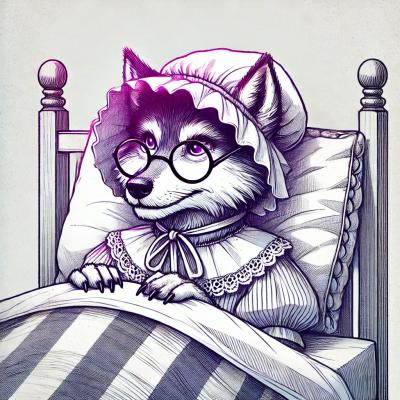
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Simple mongodb-node-expressjs API library
docker run -d --rm -p 27017:27017 --name mongo-local mongo
docker stop mongo-local
# install library
yarn add chemikata
# install other dependencies
yarn add mongoose express body-parser express-validator
(async () => {
const mongoose = require('mongoose');
const express = require('express');
const bodParser = require('body-parser');
const {body, param} = require('express-validator');
const createController = require('../controller/createController');
const genericController = require('../controller/genericController');
const UserModel = mongoose.model('User', new mongoose.Schema({
name: String,
age: Number,
email: String,
alive: Boolean,
sex: String,
createdAt: Number,
updatedAt: Number,
}));
const userFormSchema = {
create: [
body("name").isString().isLength({min: 5}),
body("age").isInt({min: 10, max: 60}),
body("email").isEmail(),
body("sex").isIn(["male", "female"]),
body("alive").optional().isBoolean()
],
update: [
body("age").isInt({min: 10, max: 60}),
body("alive").custom(value => {
if (value !== undefined) {
throw new Error("alive must not be defined")
}
return true;
}),
param("id").isMongoId()
]
}
await mongoose.connect('mongodb://localhost/test', {useNewUrlParser: true, useUnifiedTopology: true});
const app = express();
app.use(bodParser.json());
// noinspection JSCheckFunctionSignatures
app.use("/", createController(genericController(UserModel), userFormSchema));
app.listen(3000, () => {
console.log(3000, "listening");
})
})();
###
POST http://localhost:3000/
Content-Type: application/json
{
"name": "hayri",
"age": 24,
"email": "hayri@gmail.com",
"alive": true,
"sex": "male"
}
###
POST http://localhost:3000/
Content-Type: application/json
{
"name": "semsi",
"age": 34,
"email": "semsi@gmail.com",
"alive": true,
"sex": "male"
}
###
GET http://localhost:3000/?name=hayri
Accept: application/json
###
GET http://localhost:3000/?age__gt=30
Accept: application/json
###
GET http://localhost:3000/6009b2d23f29dd7558fed256
Accept: application/json
###
GET http://localhost:3000/count
Accept: application/json
###
GET http://localhost:3000/count?age__gt=30
Accept: application/json
###
PUT http://localhost:3000/6009b2d23f29dd7558fed256
Content-Type: application/json
{
"age": 44
}
###
DELETE http://localhost:3000/6009b2d23f29dd7558fed256
Content-Type: application/json
{}
override list method and allow only list handler
const controller = (model) => ({
...genericController(model),
list: () => ({message: "surprise motherfucker"})
})
app.use("/", createController(controller(UserModel), null, ["list"]));
https://express-validator.github.io/docs/
https://github.com/validatorjs/validator.js
FAQs
Simple mongodb-node-expressjs API library
The npm package chemikata receives a total of 1 weekly downloads. As such, chemikata popularity was classified as not popular.
We found that chemikata demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.