chartjs-plugin-dragdata
Advanced tools
Comparing version 2.2.5 to 2.3.0
@@ -0,6 +1,12 @@ | ||
/*! | ||
* chartjs-plugin-dragdata v2.3.0 | ||
* https://github.com/artus9033/chartjs-plugin-dragdata.git | ||
* (c) 2018-2024 chartjs-plugin-dragdata contributors | ||
* Released under the MIT license | ||
*/ | ||
(function (global, factory) { | ||
typeof exports === 'object' && typeof module !== 'undefined' ? module.exports = factory(require('chart.js')) : | ||
typeof define === 'function' && define.amd ? define(['chart.js'], factory) : | ||
(global = typeof globalThis !== 'undefined' ? globalThis : global || self, global.index = factory(global.Chart)); | ||
})(this, (function (chart_js) { 'use strict'; | ||
typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports, require('chart.js/helpers'), require('chart.js')) : | ||
typeof define === 'function' && define.amd ? define(['exports', 'chart.js/helpers', 'chart.js'], factory) : | ||
(global = typeof globalThis !== 'undefined' ? globalThis : global || self, factory(global.ChartJSDragDataPlugin = {}, global.Chart.helpers, global.Chart)); | ||
})(this, (function (exports, helpers, chart_js) { 'use strict'; | ||
@@ -1294,248 +1300,425 @@ var noop = {value: () => {}}; | ||
let element, yAxisID, xAxisID, rAxisID, type, stacked, floatingBar, initValue, curDatasetIndex, curIndex, eventSettings; | ||
let isDragging = false; | ||
var ChartJSDragDataPlugin = { | ||
id: "dragdata", | ||
statesStore: new Map(), | ||
afterInit: function dragDataAfterInit(chartInstance) { | ||
var state = { | ||
curIndex: undefined, | ||
curDatasetIndex: undefined, | ||
element: null, | ||
eventSettings: false, | ||
floatingBar: false, | ||
initValue: 0, | ||
xAxisID: "", | ||
yAxisID: "", | ||
rAxisID: "", | ||
stacked: false, | ||
type: undefined, | ||
isDragging: false, | ||
}; | ||
ChartJSDragDataPlugin.statesStore.set(chartInstance.id, state); | ||
select(chartInstance.canvas).call(drag() | ||
.container(chartInstance.canvas) | ||
.on("start", function (e) { | ||
return getElement(e.sourceEvent, chartInstance, state); | ||
}) | ||
.on("drag", function (e) { return updateData(e.sourceEvent, chartInstance, state); }) | ||
.on("end", function (e) { | ||
return dragEndCallback(e.sourceEvent, chartInstance, state); | ||
})); | ||
}, | ||
beforeEvent: function dragDataBeforeEvent(chartInstance) { | ||
var _a; | ||
var state = ChartJSDragDataPlugin.statesStore.get(chartInstance.id); | ||
if (state === null || state === void 0 ? void 0 : state.isDragging) { | ||
(_a = chartInstance.tooltip) === null || _a === void 0 ? void 0 : _a.update(); | ||
return false; | ||
} | ||
}, | ||
}; | ||
// TODO: in a future major release, stop auto-registering the plugin and require users to manually register it | ||
// see https://chartjs-plugin-datalabels.netlify.app/guide/getting-started.html#registration | ||
chart_js.Chart.register(ChartJSDragDataPlugin); | ||
function getSafe(func) { | ||
try { | ||
return func() | ||
} catch (e) { | ||
return '' | ||
} | ||
function roundValue(value, pos) { | ||
if (pos === undefined || isNaN(pos) || pos < 0) | ||
return value; | ||
return Math.round(value * Math.pow(10, pos)) / Math.pow(10, pos); | ||
} | ||
const getElement = (e, chartInstance, callback) => { | ||
element = chartInstance.getElementsAtEventForMode(e, 'nearest', { intersect: true }, false)[0]; | ||
type = chartInstance.config.type; | ||
/** | ||
* Clips a value between a minimum and maximum value. | ||
* @param value the value to be clipped | ||
* @param min the minimum value | ||
* @param max the maximum value | ||
* @returns value in range [min, max] | ||
*/ | ||
function clipValue(value, min, max) { | ||
return Math.min(Math.max(value, min), max); | ||
} | ||
if (element) { | ||
let datasetIndex = element.datasetIndex; | ||
let index = element.index; | ||
// save element settings | ||
eventSettings = getSafe(() => chartInstance.config.options.plugins.tooltip.animation); | ||
function calcRadialLinear(event, chartInstance, curIndex, rAxisID, state) { | ||
var _a, _b, _c; | ||
if (state === void 0) { state = ChartJSDragDataPlugin.statesStore.get(chartInstance.id); } | ||
var _d = helpers.getRelativePosition(event, chartInstance), cursorX = _d.x, cursorY = _d.y; | ||
var rScale = chartInstance.scales[rAxisID]; | ||
var axisAngleRad = rScale.getPointPositionForValue( | ||
// the radar chart has points draggable along primary axes that are aligned with | ||
// scales' lines; the polarArea chart, however, is draggable along lines placed in the center | ||
// between major lines, thus the +0.5 of index is added for the helper to calculate the angle | ||
// of this center guide line (the helper accept a continuous argument, in spite of the name "index") | ||
curIndex + ((state === null || state === void 0 ? void 0 : state.type) === "polarArea" ? 0.5 : 0), chartInstance.scales[rAxisID].max).angle; | ||
var xCenter = rScale.xCenter, yCenter = rScale.yCenter; | ||
// we calculate the dot product of the vector from center to cursor & the axis direction vector | ||
// center-to-cursor vector v | ||
var vx = cursorX - xCenter; | ||
var vy = cursorY - yCenter; | ||
// axis direction vector d | ||
var dx = Math.cos(axisAngleRad); | ||
var dy = Math.sin(axisAngleRad); | ||
// dot product of v & d | ||
var dotProduct = vx * dx + vy * dy; | ||
var d = | ||
// if dot product <= 0, then the point is on the opposite side of the center than the direction of the axis | ||
dotProduct > 0 | ||
? // Euclidean distance between cursor & center | ||
Math.sqrt(Math.pow(cursorX - xCenter, 2) + Math.pow(cursorY - yCenter, 2)) | ||
: 0; | ||
// calculate the value from distance | ||
var v = rScale.getValueForDistanceFromCenter(d); | ||
// apply rounding | ||
v = roundValue(v, (_c = (_b = (_a = chartInstance.config.options) === null || _a === void 0 ? void 0 : _a.plugins) === null || _b === void 0 ? void 0 : _b.dragData) === null || _c === void 0 ? void 0 : _c.round); | ||
v = clipValue(v, chartInstance.scales[rAxisID].min, chartInstance.scales[rAxisID].max); | ||
return v; | ||
} | ||
const dataset = chartInstance.data.datasets[datasetIndex]; | ||
const datasetMeta = chartInstance.getDatasetMeta(datasetIndex); | ||
let curValue = dataset.data[index]; | ||
// get the id of the datasets scale | ||
xAxisID = datasetMeta.xAxisID; | ||
yAxisID = datasetMeta.yAxisID; | ||
rAxisID = datasetMeta.rAxisID; | ||
// check if dragging the dataset or datapoint is prohibited | ||
if (dataset.dragData === false || | ||
(chartInstance.config.options.scales[xAxisID] && chartInstance.config.options.scales[xAxisID].dragData === false) || | ||
(chartInstance.config.options.scales[yAxisID] && chartInstance.config.options.scales[yAxisID].dragData === false) || | ||
(chartInstance.config.options.scales[rAxisID] && chartInstance.config.options.scales[rAxisID].rAxisID === false) || | ||
dataset.data[element.index].dragData === false | ||
) { | ||
element = null; | ||
return | ||
} | ||
/****************************************************************************** | ||
Copyright (c) Microsoft Corporation. | ||
if (type === 'bar') { | ||
stacked = chartInstance.config.options.scales[xAxisID].stacked; | ||
Permission to use, copy, modify, and/or distribute this software for any | ||
purpose with or without fee is hereby granted. | ||
// if a bar has a data point that is an array of length 2, it's a floating bar | ||
const samplePoint = chartInstance.data.datasets[0].data[0]; | ||
floatingBar = (samplePoint !== null) && Array.isArray(samplePoint) && samplePoint.length == 2; | ||
let newPos = calcPosition(e, chartInstance, datasetIndex, index); | ||
initValue = newPos - curValue; | ||
} | ||
THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH | ||
REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY | ||
AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT, | ||
INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM | ||
LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR | ||
OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR | ||
PERFORMANCE OF THIS SOFTWARE. | ||
***************************************************************************** */ | ||
/* global Reflect, Promise, SuppressedError, Symbol */ | ||
// disable the tooltip animation | ||
if (chartInstance.config.options.plugins.dragData.showTooltip === undefined || chartInstance.config.options.plugins.dragData.showTooltip) { | ||
if (!chartInstance.config.options.plugins.tooltip) chartInstance.config.options.plugins.tooltip = {}; | ||
chartInstance.config.options.plugins.tooltip.animation = false; | ||
} | ||
if (typeof callback === 'function' && element) { | ||
if (callback(e, datasetIndex, index, curValue) === false) { | ||
element = null; | ||
} | ||
} | ||
} | ||
var __assign = function() { | ||
__assign = Object.assign || function __assign(t) { | ||
for (var s, i = 1, n = arguments.length; i < n; i++) { | ||
s = arguments[i]; | ||
for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) t[p] = s[p]; | ||
} | ||
return t; | ||
}; | ||
return __assign.apply(this, arguments); | ||
}; | ||
function roundValue(value, pos) { | ||
if (!isNaN(pos)) { | ||
return Math.round(value * Math.pow(10, pos)) / Math.pow(10, pos) | ||
} | ||
return value | ||
function __spreadArray(to, from, pack) { | ||
if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) { | ||
if (ar || !(i in from)) { | ||
if (!ar) ar = Array.prototype.slice.call(from, 0, i); | ||
ar[i] = from[i]; | ||
} | ||
} | ||
return to.concat(ar || Array.prototype.slice.call(from)); | ||
} | ||
function calcRadar(e, chartInstance) { | ||
let x, y, v; | ||
if (e.touches) { | ||
x = e.touches[0].clientX - chartInstance.canvas.getBoundingClientRect().left; | ||
y = e.touches[0].clientY - chartInstance.canvas.getBoundingClientRect().top; | ||
} else { | ||
x = e.clientX - chartInstance.canvas.getBoundingClientRect().left; | ||
y = e.clientY - chartInstance.canvas.getBoundingClientRect().top; | ||
} | ||
let rScale = chartInstance.scales[rAxisID]; | ||
let d = Math.sqrt(Math.pow(x - rScale.xCenter, 2) + Math.pow(y - rScale.yCenter, 2)); | ||
let scalingFactor = rScale.drawingArea / (rScale.max - rScale.min); | ||
if (rScale.options.ticks.reverse) { | ||
v = rScale.max - (d / scalingFactor); | ||
} else { | ||
v = rScale.min + (d / scalingFactor); | ||
} | ||
typeof SuppressedError === "function" ? SuppressedError : function (error, suppressed, message) { | ||
var e = new Error(message); | ||
return e.name = "SuppressedError", e.error = error, e.suppressed = suppressed, e; | ||
}; | ||
v = roundValue(v, chartInstance.config.options.plugins.dragData.round); | ||
v = v > chartInstance.scales[rAxisID].max ? chartInstance.scales[rAxisID].max : v; | ||
v = v < chartInstance.scales[rAxisID].min ? chartInstance.scales[rAxisID].min : v; | ||
return v | ||
function cloneDataPoint(source) { | ||
if (Array.isArray(source)) | ||
return __spreadArray([], source, true); | ||
else if (typeof source === "object") | ||
return __assign({}, source); | ||
// below: typeof source === "number" | ||
return source; | ||
} | ||
function calcPosition(e, chartInstance, datasetIndex, index, data) { | ||
let x, y; | ||
const dataPoint = chartInstance.data.datasets[datasetIndex].data[index]; | ||
if (e.touches) { | ||
x = chartInstance.scales[xAxisID].getValueForPixel(e.touches[0].clientX - chartInstance.canvas.getBoundingClientRect().left); | ||
y = chartInstance.scales[yAxisID].getValueForPixel(e.touches[0].clientY - chartInstance.canvas.getBoundingClientRect().top); | ||
} else { | ||
x = chartInstance.scales[xAxisID].getValueForPixel(e.clientX - chartInstance.canvas.getBoundingClientRect().left); | ||
y = chartInstance.scales[yAxisID].getValueForPixel(e.clientY - chartInstance.canvas.getBoundingClientRect().top); | ||
} | ||
x = roundValue(x, chartInstance.config.options.plugins.dragData.round); | ||
y = roundValue(y, chartInstance.config.options.plugins.dragData.round); | ||
x = x > chartInstance.scales[xAxisID].max ? chartInstance.scales[xAxisID].max : x; | ||
x = x < chartInstance.scales[xAxisID].min ? chartInstance.scales[xAxisID].min : x; | ||
y = y > chartInstance.scales[yAxisID].max ? chartInstance.scales[yAxisID].max : y; | ||
y = y < chartInstance.scales[yAxisID].min ? chartInstance.scales[yAxisID].min : y; | ||
if (floatingBar) { | ||
// x contains the new value for one end of the floating bar | ||
// dataPoint contains the old interval [left, right] of the floating bar | ||
// calculate difference between the new value and both sides | ||
// the side with the smallest difference from the new value was the one that was dragged | ||
// return an interval with new value on the dragged side and old value on the other side | ||
let newVal; | ||
// choose the right variable based on the orientation of the graph(vertical, horizontal) | ||
if (chartInstance.config.options.indexAxis === 'y') { | ||
newVal = x; | ||
} else { | ||
newVal = y; | ||
function calcCartesian(event, chartInstance, data, _a, state) { | ||
var _b, _c, _d, _e, _f; | ||
var xAxisDraggingDisabled = _a.xAxisDraggingDisabled, yAxisDraggingDisabled = _a.yAxisDraggingDisabled; | ||
if (state === void 0) { state = ChartJSDragDataPlugin.statesStore.get(chartInstance.id); } | ||
if (!state) | ||
return data; | ||
var dataPoint = cloneDataPoint(data); | ||
var _g = helpers.getRelativePosition(event, chartInstance), cursorX = _g.x, cursorY = _g.y; | ||
var x = chartInstance.scales[state.xAxisID].getValueForPixel(cursorX); | ||
var y = chartInstance.scales[state.yAxisID].getValueForPixel(cursorY); | ||
var rounding = (_d = (_c = (_b = chartInstance.config.options) === null || _b === void 0 ? void 0 : _b.plugins) === null || _c === void 0 ? void 0 : _c.dragData) === null || _d === void 0 ? void 0 : _d.round; | ||
x = roundValue(x, rounding); | ||
y = roundValue(y, rounding); | ||
x = clipValue(x, chartInstance.scales[state.xAxisID].min, chartInstance.scales[state.xAxisID].max); | ||
y = clipValue(y, chartInstance.scales[state.yAxisID].min, chartInstance.scales[state.yAxisID].max); | ||
if (state.floatingBar) { | ||
// x contains the new value for one end of the floating bar | ||
// dataPoint contains the old interval [left, right] of the floating bar | ||
// calculate difference between the new value and both sides | ||
// the side with the smallest difference from the new value was the one that was dragged | ||
// return an interval with new value on the dragged side and old value on the other side | ||
var newVal = void 0; | ||
// choose the right variable based on the orientation of the graph (vertical, horizontal) | ||
if (((_e = chartInstance.config.options) === null || _e === void 0 ? void 0 : _e.indexAxis) === "y") { | ||
newVal = x; | ||
} | ||
else { | ||
newVal = y; | ||
} | ||
var diffFromLeft = Math.abs(newVal - dataPoint[0]); | ||
var diffFromRight = Math.abs(newVal - dataPoint[1]); | ||
if (diffFromLeft <= diffFromRight) { | ||
dataPoint[0] = newVal; | ||
} | ||
else { | ||
dataPoint[1] = newVal; | ||
} | ||
return dataPoint; | ||
} | ||
const diffFromLeft = Math.abs(newVal - dataPoint[0]); | ||
const diffFromRight = Math.abs(newVal - dataPoint[1]); | ||
if (dataPoint.x !== undefined && !xAxisDraggingDisabled) { | ||
dataPoint.x = x; | ||
} | ||
if (dataPoint.y !== undefined) { | ||
if (!yAxisDraggingDisabled) { | ||
dataPoint.y = y; | ||
} | ||
return dataPoint; | ||
} | ||
else { | ||
if (((_f = chartInstance.config.options) === null || _f === void 0 ? void 0 : _f.indexAxis) === "y") { | ||
if (!xAxisDraggingDisabled) { | ||
return x; | ||
} | ||
else { | ||
return dataPoint; | ||
} | ||
} | ||
else { | ||
if (!yAxisDraggingDisabled) { | ||
return y; | ||
} | ||
else { | ||
return dataPoint; | ||
} | ||
} | ||
} | ||
} | ||
if (diffFromLeft <= diffFromRight) { | ||
return [newVal, dataPoint[1]] | ||
} else { | ||
return [dataPoint[0], newVal] | ||
/** | ||
* Updates values to the nearest values | ||
* @param chartInstance the chart instance | ||
* @param datasetIndex the dataset index | ||
* @param index the data point index | ||
* @returns value after applying magnet or unchanged if not magnet is configured | ||
*/ | ||
function applyMagnet(chartInstance, datasetIndex, index) { | ||
var _a, _b; | ||
var pluginOptions = (_b = (_a = chartInstance.config.options) === null || _a === void 0 ? void 0 : _a.plugins) === null || _b === void 0 ? void 0 : _b.dragData; | ||
if (pluginOptions === null || pluginOptions === void 0 ? void 0 : pluginOptions.magnet) { | ||
var magnet = pluginOptions === null || pluginOptions === void 0 ? void 0 : pluginOptions.magnet; | ||
if (typeof magnet.to === "function") { | ||
var data = chartInstance.data.datasets[datasetIndex].data[index]; | ||
data = magnet.to(data); | ||
chartInstance.data.datasets[datasetIndex].data[index] = data; | ||
chartInstance.update("none"); | ||
return data; | ||
} | ||
return null; | ||
} | ||
} | ||
else { | ||
return chartInstance.data.datasets[datasetIndex].data[index]; | ||
} | ||
} | ||
if (dataPoint.x !== undefined && chartInstance.config.options.plugins.dragData.dragX) { | ||
dataPoint.x = x; | ||
} | ||
if (dataPoint.y !== undefined) { | ||
if (chartInstance.config.options.plugins.dragData.dragY !== false) { | ||
dataPoint.y = y; | ||
} | ||
return dataPoint | ||
} else { | ||
if (chartInstance.config.options.indexAxis === 'y') { | ||
return x | ||
} else { | ||
return y | ||
function checkDraggingConfiguration(chartInstance, datasetIndex, dataPointIndex, state) { | ||
var _a, _b, _c, _d, _e, _f, _g, _h, _j, _k, _l, _m, _o, _p, _q; | ||
if (state === void 0) { state = ChartJSDragDataPlugin.statesStore.get(chartInstance.id); } | ||
if (!state) | ||
return { | ||
chartDraggingDisabled: true, | ||
datasetDraggingDisabled: true, | ||
xAxisDraggingDisabled: true, | ||
yAxisDraggingDisabled: true, | ||
dataPointDraggingDisabled: true, | ||
}; | ||
var dataset = chartInstance.data.datasets[datasetIndex]; | ||
/** per-chart option */ | ||
var chartDraggingDisabled = ((_b = (_a = chartInstance.config.options) === null || _a === void 0 ? void 0 : _a.plugins) === null || _b === void 0 ? void 0 : _b.dragData) === false; | ||
/** per-dataset option */ | ||
var datasetDraggingDisabled = chartDraggingDisabled || dataset.dragData === false; | ||
/** x-axis option (per-axis); dragging on the x-axis is disabled by default */ | ||
var _xAxisDraggingPerAxisOptionValue = (_e = (_d = (_c = chartInstance.config.options) === null || _c === void 0 ? void 0 : _c.scales) === null || _d === void 0 ? void 0 : _d[state.xAxisID]) === null || _e === void 0 ? void 0 : _e.dragData; | ||
/** x-axis option (per-axis); dragging on the x-axis is disabled by default */ | ||
var xAxisDraggingDisabled = true; | ||
if (!datasetDraggingDisabled && | ||
(_xAxisDraggingPerAxisOptionValue === true || // finally, dragging can be enabled on the x-axis by the plugin options, | ||
// unless it's explicitly disabled in x-axis options | ||
(((_h = (_g = (_f = chartInstance.config.options) === null || _f === void 0 ? void 0 : _f.plugins) === null || _g === void 0 ? void 0 : _g.dragData) === null || _h === void 0 ? void 0 : _h.dragX) === true && | ||
_xAxisDraggingPerAxisOptionValue !== false))) { | ||
xAxisDraggingDisabled = false; | ||
} | ||
} | ||
/** y-axis option (per-axis); dragging on the y-axis is enabled by default */ | ||
var yAxisDraggingDisabled = datasetDraggingDisabled || | ||
((_l = (_k = (_j = chartInstance.config.options) === null || _j === void 0 ? void 0 : _j.plugins) === null || _k === void 0 ? void 0 : _k.dragData) === null || _l === void 0 ? void 0 : _l.dragY) === false || | ||
((_p = (_o = (_m = chartInstance.config.options) === null || _m === void 0 ? void 0 : _m.scales) === null || _o === void 0 ? void 0 : _o[state.yAxisID]) === null || _p === void 0 ? void 0 : _p.dragData) === false; | ||
/** per-data-point option */ | ||
var dataPointDraggingDisabled = datasetDraggingDisabled || | ||
((_q = dataset.data[dataPointIndex]) === null || _q === void 0 ? void 0 : _q.dragData) === | ||
false; | ||
return { | ||
chartDraggingDisabled: chartDraggingDisabled, | ||
datasetDraggingDisabled: datasetDraggingDisabled, | ||
xAxisDraggingDisabled: xAxisDraggingDisabled, | ||
yAxisDraggingDisabled: yAxisDraggingDisabled, | ||
dataPointDraggingDisabled: dataPointDraggingDisabled, | ||
}; | ||
} | ||
const updateData = (e, chartInstance, pluginOptions, callback) => { | ||
if (element) { | ||
curDatasetIndex = element.datasetIndex; | ||
curIndex = element.index; | ||
isDragging = true; | ||
let dataPoint = chartInstance.data.datasets[curDatasetIndex].data[curIndex]; | ||
if (type === 'radar' || type === 'polarArea') { | ||
dataPoint = calcRadar(e, chartInstance); | ||
} else if (stacked) { | ||
let cursorPos = calcPosition(e, chartInstance, curDatasetIndex, curIndex); | ||
dataPoint = roundValue(cursorPos - initValue, pluginOptions.round); | ||
} else if (floatingBar) { | ||
dataPoint = calcPosition(e, chartInstance, curDatasetIndex, curIndex); | ||
} else { | ||
dataPoint = calcPosition(e, chartInstance, curDatasetIndex, curIndex); | ||
function dragEndCallback(event, chartInstance, state) { | ||
var _a, _b, _c, _d, _e; | ||
if (state === void 0) { state = ChartJSDragDataPlugin.statesStore.get(chartInstance.id); } | ||
if (!state) | ||
return; | ||
var callback = (_c = (_b = (_a = chartInstance.options) === null || _a === void 0 ? void 0 : _a.plugins) === null || _b === void 0 ? void 0 : _b.dragData) === null || _c === void 0 ? void 0 : _c.onDragEnd; | ||
state.curIndex = undefined; | ||
state.isDragging = false; | ||
// re-enable the tooltip animation | ||
if ((_e = (_d = chartInstance.config.options) === null || _d === void 0 ? void 0 : _d.plugins) === null || _e === void 0 ? void 0 : _e.tooltip) { | ||
chartInstance.config.options.plugins.tooltip.animation = | ||
state.eventSettings; | ||
chartInstance.update("none"); | ||
} | ||
if (!callback || (typeof callback === 'function' && callback(e, curDatasetIndex, curIndex, dataPoint) !== false)) { | ||
chartInstance.data.datasets[curDatasetIndex].data[curIndex] = dataPoint; | ||
chartInstance.update('none'); | ||
if (typeof callback === "function" && state.element) { | ||
var datasetIndex = state.element.datasetIndex; | ||
var index = state.element.index; | ||
var value = applyMagnet(chartInstance, datasetIndex, index); | ||
return callback(event, datasetIndex, index, value); | ||
} | ||
} | ||
}; | ||
} | ||
// Update values to the nearest values | ||
function applyMagnet(chartInstance, i, j) { | ||
const pluginOptions = chartInstance.config.options.plugins.dragData; | ||
if (pluginOptions.magnet) { | ||
const magnet = pluginOptions.magnet; | ||
if (magnet.to && typeof magnet.to === 'function') { | ||
let data = chartInstance.data.datasets[i].data[j]; | ||
data = magnet.to(data); | ||
chartInstance.data.datasets[i].data[j] = data; | ||
chartInstance.update('none'); | ||
return data | ||
function getElement(event, chartInstance, state) { | ||
var _a, _b, _c, _d, _e, _f, _g, _h, _j, _k, _l, _m, _o, _p, _q, _r, _s, _t, _u, _v, _w, _x, _y; | ||
var _z, _0, _1; | ||
if (state === void 0) { state = ChartJSDragDataPlugin.statesStore.get(chartInstance.id); } | ||
var callback = (_c = (_b = (_a = chartInstance.options) === null || _a === void 0 ? void 0 : _a.plugins) === null || _b === void 0 ? void 0 : _b.dragData) === null || _c === void 0 ? void 0 : _c.onDragStart; | ||
if (!state) | ||
return; | ||
var searchMode = (_f = (_e = (_d = chartInstance.config.options) === null || _d === void 0 ? void 0 : _d.interaction) === null || _e === void 0 ? void 0 : _e.mode) !== null && _f !== void 0 ? _f : "nearest", searchOptions = (_h = (_g = chartInstance.config.options) === null || _g === void 0 ? void 0 : _g.interaction) !== null && _h !== void 0 ? _h : { | ||
intersect: true, | ||
}; | ||
state.element = chartInstance.getElementsAtEventForMode(event, searchMode, searchOptions, false)[0]; | ||
if (state.element) { | ||
var datasetIndex = state.element.datasetIndex; | ||
var index = state.element.index; | ||
// note: type may be absent if config is ChartConfigurationCustomTypesPerDataset, in which case we pull this value from the dataset | ||
state.type = | ||
(_k = (_j = chartInstance.config.type) !== null && _j !== void 0 ? _j : chartInstance.data.datasets[datasetIndex].type) !== null && _k !== void 0 ? _k : undefined; | ||
// save element settings | ||
state.eventSettings = | ||
(_o = (_m = (_l = chartInstance.config.options) === null || _l === void 0 ? void 0 : _l.plugins) === null || _m === void 0 ? void 0 : _m.tooltip) === null || _o === void 0 ? void 0 : _o.animation; | ||
var dataset = chartInstance.data.datasets[datasetIndex]; | ||
var datasetMeta = chartInstance.getDatasetMeta(datasetIndex); | ||
var curValue = dataset.data[index]; | ||
// get the id of the datasets scale | ||
state.xAxisID = datasetMeta.xAxisID; | ||
state.yAxisID = datasetMeta.yAxisID; | ||
state.rAxisID = datasetMeta.rAxisID; | ||
var draggingConfiguration = checkDraggingConfiguration(chartInstance, datasetIndex, index), datasetDraggingDisabled = draggingConfiguration.datasetDraggingDisabled, xAxisDraggingDisabled = draggingConfiguration.xAxisDraggingDisabled, yAxisDraggingDisabled = draggingConfiguration.yAxisDraggingDisabled, dataPointDraggingDisabled = draggingConfiguration.dataPointDraggingDisabled; | ||
// check if dragging the dataset or datapoint is prohibited | ||
if (datasetDraggingDisabled || | ||
// dragging disabled on all scales | ||
(xAxisDraggingDisabled && yAxisDraggingDisabled) || | ||
dataPointDraggingDisabled) { | ||
state.element = null; | ||
return; | ||
} | ||
if (state.type === "bar") { | ||
// note: stacked may be missing in RadialLinearScaleOptions | ||
state.stacked = | ||
(_s = (_r = (_q = (_p = chartInstance.config.options) === null || _p === void 0 ? void 0 : _p.scales) === null || _q === void 0 ? void 0 : _q[state.xAxisID]) === null || _r === void 0 ? void 0 : _r.stacked) !== null && _s !== void 0 ? _s : undefined; | ||
// if a bar has a data point that is an array of length 2, it's a floating bar | ||
var samplePoint = chartInstance.data.datasets[0].data[0]; | ||
state.floatingBar = | ||
samplePoint !== null && | ||
Array.isArray(samplePoint) && | ||
samplePoint.length >= 2; | ||
var dataPoint = chartInstance.data.datasets[datasetIndex].data[index]; | ||
var newPos = calcCartesian(event, chartInstance, dataPoint, draggingConfiguration, state); | ||
state.initValue = newPos - curValue; | ||
} | ||
// disable the tooltip animation | ||
var showTooltipOptionValue = (_v = (_u = (_t = chartInstance.config.options) === null || _t === void 0 ? void 0 : _t.plugins) === null || _u === void 0 ? void 0 : _u.dragData) === null || _v === void 0 ? void 0 : _v.showTooltip; | ||
if (showTooltipOptionValue === undefined || | ||
showTooltipOptionValue === true) { | ||
(_w = (_z = chartInstance.config).options) !== null && _w !== void 0 ? _w : (_z.options = {}); | ||
(_x = (_0 = chartInstance.config.options).plugins) !== null && _x !== void 0 ? _x : (_0.plugins = {}); | ||
(_y = (_1 = chartInstance.config.options.plugins).tooltip) !== null && _y !== void 0 ? _y : (_1.tooltip = {}); | ||
chartInstance.config.options.plugins.tooltip.animation = false; | ||
} | ||
if (typeof callback === "function" && state.element) { | ||
if (callback(event, datasetIndex, index, curValue) === false) { | ||
state.element = null; | ||
} | ||
} | ||
} | ||
} else { | ||
return chartInstance.data.datasets[i].data[j] | ||
} | ||
} | ||
const dragEndCallback = (e, chartInstance, callback) => { | ||
curIndex = undefined; | ||
isDragging = false; | ||
// re-enable the tooltip animation | ||
if (chartInstance.config.options.plugins.tooltip) { | ||
chartInstance.config.options.plugins.tooltip.animation = eventSettings; | ||
chartInstance.update('none'); | ||
} | ||
// chartInstance.update('none') | ||
if (typeof callback === 'function' && element) { | ||
const datasetIndex = element.datasetIndex; | ||
const index = element.index; | ||
let value = applyMagnet(chartInstance, datasetIndex, index); | ||
return callback(e, datasetIndex, index, value) | ||
} | ||
}; | ||
const ChartJSdragDataPlugin = { | ||
id: 'dragdata', | ||
afterInit: function (chartInstance) { | ||
if (chartInstance.config.options.plugins && chartInstance.config.options.plugins.dragData) { | ||
const pluginOptions = chartInstance.config.options.plugins.dragData; | ||
select(chartInstance.canvas).call( | ||
drag().container(chartInstance.canvas) | ||
.on('start', e => getElement(e.sourceEvent, chartInstance, pluginOptions.onDragStart)) | ||
.on('drag', e => updateData(e.sourceEvent, chartInstance, pluginOptions, pluginOptions.onDrag)) | ||
.on('end', e => dragEndCallback(e.sourceEvent, chartInstance, pluginOptions.onDragEnd)) | ||
); | ||
function updateData(event, chartInstance, state) { | ||
var _a, _b; | ||
if (state === void 0) { state = ChartJSDragDataPlugin.statesStore.get(chartInstance.id); } | ||
if (!state) | ||
return; | ||
var pluginOptions = (_b = (_a = chartInstance.options) === null || _a === void 0 ? void 0 : _a.plugins) === null || _b === void 0 ? void 0 : _b.dragData; | ||
var callback = pluginOptions === null || pluginOptions === void 0 ? void 0 : pluginOptions.onDrag; | ||
if (state.element) { | ||
state.curDatasetIndex = state.element.datasetIndex; | ||
state.curIndex = state.element.index; | ||
state.isDragging = true; | ||
var dataPoint = chartInstance.data.datasets[state.curDatasetIndex].data[state.curIndex]; | ||
var draggingConfiguration = checkDraggingConfiguration(chartInstance, state.curDatasetIndex, state.curIndex); | ||
if (state.type === "radar" || state.type === "polarArea") { | ||
dataPoint = calcRadialLinear(event, chartInstance, state.curIndex, state.rAxisID, state); | ||
} | ||
else if (state.stacked) { | ||
var cursorPos = calcCartesian(event, chartInstance, dataPoint, draggingConfiguration, state); | ||
dataPoint = roundValue(cursorPos - state.initValue, pluginOptions === null || pluginOptions === void 0 ? void 0 : pluginOptions.round); | ||
} | ||
else { | ||
dataPoint = calcCartesian(event, chartInstance, dataPoint, draggingConfiguration, state); | ||
} | ||
if (typeof callback === "function" | ||
? callback(event, state.curDatasetIndex, state.curIndex, dataPoint) !== | ||
false | ||
: true) { | ||
chartInstance.data.datasets[state.curDatasetIndex].data[state.curIndex] = | ||
dataPoint; | ||
chartInstance.update("none"); | ||
} | ||
} | ||
}, | ||
beforeEvent: function (chart) { | ||
if (isDragging) { | ||
if (chart.tooltip) chart.tooltip.update(); | ||
return false | ||
} | ||
}, | ||
}; | ||
chart_js.Chart.register(ChartJSdragDataPlugin); | ||
} | ||
return ChartJSdragDataPlugin; | ||
// export all utility functions | ||
exports.applyMagnet = applyMagnet; | ||
exports.calcCartesian = calcCartesian; | ||
exports.calcRadialLinear = calcRadialLinear; | ||
exports.checkDraggingConfiguration = checkDraggingConfiguration; | ||
exports.clipValue = clipValue; | ||
exports.cloneDataPoint = cloneDataPoint; | ||
exports.default = ChartJSDragDataPlugin; | ||
exports.dragEndCallback = dragEndCallback; | ||
exports.getElement = getElement; | ||
exports.roundValue = roundValue; | ||
exports.updateData = updateData; | ||
Object.defineProperty(exports, '__esModule', { value: true }); | ||
})); |
@@ -1,1 +0,7 @@ | ||
!function(t,n){"object"==typeof exports&&"undefined"!=typeof module?module.exports=n(require("chart.js")):"function"==typeof define&&define.amd?define(["chart.js"],n):(t="undefined"!=typeof globalThis?globalThis:t||self).index=n(t.Chart)}(this,(function(t){"use strict";var n={value:()=>{}};function e(){for(var t,n=0,e=arguments.length,i={};n<e;++n){if(!(t=arguments[n]+"")||t in i||/[\s.]/.test(t))throw new Error("illegal type: "+t);i[t]=[]}return new r(i)}function r(t){this._=t}function i(t,n){return t.trim().split(/^|\s+/).map((function(t){var e="",r=t.indexOf(".");if(r>=0&&(e=t.slice(r+1),t=t.slice(0,r)),t&&!n.hasOwnProperty(t))throw new Error("unknown type: "+t);return{type:t,name:e}}))}function o(t,n){for(var e,r=0,i=t.length;r<i;++r)if((e=t[r]).name===n)return e.value}function a(t,e,r){for(var i=0,o=t.length;i<o;++i)if(t[i].name===e){t[i]=n,t=t.slice(0,i).concat(t.slice(i+1));break}return null!=r&&t.push({name:e,value:r}),t}r.prototype=e.prototype={constructor:r,on:function(t,n){var e,r=this._,u=i(t+"",r),s=-1,c=u.length;if(!(arguments.length<2)){if(null!=n&&"function"!=typeof n)throw new Error("invalid callback: "+n);for(;++s<c;)if(e=(t=u[s]).type)r[e]=a(r[e],t.name,n);else if(null==n)for(e in r)r[e]=a(r[e],t.name,null);return this}for(;++s<c;)if((e=(t=u[s]).type)&&(e=o(r[e],t.name)))return e},copy:function(){var t={},n=this._;for(var e in n)t[e]=n[e].slice();return new r(t)},call:function(t,n){if((e=arguments.length-2)>0)for(var e,r,i=new Array(e),o=0;o<e;++o)i[o]=arguments[o+2];if(!this._.hasOwnProperty(t))throw new Error("unknown type: "+t);for(o=0,e=(r=this._[t]).length;o<e;++o)r[o].value.apply(n,i)},apply:function(t,n,e){if(!this._.hasOwnProperty(t))throw new Error("unknown type: "+t);for(var r=this._[t],i=0,o=r.length;i<o;++i)r[i].value.apply(n,e)}};var u="http://www.w3.org/1999/xhtml",s={svg:"http://www.w3.org/2000/svg",xhtml:u,xlink:"http://www.w3.org/1999/xlink",xml:"http://www.w3.org/XML/1998/namespace",xmlns:"http://www.w3.org/2000/xmlns/"};function c(t){var n=t+="",e=n.indexOf(":");return e>=0&&"xmlns"!==(n=t.slice(0,e))&&(t=t.slice(e+1)),s.hasOwnProperty(n)?{space:s[n],local:t}:t}function l(t){return function(){var n=this.ownerDocument,e=this.namespaceURI;return e===u&&n.documentElement.namespaceURI===u?n.createElement(t):n.createElementNS(e,t)}}function f(t){return function(){return this.ownerDocument.createElementNS(t.space,t.local)}}function h(t){var n=c(t);return(n.local?f:l)(n)}function p(){}function d(t){return null==t?p:function(){return this.querySelector(t)}}function g(t){return null==t?[]:Array.isArray(t)?t:Array.from(t)}function v(){return[]}function y(t){return function(n){return n.matches(t)}}var m=Array.prototype.find;function _(){return this.firstElementChild}var w=Array.prototype.filter;function x(){return Array.from(this.children)}function b(t){return new Array(t.length)}function A(t,n){this.ownerDocument=t.ownerDocument,this.namespaceURI=t.namespaceURI,this._next=null,this._parent=t,this.__data__=n}function E(t){return function(){return t}}function D(t,n,e,r,i,o){for(var a,u=0,s=n.length,c=o.length;u<c;++u)(a=n[u])?(a.__data__=o[u],r[u]=a):e[u]=new A(t,o[u]);for(;u<s;++u)(a=n[u])&&(i[u]=a)}function C(t,n,e,r,i,o,a){var u,s,c,l=new Map,f=n.length,h=o.length,p=new Array(f);for(u=0;u<f;++u)(s=n[u])&&(p[u]=c=a.call(s,s.__data__,u,n)+"",l.has(c)?i[u]=s:l.set(c,s));for(u=0;u<h;++u)c=a.call(t,o[u],u,o)+"",(s=l.get(c))?(r[u]=s,s.__data__=o[u],l.delete(c)):e[u]=new A(t,o[u]);for(u=0;u<f;++u)(s=n[u])&&l.get(p[u])===s&&(i[u]=s)}function S(t){return t.__data__}function M(t){return"object"==typeof t&&"length"in t?t:Array.from(t)}function N(t,n){return t<n?-1:t>n?1:t>=n?0:NaN}function P(t){return function(){this.removeAttribute(t)}}function T(t){return function(){this.removeAttributeNS(t.space,t.local)}}function B(t,n){return function(){this.setAttribute(t,n)}}function k(t,n){return function(){this.setAttributeNS(t.space,t.local,n)}}function I(t,n){return function(){var e=n.apply(this,arguments);null==e?this.removeAttribute(t):this.setAttribute(t,e)}}function R(t,n){return function(){var e=n.apply(this,arguments);null==e?this.removeAttributeNS(t.space,t.local):this.setAttributeNS(t.space,t.local,e)}}function j(t){return t.ownerDocument&&t.ownerDocument.defaultView||t.document&&t||t.defaultView}function L(t){return function(){this.style.removeProperty(t)}}function O(t,n,e){return function(){this.style.setProperty(t,n,e)}}function V(t,n,e){return function(){var r=n.apply(this,arguments);null==r?this.style.removeProperty(t):this.style.setProperty(t,r,e)}}function X(t,n){return t.style.getPropertyValue(n)||j(t).getComputedStyle(t,null).getPropertyValue(n)}function q(t){return function(){delete this[t]}}function Y(t,n){return function(){this[t]=n}}function U(t,n){return function(){var e=n.apply(this,arguments);null==e?delete this[t]:this[t]=e}}function F(t){return t.trim().split(/^|\s+/)}function z(t){return t.classList||new H(t)}function H(t){this._node=t,this._names=F(t.getAttribute("class")||"")}function G(t,n){for(var e=z(t),r=-1,i=n.length;++r<i;)e.add(n[r])}function K(t,n){for(var e=z(t),r=-1,i=n.length;++r<i;)e.remove(n[r])}function J(t){return function(){G(this,t)}}function Q(t){return function(){K(this,t)}}function W(t,n){return function(){(n.apply(this,arguments)?G:K)(this,t)}}function Z(){this.textContent=""}function $(t){return function(){this.textContent=t}}function tt(t){return function(){var n=t.apply(this,arguments);this.textContent=null==n?"":n}}function nt(){this.innerHTML=""}function et(t){return function(){this.innerHTML=t}}function rt(t){return function(){var n=t.apply(this,arguments);this.innerHTML=null==n?"":n}}function it(){this.nextSibling&&this.parentNode.appendChild(this)}function ot(){this.previousSibling&&this.parentNode.insertBefore(this,this.parentNode.firstChild)}function at(){return null}function ut(){var t=this.parentNode;t&&t.removeChild(this)}function st(){var t=this.cloneNode(!1),n=this.parentNode;return n?n.insertBefore(t,this.nextSibling):t}function ct(){var t=this.cloneNode(!0),n=this.parentNode;return n?n.insertBefore(t,this.nextSibling):t}function lt(t){return t.trim().split(/^|\s+/).map((function(t){var n="",e=t.indexOf(".");return e>=0&&(n=t.slice(e+1),t=t.slice(0,e)),{type:t,name:n}}))}function ft(t){return function(){var n=this.__on;if(n){for(var e,r=0,i=-1,o=n.length;r<o;++r)e=n[r],t.type&&e.type!==t.type||e.name!==t.name?n[++i]=e:this.removeEventListener(e.type,e.listener,e.options);++i?n.length=i:delete this.__on}}}function ht(t,n,e){return function(){var r,i=this.__on,o=function(t){return function(n){t.call(this,n,this.__data__)}}(n);if(i)for(var a=0,u=i.length;a<u;++a)if((r=i[a]).type===t.type&&r.name===t.name)return this.removeEventListener(r.type,r.listener,r.options),this.addEventListener(r.type,r.listener=o,r.options=e),void(r.value=n);this.addEventListener(t.type,o,e),r={type:t.type,name:t.name,value:n,listener:o,options:e},i?i.push(r):this.__on=[r]}}function pt(t,n,e){var r=j(t),i=r.CustomEvent;"function"==typeof i?i=new i(n,e):(i=r.document.createEvent("Event"),e?(i.initEvent(n,e.bubbles,e.cancelable),i.detail=e.detail):i.initEvent(n,!1,!1)),t.dispatchEvent(i)}function dt(t,n){return function(){return pt(this,t,n)}}function gt(t,n){return function(){return pt(this,t,n.apply(this,arguments))}}A.prototype={constructor:A,appendChild:function(t){return this._parent.insertBefore(t,this._next)},insertBefore:function(t,n){return this._parent.insertBefore(t,n)},querySelector:function(t){return this._parent.querySelector(t)},querySelectorAll:function(t){return this._parent.querySelectorAll(t)}},H.prototype={add:function(t){this._names.indexOf(t)<0&&(this._names.push(t),this._node.setAttribute("class",this._names.join(" ")))},remove:function(t){var n=this._names.indexOf(t);n>=0&&(this._names.splice(n,1),this._node.setAttribute("class",this._names.join(" ")))},contains:function(t){return this._names.indexOf(t)>=0}};var vt=[null];function yt(t,n){this._groups=t,this._parents=n}function mt(t){return"string"==typeof t?new yt([[document.querySelector(t)]],[document.documentElement]):new yt([[t]],vt)}function _t(t,n){if(t=function(t){let n;for(;n=t.sourceEvent;)t=n;return t}(t),void 0===n&&(n=t.currentTarget),n){var e=n.ownerSVGElement||n;if(e.createSVGPoint){var r=e.createSVGPoint();return r.x=t.clientX,r.y=t.clientY,[(r=r.matrixTransform(n.getScreenCTM().inverse())).x,r.y]}if(n.getBoundingClientRect){var i=n.getBoundingClientRect();return[t.clientX-i.left-n.clientLeft,t.clientY-i.top-n.clientTop]}}return[t.pageX,t.pageY]}yt.prototype={constructor:yt,select:function(t){"function"!=typeof t&&(t=d(t));for(var n=this._groups,e=n.length,r=new Array(e),i=0;i<e;++i)for(var o,a,u=n[i],s=u.length,c=r[i]=new Array(s),l=0;l<s;++l)(o=u[l])&&(a=t.call(o,o.__data__,l,u))&&("__data__"in o&&(a.__data__=o.__data__),c[l]=a);return new yt(r,this._parents)},selectAll:function(t){t="function"==typeof t?function(t){return function(){return g(t.apply(this,arguments))}}(t):function(t){return null==t?v:function(){return this.querySelectorAll(t)}}(t);for(var n=this._groups,e=n.length,r=[],i=[],o=0;o<e;++o)for(var a,u=n[o],s=u.length,c=0;c<s;++c)(a=u[c])&&(r.push(t.call(a,a.__data__,c,u)),i.push(a));return new yt(r,i)},selectChild:function(t){return this.select(null==t?_:function(t){return function(){return m.call(this.children,t)}}("function"==typeof t?t:y(t)))},selectChildren:function(t){return this.selectAll(null==t?x:function(t){return function(){return w.call(this.children,t)}}("function"==typeof t?t:y(t)))},filter:function(t){"function"!=typeof t&&(t=function(t){return function(){return this.matches(t)}}(t));for(var n=this._groups,e=n.length,r=new Array(e),i=0;i<e;++i)for(var o,a=n[i],u=a.length,s=r[i]=[],c=0;c<u;++c)(o=a[c])&&t.call(o,o.__data__,c,a)&&s.push(o);return new yt(r,this._parents)},data:function(t,n){if(!arguments.length)return Array.from(this,S);var e=n?C:D,r=this._parents,i=this._groups;"function"!=typeof t&&(t=E(t));for(var o=i.length,a=new Array(o),u=new Array(o),s=new Array(o),c=0;c<o;++c){var l=r[c],f=i[c],h=f.length,p=M(t.call(l,l&&l.__data__,c,r)),d=p.length,g=u[c]=new Array(d),v=a[c]=new Array(d),y=s[c]=new Array(h);e(l,f,g,v,y,p,n);for(var m,_,w=0,x=0;w<d;++w)if(m=g[w]){for(w>=x&&(x=w+1);!(_=v[x])&&++x<d;);m._next=_||null}}return(a=new yt(a,r))._enter=u,a._exit=s,a},enter:function(){return new yt(this._enter||this._groups.map(b),this._parents)},exit:function(){return new yt(this._exit||this._groups.map(b),this._parents)},join:function(t,n,e){var r=this.enter(),i=this,o=this.exit();return"function"==typeof t?(r=t(r))&&(r=r.selection()):r=r.append(t+""),null!=n&&(i=n(i))&&(i=i.selection()),null==e?o.remove():e(o),r&&i?r.merge(i).order():i},merge:function(t){for(var n=t.selection?t.selection():t,e=this._groups,r=n._groups,i=e.length,o=r.length,a=Math.min(i,o),u=new Array(i),s=0;s<a;++s)for(var c,l=e[s],f=r[s],h=l.length,p=u[s]=new Array(h),d=0;d<h;++d)(c=l[d]||f[d])&&(p[d]=c);for(;s<i;++s)u[s]=e[s];return new yt(u,this._parents)},selection:function(){return this},order:function(){for(var t=this._groups,n=-1,e=t.length;++n<e;)for(var r,i=t[n],o=i.length-1,a=i[o];--o>=0;)(r=i[o])&&(a&&4^r.compareDocumentPosition(a)&&a.parentNode.insertBefore(r,a),a=r);return this},sort:function(t){function n(n,e){return n&&e?t(n.__data__,e.__data__):!n-!e}t||(t=N);for(var e=this._groups,r=e.length,i=new Array(r),o=0;o<r;++o){for(var a,u=e[o],s=u.length,c=i[o]=new Array(s),l=0;l<s;++l)(a=u[l])&&(c[l]=a);c.sort(n)}return new yt(i,this._parents).order()},call:function(){var t=arguments[0];return arguments[0]=this,t.apply(null,arguments),this},nodes:function(){return Array.from(this)},node:function(){for(var t=this._groups,n=0,e=t.length;n<e;++n)for(var r=t[n],i=0,o=r.length;i<o;++i){var a=r[i];if(a)return a}return null},size:function(){let t=0;for(const n of this)++t;return t},empty:function(){return!this.node()},each:function(t){for(var n=this._groups,e=0,r=n.length;e<r;++e)for(var i,o=n[e],a=0,u=o.length;a<u;++a)(i=o[a])&&t.call(i,i.__data__,a,o);return this},attr:function(t,n){var e=c(t);if(arguments.length<2){var r=this.node();return e.local?r.getAttributeNS(e.space,e.local):r.getAttribute(e)}return this.each((null==n?e.local?T:P:"function"==typeof n?e.local?R:I:e.local?k:B)(e,n))},style:function(t,n,e){return arguments.length>1?this.each((null==n?L:"function"==typeof n?V:O)(t,n,null==e?"":e)):X(this.node(),t)},property:function(t,n){return arguments.length>1?this.each((null==n?q:"function"==typeof n?U:Y)(t,n)):this.node()[t]},classed:function(t,n){var e=F(t+"");if(arguments.length<2){for(var r=z(this.node()),i=-1,o=e.length;++i<o;)if(!r.contains(e[i]))return!1;return!0}return this.each(("function"==typeof n?W:n?J:Q)(e,n))},text:function(t){return arguments.length?this.each(null==t?Z:("function"==typeof t?tt:$)(t)):this.node().textContent},html:function(t){return arguments.length?this.each(null==t?nt:("function"==typeof t?rt:et)(t)):this.node().innerHTML},raise:function(){return this.each(it)},lower:function(){return this.each(ot)},append:function(t){var n="function"==typeof t?t:h(t);return this.select((function(){return this.appendChild(n.apply(this,arguments))}))},insert:function(t,n){var e="function"==typeof t?t:h(t),r=null==n?at:"function"==typeof n?n:d(n);return this.select((function(){return this.insertBefore(e.apply(this,arguments),r.apply(this,arguments)||null)}))},remove:function(){return this.each(ut)},clone:function(t){return this.select(t?ct:st)},datum:function(t){return arguments.length?this.property("__data__",t):this.node().__data__},on:function(t,n,e){var r,i,o=lt(t+""),a=o.length;if(!(arguments.length<2)){for(u=n?ht:ft,r=0;r<a;++r)this.each(u(o[r],n,e));return this}var u=this.node().__on;if(u)for(var s,c=0,l=u.length;c<l;++c)for(r=0,s=u[c];r<a;++r)if((i=o[r]).type===s.type&&i.name===s.name)return s.value},dispatch:function(t,n){return this.each(("function"==typeof n?gt:dt)(t,n))},[Symbol.iterator]:function*(){for(var t=this._groups,n=0,e=t.length;n<e;++n)for(var r,i=t[n],o=0,a=i.length;o<a;++o)(r=i[o])&&(yield r)}};const wt={passive:!1},xt={capture:!0,passive:!1};function bt(t){t.stopImmediatePropagation()}function At(t){t.preventDefault(),t.stopImmediatePropagation()}var Et=t=>()=>t;function Dt(t,{sourceEvent:n,subject:e,target:r,identifier:i,active:o,x:a,y:u,dx:s,dy:c,dispatch:l}){Object.defineProperties(this,{type:{value:t,enumerable:!0,configurable:!0},sourceEvent:{value:n,enumerable:!0,configurable:!0},subject:{value:e,enumerable:!0,configurable:!0},target:{value:r,enumerable:!0,configurable:!0},identifier:{value:i,enumerable:!0,configurable:!0},active:{value:o,enumerable:!0,configurable:!0},x:{value:a,enumerable:!0,configurable:!0},y:{value:u,enumerable:!0,configurable:!0},dx:{value:s,enumerable:!0,configurable:!0},dy:{value:c,enumerable:!0,configurable:!0},_:{value:l}})}function Ct(t){return!t.ctrlKey&&!t.button}function St(){return this.parentNode}function Mt(t,n){return null==n?{x:t.x,y:t.y}:n}function Nt(){return navigator.maxTouchPoints||"ontouchstart"in this}function Pt(){var t,n,r,i,o=Ct,a=St,u=Mt,s=Nt,c={},l=e("start","drag","end"),f=0,h=0;function p(t){t.on("mousedown.drag",d).filter(s).on("touchstart.drag",y).on("touchmove.drag",m,wt).on("touchend.drag touchcancel.drag",_).style("touch-action","none").style("-webkit-tap-highlight-color","rgba(0,0,0,0)")}function d(e,u){if(!i&&o.call(this,e,u)){var s=w(this,a.call(this,e,u),e,u,"mouse");s&&(mt(e.view).on("mousemove.drag",g,xt).on("mouseup.drag",v,xt),function(t){var n=t.document.documentElement,e=mt(t).on("dragstart.drag",At,xt);"onselectstart"in n?e.on("selectstart.drag",At,xt):(n.__noselect=n.style.MozUserSelect,n.style.MozUserSelect="none")}(e.view),bt(e),r=!1,t=e.clientX,n=e.clientY,s("start",e))}}function g(e){if(At(e),!r){var i=e.clientX-t,o=e.clientY-n;r=i*i+o*o>h}c.mouse("drag",e)}function v(t){mt(t.view).on("mousemove.drag mouseup.drag",null),function(t,n){var e=t.document.documentElement,r=mt(t).on("dragstart.drag",null);n&&(r.on("click.drag",At,xt),setTimeout((function(){r.on("click.drag",null)}),0)),"onselectstart"in e?r.on("selectstart.drag",null):(e.style.MozUserSelect=e.__noselect,delete e.__noselect)}(t.view,r),At(t),c.mouse("end",t)}function y(t,n){if(o.call(this,t,n)){var e,r,i=t.changedTouches,u=a.call(this,t,n),s=i.length;for(e=0;e<s;++e)(r=w(this,u,t,n,i[e].identifier,i[e]))&&(bt(t),r("start",t,i[e]))}}function m(t){var n,e,r=t.changedTouches,i=r.length;for(n=0;n<i;++n)(e=c[r[n].identifier])&&(At(t),e("drag",t,r[n]))}function _(t){var n,e,r=t.changedTouches,o=r.length;for(i&&clearTimeout(i),i=setTimeout((function(){i=null}),500),n=0;n<o;++n)(e=c[r[n].identifier])&&(bt(t),e("end",t,r[n]))}function w(t,n,e,r,i,o){var a,s,h,d=l.copy(),g=_t(o||e,n);if(null!=(h=u.call(t,new Dt("beforestart",{sourceEvent:e,target:p,identifier:i,active:f,x:g[0],y:g[1],dx:0,dy:0,dispatch:d}),r)))return a=h.x-g[0]||0,s=h.y-g[1]||0,function e(o,u,l){var v,y=g;switch(o){case"start":c[i]=e,v=f++;break;case"end":delete c[i],--f;case"drag":g=_t(l||u,n),v=f}d.call(o,t,new Dt(o,{sourceEvent:u,subject:h,target:p,identifier:i,active:v,x:g[0]+a,y:g[1]+s,dx:g[0]-y[0],dy:g[1]-y[1],dispatch:d}),r)}}return p.filter=function(t){return arguments.length?(o="function"==typeof t?t:Et(!!t),p):o},p.container=function(t){return arguments.length?(a="function"==typeof t?t:Et(t),p):a},p.subject=function(t){return arguments.length?(u="function"==typeof t?t:Et(t),p):u},p.touchable=function(t){return arguments.length?(s="function"==typeof t?t:Et(!!t),p):s},p.on=function(){var t=l.on.apply(l,arguments);return t===l?p:t},p.clickDistance=function(t){return arguments.length?(h=(t=+t)*t,p):Math.sqrt(h)},p}let Tt,Bt,kt,It,Rt,jt,Lt,Ot,Vt,Xt,qt;Dt.prototype.on=function(){var t=this._.on.apply(this._,arguments);return t===this._?this:t};let Yt=!1;function Ut(t,n){return isNaN(n)?t:Math.round(t*Math.pow(10,n))/Math.pow(10,n)}function Ft(t,n,e,r,i){let o,a;const u=n.data.datasets[e].data[r];if(t.touches?(o=n.scales[kt].getValueForPixel(t.touches[0].clientX-n.canvas.getBoundingClientRect().left),a=n.scales[Bt].getValueForPixel(t.touches[0].clientY-n.canvas.getBoundingClientRect().top)):(o=n.scales[kt].getValueForPixel(t.clientX-n.canvas.getBoundingClientRect().left),a=n.scales[Bt].getValueForPixel(t.clientY-n.canvas.getBoundingClientRect().top)),o=Ut(o,n.config.options.plugins.dragData.round),a=Ut(a,n.config.options.plugins.dragData.round),o=o>n.scales[kt].max?n.scales[kt].max:o,o=o<n.scales[kt].min?n.scales[kt].min:o,a=a>n.scales[Bt].max?n.scales[Bt].max:a,a=a<n.scales[Bt].min?n.scales[Bt].min:a,Lt){let t;t="y"===n.config.options.indexAxis?o:a;return Math.abs(t-u[0])<=Math.abs(t-u[1])?[t,u[1]]:[u[0],t]}return void 0!==u.x&&n.config.options.plugins.dragData.dragX&&(u.x=o),void 0!==u.y?(!1!==n.config.options.plugins.dragData.dragY&&(u.y=a),u):"y"===n.config.options.indexAxis?o:a}const zt=(t,n,e,r)=>{if(Tt){Vt=Tt.datasetIndex,Xt=Tt.index,Yt=!0;let i=n.data.datasets[Vt].data[Xt];if("radar"===Rt||"polarArea"===Rt)i=function(t,n){let e,r,i;t.touches?(e=t.touches[0].clientX-n.canvas.getBoundingClientRect().left,r=t.touches[0].clientY-n.canvas.getBoundingClientRect().top):(e=t.clientX-n.canvas.getBoundingClientRect().left,r=t.clientY-n.canvas.getBoundingClientRect().top);let o=n.scales[It],a=Math.sqrt(Math.pow(e-o.xCenter,2)+Math.pow(r-o.yCenter,2)),u=o.drawingArea/(o.max-o.min);return i=o.options.ticks.reverse?o.max-a/u:o.min+a/u,i=Ut(i,n.config.options.plugins.dragData.round),i=i>n.scales[It].max?n.scales[It].max:i,i=i<n.scales[It].min?n.scales[It].min:i,i}(t,n);else if(jt){i=Ut(Ft(t,n,Vt,Xt)-Ot,e.round)}else i=Ft(t,n,Vt,Xt);(!r||"function"==typeof r&&!1!==r(t,Vt,Xt,i))&&(n.data.datasets[Vt].data[Xt]=i,n.update("none"))}};const Ht={id:"dragdata",afterInit:function(t){if(t.config.options.plugins&&t.config.options.plugins.dragData){const n=t.config.options.plugins.dragData;mt(t.canvas).call(Pt().container(t.canvas).on("start",(e=>((t,n,e)=>{if(Tt=n.getElementsAtEventForMode(t,"nearest",{intersect:!0},!1)[0],Rt=n.config.type,Tt){let r=Tt.datasetIndex,i=Tt.index;qt=function(t){try{return t()}catch(t){return""}}((()=>n.config.options.plugins.tooltip.animation));const o=n.data.datasets[r],a=n.getDatasetMeta(r);let u=o.data[i];if(kt=a.xAxisID,Bt=a.yAxisID,It=a.rAxisID,!1===o.dragData||n.config.options.scales[kt]&&!1===n.config.options.scales[kt].dragData||n.config.options.scales[Bt]&&!1===n.config.options.scales[Bt].dragData||n.config.options.scales[It]&&!1===n.config.options.scales[It].rAxisID||!1===o.data[Tt.index].dragData)return void(Tt=null);if("bar"===Rt){jt=n.config.options.scales[kt].stacked;const e=n.data.datasets[0].data[0];Lt=null!==e&&Array.isArray(e)&&2==e.length;let o=Ft(t,n,r,i);Ot=o-u}(void 0===n.config.options.plugins.dragData.showTooltip||n.config.options.plugins.dragData.showTooltip)&&(n.config.options.plugins.tooltip||(n.config.options.plugins.tooltip={}),n.config.options.plugins.tooltip.animation=!1),"function"==typeof e&&Tt&&!1===e(t,r,i,u)&&(Tt=null)}})(e.sourceEvent,t,n.onDragStart))).on("drag",(e=>zt(e.sourceEvent,t,n,n.onDrag))).on("end",(e=>((t,n,e)=>{if(Xt=void 0,Yt=!1,n.config.options.plugins.tooltip&&(n.config.options.plugins.tooltip.animation=qt,n.update("none")),"function"==typeof e&&Tt){const r=Tt.datasetIndex,i=Tt.index;let o=function(t,n,e){const r=t.config.options.plugins.dragData;if(!r.magnet)return t.data.datasets[n].data[e];{const i=r.magnet;if(i.to&&"function"==typeof i.to){let r=t.data.datasets[n].data[e];return r=i.to(r),t.data.datasets[n].data[e]=r,t.update("none"),r}}}(n,r,i);return e(t,r,i,o)}})(e.sourceEvent,t,n.onDragEnd))))}},beforeEvent:function(t){if(Yt)return t.tooltip&&t.tooltip.update(),!1}};return t.Chart.register(Ht),Ht})); | ||
/*! | ||
* chartjs-plugin-dragdata v2.3.0 | ||
* https://github.com/artus9033/chartjs-plugin-dragdata.git | ||
* (c) 2018-2024 chartjs-plugin-dragdata contributors | ||
* Released under the MIT license | ||
*/ | ||
!function(t,n){"object"==typeof exports&&"undefined"!=typeof module?n(exports,require("chart.js/helpers"),require("chart.js")):"function"==typeof define&&define.amd?define(["exports","chart.js/helpers","chart.js"],n):n((t="undefined"!=typeof globalThis?globalThis:t||self).ChartJSDragDataPlugin={},t.Chart.helpers,t.Chart)}(this,(function(t,n,e){"use strict";var i={value:()=>{}};function r(){for(var t,n=0,e=arguments.length,i={};n<e;++n){if(!(t=arguments[n]+"")||t in i||/[\s.]/.test(t))throw new Error("illegal type: "+t);i[t]=[]}return new o(i)}function o(t){this._=t}function a(t,n){for(var e,i=0,r=t.length;i<r;++i)if((e=t[i]).name===n)return e.value}function u(t,n,e){for(var r=0,o=t.length;r<o;++r)if(t[r].name===n){t[r]=i,t=t.slice(0,r).concat(t.slice(r+1));break}return null!=e&&t.push({name:n,value:e}),t}o.prototype=r.prototype={constructor:o,on:function(t,n){var e,i,r=this._,o=(i=r,(t+"").trim().split(/^|\s+/).map((function(t){var n="",e=t.indexOf(".");if(e>=0&&(n=t.slice(e+1),t=t.slice(0,e)),t&&!i.hasOwnProperty(t))throw new Error("unknown type: "+t);return{type:t,name:n}}))),l=-1,s=o.length;if(!(arguments.length<2)){if(null!=n&&"function"!=typeof n)throw new Error("invalid callback: "+n);for(;++l<s;)if(e=(t=o[l]).type)r[e]=u(r[e],t.name,n);else if(null==n)for(e in r)r[e]=u(r[e],t.name,null);return this}for(;++l<s;)if((e=(t=o[l]).type)&&(e=a(r[e],t.name)))return e},copy:function(){var t={},n=this._;for(var e in n)t[e]=n[e].slice();return new o(t)},call:function(t,n){if((e=arguments.length-2)>0)for(var e,i,r=new Array(e),o=0;o<e;++o)r[o]=arguments[o+2];if(!this._.hasOwnProperty(t))throw new Error("unknown type: "+t);for(o=0,e=(i=this._[t]).length;o<e;++o)i[o].value.apply(n,r)},apply:function(t,n,e){if(!this._.hasOwnProperty(t))throw new Error("unknown type: "+t);for(var i=this._[t],r=0,o=i.length;r<o;++r)i[r].value.apply(n,e)}};var l="http://www.w3.org/1999/xhtml",s={svg:"http://www.w3.org/2000/svg",xhtml:l,xlink:"http://www.w3.org/1999/xlink",xml:"http://www.w3.org/XML/1998/namespace",xmlns:"http://www.w3.org/2000/xmlns/"};function c(t){var n=t+="",e=n.indexOf(":");return e>=0&&"xmlns"!==(n=t.slice(0,e))&&(t=t.slice(e+1)),s.hasOwnProperty(n)?{space:s[n],local:t}:t}function f(t){return function(){var n=this.ownerDocument,e=this.namespaceURI;return e===l&&n.documentElement.namespaceURI===l?n.createElement(t):n.createElementNS(e,t)}}function d(t){return function(){return this.ownerDocument.createElementNS(t.space,t.local)}}function h(t){var n=c(t);return(n.local?d:f)(n)}function v(){}function p(t){return null==t?v:function(){return this.querySelector(t)}}function g(){return[]}function y(t){return function(){return null==(n=t.apply(this,arguments))?[]:Array.isArray(n)?n:Array.from(n);var n}}function m(t){return function(n){return n.matches(t)}}var _=Array.prototype.find;function x(){return this.firstElementChild}var w=Array.prototype.filter;function D(){return Array.from(this.children)}function b(t){return new Array(t.length)}function A(t,n){this.ownerDocument=t.ownerDocument,this.namespaceURI=t.namespaceURI,this._next=null,this._parent=t,this.__data__=n}function I(t,n,e,i,r,o){for(var a,u=0,l=n.length,s=o.length;u<s;++u)(a=n[u])?(a.__data__=o[u],i[u]=a):e[u]=new A(t,o[u]);for(;u<l;++u)(a=n[u])&&(r[u]=a)}function S(t,n,e,i,r,o,a){var u,l,s,c=new Map,f=n.length,d=o.length,h=new Array(f);for(u=0;u<f;++u)(l=n[u])&&(h[u]=s=a.call(l,l.__data__,u,n)+"",c.has(s)?r[u]=l:c.set(s,l));for(u=0;u<d;++u)s=a.call(t,o[u],u,o)+"",(l=c.get(s))?(i[u]=l,l.__data__=o[u],c.delete(s)):e[u]=new A(t,o[u]);for(u=0;u<f;++u)(l=n[u])&&c.get(h[u])===l&&(r[u]=l)}function E(t){return t.__data__}function P(t){return"object"==typeof t&&"length"in t?t:Array.from(t)}function M(t,n){return t<n?-1:t>n?1:t>=n?0:NaN}function C(t){return function(){this.removeAttribute(t)}}function N(t){return function(){this.removeAttributeNS(t.space,t.local)}}function j(t,n){return function(){this.setAttribute(t,n)}}function k(t,n){return function(){this.setAttributeNS(t.space,t.local,n)}}function T(t,n){return function(){var e=n.apply(this,arguments);null==e?this.removeAttribute(t):this.setAttribute(t,e)}}function V(t,n){return function(){var e=n.apply(this,arguments);null==e?this.removeAttributeNS(t.space,t.local):this.setAttributeNS(t.space,t.local,e)}}function O(t){return t.ownerDocument&&t.ownerDocument.defaultView||t.document&&t||t.defaultView}function B(t){return function(){this.style.removeProperty(t)}}function L(t,n,e){return function(){this.style.setProperty(t,n,e)}}function q(t,n,e){return function(){var i=n.apply(this,arguments);null==i?this.style.removeProperty(t):this.style.setProperty(t,i,e)}}function R(t){return function(){delete this[t]}}function U(t,n){return function(){this[t]=n}}function X(t,n){return function(){var e=n.apply(this,arguments);null==e?delete this[t]:this[t]=e}}function F(t){return t.trim().split(/^|\s+/)}function Y(t){return t.classList||new z(t)}function z(t){this._node=t,this._names=F(t.getAttribute("class")||"")}function H(t,n){for(var e=Y(t),i=-1,r=n.length;++i<r;)e.add(n[i])}function G(t,n){for(var e=Y(t),i=-1,r=n.length;++i<r;)e.remove(n[i])}function J(t){return function(){H(this,t)}}function K(t){return function(){G(this,t)}}function Q(t,n){return function(){(n.apply(this,arguments)?H:G)(this,t)}}function W(){this.textContent=""}function Z(t){return function(){this.textContent=t}}function $(t){return function(){var n=t.apply(this,arguments);this.textContent=null==n?"":n}}function tt(){this.innerHTML=""}function nt(t){return function(){this.innerHTML=t}}function et(t){return function(){var n=t.apply(this,arguments);this.innerHTML=null==n?"":n}}function it(){this.nextSibling&&this.parentNode.appendChild(this)}function rt(){this.previousSibling&&this.parentNode.insertBefore(this,this.parentNode.firstChild)}function ot(){return null}function at(){var t=this.parentNode;t&&t.removeChild(this)}function ut(){var t=this.cloneNode(!1),n=this.parentNode;return n?n.insertBefore(t,this.nextSibling):t}function lt(){var t=this.cloneNode(!0),n=this.parentNode;return n?n.insertBefore(t,this.nextSibling):t}function st(t){return function(){var n=this.__on;if(n){for(var e,i=0,r=-1,o=n.length;i<o;++i)e=n[i],t.type&&e.type!==t.type||e.name!==t.name?n[++r]=e:this.removeEventListener(e.type,e.listener,e.options);++r?n.length=r:delete this.__on}}}function ct(t,n,e){return function(){var i,r=this.__on,o=function(t){return function(n){t.call(this,n,this.__data__)}}(n);if(r)for(var a=0,u=r.length;a<u;++a)if((i=r[a]).type===t.type&&i.name===t.name)return this.removeEventListener(i.type,i.listener,i.options),this.addEventListener(i.type,i.listener=o,i.options=e),void(i.value=n);this.addEventListener(t.type,o,e),i={type:t.type,name:t.name,value:n,listener:o,options:e},r?r.push(i):this.__on=[i]}}function ft(t,n,e){var i=O(t),r=i.CustomEvent;"function"==typeof r?r=new r(n,e):(r=i.document.createEvent("Event"),e?(r.initEvent(n,e.bubbles,e.cancelable),r.detail=e.detail):r.initEvent(n,!1,!1)),t.dispatchEvent(r)}function dt(t,n){return function(){return ft(this,t,n)}}function ht(t,n){return function(){return ft(this,t,n.apply(this,arguments))}}A.prototype={constructor:A,appendChild:function(t){return this._parent.insertBefore(t,this._next)},insertBefore:function(t,n){return this._parent.insertBefore(t,n)},querySelector:function(t){return this._parent.querySelector(t)},querySelectorAll:function(t){return this._parent.querySelectorAll(t)}},z.prototype={add:function(t){this._names.indexOf(t)<0&&(this._names.push(t),this._node.setAttribute("class",this._names.join(" ")))},remove:function(t){var n=this._names.indexOf(t);n>=0&&(this._names.splice(n,1),this._node.setAttribute("class",this._names.join(" ")))},contains:function(t){return this._names.indexOf(t)>=0}};var vt=[null];function pt(t,n){this._groups=t,this._parents=n}function gt(t){return"string"==typeof t?new pt([[document.querySelector(t)]],[document.documentElement]):new pt([[t]],vt)}function yt(t,n){if(t=function(t){let n;for(;n=t.sourceEvent;)t=n;return t}(t),void 0===n&&(n=t.currentTarget),n){var e=n.ownerSVGElement||n;if(e.createSVGPoint){var i=e.createSVGPoint();return i.x=t.clientX,i.y=t.clientY,[(i=i.matrixTransform(n.getScreenCTM().inverse())).x,i.y]}if(n.getBoundingClientRect){var r=n.getBoundingClientRect();return[t.clientX-r.left-n.clientLeft,t.clientY-r.top-n.clientTop]}}return[t.pageX,t.pageY]}pt.prototype={constructor:pt,select:function(t){"function"!=typeof t&&(t=p(t));for(var n=this._groups,e=n.length,i=new Array(e),r=0;r<e;++r)for(var o,a,u=n[r],l=u.length,s=i[r]=new Array(l),c=0;c<l;++c)(o=u[c])&&(a=t.call(o,o.__data__,c,u))&&("__data__"in o&&(a.__data__=o.__data__),s[c]=a);return new pt(i,this._parents)},selectAll:function(t){t="function"==typeof t?y(t):function(t){return null==t?g:function(){return this.querySelectorAll(t)}}(t);for(var n=this._groups,e=n.length,i=[],r=[],o=0;o<e;++o)for(var a,u=n[o],l=u.length,s=0;s<l;++s)(a=u[s])&&(i.push(t.call(a,a.__data__,s,u)),r.push(a));return new pt(i,r)},selectChild:function(t){return this.select(null==t?x:function(t){return function(){return _.call(this.children,t)}}("function"==typeof t?t:m(t)))},selectChildren:function(t){return this.selectAll(null==t?D:function(t){return function(){return w.call(this.children,t)}}("function"==typeof t?t:m(t)))},filter:function(t){"function"!=typeof t&&(t=function(t){return function(){return this.matches(t)}}(t));for(var n=this._groups,e=n.length,i=new Array(e),r=0;r<e;++r)for(var o,a=n[r],u=a.length,l=i[r]=[],s=0;s<u;++s)(o=a[s])&&t.call(o,o.__data__,s,a)&&l.push(o);return new pt(i,this._parents)},data:function(t,n){if(!arguments.length)return Array.from(this,E);var e,i=n?S:I,r=this._parents,o=this._groups;"function"!=typeof t&&(e=t,t=function(){return e});for(var a=o.length,u=new Array(a),l=new Array(a),s=new Array(a),c=0;c<a;++c){var f=r[c],d=o[c],h=d.length,v=P(t.call(f,f&&f.__data__,c,r)),p=v.length,g=l[c]=new Array(p),y=u[c]=new Array(p);i(f,d,g,y,s[c]=new Array(h),v,n);for(var m,_,x=0,w=0;x<p;++x)if(m=g[x]){for(x>=w&&(w=x+1);!(_=y[w])&&++w<p;);m._next=_||null}}return(u=new pt(u,r))._enter=l,u._exit=s,u},enter:function(){return new pt(this._enter||this._groups.map(b),this._parents)},exit:function(){return new pt(this._exit||this._groups.map(b),this._parents)},join:function(t,n,e){var i=this.enter(),r=this,o=this.exit();return"function"==typeof t?(i=t(i))&&(i=i.selection()):i=i.append(t+""),null!=n&&(r=n(r))&&(r=r.selection()),null==e?o.remove():e(o),i&&r?i.merge(r).order():r},merge:function(t){for(var n=t.selection?t.selection():t,e=this._groups,i=n._groups,r=e.length,o=i.length,a=Math.min(r,o),u=new Array(r),l=0;l<a;++l)for(var s,c=e[l],f=i[l],d=c.length,h=u[l]=new Array(d),v=0;v<d;++v)(s=c[v]||f[v])&&(h[v]=s);for(;l<r;++l)u[l]=e[l];return new pt(u,this._parents)},selection:function(){return this},order:function(){for(var t=this._groups,n=-1,e=t.length;++n<e;)for(var i,r=t[n],o=r.length-1,a=r[o];--o>=0;)(i=r[o])&&(a&&4^i.compareDocumentPosition(a)&&a.parentNode.insertBefore(i,a),a=i);return this},sort:function(t){function n(n,e){return n&&e?t(n.__data__,e.__data__):!n-!e}t||(t=M);for(var e=this._groups,i=e.length,r=new Array(i),o=0;o<i;++o){for(var a,u=e[o],l=u.length,s=r[o]=new Array(l),c=0;c<l;++c)(a=u[c])&&(s[c]=a);s.sort(n)}return new pt(r,this._parents).order()},call:function(){var t=arguments[0];return arguments[0]=this,t.apply(null,arguments),this},nodes:function(){return Array.from(this)},node:function(){for(var t=this._groups,n=0,e=t.length;n<e;++n)for(var i=t[n],r=0,o=i.length;r<o;++r){var a=i[r];if(a)return a}return null},size:function(){let t=0;for(const n of this)++t;return t},empty:function(){return!this.node()},each:function(t){for(var n=this._groups,e=0,i=n.length;e<i;++e)for(var r,o=n[e],a=0,u=o.length;a<u;++a)(r=o[a])&&t.call(r,r.__data__,a,o);return this},attr:function(t,n){var e=c(t);if(arguments.length<2){var i=this.node();return e.local?i.getAttributeNS(e.space,e.local):i.getAttribute(e)}return this.each((null==n?e.local?N:C:"function"==typeof n?e.local?V:T:e.local?k:j)(e,n))},style:function(t,n,e){return arguments.length>1?this.each((null==n?B:"function"==typeof n?q:L)(t,n,null==e?"":e)):function(t,n){return t.style.getPropertyValue(n)||O(t).getComputedStyle(t,null).getPropertyValue(n)}(this.node(),t)},property:function(t,n){return arguments.length>1?this.each((null==n?R:"function"==typeof n?X:U)(t,n)):this.node()[t]},classed:function(t,n){var e=F(t+"");if(arguments.length<2){for(var i=Y(this.node()),r=-1,o=e.length;++r<o;)if(!i.contains(e[r]))return!1;return!0}return this.each(("function"==typeof n?Q:n?J:K)(e,n))},text:function(t){return arguments.length?this.each(null==t?W:("function"==typeof t?$:Z)(t)):this.node().textContent},html:function(t){return arguments.length?this.each(null==t?tt:("function"==typeof t?et:nt)(t)):this.node().innerHTML},raise:function(){return this.each(it)},lower:function(){return this.each(rt)},append:function(t){var n="function"==typeof t?t:h(t);return this.select((function(){return this.appendChild(n.apply(this,arguments))}))},insert:function(t,n){var e="function"==typeof t?t:h(t),i=null==n?ot:"function"==typeof n?n:p(n);return this.select((function(){return this.insertBefore(e.apply(this,arguments),i.apply(this,arguments)||null)}))},remove:function(){return this.each(at)},clone:function(t){return this.select(t?lt:ut)},datum:function(t){return arguments.length?this.property("__data__",t):this.node().__data__},on:function(t,n,e){var i,r,o=function(t){return t.trim().split(/^|\s+/).map((function(t){var n="",e=t.indexOf(".");return e>=0&&(n=t.slice(e+1),t=t.slice(0,e)),{type:t,name:n}}))}(t+""),a=o.length;if(!(arguments.length<2)){for(u=n?ct:st,i=0;i<a;++i)this.each(u(o[i],n,e));return this}var u=this.node().__on;if(u)for(var l,s=0,c=u.length;s<c;++s)for(i=0,l=u[s];i<a;++i)if((r=o[i]).type===l.type&&r.name===l.name)return l.value},dispatch:function(t,n){return this.each(("function"==typeof n?ht:dt)(t,n))},[Symbol.iterator]:function*(){for(var t=this._groups,n=0,e=t.length;n<e;++n)for(var i,r=t[n],o=0,a=r.length;o<a;++o)(i=r[o])&&(yield i)}};const mt={passive:!1},_t={capture:!0,passive:!1};function xt(t){t.stopImmediatePropagation()}function wt(t){t.preventDefault(),t.stopImmediatePropagation()}var Dt=t=>()=>t;function bt(t,{sourceEvent:n,subject:e,target:i,identifier:r,active:o,x:a,y:u,dx:l,dy:s,dispatch:c}){Object.defineProperties(this,{type:{value:t,enumerable:!0,configurable:!0},sourceEvent:{value:n,enumerable:!0,configurable:!0},subject:{value:e,enumerable:!0,configurable:!0},target:{value:i,enumerable:!0,configurable:!0},identifier:{value:r,enumerable:!0,configurable:!0},active:{value:o,enumerable:!0,configurable:!0},x:{value:a,enumerable:!0,configurable:!0},y:{value:u,enumerable:!0,configurable:!0},dx:{value:l,enumerable:!0,configurable:!0},dy:{value:s,enumerable:!0,configurable:!0},_:{value:c}})}function At(t){return!t.ctrlKey&&!t.button}function It(){return this.parentNode}function St(t,n){return null==n?{x:t.x,y:t.y}:n}function Et(){return navigator.maxTouchPoints||"ontouchstart"in this}function Pt(){var t,n,e,i,o=At,a=It,u=St,l=Et,s={},c=r("start","drag","end"),f=0,d=0;function h(t){t.on("mousedown.drag",v).filter(l).on("touchstart.drag",y).on("touchmove.drag",m,mt).on("touchend.drag touchcancel.drag",_).style("touch-action","none").style("-webkit-tap-highlight-color","rgba(0,0,0,0)")}function v(r,u){if(!i&&o.call(this,r,u)){var l=x(this,a.call(this,r,u),r,u,"mouse");l&&(gt(r.view).on("mousemove.drag",p,_t).on("mouseup.drag",g,_t),function(t){var n=t.document.documentElement,e=gt(t).on("dragstart.drag",wt,_t);"onselectstart"in n?e.on("selectstart.drag",wt,_t):(n.__noselect=n.style.MozUserSelect,n.style.MozUserSelect="none")}(r.view),xt(r),e=!1,t=r.clientX,n=r.clientY,l("start",r))}}function p(i){if(wt(i),!e){var r=i.clientX-t,o=i.clientY-n;e=r*r+o*o>d}s.mouse("drag",i)}function g(t){gt(t.view).on("mousemove.drag mouseup.drag",null),function(t,n){var e=t.document.documentElement,i=gt(t).on("dragstart.drag",null);n&&(i.on("click.drag",wt,_t),setTimeout((function(){i.on("click.drag",null)}),0)),"onselectstart"in e?i.on("selectstart.drag",null):(e.style.MozUserSelect=e.__noselect,delete e.__noselect)}(t.view,e),wt(t),s.mouse("end",t)}function y(t,n){if(o.call(this,t,n)){var e,i,r=t.changedTouches,u=a.call(this,t,n),l=r.length;for(e=0;e<l;++e)(i=x(this,u,t,n,r[e].identifier,r[e]))&&(xt(t),i("start",t,r[e]))}}function m(t){var n,e,i=t.changedTouches,r=i.length;for(n=0;n<r;++n)(e=s[i[n].identifier])&&(wt(t),e("drag",t,i[n]))}function _(t){var n,e,r=t.changedTouches,o=r.length;for(i&&clearTimeout(i),i=setTimeout((function(){i=null}),500),n=0;n<o;++n)(e=s[r[n].identifier])&&(xt(t),e("end",t,r[n]))}function x(t,n,e,i,r,o){var a,l,d,v=c.copy(),p=yt(o||e,n);if(null!=(d=u.call(t,new bt("beforestart",{sourceEvent:e,target:h,identifier:r,active:f,x:p[0],y:p[1],dx:0,dy:0,dispatch:v}),i)))return a=d.x-p[0]||0,l=d.y-p[1]||0,function e(o,u,c){var g,y=p;switch(o){case"start":s[r]=e,g=f++;break;case"end":delete s[r],--f;case"drag":p=yt(c||u,n),g=f}v.call(o,t,new bt(o,{sourceEvent:u,subject:d,target:h,identifier:r,active:g,x:p[0]+a,y:p[1]+l,dx:p[0]-y[0],dy:p[1]-y[1],dispatch:v}),i)}}return h.filter=function(t){return arguments.length?(o="function"==typeof t?t:Dt(!!t),h):o},h.container=function(t){return arguments.length?(a="function"==typeof t?t:Dt(t),h):a},h.subject=function(t){return arguments.length?(u="function"==typeof t?t:Dt(t),h):u},h.touchable=function(t){return arguments.length?(l="function"==typeof t?t:Dt(!!t),h):l},h.on=function(){var t=c.on.apply(c,arguments);return t===c?h:t},h.clickDistance=function(t){return arguments.length?(d=(t=+t)*t,h):Math.sqrt(d)},h}bt.prototype.on=function(){var t=this._.on.apply(this._,arguments);return t===this._?this:t};var Mt={id:"dragdata",statesStore:new Map,afterInit:function(t){var n={curIndex:void 0,curDatasetIndex:void 0,element:null,eventSettings:!1,floatingBar:!1,initValue:0,xAxisID:"",yAxisID:"",rAxisID:"",stacked:!1,type:void 0,isDragging:!1};Mt.statesStore.set(t.id,n),gt(t.canvas).call(Pt().container(t.canvas).on("start",(function(e){return qt(e.sourceEvent,t,n)})).on("drag",(function(e){return Rt(e.sourceEvent,t,n)})).on("end",(function(e){return Lt(e.sourceEvent,t,n)})))},beforeEvent:function(t){var n,e=Mt.statesStore.get(t.id);if(null==e?void 0:e.isDragging)return null===(n=t.tooltip)||void 0===n||n.update(),!1}};function Ct(t,n){return void 0===n||isNaN(n)||n<0?t:Math.round(t*Math.pow(10,n))/Math.pow(10,n)}function Nt(t,n,e){return Math.min(Math.max(t,n),e)}function jt(t,e,i,r,o){var a,u,l;void 0===o&&(o=Mt.statesStore.get(e.id));var s=n.getRelativePosition(t,e),c=s.x,f=s.y,d=e.scales[r],h=d.getPointPositionForValue(i+("polarArea"===(null==o?void 0:o.type)?.5:0),e.scales[r].max).angle,v=d.xCenter,p=d.yCenter,g=f-p,y=(c-v)*Math.cos(h)+g*Math.sin(h)>0?Math.sqrt(Math.pow(c-v,2)+Math.pow(f-p,2)):0,m=d.getValueForDistanceFromCenter(y);return m=Nt(m=Ct(m,null===(l=null===(u=null===(a=e.config.options)||void 0===a?void 0:a.plugins)||void 0===u?void 0:u.dragData)||void 0===l?void 0:l.round),e.scales[r].min,e.scales[r].max)}e.Chart.register(Mt);var kt=function(){return kt=Object.assign||function(t){for(var n,e=1,i=arguments.length;e<i;e++)for(var r in n=arguments[e])Object.prototype.hasOwnProperty.call(n,r)&&(t[r]=n[r]);return t},kt.apply(this,arguments)};function Tt(t){return Array.isArray(t)?function(t,n,e){if(e||2===arguments.length)for(var i,r=0,o=n.length;r<o;r++)!i&&r in n||(i||(i=Array.prototype.slice.call(n,0,r)),i[r]=n[r]);return t.concat(i||Array.prototype.slice.call(n))}([],t,!0):"object"==typeof t?kt({},t):t}function Vt(t,e,i,r,o){var a,u,l,s,c,f=r.xAxisDraggingDisabled,d=r.yAxisDraggingDisabled;if(void 0===o&&(o=Mt.statesStore.get(e.id)),!o)return i;var h=Tt(i),v=n.getRelativePosition(t,e),p=v.x,g=v.y,y=e.scales[o.xAxisID].getValueForPixel(p),m=e.scales[o.yAxisID].getValueForPixel(g),_=null===(l=null===(u=null===(a=e.config.options)||void 0===a?void 0:a.plugins)||void 0===u?void 0:u.dragData)||void 0===l?void 0:l.round;if(y=Ct(y,_),m=Ct(m,_),y=Nt(y,e.scales[o.xAxisID].min,e.scales[o.xAxisID].max),m=Nt(m,e.scales[o.yAxisID].min,e.scales[o.yAxisID].max),o.floatingBar){var x=void 0;return x="y"===(null===(s=e.config.options)||void 0===s?void 0:s.indexAxis)?y:m,Math.abs(x-h[0])<=Math.abs(x-h[1])?h[0]=x:h[1]=x,h}return void 0===h.x||f||(h.x=y),void 0!==h.y?(d||(h.y=m),h):"y"===(null===(c=e.config.options)||void 0===c?void 0:c.indexAxis)?f?h:y:d?h:m}function Ot(t,n,e){var i,r,o=null===(r=null===(i=t.config.options)||void 0===i?void 0:i.plugins)||void 0===r?void 0:r.dragData;if(null==o?void 0:o.magnet){var a=null==o?void 0:o.magnet;if("function"==typeof a.to){var u=t.data.datasets[n].data[e];return u=a.to(u),t.data.datasets[n].data[e]=u,t.update("none"),u}return null}return t.data.datasets[n].data[e]}function Bt(t,n,e,i){var r,o,a,u,l,s,c,f,d,h,v,p,g,y,m;if(void 0===i&&(i=Mt.statesStore.get(t.id)),!i)return{chartDraggingDisabled:!0,datasetDraggingDisabled:!0,xAxisDraggingDisabled:!0,yAxisDraggingDisabled:!0,dataPointDraggingDisabled:!0};var _=t.data.datasets[n],x=!1===(null===(o=null===(r=t.config.options)||void 0===r?void 0:r.plugins)||void 0===o?void 0:o.dragData),w=x||!1===_.dragData,D=null===(l=null===(u=null===(a=t.config.options)||void 0===a?void 0:a.scales)||void 0===u?void 0:u[i.xAxisID])||void 0===l?void 0:l.dragData,b=!0;return w||!0!==D&&(!0!==(null===(f=null===(c=null===(s=t.config.options)||void 0===s?void 0:s.plugins)||void 0===c?void 0:c.dragData)||void 0===f?void 0:f.dragX)||!1===D)||(b=!1),{chartDraggingDisabled:x,datasetDraggingDisabled:w,xAxisDraggingDisabled:b,yAxisDraggingDisabled:w||!1===(null===(v=null===(h=null===(d=t.config.options)||void 0===d?void 0:d.plugins)||void 0===h?void 0:h.dragData)||void 0===v?void 0:v.dragY)||!1===(null===(y=null===(g=null===(p=t.config.options)||void 0===p?void 0:p.scales)||void 0===g?void 0:g[i.yAxisID])||void 0===y?void 0:y.dragData),dataPointDraggingDisabled:w||!1===(null===(m=_.data[e])||void 0===m?void 0:m.dragData)}}function Lt(t,n,e){var i,r,o,a,u;if(void 0===e&&(e=Mt.statesStore.get(n.id)),e){var l=null===(o=null===(r=null===(i=n.options)||void 0===i?void 0:i.plugins)||void 0===r?void 0:r.dragData)||void 0===o?void 0:o.onDragEnd;if(e.curIndex=void 0,e.isDragging=!1,(null===(u=null===(a=n.config.options)||void 0===a?void 0:a.plugins)||void 0===u?void 0:u.tooltip)&&(n.config.options.plugins.tooltip.animation=e.eventSettings,n.update("none")),"function"==typeof l&&e.element){var s=e.element.datasetIndex,c=e.element.index;return l(t,s,c,Ot(n,s,c))}}}function qt(t,n,e){var i,r,o,a,u,l,s,c,f,d,h,v,p,g,y,m,_,x,w,D,b,A,I,S,E,P;void 0===e&&(e=Mt.statesStore.get(n.id));var M=null===(o=null===(r=null===(i=n.options)||void 0===i?void 0:i.plugins)||void 0===r?void 0:r.dragData)||void 0===o?void 0:o.onDragStart;if(e){var C=null!==(l=null===(u=null===(a=n.config.options)||void 0===a?void 0:a.interaction)||void 0===u?void 0:u.mode)&&void 0!==l?l:"nearest",N=null!==(c=null===(s=n.config.options)||void 0===s?void 0:s.interaction)&&void 0!==c?c:{intersect:!0};if(e.element=n.getElementsAtEventForMode(t,C,N,!1)[0],e.element){var j=e.element.datasetIndex,k=e.element.index;e.type=null!==(d=null!==(f=n.config.type)&&void 0!==f?f:n.data.datasets[j].type)&&void 0!==d?d:void 0,e.eventSettings=null===(p=null===(v=null===(h=n.config.options)||void 0===h?void 0:h.plugins)||void 0===v?void 0:v.tooltip)||void 0===p?void 0:p.animation;var T=n.data.datasets[j],V=n.getDatasetMeta(j),O=T.data[k];e.xAxisID=V.xAxisID,e.yAxisID=V.yAxisID,e.rAxisID=V.rAxisID;var B=Bt(n,j,k),L=B.datasetDraggingDisabled,q=B.xAxisDraggingDisabled,R=B.yAxisDraggingDisabled,U=B.dataPointDraggingDisabled;if(L||q&&R||U)return void(e.element=null);if("bar"===e.type){e.stacked=null!==(_=null===(m=null===(y=null===(g=n.config.options)||void 0===g?void 0:g.scales)||void 0===y?void 0:y[e.xAxisID])||void 0===m?void 0:m.stacked)&&void 0!==_?_:void 0;var X=n.data.datasets[0].data[0];e.floatingBar=null!==X&&Array.isArray(X)&&X.length>=2;var F=Vt(t,n,n.data.datasets[j].data[k],B,e);e.initValue=F-O}var Y=null===(D=null===(w=null===(x=n.config.options)||void 0===x?void 0:x.plugins)||void 0===w?void 0:w.dragData)||void 0===D?void 0:D.showTooltip;void 0!==Y&&!0!==Y||(null!==(b=(S=n.config).options)&&void 0!==b||(S.options={}),null!==(A=(E=n.config.options).plugins)&&void 0!==A||(E.plugins={}),null!==(I=(P=n.config.options.plugins).tooltip)&&void 0!==I||(P.tooltip={}),n.config.options.plugins.tooltip.animation=!1),"function"==typeof M&&e.element&&!1===M(t,j,k,O)&&(e.element=null)}}}function Rt(t,n,e){var i,r;if(void 0===e&&(e=Mt.statesStore.get(n.id)),e){var o=null===(r=null===(i=n.options)||void 0===i?void 0:i.plugins)||void 0===r?void 0:r.dragData,a=null==o?void 0:o.onDrag;if(e.element){e.curDatasetIndex=e.element.datasetIndex,e.curIndex=e.element.index,e.isDragging=!0;var u=n.data.datasets[e.curDatasetIndex].data[e.curIndex],l=Bt(n,e.curDatasetIndex,e.curIndex);if("radar"===e.type||"polarArea"===e.type)u=jt(t,n,e.curIndex,e.rAxisID,e);else if(e.stacked){u=Ct(Vt(t,n,u,l,e)-e.initValue,null==o?void 0:o.round)}else u=Vt(t,n,u,l,e);"function"==typeof a&&!1===a(t,e.curDatasetIndex,e.curIndex,u)||(n.data.datasets[e.curDatasetIndex].data[e.curIndex]=u,n.update("none"))}}}"function"==typeof SuppressedError&&SuppressedError,t.applyMagnet=Ot,t.calcCartesian=Vt,t.calcRadialLinear=jt,t.checkDraggingConfiguration=Bt,t.clipValue=Nt,t.cloneDataPoint=Tt,t.default=Mt,t.dragEndCallback=Lt,t.getElement=qt,t.roundValue=Ct,t.updateData=Rt,Object.defineProperty(t,"__esModule",{value:!0})})); |
179
package.json
{ | ||
"name": "chartjs-plugin-dragdata", | ||
"version": "2.2.5", | ||
"description": "Draggable data points for Chart.js", | ||
"main": "dist/chartjs-plugin-dragdata.js", | ||
"module": "dist/chartjs-plugin-dragdatas.esm.js", | ||
"browser": "dist/chartjs-plugin-dragdata.min.js", | ||
"scripts": { | ||
"build": "rollup -c", | ||
"dev": "rollup -c -w", | ||
"test": "node test/test.js", | ||
"pretest": "npm run build" | ||
}, | ||
"repository": { | ||
"type": "git", | ||
"url": "https://github.com/chrispahm/chartjs-plugin-dragdata.git" | ||
}, | ||
"keywords": [ | ||
"chartjs", | ||
"plugin", | ||
"drag", | ||
"draggable", | ||
"data" | ||
], | ||
"author": "Christoph Pahmeyer <christoph.pahmeyer@ilr.uni-bonn.de>", | ||
"license": "MIT", | ||
"dependencies": { | ||
"chart.js": "^3.9.1", | ||
"d3-drag": "^3.0.0", | ||
"d3-selection": "^3.0.0" | ||
}, | ||
"devDependencies": { | ||
"@rollup/plugin-commonjs": "^23.0.3", | ||
"@rollup/plugin-node-resolve": "^15.0.1", | ||
"@rollup/plugin-terser": "^0.1.0", | ||
"eslint": "^8.28.0", | ||
"eslint-config-standard": "^17.0.0", | ||
"eslint-plugin-import": "^2.26.0", | ||
"eslint-plugin-node": "^11.1.0", | ||
"eslint-plugin-promise": "^6.1.1", | ||
"playwright": "^1.28.1", | ||
"rollup": "^3.5.0" | ||
} | ||
"name": "chartjs-plugin-dragdata", | ||
"version": "2.3.0", | ||
"description": "Draggable data points for Chart.js", | ||
"exports": { | ||
"import": "./dist/chartjs-plugin-dragdata.esm.js", | ||
"require": "./dist/chartjs-plugin-dragdata.js", | ||
"types": "./dist/typeAugmentations.d.ts" | ||
}, | ||
"main": "dist/chartjs-plugin-dragdata.js", | ||
"module": "dist/chartjs-plugin-dragdata.esm.js", | ||
"browser": "dist/chartjs-plugin-dragdata.min.js", | ||
"types": "dist/typeAugmentations.d.ts", | ||
"scripts": { | ||
"build": "npm run rollup:base", | ||
"build:no-coverage": "npx cross-env DISABLE_ISTANBUL_COVERAGE_AT_BUILD=true npm run rollup:base", | ||
"build:watch": "npm run rollup:base -- --watch", | ||
"build:watch:no-coverage": "npx cross-env DISABLE_ISTANBUL_COVERAGE_AT_BUILD=true npm run rollup:base -- --watch", | ||
"build:pages": "npx tsx pages/src/bundle.ts", | ||
"build:pages:watch": "npx tsx pages/src/watch.ts", | ||
"cleanCoverage": "npx tsx scripts/cleanCoverage.ts", | ||
"collectCoverage": "npx tsx scripts/collectCoverage.ts && npx nyc merge coverage/merged/src coverage/merged/report/merged-coverage.json && npx nyc report -t coverage/merged/report --report-dir coverage/merged/report/html --reporter=html", | ||
"lint": "npx eslint .", | ||
"lint:fix": "npx eslint . --fix", | ||
"nyc:base": "npx cross-env NODE_ENV=test nyc", | ||
"pretest": "npm run build && npm run build:pages && npm run cleanCoverage", | ||
"prepack": "npm run build:no-coverage", | ||
"prepare": "npx patch-package && npx playwright install && npx lefthook install", | ||
"posttest": "npm run collectCoverage", | ||
"rollup:base": "rollup --config rollup.config.js", | ||
"test": "npm run test:unit && npm run test:integration && npm run test:e2e", | ||
"test:unit": "npx jest --coverage --coverageDirectory=coverage/unit --config jest.config.ts --selectProjects unit", | ||
"test:integration": "npx jest --coverage --coverageDirectory=coverage/integration --config jest.config.ts --selectProjects integration", | ||
"test:e2e": "npm run test:e2e:base", | ||
"test:e2e:base": "npm run nyc:base -- --report-dir=coverage/e2e playwright test", | ||
"test:e2e:ui": "npm run test:e2e:base -- --ui", | ||
"test:e2e:headed": "npm run test:e2e:base --headed", | ||
"test:e2e:updateSnapshots": "npm run test:e2e -- -- --update-snapshots", | ||
"release": "release-it" | ||
}, | ||
"repository": { | ||
"type": "git", | ||
"url": "https://github.com/artus9033/chartjs-plugin-dragdata.git" | ||
}, | ||
"keywords": [ | ||
"chartjs", | ||
"plugin", | ||
"drag", | ||
"draggable", | ||
"data" | ||
], | ||
"author": "Christoph Pahmeyer <christoph.pahmeyer@ilr.uni-bonn.de>", | ||
"license": "MIT", | ||
"peerDependencies": { | ||
"chart.js": "^3.9.1 || ^4.0.1" | ||
}, | ||
"dependencies": { | ||
"d3-drag": "^3.0.0", | ||
"d3-selection": "^3.0.0" | ||
}, | ||
"devDependencies": { | ||
"@babel/core": "^7.24.9", | ||
"@babel/plugin-transform-modules-commonjs": "^7.24.8", | ||
"@babel/preset-env": "^7.25.0", | ||
"@babel/preset-react": "^7.24.7", | ||
"@babel/preset-typescript": "^7.24.7", | ||
"@callstack/eslint-config": "^14.2.0", | ||
"@commitlint/config-conventional": "^19.2.2", | ||
"@playwright/test": "^1.45.3", | ||
"@release-it/conventional-changelog": "^8.0.1", | ||
"@rollup/plugin-commonjs": "^26.0.1", | ||
"@rollup/plugin-node-resolve": "^15.2.3", | ||
"@rollup/plugin-terser": "^0.4.4", | ||
"@rollup/plugin-typescript": "^11.1.6", | ||
"@testing-library/jest-dom": "^6.4.8", | ||
"@testing-library/react": "^16.0.0", | ||
"@testing-library/vue": "^8.1.0", | ||
"@types/config": "^3.3.4", | ||
"@types/d3-drag": "^3.0.7", | ||
"@types/d3-selection": "^3.0.10", | ||
"@types/ejs": "^3.1.5", | ||
"@types/jest": "^29.5.12", | ||
"@types/lodash": "^4.17.7", | ||
"@types/react": "^18.3.3", | ||
"@typescript-eslint/eslint-plugin": "^7.17.0", | ||
"@typescript-eslint/parser": "^7.17.0", | ||
"@vue/vue3-jest": "^29.2.6", | ||
"babel-jest": "^29.7.0", | ||
"canvas": "^2.11.2", | ||
"chart.js": "^4.4.3", | ||
"chartjs-adapter-date-fns": "^3.0.0", | ||
"chartjs-plugin-datalabels": "^2.2.0", | ||
"chokidar": "^3.6.0", | ||
"commitlint": "^19.3.0", | ||
"config": "^3.3.12", | ||
"cross-env": "^7.0.3", | ||
"d3-drag": "^3.0.0", | ||
"d3-selection": "^3.0.0", | ||
"date-fns": "^3.6.0", | ||
"ejs": "^3.1.10", | ||
"eslint": "^8.57.0", | ||
"eslint-config-prettier": "^9.1.0", | ||
"eslint-plugin-prettier": "^5.2.1", | ||
"jest": "^29.7.0", | ||
"jest-environment-jsdom": "^29.7.0", | ||
"jest-extended": "^4.0.2", | ||
"lefthook": "^1.7.9", | ||
"lint-staged": "^15.2.7", | ||
"lodash": "^4.17.21", | ||
"node-sass": "^9.0.0", | ||
"nyc": "^17.0.0", | ||
"patch-package": "^8.0.0", | ||
"playwright": "^1.45.3", | ||
"playwright-test-coverage": "^1.2.12", | ||
"prettier": "^3.3.3", | ||
"react": "^18.3.1", | ||
"react-chartjs-2": "^5.2.0", | ||
"react-dom": "^18.3.1", | ||
"release-it": "^17.2.1", | ||
"resize-observer-polyfill": "^1.5.1", | ||
"rollup": "^4.19.1", | ||
"rollup-plugin-copy": "^3.5.0", | ||
"rollup-plugin-istanbul": "^5.0.0", | ||
"ts-node": "^10.9.2", | ||
"tslib": "^2.6.3", | ||
"tsx": "^4.16.2", | ||
"typescript": "^5.5.4", | ||
"vue": "^3.4.34", | ||
"vue-chartjs": "^5.3.1" | ||
}, | ||
"files": [ | ||
"dist/**/*", | ||
"!dist/test/**/*", | ||
"package.json", | ||
"LICENSE", | ||
"README.md", | ||
"CHANGELOG.md" | ||
] | ||
} |
313
README.md
# chartjs-plugin-dragdata.js | ||
**Now compatible with Chart.js v3 🎉** | ||
**Looking for a version compatible to Chart.js < 2.9.x? Then visit the [v2 branch](https://github.com/chrispahm/chartjs-plugin-dragdata/tree/v2)!** | ||
 | ||
[](https://github.com/artus9033/chartjs-plugin-dragdata/actions/workflows/ci.yml) | ||
[](https://github.com/artus9033/chartjs-plugin-dragdata/releases) | ||
[](https://www.npmjs.com/package/chartjs-plugin-dragdata/v/latest) | ||
 | ||
 | ||
[](https://codecov.io/gh/artus9033/chartjs-plugin-dragdata) | ||
<a href="https://github.com/chartjs/awesome"><img src="https://awesome.re/badge-flat2.svg" alt="Awesome"></a> | ||
A plugin for Chart.js >= 2.4.0 | ||
Makes data points draggable. Supports touch events. | ||
A plugin for Chart.js that makes data points draggable. Supports touch events & arbitrary Chart.js [interaction modes](https://www.chartjs.org/docs/latest/samples/tooltip/interactions.html). | ||
**Compatible with Chart.js v4, v3 & v2.4+ 🎉** | ||
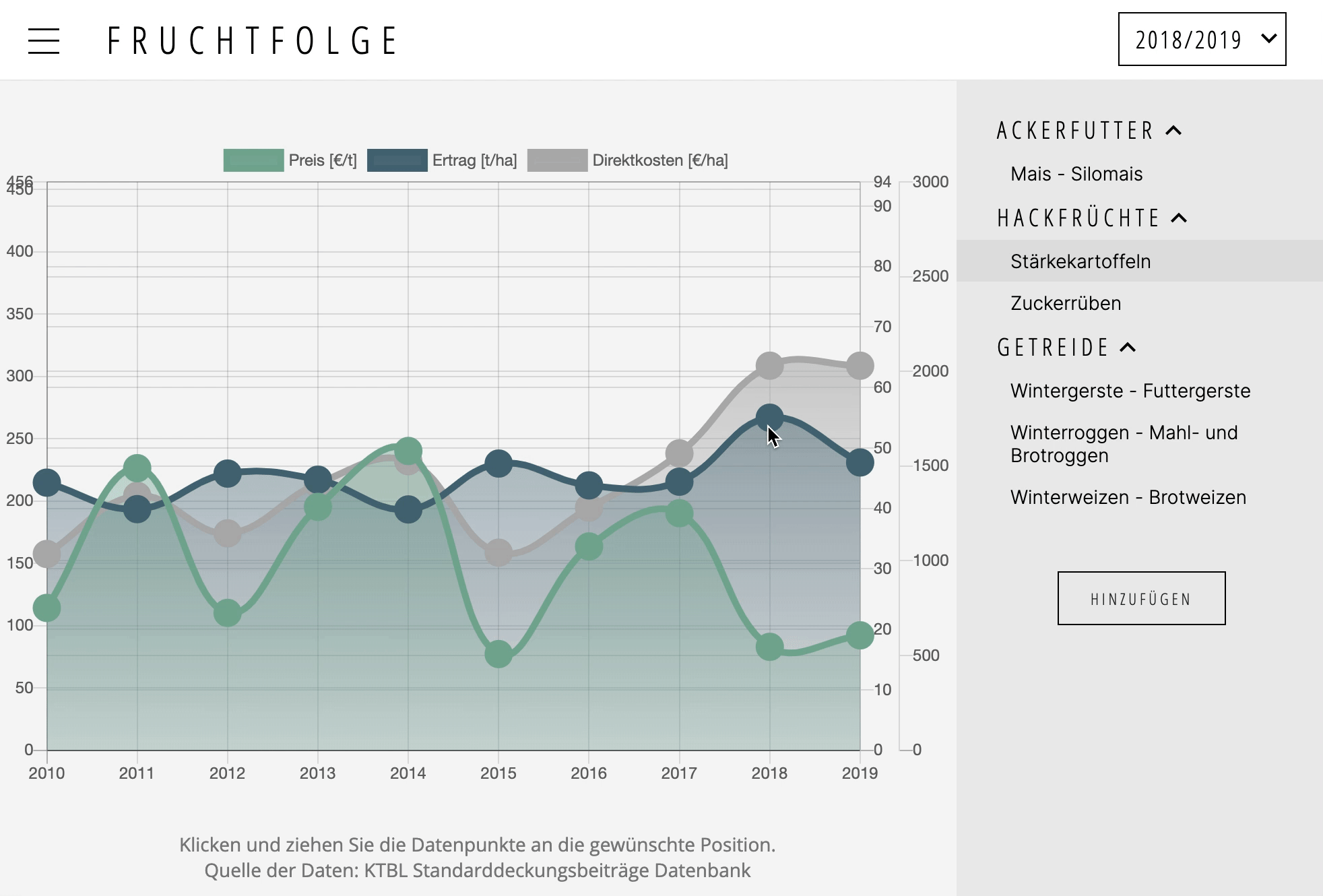 | ||
### Table of contents | ||
- [chartjs-plugin-dragdata.js](#chartjs-plugin-dragdatajs) | ||
- [Table of contents](#table-of-contents) | ||
- [Chart.js version compatibility](#chartjs-version-compatibility) | ||
- [Online demos](#online-demos) | ||
- [Installation](#installation) | ||
- [npm](#npm) | ||
- [CDN](#cdn) | ||
- [Configuration](#configuration) | ||
- [Applying a 'magnet'](#applying-a-magnet) | ||
- [Touch devices](#touch-devices) | ||
- [Gotchas](#gotchas) | ||
- [Contributing](#contributing) | ||
- [Additional scripts](#additional-scripts) | ||
- [License](#license) | ||
--- | ||
### Chart.js version compatibility | ||
| Chart.js version | chartjs-plugin-dragdata version | git branch | | ||
| :--------------: | :-----------------------------: | :-------------------------------------------------------------------------------: | | ||
| 4.x | 2.x | [master branch](https://github.com/artus9033/chartjs-plugin-dragdata/tree/master) | | ||
| 3.x | 2.x | [v3 branch](https://github.com/artus9033/chartjs-plugin-dragdata/tree/v3) | | ||
| 2.4.x ~ 2.9.4 | 1.x | [v2 branch](https://github.com/artus9033/chartjs-plugin-dragdata/tree/v2) | | ||
### Online demos | ||
| Chart Type | Demo | Source | | ||
|:-------------------------------------------------------------------------------------------------------------------------------|:---------------------------------------------------------------------------------------|:---------------------------------------------------------------------------------------------------------------------| | ||
| Bar - Simple Bar | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/bar.html) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/bar.html) | | ||
| Bubble - Simple Bubble | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/bubble.html) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/bubble.html) | | ||
| Floating bar - simple floating bars | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/floatingBar.html) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/floatingBar.html) | | ||
| Floating bar - simple floating bars, horizontal | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/horizontalFloatingBar.html) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/horizontalFloatingBar.html) | | ||
| Horizontal Bar - Simple Horizontal Bar | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/horizontalBar.html) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/horizontalBar.html) | | ||
| Line - Single Y-Axis | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/index.html) | | ||
| Line - Dual Y-Axis | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/dualAxis.html) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/dualAxis.html) | | ||
| Line - Drag multiple points | [demo](https://jsfiddle.net/45nurh9L/3/) | [source](https://jsfiddle.net/45nurh9L/3/) | | ||
| Line - Small | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/smallChart.html) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/smallChart.html) | | ||
| Line - React Fiddle | [demo](https://jsfiddle.net/16kvxd4u/3/) | [source](https://jsfiddle.net/16kvxd4u/3/) | | ||
| Line - Drag x-, and y-axis (scatter chart) | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/scatter.html) | [source](https://chrispahm.github.io/chartjs-plugin-dragdata/scatter.html) | | ||
| Line - Drag dates (x and y axis) | [demo](https://jsfiddle.net/f72kz348/9/) | [source](https://jsfiddle.net/f72kz348/9/) | | ||
| Line - Zoom, Pan, and drag data points (combination with [chartjs-plugin-zoom](https://github.com/chartjs/chartjs-plugin-zoom) | [demo](https://jsfiddle.net/s6xn3q9f/1/) | [source](https://jsfiddle.net/s6xn3q9f/1/) | | ||
| Mixed - Bar, Bubble, and line Chart | [demo](https://jsfiddle.net/rqbcs6ep/3/) | [source](https://jsfiddle.net/rqbcs6ep/3/) | | ||
| Radar - Simple Radar | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/radar.html) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/radar.html) | | ||
| Polar - Simple Polar Area Chart | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/polar.html) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/polar.html) | | ||
| Stacked Bar - Simple Stacked Bar | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/stackedBar.html) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/stackedBar.html) | | ||
| Stacked Bar - GANTT Chart | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/ganttChart.html) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/ganttChart.html) | | ||
| Stacked Horizontal Bar - Simple Stacked Horizontal Bar | [demo](https://chrispahm.github.io/chartjs-plugin-dragdata/stackedHorizontalBar.html) | [source](https://raw.githubusercontent.com/chrispahm/chartjs-plugin-dragdata/master/docs/stackedHorizontalBar.html) | | ||
| Chart Type | Demo | Source | | ||
| :--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- | :---------------------------------------------------------------------------------------------- | :----------------------------------------------------------------- | | ||
| Bar - simple bar | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/bar.html) | [source](pages/dist-demos/bar.html#L36) | | ||
| Horizontal Bar - simple horizontal Bar | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/bar-horizontal.html) | [source](pages/dist-demos/bar-horizontal.html#L36) | | ||
| Floating bar - simple floating bars | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/bar-floating.html) | [source](pages/dist-demos/bar-floating.html#L36) | | ||
| Floating bar - simple floating bars, horizontal | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/bar-floating-horizontal.html) | [source](pages/dist-demos/bar-floating-horizontal.html#L38) | | ||
| Stacked Bar - simple stacked bar | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/bar-stacked.html) | [source](pages/dist-demos/bar-stacked.html#L36) | | ||
| Stacked Horizontal Bar - simple stacked horizontal bar | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/bar-stacked-horizontal.html) | [source](pages/dist-demos/bar-stacked-horizontal.html#L38) | | ||
| Stacked Bar - GANTT chart | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/gantt.html) | [source](pages/dist-demos/gantt.html#L36) | | ||
| Bubble - simple bubble | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/bubble.html) | [source](pages/dist-demos/bubble.html#L36) | | ||
| Bubble - draggable x-axis | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/bubble-x-only.html) | [source](pages/dist-demos/bubble-x-only.html#L36) | | ||
| Line - simple, single y-axis | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/line-linear.html) | [source](pages/dist-demos/line-linear.html#L36) | | ||
| Line - dual y-axis | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/line-dual-y-axis.html) | [source](pages/dist-demos/line-dual-y-axis.html#L36) | | ||
| Line - single y-axis, categorical x-axis | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/line-categorical.html) | [source](pages/dist-demos/line-categorical.html#L36) | | ||
| Line - single y-axis, [custom (max value)](pages/dist-demos/line-linear-custom-interaction.html#L39) [interaction mode](https://www.chartjs.org/docs/latest/samples/tooltip/interactions.html) | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/line-linear-custom-interaction.html) | [source](pages/dist-demos/line-linear-custom-interaction.html#L63) | | ||
| Line - drag multiple points | [demo](https://jsfiddle.net/45nurh9L/3/) | [source](https://jsfiddle.net/45nurh9L/3/) | | ||
| Line - react fiddle | [demo](https://jsfiddle.net/16kvxd4u/3/) | [source](https://jsfiddle.net/16kvxd4u/3/) | | ||
| Line - drag x-, and y-axis (scatter chart) | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/scatter.html) | [source](pages/dist-demos/scatter.html#L36) | | ||
| Line - drag dates (x and y axis) | [demo](https://jsfiddle.net/f72kz348/9/) | [source](https://jsfiddle.net/f72kz348/9/) | | ||
| Line - zoom, pan, and drag data points (combination with [chartjs-plugin-zoom](https://github.com/chartjs/chartjs-plugin-zoom)) | [demo](https://jsfiddle.net/s6xn3q9f/1/) | [source](https://jsfiddle.net/s6xn3q9f/1/) | | ||
| Mixed - bar, bubble, and line chart | [demo](https://jsfiddle.net/rqbcs6ep/3/) | [source](https://jsfiddle.net/rqbcs6ep/3/) | | ||
| Radar - simple radar | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/radar.html) | [source](pages/dist-demos/radar.html#L36) | | ||
| Polar - simple polar area chart | [demo](https://artus9033.github.io/chartjs-plugin-dragdata/polar.html) | [source](pages/dist-demos/polar.html#L36) | | ||
Click here to learn [how to use this plugin in an Observable notebook](https://observablehq.com/@chrispahm/draggable-data-charts). | ||
@@ -40,17 +77,25 @@ | ||
``` | ||
```bash | ||
npm install chartjs-plugin-dragdata | ||
``` | ||
### yarn | ||
```bash | ||
yarn add chartjs-plugin-dragdata | ||
``` | ||
### CDN | ||
In browsers, you may use the following script tag: | ||
In browsers, you may simply add the following script tag: | ||
```html | ||
<script src="https://cdn.jsdelivr.net/npm/chartjs-plugin-dragdata@latest/dist/chartjs-plugin-dragdata.min.js"></script> | ||
``` | ||
<script src="https://cdn.jsdelivr.net/npm/chartjs-plugin-dragdata@2.2.3/dist/chartjs-plugin-dragdata.min.js"></script> | ||
``` | ||
Or, download a release archive file from the dist folder. | ||
Or, download a release archive file from [releases](https://github.com/artus9033/chartjs-plugin-dragdata/releases). | ||
## Configuration | ||
The following Chart.js sample configuration displays (*most*) of the available | ||
The following Chart.js sample configuration displays (_most_) of the available | ||
configuration options of the `dragdata` plugin. | ||
@@ -60,59 +105,56 @@ | ||
const draggableChart = new Chart(ctx, { | ||
type: 'line', | ||
data: { | ||
labels: ["Red", "Blue", "Yellow", "Green", "Purple", "Orange"], | ||
datasets: [{ | ||
label: '# of Votes', | ||
data: [12, 19, 3, 5, 2, 3], | ||
fill: true, | ||
tension: 0.4, | ||
borderWidth: 1, | ||
pointHitRadius: 25 // for improved touch support | ||
// dragData: false // prohibit dragging this dataset | ||
// same as returning `false` in the onDragStart callback | ||
// for this datsets index position | ||
}] | ||
}, | ||
options: { | ||
plugins: { | ||
dragData: { | ||
round: 1, // rounds the values to n decimal places | ||
// in this case 1, e.g 0.1234 => 0.1) | ||
showTooltip: true, // show the tooltip while dragging [default = true] | ||
// dragX: true // also enable dragging along the x-axis. | ||
// this solely works for continous, numerical x-axis scales (no categories or dates)! | ||
onDragStart: function(e, element) { | ||
/* | ||
// e = event, element = datapoint that was dragged | ||
// you may use this callback to prohibit dragging certain datapoints | ||
// by returning false in this callback | ||
if (element.datasetIndex === 0 && element.index === 0) { | ||
// this would prohibit dragging the first datapoint in the first | ||
// dataset entirely | ||
return false | ||
} | ||
*/ | ||
}, | ||
onDrag: function(e, datasetIndex, index, value) { | ||
/* | ||
// you may control the range in which datapoints are allowed to be | ||
// dragged by returning `false` in this callback | ||
if (value < 0) return false // this only allows positive values | ||
if (datasetIndex === 0 && index === 0 && value > 20) return false | ||
*/ | ||
}, | ||
onDragEnd: function(e, datasetIndex, index, value) { | ||
// you may use this callback to store the final datapoint value | ||
// (after dragging) in a database, or update other UI elements that | ||
// dependent on it | ||
}, | ||
} | ||
}, | ||
scales: { | ||
y: { | ||
// dragData: false // disables datapoint dragging for the entire axis | ||
} | ||
} | ||
} | ||
}) | ||
type: "line", | ||
data: { | ||
labels: ["Red", "Blue", "Yellow", "Green", "Purple", "Orange"], | ||
datasets: [ | ||
{ | ||
label: "# of Votes", | ||
data: [12, 19, 3, 5, 2, 3], | ||
fill: true, | ||
tension: 0.4, | ||
borderWidth: 1, | ||
pointHitRadius: 25, // for improved touch support | ||
// dragData: false // prohibit dragging this dataset | ||
// same as returning `false` in the onDragStart callback | ||
// for this datsets index position | ||
}, | ||
], | ||
}, | ||
options: { | ||
plugins: { | ||
dragData: { | ||
round: 1, // rounds the values to n decimal places | ||
// in this case 1, e.g 0.1234 => 0.1) | ||
showTooltip: true, // show the tooltip while dragging [default = true] | ||
// dragX: true // also enable dragging along the x-axis. | ||
// this solely works for continous, numerical x-axis scales (no categories or dates)! | ||
onDragStart: function (event, datasetIndex, index, value) { | ||
// you may use this callback to prohibit dragging certain datapoints | ||
// by returning false in this callback | ||
if (element.datasetIndex === 0 && element.index === 0) { | ||
// this would prohibit dragging the first datapoint in the first | ||
//dataset entirely | ||
return false; | ||
} | ||
}, | ||
onDrag: function (event, datasetIndex, index, value) { | ||
// you may control the range in which datapoints are allowed to be | ||
// dragged by returning `false` in this callback | ||
if (value < 0) return false; // this only allows positive values | ||
if (datasetIndex === 0 && index === 0 && value > 20) return false; | ||
}, | ||
onDragEnd: function (event, datasetIndex, index, value) { | ||
// you may use this callback to store the final datapoint value | ||
// (after dragging) in a database, or update other UI elements that | ||
// dependent on it | ||
}, | ||
}, | ||
}, | ||
scales: { | ||
y: { | ||
dragData: false, // disables datapoint dragging for the entire axis | ||
}, | ||
}, | ||
}, | ||
}); | ||
``` | ||
@@ -125,3 +167,3 @@ | ||
type: 'line', // or radar, bar, horizontalBar, bubble | ||
data: {...}, | ||
data: {...}, | ||
options: { | ||
@@ -139,3 +181,2 @@ plugins: {dragData: true}, | ||
### Applying a 'magnet' | ||
@@ -150,3 +191,3 @@ | ||
type: 'line', // or radar, bar, bubble | ||
data: {...}, | ||
data: {...}, | ||
options: { | ||
@@ -156,3 +197,3 @@ plugins: { | ||
magnet: { | ||
to: Math.round // to: (value) => value + 5 | ||
to: Math.round // to: (value) => value + 5 | ||
} | ||
@@ -166,6 +207,8 @@ } | ||
## Touch devices | ||
In order to support touch events, the [`pointHitRadius`](https://www.chartjs.org/docs/latest/charts/line.html#point-styling) option should be set to a value greater than `25`. You can find working example configurations in the `docs/*.html` files. Also note, that mobile devices (and thus touch events) can be simulated with the [device mode in the Chrome DevTools](https://developers.google.com/web/tools/chrome-devtools/device-mode/). | ||
In order to support touch events, the [`pointHitRadius`](https://www.chartjs.org/demos/dist/latest/charts/line.html#point-styling) option should be set to a value greater than `25`. You can find working example configurations in the `pages/dist-demos/*.html` files. Also note, that mobile devices (and thus touch events) can be simulated with the [device mode in the Chrome DevTools](https://developers.google.com/web/tools/chrome-devtools/device-mode/). | ||
## Gotchas | ||
When working with a module bundler (e.g. Rollup/Webpack) and a framework (e.g. Vue.js/React/Angular), you still need to import the plugin library after installing. | ||
When working with a module bundler (e.g. Rollup/Webpack) and a framework (e.g. Vue.js/React/Angular), you still need to import the plugin library after installing. | ||
Here's a small example for a Vue.js component | ||
@@ -179,42 +222,68 @@ | ||
</template> | ||
<script> | ||
import { Chart, registerables } from 'chart.js' | ||
// load the options file externally for better readability of the component. | ||
// In the chartOptions object, make sure to add "dragData: true" etc. | ||
import chartOptions from '~/assets/js/labour.js' | ||
import 'chartjs-plugin-dragdata' | ||
import { Chart, registerables } from 'chart.js' | ||
// Load the options file externally for better readability of the component. | ||
// In the chartOptions object, make sure to add "dragData: true" etc. | ||
import chartOptions from '~/assets/js/labour.js' | ||
import 'chartjs-plugin-dragdata' | ||
export default { | ||
data() { | ||
return { | ||
chartOptions | ||
export default { | ||
data() { | ||
return { | ||
chartOptions | ||
} | ||
}, | ||
mounted() { | ||
Chart.register(...registerables) | ||
this.createChart('chart', this.chartOptions) | ||
}, | ||
methods: { | ||
createChart(chartId, chartData) { | ||
const ctx = document.getElementById(chartId) | ||
const myChart = new Chart(ctx, { | ||
type: chartData.type, | ||
data: chartData.data, | ||
options: chartData.options, | ||
}) | ||
} | ||
} | ||
}, | ||
mounted() { | ||
Chart.register(...registerables) | ||
this.createChart('chart', this.chartOptions) | ||
}, | ||
methods: { | ||
createChart(chartId, chartData) { | ||
const ctx = document.getElementById(chartId) | ||
const myChart = new Chart(ctx, { | ||
type: chartData.type, | ||
data: chartData.data, | ||
options: chartData.options, | ||
}) | ||
} | ||
} | ||
} | ||
</script> | ||
<style> | ||
</style> | ||
``` | ||
## Contributing | ||
Please feel free to submit an issue or a pull request! | ||
If you make changes to the src/index.js file, don't forget to `npm run build` and | ||
manually test your changes against all demos in the docs folder. | ||
If you make changes to the source files, don't forget to: | ||
- `npm run build` to build the library (outputs will be written to `dist/`) or `npm run build:watch` to run the rollup packager in watch mode and build the library each time the source files change | ||
- `npm run build:pages` or `npm run build:pages:watch` to build the demo & E2E test pages files; outputs will be written to `pages/dist-demos/` for demos, and to `/pages/dist-e2e` for E2E tests (the latter ones containing `eval`-using code for injecting data from Playwright) | ||
- run unit, integration & E2E tests with `npm run test` (or separately with `npm run test:unit`, `npm run test:integration`, `npm run test:e2e`) | ||
- if your changes do change the chart's appearance after performing some interaction, update snapshots by running the command `npm run test:e2e:updateSnapshots` | ||
- manually test your changes to ensure that they do work and don't break existing features | ||
- when committing, please remember that the commit message must match the [conventional commits](https://www.conventionalcommits.org/en/v1.0.0/) convention; lefthook will check that for you automatically | ||
- create a PR | ||
### Additional scripts | ||
The build command comes in four variants: | ||
- `build` which builds bundles for all targets: | ||
- `chartjs-plugin-dragdata.esm.js`- ESM production, minified (tersed) bundle | ||
- `chartjs-plugin-dragdata.js`- UMD production, non-minified bundle | ||
- `chartjs-plugin-dragdata.min.js` - UMD production, minified (tersed) bundle | ||
- `chartjs-plugin-dragdata-test.js` - bundle for Jest unit tests with coverage instrumentation code injected by `rollup-istanbul-plugin` | ||
- `chartjs-plugin-dragdata-test-browser.js` - bundle for E2E test with additional test-only exports used for automatic tests, allows for injection of urlencoded configuration for Playwright and with coverage instrumentation code injected by `rollup-istanbul-plugin` | ||
- `build:no-coverage` which works like `build`, but does not include the `rollup-istanbul-plugin`, which may sometimes be helpful when you alter the code and encounter an error when running tests, making the result bundle not contain rubbish code injected by Istanbul | ||
- `build:watch` which works as `build`, but watches source files for changes and triggers a rebuild whenever they change | ||
- `build:watch:no-coverage` which works like a mix of `build:watch` and `build:no-coverage` | ||
Scripts for linting are also provided: | ||
- `lint` which runs ESLint on the project | ||
- `lint:fix` which runs ESLint on the project in fix mode | ||
## License | ||
chartjs-plugin-dragdata.js is available under the [MIT license](http://opensource.org/licenses/MIT). |
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
Debug access
Supply chain riskUses debug, reflection and dynamic code execution features.
Found 1 instance in 1 package
Minified code
QualityThis package contains minified code. This may be harmless in some cases where minified code is included in packaged libraries, however packages on npm should not minify code.
Found 1 instance in 1 package
1984
2
282
0
168255
68
28
1
+ Added@kurkle/color@0.3.4(transitive)
+ Addedchart.js@4.4.7(transitive)
- Removedchart.js@^3.9.1
- Removedchart.js@3.9.1(transitive)