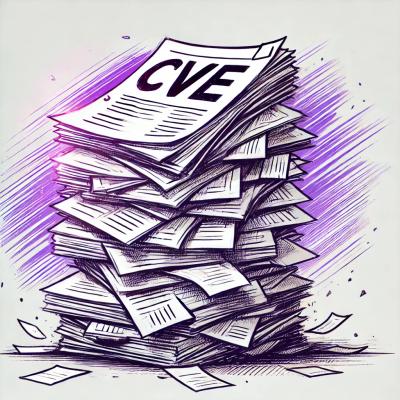
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Conveniently create net/tls server/client sockets with helpful listeners providing common functionality.
This will do all the work for you to create client or server sockets setup for:
tls
module and private/public/root certificateslisten()
when the EADDRINUSE
error occursAdditional features available in @cio
scope:
See chain-builder for more on how each worker chain operates.
See ordering for more on how the workers are ordered in the chain.
npm install cio --save
TODO: Create Table of Contents
The module accepts options to future proof it. This means the require call returns a builder function which makes the cio
instance you'll use.
// one thing at a time:
var buildCio = require('cio') // #1
var cio = buildCio(moduleOptions) // #2
var server = cio.server(options) // #3
// combine #1 and #2
var cio = require('cio')()
// and again with module options
var cio = require('cio')(cioModuleOptions)
var clientOptions = { /* use nothing */ }
, client = cio.client(clientOptions);
// the result is a client socket created by `net.connect()`
var clientOptions = {
port : 12345
, host: 'localhost'
}
, client = cio.client(clientOptions);
// the result is a client socket created by:
// `net.connect({port:12345, host:'localhost'})`
var serverOptions = { /* nothing */ }
, server = cio.server(serverOptions);
// the result is a server socket created by:
// `net.createServer()`
All the above can be changed to perform secured communication by providing the necessary certificates as described in the Node TLS documentation (and createServer()).
Then cio
will use the tls
module, instead of net
, to create the sockets. The options object is passed on to the tls
functions as-is so all options supported by those modules may be specified.
Node uses the names: 'key', 'cert', and 'ca'. I prefer the names: 'private', 'public', and 'root'. So, either may be used.
You may specify the file path to the certificate and it will be read into a buffer for you. Or, you may provide the buffer yourself. These options are provided directly to the Node modules so options described by them are allowed. This is a convenience so you don't have to write the file reading part.
// this uses my preferred aliases. You can use the Node names: key, cert, ca.
var clientOrServerOptions = {
// example of specifying a path
private: 'path/to/private/key/file'
// example of getting the buffer yourself
, public: getPublicCertAsBuffer()
// example of having the buffer already and placing into an array
, root: [rootCertBuffer]
// optionally, add this for either:
, rejectUnauthorized: true
// or these for server:
, requestCert: true
, isClientAllowed: function allowSomeClients(clientName) {
return clientName.indexOf('noblah') > -1;
}
, rejectClient: function rejectWithMessage(connection, clientName) {
connection.end('Sorry, you are not allowed, '+clientName);
}
};
// these will now use `tls`
var client = cio.client(clientOrServerOptions);
var server = cio.server(clientOrServerOptions);
Both client and server also allow rejectUnauthorized
which makes it require certificates and secured communication. See the Node TLS documentation.
The server also supports the requestCert
for client whitelist/blacklist support. See the authenticate-client listener.
Provide additional functionality by adding listeners for new sockets. There are three types of listeners:
connect()
(net or tls)createServer()
(net or tls)connection
or secureConnection
events.Add listeners with corresponding functions:
cio.onClient(listener)
cio.onServer(listener)
cio.onServerClient(listener)
These functions also accept a string. They will attempt to require()
it to get the listener function. You may load addons like:
var cio = buildCio();
// add the module @cio/transformer:
cio.onClient('@cio/transformer');
// OR:
var transformer = require('@cio/transformer');
cio.onClient(transformer);
// or add your own listeners:
cio.onClient(function(control, context) {
// do something with:
// this.client
// options are in the `context` object *or* `this`
});
cio.onServer(function(control, context) {
// do something with:
// this.server
// options are in the `context` object *or* `this`
});
cio.onServerClient(function(control, context) {
// do something with: (the server client connection)
// this.connection
// options are in the `context` object *or* `this`
});
See chain-builder for more on what the control
, context
, and this
are in your listeners.
By default new listeners are added at the end of the work chain. You may alter how the functions are ordered by setting order constraints onto the functions. See ordering for full documentation.
TODO: List the core listener ID's by work chain and show an example of using function options to alter ordering.
// example coming...
There is little difference between client()
and server()
.
The server has:
requestCert
to deny clients.isClientAllowed
and rejectClient
for whitelist/blacklist of clientsnoRelisten
to disable the default behavior of retrying the listen()
when the error is EADDRINUSE
retryDelay
and maxRetries
to control the retry behaviorThe client has:
reconnect
for automatically reconnecting (not yet implemented)The rest are shared by both.
All defaults are false or undefined.
Name | type | Client/Server | Description |
---|---|---|---|
noRelisten | bool | server | server socket gets an error listener for EADDRINUSE which will retry three times to listen() before exiting. Set this to true to turn that off |
retryDelay | int | server | Defaults to 3 second delay before retrying listen() |
maxRetries | int | server | Defaults to 3 tries before quitting |
requestCert | bool | server | will trigger using tls instead of net . Used for server only. Adds a listener which will get client name and check if they're allowed. Must specify isClientAllowed function. May specify rejectClient function. Default emits an error event with a message including the name of client rejected. |
rejectUnauthorized | bool | both | requires proper certificates |
key or private | file path or buffer | both | private key for TLS |
cert or public | file path or buffer | both | public key for TLS |
ca or root | file path or buffer | both | ca/root key |
isClientAllowed | Function | server | Receives the client name for the certificate. Returning false will cause the client connection to be rejected (closed). |
rejectClient | Function | server | When isClientAllowed returns false this function is called with client name and socket. When not specified and isClientAllowed returns false then an 'error' event is emitted ('Client Rejected: ' + clientName ). |
Not yet implemented (X), or, being moved to the @cio
scope (!):
? | Name | type | Client/Server | Description |
---|---|---|---|---|
X | reconnect | bool | client | use reconnect-net to handle reconnecting. not yet implemented |
! | multiplex | bool | both | use mux-demux for multiplexing connection |
! | eventor | bool | both | use duplex-emitter . if multiplex is true, create 'events' stream |
! | jsonify | bool | both | run connection thru json-duplex-stream for in+out |
! | transform | Transform | both | pipe connection thru Transform and back to connection. if jsonify is true then the Transform is in the middle: conn -> json.in -> transform -> json.out -> conn |
Not yet, these are being moved to new modules in @cio
scope. They will emit these events once they are available.
multiplex
is on, 'mux' is emitted on a new socket after the 'on connect' listeners have run. Use this to configure the mux instance. For example, adding more streams to it and associated handlers.eventor
is on, 'eventor' is emitted on a new socket after the 'on connect' listeners have run. Use this to setup event handlers on the socket specific eventor
.json-duplex-stream
has been setup with the socket piping to its input and its output piping to the socket. Use this to specify what should happen in the middle, after the jsonified input and how it gets back to the jsonify output.FAQs
Conveniently create net/tls server/client sockets.
The npm package cio receives a total of 0 weekly downloads. As such, cio popularity was classified as not popular.
We found that cio demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.