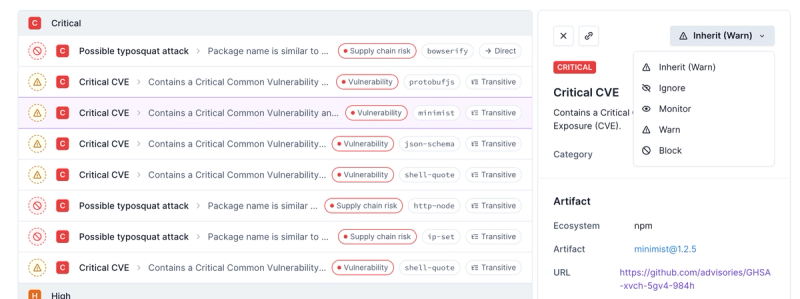
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
chakra-react-select
Advanced tools
Package description
chakra-react-select is a package that integrates the popular react-select library with Chakra UI, providing a set of customizable and accessible select components that are styled using Chakra UI's design system.
Basic Select
This code demonstrates a basic select component using chakra-react-select. It provides a dropdown with options for Chocolate, Strawberry, and Vanilla.
import React from 'react';
import { ChakraProvider } from '@chakra-ui/react';
import { Select } from 'chakra-react-select';
const App = () => (
<ChakraProvider>
<Select
options={[
{ value: 'chocolate', label: 'Chocolate' },
{ value: 'strawberry', label: 'Strawberry' },
{ value: 'vanilla', label: 'Vanilla' }
]}
/>
</ChakraProvider>
);
export default App;
Multi-Select
This code demonstrates a multi-select component using chakra-react-select. It allows users to select multiple options from the dropdown.
import React from 'react';
import { ChakraProvider } from '@chakra-ui/react';
import { Select } from 'chakra-react-select';
const App = () => (
<ChakraProvider>
<Select
isMulti
options={[
{ value: 'chocolate', label: 'Chocolate' },
{ value: 'strawberry', label: 'Strawberry' },
{ value: 'vanilla', label: 'Vanilla' }
]}
/>
</ChakraProvider>
);
export default App;
Custom Styles
This code demonstrates how to apply custom styles to the select component using chakra-react-select. The control and options are styled with custom colors.
import React from 'react';
import { ChakraProvider } from '@chakra-ui/react';
import { Select } from 'chakra-react-select';
const customStyles = {
control: (provided) => ({
...provided,
backgroundColor: 'lightblue'
}),
option: (provided, state) => ({
...provided,
color: state.isSelected ? 'white' : 'black',
backgroundColor: state.isSelected ? 'blue' : 'white'
})
};
const App = () => (
<ChakraProvider>
<Select
styles={customStyles}
options={[
{ value: 'chocolate', label: 'Chocolate' },
{ value: 'strawberry', label: 'Strawberry' },
{ value: 'vanilla', label: 'Vanilla' }
]}
/>
</ChakraProvider>
);
export default App;
react-select is a flexible and customizable select component for React applications. It provides a wide range of features including single and multi-select, async options, and custom styling. chakra-react-select builds on top of react-select by integrating it with Chakra UI's design system.
downshift is a library that provides primitives to build flexible and accessible autocomplete, combobox, and select components. Unlike chakra-react-select, downshift does not come with built-in styles and requires more manual setup for styling and configuration.
react-autosuggest is a library for building autocomplete and autosuggest components in React. It focuses on providing a robust and accessible autocomplete experience. While it offers similar functionality to chakra-react-select, it does not integrate with Chakra UI and requires custom styling.
Readme
This component is a wrapper for the popular react component react-select made using the UI library Chakra UI.
Check out the demo here: https://codesandbox.io/s/chakra-react-select-demo-65ohb?file=/example.js
In order to use this package, you'll need to have @chakra-ui/react
set up like in the guide in their docs. Then install this package:
npm i chakra-react-select
Then you can import the base select package, the async select, the creatable select or the async creatable select:
import {
AsyncCreatableSelect,
AsyncSelect,
CreatableSelect,
Select,
} from "chakra-react-select";
In order to use this component, you can implement it and use it like you would normally use react-select. It should accept almost all of the props that the original takes, with a few additions and exceptions.
size
prop with either sm
, md
, or lg
(default is md
). These will reflect the sizes available on the Chakra <Input />
component (with the exception of xs
because it's too small to work).return (
<>
<Select size="sm" />
<Select size="md" /> {/* Default */}
<Select size="lg" />
</>
);
colorScheme
prop to the select component to change all of the selected options tags' colors. You can view the whole list of available color schemes in the Chakra docs, or if you have a custom color palette, any of the custom color names in that will be available instead.
colorScheme
key to any of your options objects and it will only style that option when selected.return (
<Select
{/* The global color scheme */}
colorScheme="purple"
options={[
{
label: "I am red",
value: "i-am-red",
colorScheme: "red", // The option color scheme overrides the global
},
{
label: "I fallback to purple",
value: "i-am-purple",
},
]}
/>
);
tagVariant
prop with either subtle
, solid
, or outline
(default is subtle
). These will reflect the variant
prop available on the Chakra <Tag />
component.
variant
key to any of your options objects and it will only style that option when selected. This will override the tagVariant
prop on the select if both are setreturn (
<Select
{/* The global variant */}
tagVariant="solid"
options={[
{
label: "I have the outline style",
value: "i-am-outlined",
variant: "outline", // The option variant overrides the global
},
{
label: "I fallback to the global `solid`",
value: "i-am-solid",
},
]}
/>
);
isInvalid
to the select component to style it like the Chakra <Input />
is styled when it receives the same prop.
isInvalid
or isDisabled
to a <FormControl />
which surrounds this component and it will output their corresponding <Input />
styles.return (
<>
{/* This will show up with a red border */}
<Select isInvalid />
{/* This will show up with a red border, and grayed out */}
<FormControl isInvalid isDisabled>
<FormLabel>Invalid & Disabled Select</FormLabel>
<Select />
<FormErrorMessage>
This error message shows because of an invalid FormControl
</FormErrorMessage>
</FormControl>
</>
);
hasStickyGroupHeaders
prop to the select component.
return <Select hasStickyGroupHeaders />;
isFixed: true
to emulate the example in the react-select docs. This will prevent the options which have this flag from having the remove button on its corresponding tag. This only applies when using isMulti
.return (
<Select
isMulti
options={[
{
label: "I can't be removed",
value: "fixed",
isFixed: true,
},
{
label: "I can be removed",
value: "not-fixed",
},
]}
/>
);
v1.3.0
you can now pass the prop selectedOptionStyle
with either "color"
or "check"
(defaults to "color"
). Until this version I had forgotten to style the selected options in the menu for both the single select, or the multi-select with hideSelectedOptions
set to false
. The default option "color"
will style a selected option similar to how react-select does it, by highlighting the selected option in the color blue. Alternatively if you pass "check"
for the value, the selected option will be styled like the Chakra UI Menu component and include a check icon next to the selected option(s). If isMulti
and selectedOptionStyle="check"
are passed, space will only be added for the check marks if hideSelectedOptions={false}
is also passed.return (
<>
<Select selectedOptionStyle="color" /> {/* Default */}
<Select selectedOptionStyle="check" /> {/* Chakra UI Menu Style */}
</>
);
selectedOptionStyle="color"
, you have one additional styling option. If you do not like the default of blue for the highlight color, you can pass the selectedOptionColor
prop to change it. This prop will accept any named color from your color theme, and it will use the 500
value in light mode or the 300
value in dark mode.return (
<>
<Select selectedOptionColor="blue" /> {/* Default */}
<Select selectedOptionColor="purple" />
</>
);
focusBorderColor
and errorBorderColor
can be passed with Chakra color strings which will emulate the respective props being passed to Chakra's <Input />
component.return (
<>
<Select focusBorderColor="green.500" />
<Select errorBorderColor="orange.500" />
</>
);
If you have any other questions or requests, leave it as an issue. I'm sure there are some features of react-select
that I missed and I definitely want to make this wrapper as good as it can be!
There are a few methods of customizing the styles for this package. The first thing to note is that this package does not use the styles
or theme
props that are utilized by the original package. The theme
prop isn't used as most of the base styles are set up to align with your Chakra theme, and customizing your base theme (such as colors or components) should in turn change the styles in this package.
This package does however offer an alternative to the styles
prop, chakraStyles
. It mostly emulates the behavior of the original styles
prop with some slight tweaks, and because its not identical I decided it best to name it differently to avoid confusion.
chakraStyles
In order to use the chakraStyles
prop, first check the documentation for the original styles
prop from the react-select docs. This package offers an identical API for the chakraStyles
prop, however the provided
and output style objects use Chakra's sx
prop instead of the default emotion styles the original package offers. This allows you to both use the shorthand styling props you'd normally use to style Chakra components, as well as tokens from your theme such as named colors.
The API for an individual style function looks like this:
/**
* @param {SystemStyleObject} provided -- The component's default Chakra styles
* @param {Object} state -- The component's current state e.g. `isFocused` (this gives all of the same props that are passed into the component)
* @returns {SystemStyleObject} An output style object which is forwarded to the component's `sx` prop
*/
function option(provided, state) {
return {
...provided,
color: state.isFocused ? "blue.500" : "red.400",
};
}
All of the style keys offered in the original package can be used here, except for input
as that does not allow me to use the chakraStyles
from the select props. The input
styles are also much more dynamic and should be left alone for the most part.
Most of the components rendered by this package use the basic Chakra <Box />
component with a few exceptions. Here are the style keys offered and the corresponding Chakra component that is rendered:
clearIndicator
- CloseButton
container
- Box
control
- Box
(uses theme styles for Chakra's Input
)dropdownIndicator
- Box
(uses theme styles for Chrakra's InputRightAddon
)group
- Box
groupHeading
- Box
(uses theme styles for Chakra's Menu
group title)indicatorsContainer
- Box
indicatorSeparator
- Divider
loadingIndicator
- Spinner
loadingMessage
- Box
menu
- Box
menuList
- Box
(uses theme styles for Chakra's Menu
)multiValue
- Tag
multiValueLabel
- TagLabel
multiValueRemove
- TagCloseButton
noOptionsMessage
- Box
option
- Box
(uses theme styles for Chakra's MenuItem
)placeholder
- Box
singleValue
- Box
valueContainer
- Box
If you're using typescript, define your chakraStyles
object before passing it into your component using the ChakraStylesConfig
type exported from this package:
import { ChakraStylesConfig, Select } from "chakra-react-select";
const App: React.FC = () => {
const chakraStyles: ChakraStylesConfig = {
dropdownIndicator: (provided, state) => ({
...provided,
background: state.isFocused ? "blue.100" : provided.background,
p: 0,
w: "40px",
}),
};
return <Select chakraStyles={chakraStyles} />;
};
As mentioned above, a few of the custom components this package implements either use styles from the global Chakra component theme or are themselves those components. As this package pulls directly from your Chakra theme, any changes you make to those components' themes will propagate to the components in this package. Here is a list of all components that will be affected by changes to your global styles:
react-select component | chakra-ui component styles |
---|---|
ClearIndicator | CloseButton |
Control | Input |
DropdownIndicator | InputRightAddon |
GroupHeading | Menu group title |
IndicatorSeparator | Divider |
LoadingIndicator | Spinner |
MenuList | MenuList |
MultiValueContainer | Tag |
MultiValueLabel | TagLabel |
MultiValueRemove | TagCloseButton |
Option | MenuItem |
In addition to specific component styles, any changes you make to your global color scheme will also be reflected in these custom components.
NOTE: Only make changes to your global component themes if you want them to appear in all instances of that component. Otherwise, just change the individual components' styles using the chakraStyles
prop.
className
This package implements the same classNames on the sub components as the original package so you can use these to style sub-components with CSS. Here is an excerpt from the react-select docs describing how it works:
If you provide the
className
prop to react-select, the SelectContainer will be given a className based on the provided value.If you provide the
classNamePrefix
prop to react-select, all inner elements will be given a className with the provided prefix.For example, given
className='react-select-container'
andclassNamePrefix="react-select"
, the DOM structure is similar to this:
<div class="react-select-container"> <div class="react-select__control"> <div class="react-select__value-container">...</div> <div class="react-select__indicators">...</div> </div> <div class="react-select__menu"> <div class="react-select__menu-list"> <div class="react-select__option">...</div> </div> </div> </div>
While we encourage you to use the new Styles API, you still have the option of styling via CSS classes. This ensures compatibility with styled components, CSS modules and other libraries.
Here is an example of using classNames to style the components: https://codesandbox.io/s/chakra-react-select-classnameprefix-demo-4r2pe?file=/example.js
When submitting a bug report, please include a minimum reproduction of your issue using one of these templates:
Since releasing this project, there have been a few things brought to my attention from users that I would like to update in the near future.
It was brought to my attention in this issue that react-select had a version 5 release almost immediately after I released this package (great timing right 😏). This version is rebuilt in TypeScript so you no longer need to install @types/react-select
to access the types. I made a first pass at upgrading to the new version, and the errors I faced along with some comments I have been receiving from people have made me realize that I probably set up the types incorrectly when I first made this project. I still plan to make the switch soon, however I will need to take a deep dive into the inner workings of react-select's TypeScript support along with how TypeScript works itself, as i am very new to it. If anyone would like to help me make the upgrade/fix the way my types are implemented, I'd greatly appreciate it!
FAQs
A Chakra UI wrapper for the popular library React Select
The npm package chakra-react-select receives a total of 110,525 weekly downloads. As such, chakra-react-select popularity was classified as popular.
We found that chakra-react-select demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.