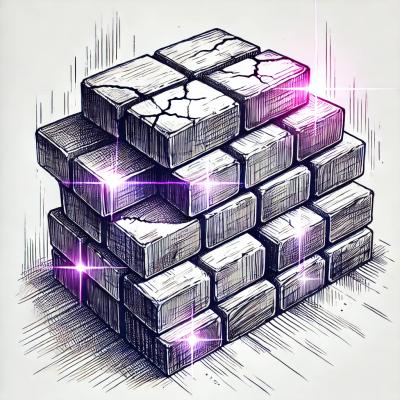
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
chai-exclude
Advanced tools
Exclude keys to compare from a deep equal operation with chai expect and assert
The chai-exclude npm package is a plugin for the Chai assertion library that allows you to exclude specific keys from objects when performing deep equality checks. This is particularly useful when you want to ignore certain properties that may vary or are not relevant to the test.
Exclude specific keys from deep equality checks
This feature allows you to exclude specific keys from deep equality checks. In the example, the key 'c' is excluded from the comparison, so the objects are considered equal even though their 'c' values differ.
const chai = require('chai');
const chaiExclude = require('chai-exclude');
chai.use(chaiExclude);
const expect = chai.expect;
const obj1 = { a: 1, b: 2, c: 3 };
const obj2 = { a: 1, b: 2, c: 4 };
expect(obj1).excluding('c').to.deep.equal(obj2);
Exclude multiple keys from deep equality checks
This feature allows you to exclude multiple keys from deep equality checks. In the example, the keys 'c' and 'd' are excluded from the comparison, so the objects are considered equal even though their 'c' and 'd' values differ.
const chai = require('chai');
const chaiExclude = require('chai-exclude');
chai.use(chaiExclude);
const expect = chai.expect;
const obj1 = { a: 1, b: 2, c: 3, d: 4 };
const obj2 = { a: 1, b: 2, c: 5, d: 6 };
expect(obj1).excluding(['c', 'd']).to.deep.equal(obj2);
Exclude nested keys from deep equality checks
This feature allows you to exclude nested keys from deep equality checks. In the example, the nested key 'b.y' is excluded from the comparison, so the objects are considered equal even though their 'b.y' values differ.
const chai = require('chai');
const chaiExclude = require('chai-exclude');
chai.use(chaiExclude);
const expect = chai.expect;
const obj1 = { a: 1, b: { x: 2, y: 3 } };
const obj2 = { a: 1, b: { x: 2, y: 4 } };
expect(obj1).excluding('b.y').to.deep.equal(obj2);
The deep-equal package provides deep equality comparison for Node.js and browsers. It does not offer the ability to exclude specific keys from the comparison, making chai-exclude more flexible for tests where certain properties should be ignored.
Lodash's isEqual function performs a deep comparison between two values to determine if they are equivalent. While powerful, it does not natively support excluding specific keys from the comparison, which is a feature provided by chai-exclude.
Exclude keys to compare from a deep equal operation with chai expect or assert.
Sometimes you'll need to exclude object properties that generate unique values while doing a deep equal operation. This plugin makes it easier to remove those properties from comparison.
https://github.com/chaijs/chai/issues/885
Works with both array of objects and objects.
npm install chai-exclude --only=dev
yarn add chai-exclude --dev
const chai = require('chai');
const chaiExclude = require('chai-exclude');
chai.use(chaiExclude);
import { use } from 'chai';
import chaiExclude from 'chai-exclude';
use(chaiExclude);
import { use } from 'chai';
import chaiExclude = require('chai-exclude');
use(chaiExclude);
// The typings for chai-exclude are included with the package itself.
// Object
assert.deepEqualExcluding({ a: 'a', b: 'b' }, { b: 'b' }, 'a')
assert.deepEqualExcluding({ a: 'a', b: 'b' }, { a: 'z', b: 'b' }, 'a')
expect({ a: 'a', b: 'b' }).excluding('a').to.deep.equal({ b: 'b' })
expect({ a: 'a', b: 'b' }).excluding('a').to.deep.equal({ a: 'z', b: 'b' })
// Array
assert.deepEqualExcluding([{ a: 'a', b: 'b' }], [{ b: 'b' }], 'a')
assert.deepEqualExcluding([{ a: 'a', b: 'b' }], [{ a: 'z', b: 'b' }], 'a')
expect([{ a: 'a', b: 'b' }]).excluding('a').to.deep.equal([{ b: 'b' }])
expect([{ a: 'a', b: 'b' }]).excluding('a').to.deep.equal([{ a: 'z', b: 'b' }])
const obj = {
a: 'a',
b: 'b',
c: {
d: 'd',
e: 'e'
}
}
// Object
assert.deepEqualExcluding(obj, { b: 'b' }, ['a', 'c'])
assert.deepEqualExcluding(obj, { a: 'z', b: 'b' }, ['a', 'c'])
expect(obj).excluding(['a', 'c']).to.deep.equal({ b: 'b' })
expect(obj).excluding(['a', 'c']).to.deep.equal({ a: 'z', b: 'b' })
const array = [
{
a: 'a',
b: 'b',
c: {
d: 'd',
e: 'e'
}
}
]
// Array
assert.deepEqualExcluding(array, [{ b: 'b' }], ['a', 'c'])
assert.deepEqualExcluding(array, [{ a: 'z', b: 'b' }], ['a', 'c'])
expect(array).excluding(['a', 'c']).to.deep.equal([{ b: 'b' }])
expect(array).excluding(['a', 'c']).to.deep.equal([{ a: 'z', b: 'b' }])
const actualObj = {
a: 'a',
b: 'b',
c: {
a: 'a',
b: {
a: 'a',
d: {
a: 'a',
b: 'b',
d: null
}
}
},
d: ['a', 'c']
}
const actualArray = [actualObj]
const expectedObj = {
a: 'z', // a is excluded from comparison
b: 'b',
c: {
b: {
d: {
b: 'b',
d: null
}
}
},
d: ['a', 'c']
}
const expectedArray = [expectedObj]
// Object
assert.deepEqualExcludingEvery(actualObj, expectedObj, 'a')
expect(actualObj).excludingEvery('a').to.deep.equal(expectedObj)
// Array
assert.deepEqualExcludingEvery(actualArray, expectedArray, 'a')
expect(actualArray).excludingEvery('a').to.deep.equal(expectedArray)
const actualObj = {
a: 'a',
b: 'b',
c: {
a: 'a',
b: {
a: 'a',
d: {
a: 'a',
b: 'b',
d: null
}
}
},
d: ['a', 'c']
}
const actualArray = [actualObj]
const expectedObj = {
b: 'b',
c: {
b: {
}
}
}
const expectedArray = [expectedObj]
// Object
assert.deepEqualExcludingEvery(actualObj, expectedObj, ['a', 'd'])
expect(actualObj).excludingEvery(['a', 'd']).to.deep.equal(expectedObj)
// Array
assert.deepEqualExcludingEvery(actualArray, expectedArray, ['a', 'd'])
expect(actualArray).excludingEvery(['a', 'd']).to.deep.equal(expectedArray)
Contributions are welcome. If you have any questions create an issue here.
FAQs
Exclude keys to compare from a deep equal operation with chai expect and assert
We found that chai-exclude demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.