Comparing version 0.1.1 to 0.2.0
@@ -0,1 +1,11 @@ | ||
<a name="0.2.0"></a> | ||
# [0.2.0](https://github.com/ipfs/js-cid/compare/v0.1.1...v0.2.0) (2016-10-24) | ||
### Features | ||
* cidV0 and cidV1 support ([211970b](https://github.com/ipfs/js-cid/commit/211970b)) | ||
<a name="0.1.1"></a> | ||
@@ -2,0 +12,0 @@ ## 0.1.1 (2016-09-09) |
'use strict'; | ||
/* | ||
* Consult table at: https://github.com/multiformats/multicodec | ||
*/ | ||
module.exports = { | ||
protobuf: 0, | ||
raw: 1, | ||
json: 2, | ||
cbor: 3 | ||
raw: new Buffer('00', 'hex'), | ||
'dag-pb': new Buffer('70', 'hex'), | ||
'dag-cbor': new Buffer('71', 'hex'), | ||
'eth-block': new Buffer('90', 'hex'), | ||
'eth-tx': new Buffer('91', 'hex') | ||
}; |
133
lib/index.js
'use strict'; | ||
var CID = require('./cid'); | ||
var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }(); | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } | ||
var mh = require('multihashes'); | ||
var multibase = require('multibase'); | ||
var multicodec = require('multicodec'); | ||
var codecs = require('./codecs'); | ||
// CID: <mbase><version><mcodec><mhash> | ||
var CID = function () { | ||
/* | ||
* if (str) | ||
* if (1st char is on multibase table) -> CID String | ||
* else -> bs58 encoded multihash | ||
* else if (Buffer) | ||
* if (0 or 1) -> CID | ||
* else -> multihash | ||
* else if (Number) | ||
* -> construct CID by parts | ||
* | ||
* ..if only JS had traits.. | ||
*/ | ||
function CID(version, codec, multihash) { | ||
_classCallCheck(this, CID); | ||
if (typeof version === 'string') { | ||
if (multibase.isEncoded(version)) { | ||
// CID String (encoded with multibase) | ||
var cid = multibase.decode(version); | ||
this.version = parseInt(cid.slice(0, 1).toString('hex'), 16); | ||
this.codec = multicodec.getCodec(cid.slice(1)); | ||
this.multihash = multicodec.rmPrefix(cid.slice(1)); | ||
} else { | ||
// bs58 string encoded multihash | ||
this.codec = 'dag-pb'; | ||
this.multihash = mh.fromB58String(version); | ||
this.version = 0; | ||
} | ||
} else if (Buffer.isBuffer(version)) { | ||
var firstByte = version.slice(0, 1); | ||
var v = parseInt(firstByte.toString('hex'), 16); | ||
if (v === 0 || v === 1) { | ||
// CID | ||
var _cid = version; | ||
this.version = v; | ||
this.codec = multicodec.getCodec(_cid.slice(1)); | ||
this.multihash = multicodec.rmPrefix(_cid.slice(1)); | ||
} else { | ||
// multihash | ||
this.codec = 'dag-pb'; | ||
this.multihash = version; | ||
this.version = 0; | ||
} | ||
} else if (typeof version === 'number') { | ||
if (typeof codec !== 'string') { | ||
throw new Error('codec must be string'); | ||
} | ||
if (!(version === 0 || version === 1)) { | ||
throw new Error('version must be a number equal to 0 or 1'); | ||
} | ||
mh.validate(multihash); | ||
this.codec = codec; | ||
this.version = version; | ||
this.multihash = multihash; | ||
} | ||
} | ||
_createClass(CID, [{ | ||
key: 'toV0', | ||
value: function toV0() { | ||
return this.multihash; | ||
} | ||
}, { | ||
key: 'toV1', | ||
value: function toV1() { | ||
return this.buffer; | ||
} | ||
/* defaults to base58btc */ | ||
}, { | ||
key: 'toBaseEncodedString', | ||
value: function toBaseEncodedString(base) { | ||
base = base || 'base58btc'; | ||
switch (this.version) { | ||
case 0: | ||
return mh.toB58String(this.multihash); | ||
case 1: | ||
return multibase.encode(base, this.buffer).toString(); | ||
default: | ||
throw new Error('Unsupported version'); | ||
} | ||
} | ||
}, { | ||
key: 'toJSON', | ||
value: function toJSON() { | ||
return { | ||
codec: this.codec, | ||
version: this.version, | ||
hash: this.multihash | ||
}; | ||
} | ||
}, { | ||
key: 'equals', | ||
value: function equals(other) { | ||
return this.codec === other.codec && this.version === other.version && this.multihash.equals(other.multihash); | ||
} | ||
}, { | ||
key: 'buffer', | ||
get: function get() { | ||
switch (this.version) { | ||
case 0: | ||
return this.multihash; | ||
case 1: | ||
return Buffer.concat([Buffer('01', 'hex'), Buffer(codecs[this.codec]), this.multihash]); | ||
default: | ||
throw new Error('unsupported version'); | ||
} | ||
} | ||
}]); | ||
return CID; | ||
}(); | ||
CID.codecs = codecs; | ||
CID.isCID = function (other) { | ||
return other.constructor.name === 'CID'; | ||
}; | ||
module.exports = CID; |
{ | ||
"name": "cids", | ||
"version": "0.1.1", | ||
"version": "0.2.0", | ||
"description": "cid implementation", | ||
@@ -39,2 +39,3 @@ "main": "lib/index.js", | ||
"multibase": "^0.2.0", | ||
"multicodec": "0.1.0", | ||
"multihashes": "^0.2.2" | ||
@@ -52,4 +53,6 @@ }, | ||
"contributors": [ | ||
"Friedel Ziegelmayer <dignifiedquire@gmail.com>" | ||
"David Dias <daviddias.p@gmail.com>", | ||
"Friedel Ziegelmayer <dignifiedquire@gmail.com>", | ||
"Richard Littauer <richard.littauer@gmail.com>" | ||
] | ||
} |
@@ -13,4 +13,25 @@ # js-cid | ||
## Installation | ||
## Table of Contents | ||
- [Install](#install) | ||
- [In Node.js through npm](#in-nodejs-through-npm) | ||
- [Browser: Browserify, Webpack, other bundlers](#browser-browserify-webpack-other-bundlers) | ||
- [In the Browser through `<script>` tag](#in-the-browser-through-script-tag) | ||
- [Gotchas](#gotchas) | ||
- [Usage](#usage) | ||
- [API](#api) | ||
- [`new CID(codec[, version, hash])`](#new-cidcodec-version-hash) | ||
- [`.codec`](#codec) | ||
- [`.version`](#version) | ||
- [`.hash`](#hash) | ||
- [`.buffer`](#buffer) | ||
- [`.toString()`](#tostring) | ||
- [`.toJSON()`](#tojson) | ||
- [`CID.isCID(other)`](#cidiscidother) | ||
- [`CID.codecs`](#cidcodecs) | ||
- [Contribute](#contribute) | ||
- [License](#license) | ||
## Install | ||
### In Node.js through npm | ||
@@ -58,4 +79,10 @@ | ||
### `new CID(codec[, version, hash])` | ||
### Constructor | ||
- `new CID(<version>, <codec>, <multihash>)` | ||
- `new CID(<cidStr>)` | ||
- `new CID(<cid.buffer>)` | ||
- `new CID(<multihash>)` | ||
- `new CID(<bs58 encoded multihash>)` | ||
### `.codec` | ||
@@ -69,4 +96,10 @@ | ||
### `.toString()` | ||
### `.toV0()` | ||
### `.toV1()` | ||
### `.toBaseEncodedString(base)` | ||
Defaults to 'base58btc' | ||
### `.toJSON()` | ||
@@ -78,4 +111,14 @@ | ||
## Contribute | ||
[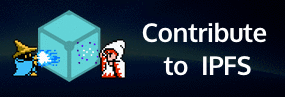](https://github.com/ipfs/community/blob/master/contributing.md) | ||
Contributions welcome. Please check out [the issues](https://github.com/ipfs/js-cid/issues). | ||
Check out our [contributing document](https://github.com/ipfs/community/blob/master/contributing.md) for more information on how we work, and about contributing in general. Please be aware that all interactions related to multiformats are subject to the IPFS [Code of Conduct](https://github.com/ipfs/community/blob/master/code-of-conduct.md). | ||
Small note: If editing the Readme, please conform to the [standard-readme](https://github.com/RichardLitt/standard-readme) specification. | ||
## License | ||
MIT |
'use strict' | ||
/* | ||
* Consult table at: https://github.com/multiformats/multicodec | ||
*/ | ||
module.exports = { | ||
protobuf: 0, | ||
raw: 1, | ||
json: 2, | ||
cbor: 3 | ||
raw: new Buffer('00', 'hex'), | ||
'dag-pb': new Buffer('70', 'hex'), | ||
'dag-cbor': new Buffer('71', 'hex'), | ||
'eth-block': new Buffer('90', 'hex'), | ||
'eth-tx': new Buffer('91', 'hex') | ||
} |
117
src/index.js
'use strict' | ||
const CID = require('./cid') | ||
const mh = require('multihashes') | ||
const multibase = require('multibase') | ||
const multicodec = require('multicodec') | ||
const codecs = require('./codecs') | ||
// CID: <mbase><version><mcodec><mhash> | ||
class CID { | ||
/* | ||
* if (str) | ||
* if (1st char is on multibase table) -> CID String | ||
* else -> bs58 encoded multihash | ||
* else if (Buffer) | ||
* if (0 or 1) -> CID | ||
* else -> multihash | ||
* else if (Number) | ||
* -> construct CID by parts | ||
* | ||
* ..if only JS had traits.. | ||
*/ | ||
constructor (version, codec, multihash) { | ||
if (typeof version === 'string') { | ||
if (multibase.isEncoded(version)) { // CID String (encoded with multibase) | ||
const cid = multibase.decode(version) | ||
this.version = parseInt(cid.slice(0, 1).toString('hex'), 16) | ||
this.codec = multicodec.getCodec(cid.slice(1)) | ||
this.multihash = multicodec.rmPrefix(cid.slice(1)) | ||
} else { // bs58 string encoded multihash | ||
this.codec = 'dag-pb' | ||
this.multihash = mh.fromB58String(version) | ||
this.version = 0 | ||
} | ||
} else if (Buffer.isBuffer(version)) { | ||
const firstByte = version.slice(0, 1) | ||
const v = parseInt(firstByte.toString('hex'), 16) | ||
if (v === 0 || v === 1) { // CID | ||
const cid = version | ||
this.version = v | ||
this.codec = multicodec.getCodec(cid.slice(1)) | ||
this.multihash = multicodec.rmPrefix(cid.slice(1)) | ||
} else { // multihash | ||
this.codec = 'dag-pb' | ||
this.multihash = version | ||
this.version = 0 | ||
} | ||
} else if (typeof version === 'number') { | ||
if (typeof codec !== 'string') { | ||
throw new Error('codec must be string') | ||
} | ||
if (!(version === 0 || version === 1)) { | ||
throw new Error('version must be a number equal to 0 or 1') | ||
} | ||
mh.validate(multihash) | ||
this.codec = codec | ||
this.version = version | ||
this.multihash = multihash | ||
} | ||
} | ||
get buffer () { | ||
switch (this.version) { | ||
case 0: | ||
return this.multihash | ||
case 1: | ||
return Buffer.concat([ | ||
Buffer('01', 'hex'), | ||
Buffer(codecs[this.codec]), | ||
this.multihash | ||
]) | ||
default: | ||
throw new Error('unsupported version') | ||
} | ||
} | ||
toV0 () { | ||
return this.multihash | ||
} | ||
toV1 () { | ||
return this.buffer | ||
} | ||
/* defaults to base58btc */ | ||
toBaseEncodedString (base) { | ||
base = base || 'base58btc' | ||
switch (this.version) { | ||
case 0: | ||
return mh.toB58String(this.multihash) | ||
case 1: | ||
return multibase.encode(base, this.buffer).toString() | ||
default: | ||
throw new Error('Unsupported version') | ||
} | ||
} | ||
toJSON () { | ||
return { | ||
codec: this.codec, | ||
version: this.version, | ||
hash: this.multihash | ||
} | ||
} | ||
equals (other) { | ||
return this.codec === other.codec && | ||
this.version === other.version && | ||
this.multihash.equals(other.multihash) | ||
} | ||
} | ||
CID.codecs = codecs | ||
CID.isCID = (other) => { | ||
return other.constructor.name === 'CID' | ||
} | ||
module.exports = CID |
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is too big to display
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
978406
10652
121
3
14
1
+ Addedmulticodec@0.1.0
+ Addedmulticodec@0.1.0(transitive)
+ Addedvarint@4.0.1(transitive)