What is cids?
The 'cids' npm package is used for handling Content Identifiers (CIDs) in the context of IPFS (InterPlanetary File System). CIDs are a fundamental part of IPFS, allowing for unique identification of content based on its cryptographic hash. This package provides utilities for creating, parsing, and working with CIDs.
What are cids's main functionalities?
Creating a CID
This feature allows you to create a new CID instance. The constructor takes the version, codec, and multihash as parameters. The example demonstrates creating a CID of version 1 with the 'dag-pb' codec.
const CID = require('cids');
const cid = new CID(1, 'dag-pb', Buffer.from('1220...'));
console.log(cid.toString());
Parsing a CID
This feature allows you to parse an existing CID string. The example shows how to parse a CID and access its version property.
const CID = require('cids');
const cid = new CID('Qm...');
console.log(cid.version);
Converting CID to different formats
This feature allows you to convert a CID to different versions. The example demonstrates converting a CID to version 1 and then converting it to a string.
const CID = require('cids');
const cid = new CID('Qm...');
console.log(cid.toV1().toString());
Other packages similar to cids
multihashes
The 'multihashes' package provides utilities for working with multihashes, which are a key component of CIDs. While 'cids' focuses on the entire CID structure, 'multihashes' is more specialized in handling the multihash part of CIDs.
multibase
The 'multibase' package is used for encoding and decoding data in various base encodings. CIDs often use multibase for encoding their string representations. This package is more focused on the encoding aspect, whereas 'cids' handles the entire CID structure.
ipld
The 'ipld' package provides tools for working with InterPlanetary Linked Data (IPLD), which is the data model used by IPFS. While 'cids' is specifically for handling CIDs, 'ipld' provides a broader set of tools for working with the data structures that CIDs point to.
js-cid

CID implementation in JavaScript.
Table of Contents
Install
In Node.js through npm
$ npm install --save cids
Browser: Browserify, Webpack, other bundlers
The code published to npm that gets loaded on require is in fact an ES5 transpiled version with the right shims added. This means that you can require it and use with your favourite bundler without having to adjust asset management process.
const CID = require('cids')
In the Browser through <script>
tag
Loading this module through a script tag will make the multihash
obj available in the global namespace.
<script src="https://unpkg.com/cids/dist/index.min.js"></script>
<!-- OR -->
<script src="https://unpkg.com/cids/dist/index.js"></script>
Gotchas
You will need to use Node.js Buffer
API compatible, if you are running inside the browser, you can access it by multihash.Buffer
or you can install Feross's Buffer.
Usage
const CID = require('cids')
const cid = new CID(CID.codecs.raw, 1, myhash)
const cid = new CID(base58Multihash)
API
Constructor
new CID(<version>, <codec>, <multihash>)
new CID(<cidStr>)
new CID(<cid.buffer>)
new CID(<multihash>)
new CID(<bs58 encoded multihash>)
.codec
.version
.hash
.buffer
.toV0()
.toV1()
.toBaseEncodedString(base)
Defaults to 'base58btc'
.toJSON()
CID.isCID(other)
CID.codecs
Contribute
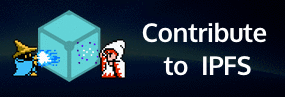
Contributions welcome. Please check out the issues.
Check out our contributing document for more information on how we work, and about contributing in general. Please be aware that all interactions related to multiformats are subject to the IPFS Code of Conduct.
Small note: If editing the Readme, please conform to the standard-readme specification.
License
MIT