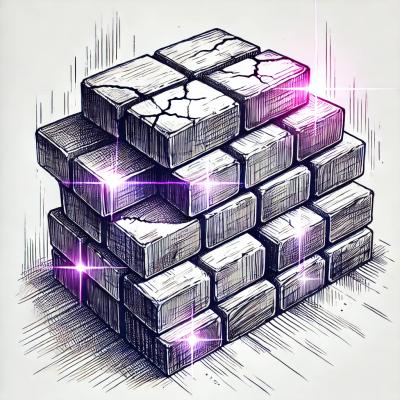
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
chakra-paginator
Advanced tools
npm i chakra-paginator
yarn add chakra-paginator
import React, { FC, ChangeEvent, useEffect, useState, useMemo } from "react";
import {
Grid,
Center,
Select,
ButtonProps,
Text,
Button,
ChakraProvider
} from "@chakra-ui/react";
import {
Paginator,
Container,
Previous,
Next,
PageGroup
} from "chakra-paginator";
const fetchPokemons = (pageSize: number, offset: number) => {
return fetch(
`https://pokeapi.co/api/v2/pokemon?limit=${pageSize}&offset=${offset}`
).then((res) => res.json());
};
const Demo: FC = () => {
// react hooks
const [currentPage, setCurrentPage] = useState<number>(1);
const [pageSize, setPageSize] = useState<number>(10);
const [pokemonsTotal, setPokemonsTotal] = useState<number | undefined>(
undefined
);
const [pokemons, setPokemons] = useState<any[]>([]);
const [isPaginatorDisabled, setIsPaginatorDisabled] = useState<boolean>(
false
);
// constants
const outerLimit = 2;
const innerLimit = 2;
// memos
const offset = useMemo(() => {
return currentPage * pageSize - pageSize;
}, [currentPage, pageSize]);
const pagesQuantity = useMemo(() => {
if (!pokemonsTotal || !pageSize) {
return undefined;
}
return Math.ceil(pokemonsTotal / pageSize);
}, [pokemonsTotal, pageSize]);
// effects
useEffect(() => {
fetchPokemons(pageSize, offset).then((pokemons) => {
setPokemonsTotal(pokemons.count);
setPokemons(pokemons.results);
});
}, [currentPage, pageSize, offset]);
// styles
const baseStyles: ButtonProps = {
w: 7,
fontSize: "sm"
};
const normalStyles: ButtonProps = {
...baseStyles,
_hover: {
bg: "green.300"
},
bg: "red.300"
};
const activeStyles: ButtonProps = {
...baseStyles,
_hover: {
bg: "blue.300"
},
bg: "green.300"
};
const separatorStyles: ButtonProps = {
w: 7,
bg: "green.200"
};
// handlers
const handlePageChange = (nextPage: number) => {
// -> request new data using the page number
setCurrentPage(nextPage);
console.log("request new data with ->", nextPage);
};
const handlePageSizeChange = (event: ChangeEvent<HTMLSelectElement>) => {
const pageSize = Number(event.target.value);
setPageSize(pageSize);
};
const handleDisableClick = () =>
setIsPaginatorDisabled((oldState) => !oldState);
return (
<ChakraProvider>
<Paginator
isDisabled={isPaginatorDisabled}
activeStyles={activeStyles}
innerLimit={innerLimit}
currentPage={currentPage}
outerLimit={outerLimit}
currentPage={currentPage}
normalStyles={normalStyles}
separatorStyles={separatorStyles}
pagesQuantity={pagesQuantity}
onPageChange={handlePageChange}
>
<Container align="center" justify="space-between" w="full" p={4}>
<Previous>
Previous
{/* Or an icon from `react-icons` */}
</Previous>
<PageGroup isInline align="center" />
<Next>
Next
{/* Or an icon from `react-icons` */}
</Next>
</Container>
</Paginator>
<Center w="full">
<Button onClick={handleDisableClick}>Disable ON / OFF</Button>
<Select w={40} ml={3} onChange={handlePageSizeChange}>
<option value="10">10</option>
<option value="25">25</option>
<option value="50">50</option>
</Select>
</Center>
<Grid
templateRows="repeat(2, 1fr)"
templateColumns="repeat(5, 1fr)"
gap={3}
px={20}
mt={20}
>
{pokemons?.map((pokemon) => (
<Center p={4} bg="green.100">
<Text>{pokemon.name}</Text>
</Center>
))}
</Grid>
</ChakraProvider>
);
};
export default Demo;
Prop | Description | Type | Default | Required |
---|---|---|---|---|
pagesQuantity | The total number of pages, calculated based on Backend data | number | 0 | yes |
onPageChange | On change handler which returns the last selected page | (nextPage: number) => void | yes | |
isDisabled | Disables all of the pagination components. You can always disable each individual component via the isDisabled prop, as the components render HTML buttons | boolean | false | no |
activeStyles | The styles of the active page button | ButtonProps | {} | no |
normalStyles | The styles of the inactive page buttons | ButtonProps | {} | no |
separatorStyles | The styles of the separator wrapper | ButtonProps | {} | no |
outerLimit | The amount of pages to show at the start and at the end | number | 0 | no |
innerLimit | The amount of pages to show from the currentPage backwards and forward | number | 0 | no |
currentPage | Manually set the currentPage of the pagination | number | 1 | no |
FAQs
## Deprecation notice: this package has been moved to [@ajna/pagination](https://www.npmjs.com/package/@ajna/pagination)
The npm package chakra-paginator receives a total of 494 weekly downloads. As such, chakra-paginator popularity was classified as not popular.
We found that chakra-paginator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.