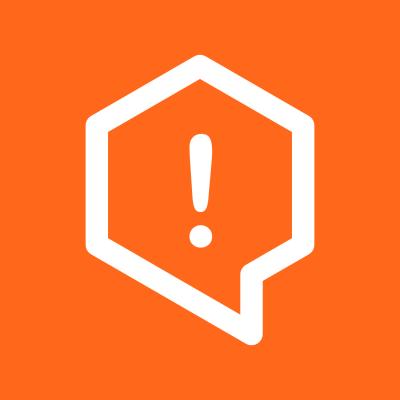
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Nock Chai extends Chai with a language for asserting facts about Nock.
Instead of manually wiring up your expectations to intercepting a nocked request:
const nockedRequest = nock('http://some-url');
nockedRequest.on('request', function(req, interceptor, body) {
expect(body).to.deep.equal({ hello: 'world' });
});
you can write code that expresses what you really mean:
return expect(nock('http://some-url')).to.have.been.requestedWith({
hello: 'world'
});
npm install chai-nock
Then add to your test setup:
const chai = require('chai');
const chaiNock = require('chai-nock');
chai.use(chaiNock);
Asserts that a request has been made to the nock.
it('requested', () => {
const requestNock = nock('http://bbc.co.uk')
.get('/')
.reply(200);
request({
uri: 'http://bbc.co.uk',
});
return expect(requestNock).to.have.been.requested;
});
Asserts that a request has been made to the nock with a body that exactly matches the object provided.
it('requestedWith', () => {
const requestNock = nock('http://bbc.co.uk')
.get('/')
.reply(200);
request({
json: true,
uri: 'http://bbc.co.uk',
body: {
hello: 'world'
}
});
return expect(requestNock).to.have.been.requestedWith({ hello: 'world' });
});
Asserts that a request has been made to the nock with headers that exactly match the object provided.
it('requestedWithHeaders', () => {
const requestNock = nock('http://bbc.co.uk')
.get('/')
.reply(200);
request({
json: true,
uri: 'http://bbc.co.uk',
headers: {
myHeader: 'myHeaderValue'
}
});
return expect(requestNock).to.have.been.requestedWithHeaders({
host: 'bbc.co.uk',
accept: 'application/json',
myHeader: 'myHeaderValue'
});
});
Asserts that a request has been made to the nock with headers that contain the key/value pairs in the object provided.
it('requestedWithHeadersMatch', () => {
const requestNock = nock('http://bbc.co.uk')
.get('/')
.reply(200);
request({
json: true,
uri: 'http://bbc.co.uk',
headers: {
myHeader: 'myHeaderValue',
otherHeader: 'otherHeaderValue'
}
});
return expect(requestNock).to.have.been.requestedWithHeadersMatch({
myHeader: 'myHeaderValue'
});
});
setTimeout
on the chaiNock
object:const chaiNock = require('chai-nock');
chai.use(chaiNock);
// Set a timeout of 10 seconds
chaiNock.setTimeout(10000);
jest.setTimeout(12000);
const { expect } = require('chai');
const nock = require('nock');
const request = require('request-promise-native');
describe('example', () => {
it('test', () => {
const requestNock = nock('http://bbc.co.uk')
.get('/')
.reply(200);
request({
json: true,
uri: 'http://bbc.co.uk',
body: {
hello: 'world'
}
});
return expect(requestNock).to.have.been.requestedWith({ hello: 'world' });
});
});
FAQs
Extends Chai with assertions for the Nock Http mocking framework
We found that chai-nock demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.