() {
this.counter++;
}
};
beforeCall(obj, 'increment', function () {
this.counter = 1;
});
obj.increment();
// 2
debounce(func, wait)
func
<Function>
wait
<Number>
Returns
<Function>
async function() {
let i = 0;
const counter = debounce(() => {
i++;
}, 200);
counter();
await sleep(100);
counter();
await sleep(100);
counter();
await sleep(200);
//=> 1 === 1
}
FAQs
A modern JavaScript utility library delivering modularity, performance, and extras.
The npm package celia receives a total of 1,080 weekly downloads. As such, celia popularity was classified as popular.
We found that celia demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.