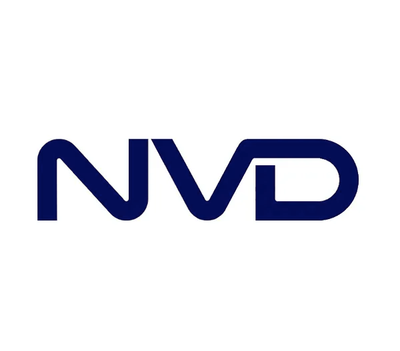
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
chai-http is a plugin for the Chai assertion library that provides an interface for testing HTTP APIs. It allows you to make HTTP requests and assertions about the responses, making it useful for testing RESTful APIs and other web services.
Making HTTP Requests
This feature allows you to make HTTP requests to your server. In this example, a GET request is made to the '/api/resource' endpoint, and assertions are made about the response status and body.
const chai = require('chai');
const chaiHttp = require('chai-http');
const server = require('../app'); // Your server file
const should = chai.should();
chai.use(chaiHttp);
describe('GET /api/resource', () => {
it('it should GET all the resources', (done) => {
chai.request(server)
.get('/api/resource')
.end((err, res) => {
res.should.have.status(200);
res.body.should.be.a('array');
done();
});
});
});
Testing POST Requests
This feature allows you to test POST requests. In this example, a POST request is made to the '/api/resource' endpoint with a sample resource object, and assertions are made about the response status and body.
const chai = require('chai');
const chaiHttp = require('chai-http');
const server = require('../app'); // Your server file
const should = chai.should();
chai.use(chaiHttp);
describe('POST /api/resource', () => {
it('it should POST a new resource', (done) => {
let resource = {
name: 'Sample Resource',
description: 'This is a sample resource'
}
chai.request(server)
.post('/api/resource')
.send(resource)
.end((err, res) => {
res.should.have.status(201);
res.body.should.be.a('object');
res.body.should.have.property('name').eql('Sample Resource');
done();
});
});
});
Testing PUT Requests
This feature allows you to test PUT requests. In this example, a PUT request is made to the '/api/resource/:id' endpoint to update an existing resource, and assertions are made about the response status and body.
const chai = require('chai');
const chaiHttp = require('chai-http');
const server = require('../app'); // Your server file
const should = chai.should();
chai.use(chaiHttp);
describe('PUT /api/resource/:id', () => {
it('it should UPDATE a resource given the id', (done) => {
let resource = new Resource({name: 'Old Resource', description: 'Old description'});
resource.save((err, resource) => {
chai.request(server)
.put('/api/resource/' + resource.id)
.send({name: 'Updated Resource', description: 'Updated description'})
.end((err, res) => {
res.should.have.status(200);
res.body.should.be.a('object');
res.body.should.have.property('name').eql('Updated Resource');
done();
});
});
});
});
Testing DELETE Requests
This feature allows you to test DELETE requests. In this example, a DELETE request is made to the '/api/resource/:id' endpoint to delete an existing resource, and assertions are made about the response status and body.
const chai = require('chai');
const chaiHttp = require('chai-http');
const server = require('../app'); // Your server file
const should = chai.should();
chai.use(chaiHttp);
describe('DELETE /api/resource/:id', () => {
it('it should DELETE a resource given the id', (done) => {
let resource = new Resource({name: 'Resource to be deleted', description: 'This resource will be deleted'});
resource.save((err, resource) => {
chai.request(server)
.delete('/api/resource/' + resource.id)
.end((err, res) => {
res.should.have.status(200);
res.body.should.be.a('object');
res.body.should.have.property('message').eql('Resource successfully deleted');
done();
});
});
});
});
Supertest is a popular library for testing HTTP servers. It provides a high-level abstraction for testing HTTP, making it easy to test APIs. Compared to chai-http, Supertest is more focused on HTTP testing and does not integrate with Chai's assertion library, but it can be used with any assertion library.
Axios is a promise-based HTTP client for the browser and Node.js. While it is not specifically designed for testing, it can be used to make HTTP requests in tests. Compared to chai-http, Axios is more general-purpose and does not provide built-in testing utilities, but it can be combined with assertion libraries like Chai for testing.
Request is a simplified HTTP client for making HTTP requests. It is not specifically designed for testing, but it can be used to make HTTP requests in tests. Compared to chai-http, Request is more general-purpose and does not provide built-in testing utilities, but it can be combined with assertion libraries like Chai for testing.
HTTP integration testing with Chai assertions.
expect
and should
interfacesThis is an addon plugin for the Chai Assertion Library. Install via npm.
npm install chai-http
Use this plugin as you would all other Chai plugins.
import chaiModule from "chai";
import chaiHttp from "chai-http";
const chai = chaiModule.use(chaiHttp);
To use Chai HTTP on a web page, please use the latest v4 version for now.
Chai HTTP provides an interface for live integration testing via superagent. To do this, you must first construct a request to an application or url.
Upon construction you are provided a chainable api that allows you to specify the http VERB request (get, post, etc) that you wish to invoke.
You may use a function (such as an express or connect app) or a node.js http(s) server as the foundation for your request. If the server is not running, chai-http will find a suitable port to listen on for a given test.
Note: This feature is only supported on Node.js, not in web browsers.
chai.request.execute(app)
.get('/')
When passing an app
to request
; it will automatically open the server for
incoming requests (by calling listen()
) and, once a request has been made
the server will automatically shut down (by calling .close()
). If you want to
keep the server open, perhaps if you're making multiple requests, you must call
.keepOpen()
after .request()
, and manually close the server down:
const requester = chai.request.Request(app).keepOpen()
Promise.all([
requester.get('/a'),
requester.get('/b'),
])
.then(responses => { /* ... */ })
.then(() => requester.close())
You may also use a base url as the foundation of your request.
chai.request.execute('http://localhost:8080')
.get('/')
Once a request is created with a given VERB (get, post, etc), you chain on these additional methods to create your request:
Method | Purpose |
---|---|
.set(key, value) | Set request headers |
.send(data) | Set request data (default type is JSON) |
.type(dataType) | Change the type of the data sent from the .send() method (xml, form, etc) |
.attach(field, file, attachment) | Attach a file |
.auth(username, password) | Add auth headers for Basic Authentication |
.query(parmasObject) | Chain on some GET parameters |
Examples:
.set()
// Set a request header
chai.request.execute(app)
.put('/user/me')
.set('Content-Type', 'application/json')
.send({ password: '123', confirmPassword: '123' })
.send()
// Send some JSON
chai.request.execute(app)
.put('/user/me')
.send({ password: '123', confirmPassword: '123' })
.type()
// Send some Form Data
chai.request.execute(app)
.post('/user/me')
.type('form')
.send({
'_method': 'put',
'password': '123',
'confirmPassword': '123'
})
.attach()
// Attach a file
chai.request.execute(app)
.post('/user/avatar')
.attach('imageField', fs.readFileSync('avatar.png'), 'avatar.png')
.auth()
// Authenticate with Basic authentication
chai.request.execute(app)
.get('/protected')
.auth('user', 'pass')
// Authenticate with Bearer Token
chai.request.execute(app)
.get('/protected')
.auth(accessToken, { type: 'bearer' })
.query()
// Chain some GET query parameters
chai.request.execute(app)
.get('/search')
.query({name: 'foo', limit: 10}) // /search?name=foo&limit=10
In the following examples we use Chai's Expect assertion library:
const { expect } = chai;
To make the request and assert on its response, the end
method can be used:
chai.request.execute(app)
.put('/user/me')
.send({ password: '123', confirmPassword: '123' })
.end((err, res) => {
expect(err).to.be.null;
expect(res).to.have.status(200);
});
Because the end
function is passed a callback, assertions are run
asynchronously. Therefore, a mechanism must be used to notify the testing
framework that the callback has completed. Otherwise, the test will pass before
the assertions are checked.
For example, in the Mocha test framework, this is
accomplished using the
done
callback, which signal that the
callback has completed, and the assertions can be verified:
it('fails, as expected', function(done) { // <= Pass in done callback
chai.request.execute('http://localhost:8080')
.get('/')
.end((err, res) => {
expect(res).to.have.status(123);
done(); // <= Call done to signal callback end
});
});
it('succeeds silently!', () => { // <= No done callback
chai.request.execute('http://localhost:8080')
.get('/')
.end((err, res) => {
expect(res).to.have.status(123); // <= Test completes before this runs
});
});
When done
is passed in, Mocha will wait until the call to done()
, or until
the timeout expires. done
also accepts an
error parameter when signaling completion.
If Promise
is available, request()
becomes a Promise capable library -
and chaining of then
s becomes possible:
chai.request.execute(app)
.put('/user/me')
.send({ password: '123', confirmPassword: '123' })
.then((res) => {
expect(res).to.have.status(200);
})
.catch((err) => {
throw err;
});
Sometimes you need to keep cookies from one request, and send them with the
next (for example, when you want to login with the first request, then access an authenticated-only resource later). For this, .request.agent()
is available:
// Log in
const agent = chai.request.agent(app)
agent
.post('/session')
.send({ username: 'me', password: '123' })
.then((res) => {
expect(res).to.have.cookie('sessionid');
// The `agent` now has the sessionid cookie saved, and will send it
// back to the server in the next request:
return agent.get('/user/me')
.then((res) => {
expect(res).to.have.status(200);
});
});
Note: The server started by chai.request.agent(app)
will not automatically close following the test(s). You should call agent.close()
after your tests to ensure your program exits.
The Chai HTTP module provides a number of assertions
for the expect
and should
interfaces.
Assert that a response has a supplied status.
expect(res).to.have.status(200);
Assert that a Response
or Request
object has a header.
If a value is provided, equality to value will be asserted.
You may also pass a regular expression to check.
Note: When running in a web browser, the same-origin policy only allows Chai HTTP to read certain headers, which can cause assertions to fail.
expect(req).to.have.header('x-api-key');
expect(req).to.have.header('content-type', 'text/plain');
expect(req).to.have.header('content-type', /^text/);
Assert that a Response
or Request
object has headers.
Note: When running in a web browser, the same-origin policy only allows Chai HTTP to read certain headers, which can cause assertions to fail.
expect(req).to.have.headers;
Assert that a string represents valid ip address.
expect('127.0.0.1').to.be.an.ip;
expect('2001:0db8:85a3:0000:0000:8a2e:0370:7334').to.be.an.ip;
Assert that a Response
or Request
object has a given content-type.
expect(req).to.be.json;
expect(req).to.be.html;
expect(req).to.be.text;
Assert that a Response
or Request
object has a given charset.
expect(req).to.have.charset('utf-8');
Assert that a Response
object has a redirect status code.
expect(res).to.redirect;
expect(res).to.not.redirect;
Assert that a Response
object redirects to the supplied location.
expect(res).to.redirectTo('http://example.com');
expect(res).to.redirectTo(/^\/search\/results\?orderBy=desc$/);
Assert that a Request
object has a query string parameter with a given
key, (optionally) equal to value
expect(req).to.have.param('orderby');
expect(req).to.have.param('orderby', 'date');
expect(req).to.not.have.param('limit');
Assert that a Request
or Response
object has a cookie header with a
given key, (optionally) equal to value
expect(req).to.have.cookie('session_id');
expect(req).to.have.cookie('session_id', '1234');
expect(req).to.not.have.cookie('PHPSESSID');
expect(res).to.have.cookie('session_id');
expect(res).to.have.cookie('session_id', '1234');
expect(res).to.not.have.cookie('PHPSESSID');
chai-http
is released with semantic-release
using the plugins:
commit-analyzer
to determine the next version from commit messages.release-notes-generator
to summarize release inchangelog
to update the CHANGELOG.md file.github
to publish a GitHub release.git
to commit release assets.npm
to publish to npm.(The MIT License)
Copyright (c) Jake Luer jake@alogicalparadox.com
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
Extend Chai Assertion library with tests for http apis
The npm package chai-http receives a total of 295,066 weekly downloads. As such, chai-http popularity was classified as popular.
We found that chai-http demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.