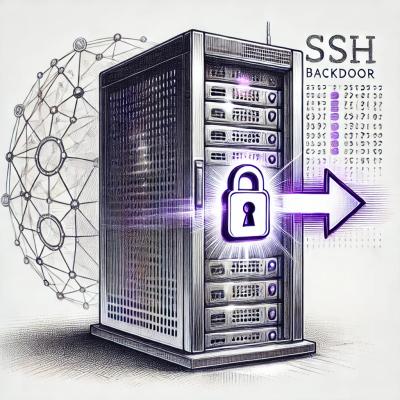
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
chemicaljs
Advanced tools
Easily create your own web proxy with no experience required.
[!IMPORTANT]
I am in the process of creating a documentation site. These docs might be outdated.
A simple example can be found in /example/
.
A example with the vite plugin can be found in /example-vite/
.
A example with all the components can be found in /example-components/
.
A real world styled example can be found in /example-styled/
.
A example with a build command and an external wisp server can be found in /example-build/
.
Create a new Node.js project and create a script file for the server.
Install Chemical npm install chemicaljs
.
Import ChemicalServer
and create a new server.
import { ChemicalServer } from "chemicaljs";
const [app, listen] = new ChemicalServer();
You can pass options to disable proxy services and set the default service.
const [app, listen] = new ChemicalServer({
default: "rammerhead",
uv: true,
scramjet: false,
rammerhead: true,
hostname_blacklist: [ /google\.com/, /reddit\.com/ ],
hostname_whitelist: [ /example\.com/ ]
});
hostname_whitelist
overrides hostname_blacklist
if you try to set them both.
app
which is an express app. You may need to import express for certain APIs.app.get("/", function(req, res){
res.send("Hello World");
});
Use app.serveChemical()
to serve Chemical routes.
Use serveChemical
after main routes but before 404 routes. Example 404 page.
app.use((req, res) => {
res.status(404);
res.send("404 Error");
});
listen
on a port of your choosing.listen(3000);
Below is an example of a simple backend. This example will setup Chemical and serve the "public" folder along with the index.html
file as /
and .html
files without the extension.
import { ChemicalServer } from "chemicaljs";
import express from "express";
const [app, listen] = new ChemicalServer();
const port = process.env.PORT || 3000;
app.use(express.static("public", {
index: "index.html",
extensions: ["html"]
}));
app.serveChemical();
app.use((req, res) => {
res.status(404);
res.send("404 Error");
});
listen(port, () => {
console.log(`Chemical demo listening on port ${port}`);
});
Want to use Chemical without using a custom wisp and/or rammerhead server or without a server at all?
Using the build command you can clone all needed assets into your build folder (like BareMux, Libcurl, and proxies enabled). Note that UV and Scramjet will need an external wisp server to function if you do not provide your own and Rammerhead will not work without your own Rammerhead server running.
ChemicalBuild
and create a new build.const build = new ChemicalBuild({
path: "dist",
default: "uv",
uv: true,
scramjet: true,
rammerhead: false,
});
Use build.write()
to write into the build path.
Use build.write(true)
to first empty the build path.
Below if a full example of building Chemical.
const build = new ChemicalBuild({
path: "dist",
default: "uv",
uv: true,
scramjet: true,
rammerhead: false,
});
await build.write(true);
In your project create a folder to store your static assets. Create an index.html file which will be the homepage of your website.
<script src="/chemical.js"></script>
If you want to set the wisp server to an external server just change the wisp
attribute.
<script data-wisp="wss://wisp.mercurywork.shop/" src="/chemical.js"></script>
If you want to set the transport just change the transport
attribute. Choose libcurl
(default becuase it supports Firefox) or epoxy
.
<script data-transport="epoxy" src="/chemical.js"></script>
chemical.encode
.await chemical.encode("https://example.com")
Optional: Change service to uv
, scramjet
, or rammerhead
. Defaults to uv
or server option.
await chemical.encode("https://example.com", {
service: "rammerhead"
})
chemical.decode
const encodedURL = await chemical.encode("https://example.com")
const decodedURL = await chemical.decode(encodedURL)
autoHttps
to make example.com
> https://example.com
await chemical.encode("example.com", {
autoHttps: true
})
searchEngine
property. Use a search engine URL with "%s" in place of query.await chemical.encode("cheese", {
searchEngine: "https://www.google.com/search?q=%s"
})
if (window.chemical.loaded) {
//Chemical is loaded
}
window.addEventListener("chemicalLoaded", function(e) {
//Chemical has loaded
});
chemical.setTransport
await chemical.setTransport("libcurl") //libcurl or epoxy
chemical.setWisp
await chemical.setWisp("wss://wisp.mercurywork.shop/")
Below is a simple example of a simple input that redirects to the encoded URL when the user presses enter. It checks if there is any input and if Chemical has loaded before loading.
<h1>Chemical Example</h1>
<input id="search" placeholder="Enter URL">
<script src="/chemical.js"></script>
<script>
const search = document.getElementById("search");
search.addEventListener("keydown", async function (e) {
if (e.key == "Enter" && chemical.loaded && e.target.value) {
window.location = await chemical.encode(e.target.value)
}
})
</script>
Fetch websites through Wisp using chemical.fetch
. This works the same as the fetch API.
Get search suggestions via DuckDuckGo using chemical.getSuggestions
await chemical.getSuggestions("google")
Create a new vite app and open vite.config.js
or vite.config.ts
Import ChemicalVitePlugin
and add it to plugins.
import { defineConfig } from "vite"
import { ChemicalVitePlugin } from "chemicaljs"
export default defineConfig({
plugins: [/*Other plugins*/ChemicalVitePlugin()],
})
You can pass options to just like on the main server.
export default defineConfig({
plugins: [
ChemicalVitePlugin({
default: "rammerhead",
uv: true,
scramjet: false,
rammerhead: true,
hostname_blacklist: [ /google\.com/, /reddit\.com/ ],
hostname_whitelist: [ /example\.com/ ]
})
],
})
Setup a proxy site with easy HTML components.
<script src="/chemical.js"></script>
<script src="/chemical.components.js"></script>
An anchor link but it automatically encodes the URL.
<a data-href="https://example.com" is="chemical-link">Link</a>
You can also add data-service="uv"
, data-autoHttps
, and data-search-engine="https://www.google.com/search?q=%s"
You can style the link as chemical is loading.
a[data-chemical-loading="true"] {
cursor: wait;
}
Opens in the current tab when the enter key is pressed.
<input data-target="_self" placeholder="Enter URL" is="chemical-input">
You can also add data-service="uv"
, data-autoHttps
, and data-search-engine="https://www.google.com/search?q=%s"
Opens in current tab when the enter key is pressed.
<input data-target="_blank" placeholder="Enter URL" is="chemical-input">
Custom action when the enter key is pressed. Change data-action
to your function name. The first parameter of the action will be the encoded URL.
<input data-action="logURL" placeholder="Enter URL" is="chemical-input">
<script>
function logURL(url) {
console.log(url)
}
</script>
Opens when the enter key is pressed or button is clicked. Set the for
attribute to the id
of the button.
<input id="my-input" data-target="_blank" placeholder="Enter URL" is="chemical-input">
<button data-for="my-input" is="chemical-button">Go!</button>
A hidden iframe that is shown when the enter key is pressed. Set the frame
attribute to the id
of the iframe.
<input data-frame="my-iframe" placeholder="Enter URL" is="chemical-input">
<iframe id="my-iframe" is="chemical-iframe"></iframe>
A hidden iframe that is shown when the enter key is pressed. Includes controls the are hidden when the iframe is hidden and can control websites in the iframe as well as hiding the iframe and controls.
Set the frame
attribute to the id
of the iframe.
Set the controls
attribute to the id
of the controls.
Set the second parameter of chemicalAction
to the id
of the iframe.
<input data-frame="my-iframe-2" placeholder="Enter URL" is="chemical-input">
<section id="my-controls-2" is="chemical-controls">
<button onclick="chemicalAction('back', 'my-iframe-2')">←</button>
<button onclick="chemicalAction('forward', 'my-iframe-2')">→</button>
<button onclick="chemicalAction('reload', 'my-iframe-2')">⟳</button>
<button onclick="chemicalAction('close', 'my-iframe-2')">🗙</button>
</section>
<iframe data-controls="my-controls-2" id="my-iframe-2" is="chemical-iframe"></iframe>
Chemical uses the AGPL 3.0 license.
FAQs
Easily create your own web proxy with no experience required.
The npm package chemicaljs receives a total of 139 weekly downloads. As such, chemicaljs popularity was classified as not popular.
We found that chemicaljs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.