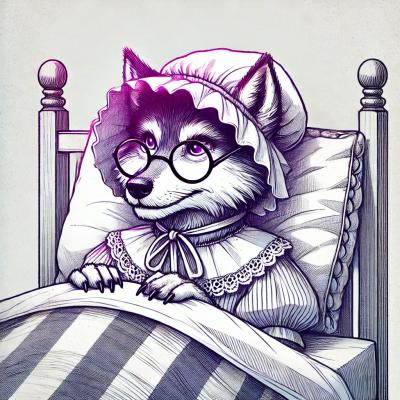
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The cf-env package provides parsing of Cloud Foundry-provided environment variables when you app is running. Provides easy access to your port, http binding host name/ip address, URL of the application, etc. Also provides useful default values when you're running locally.
cfEnv = require("cf-env") // use the cf-env package
pkg = require("./package.json") // read your package.json file
// get the core data using the package name as the default app name
cfCore = cfEnv.getCore({name: pkg.name})
...
// start the server on the given port and binding host, and print
// url to server when it starts
server.listen(cfCore.port, cfCore.bind, function() {
console.log("server starting on " + cfCore.url)
})
This code snippet will get the port, binding host, and full URL to your HTTP server and use them to bind the server and print the server URL when starting.
This package makes use of a number of environment variables that are set when your application is running in Cloud Foundry. These include:
VCAP_SERVICES
VCAP_APPLICATION
PORT
If these aren't set, the getCore()
API will still return useful values,
as appropriate. This means you can use this package in your program and
it will provide useful values when you're running in Cloud Foundry AND
when you're running locally.
The cf-env
package exports the following functions.
getCore(options)
Get the core bits of Cloud Foundry data as an object.
The options
parameter is optional, and can contain the following properties:
name
- name of the application
This value is used as the default name
property in the returned object,
and as the name passed to
the ports package getPort()
function
to get a default port.
protocol
- protocol used in the generated URLs
This value is to override the default protocol used when generating
the URLs in the returned object. It should be the same format as
node's url protocol
property.
This function returns an object with properties:
app
: object version of VCAP_APPLICATION env varservices
: object version of VCAP_SERVICES env varname
: name of the applicationport
: HTTP portbind
: hostname/ip address for bindingurls
: URLs used to access the serversurl
: first URL in urls
If no value can be determined for port
, and the name
property on the
options
parameter is not set,
a port of 3000 will be used.
If no value can be determined for port
, and the name
property on the
options
parameter is set,
that name will be passed to
the ports package getPort()
function
to get a default port.
The protocol used for the URLs will be https:
if the app
is running in Cloud Foundry, and http:
otherwise; you can
force a particular protocol by using the protocol
property
on the options
parameter.
getServices()
Return all services in an object keyed by service name.
Note that this is different than the services
property
returned from getCore()
.
For example, assume VCAP_SERVICES was set to the following:
{
"user-provided": [
{
"name": "cf-env-test",
"label": "user-provided",
"tags": [],
"credentials": {
"database": "database",
"password": "passw0rd",
"url": "https://example.com/",
"username": "userid"
},
"syslog_drain_url": "http://example.com/syslog"
}
]
}
In this case, getCore().services
would be set to that same object, but
getServices()
would return
{
"cf-env-test": {
"name": "cf-env-test",
"label": "user-provided",
"tags": [],
"credentials": {
"database": "database",
"password": "passw0rd",
"url": "https://example.com/",
"username": "userid"
},
"syslog_drain_url": "http://example.com/syslog"
}
}
getService(spec)
Return a service object by name.
The spec
parameter should be a regular expression, or a string which is the
exact name of the service. For a regular expression, the first service name
which matches the regular expression will be returned.
Returns the service object from VCAP_SERVICES or null if not found.
getServiceURL(spec, replacements)
Returns a service URL by name.
The spec
parameter should be a regular expression, or a string which is the
exact name of the service. For a regular expression, the first service name
which matches the regular expression will be returned.
The replacements
parameter is an object with the properties used in
node's url function url.format()
.
Returns a URL generated from VCAP_SERVICES or null if not found.
To generate the URL, processing first starts with a url
property
in the service credentials. You can override the url
property in the
service credentials (if no such property exists), with a replacements
property of url
, and a value which is the name of the property in
the service credentials whose value contains the base URL.
That url is parsed with
node's url function url.parse()
to get a set of initial url properties.
These properties are then overridden by entries in replacements
, using the
following operation, for a given replacement key
and value
.
url[key] = service.credentials[value]
The URL auth
replacement is a bit special, in that it's value should
be a two-element array of [userid, password], where those values are
keys in the service.credentials
For example, assume VCAP_SERVICES was set to the following:
{
"user-provided": [
{
"name": "cf-env-test",
"label": "user-provided",
"tags": [],
"credentials": {
"database": "database",
"password": "passw0rd",
"url": "https://example.com/",
"username": "userid"
},
"syslog_drain_url": "http://example.com/syslog"
}
]
}
Assume you run the following code:
url = cfEnv.getServiceURL("cf-env-test", {
pathname: "database",
auth: ["username", "password"]
})
The url
result will be https://userid:passw0rd@example.com/database
You can push this project as a Cloud Foundry project to try it out.
First, you should edit the manifest.yml
file to use a unique host
value.
Next, do an initial push of the app with cf push
.
Next, you should create a service name cf-env-test
with the following
command:
cf cups cf-env-test -p "url, username, password, database"
Next, you will be prompted for these values; enter something reasonable like:
url> http://example.com
username> userid
password> passw0rd
database> the-db
Next, bind the service with the command:
cf bind-service cf-env-test cf-env-test
Finally, push the app again with cf push
When you visit the site, you'll see the output of various cf-env calls.
If you want to modify the source to play with it, you'll also want to have the
jbuild
program installed.
To install jbuild
on Windows, use the command
npm -g install jbuild
To install jbuild
on Mac or Linux, use the command
sudo npm -g install jbuild
The jbuild
command runs tasks defined in the jbuild.coffee
file. The
task you will most likely use is watch
, which you can run with the
command:
jbuild watch
When you run this command, the application will be built from source, the server
started, and tests run. When you subsequently edit and then save one of the
source files, the application will be re-built, the server re-started, and the
tests re-run. For ever. Use Ctrl-C to exit the jbuild watch
loop.
You can run those build, server, and test tasks separately. Run jbuild
with no arguments to see what tasks are available, along with a short
description of them.
Apache License, Version 2.0
FAQs
THIS PACKGE IS DEPRECATED - USE PACKAGE cfenv INSTEAD
The npm package cf-env receives a total of 0 weekly downloads. As such, cf-env popularity was classified as not popular.
We found that cf-env demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.