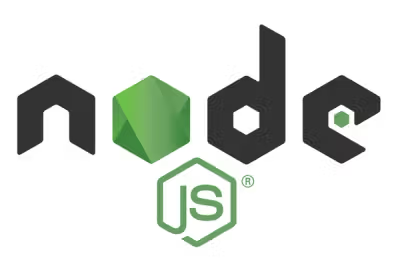
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
cherrytree-for-react
Advanced tools
Use the cherrytree router in your React applications. This project provides a React component that you should put at the root of your render tree. The component handles hot reloading.
$ npm install --save react cherrytree cherrytree-for-react
import React from 'react'
import createCherrytree from 'cherrytree'
import { Router, Link } from 'cherrytree-for-react'
import * as components from './components'
const {
Application,
About,
GithubStargazers,
GithubRepo,
GithubUser
} = components
const cherrytree = createCherrytree().map(routes)
export default class App extends React.Component {
render () {
return (
<Router router={cherrytree} />
)
}
}
function routes (route) {
route('app', { path: '/', component: Application }, () => {
route('about', { path: 'about', component: About })
route('stargazers', { path: 'stargazers', component: GithubStargazers }, () => {
route('repo', { path: ':username/:repo', component: GithubRepo })
route('user', { path: ':username', component: GithubUser })
})
})
}
The router will be injected into the context of the render tree. You can use it to generate links or initiate transitions, e.g.
let transition = this.context.router.transitionTo('repo', {username: 'facebook', repo: 'react'})
let url = this.context.router.generate('repo', {username: 'facebook', repo: 'react'})
Browse cherrytree repo for more docs and examples.
<Link>
components are used to create an <a>
element that links to a route.
Import first
import { Link } from 'cherrytree-for-react'
For example, assuming you have the following route:
route('showPost', {path: '/posts/:postID', component: Post})
You could use the following component to link to that route:
<Link to='showPost' params={{ postId: post.id }} query={{ show: true }} />
To create a link with full (external or local) url, use the href
attribute instead
<Link href={`/posts/${post.id}`} />
This component can also be used in the server side, in that case, an already started cherrytree instance needs to be passed in, e.g.
// start listening
cherrytree.listen(new cherrytree.MemoryLocation('/foo/bar')).then(function () {
React.renderToString(<Router router={cherrytree} />)
})
In this case, the <Router>
component will detect that the router has already been started and will
not call the asynchronous listen function.
For a full, working server side example, see the cherrytree/examples/server-side-example.
The typical extension point for cherrytree is the middleware mechanism. However, wrapping cherrytree in a React component is what enables the hot reloading functionality. A new cherrytree instance can be swapped in via the prop into the router during the hot reloads. The router is then kept in the component state meaning we have a reference to the old instance and can clean up using cherrytree.destroy() between the hot reloads. The middleware is still used as a way to update the state of the Router component that triggers the rerender.
There are currently two examples:
cherrytree-for-react
can be found over at cherrytree/examples/server-side-reactFAQs
An adapter for cherrytree router for using it with React
The npm package cherrytree-for-react receives a total of 2 weekly downloads. As such, cherrytree-for-react popularity was classified as not popular.
We found that cherrytree-for-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.