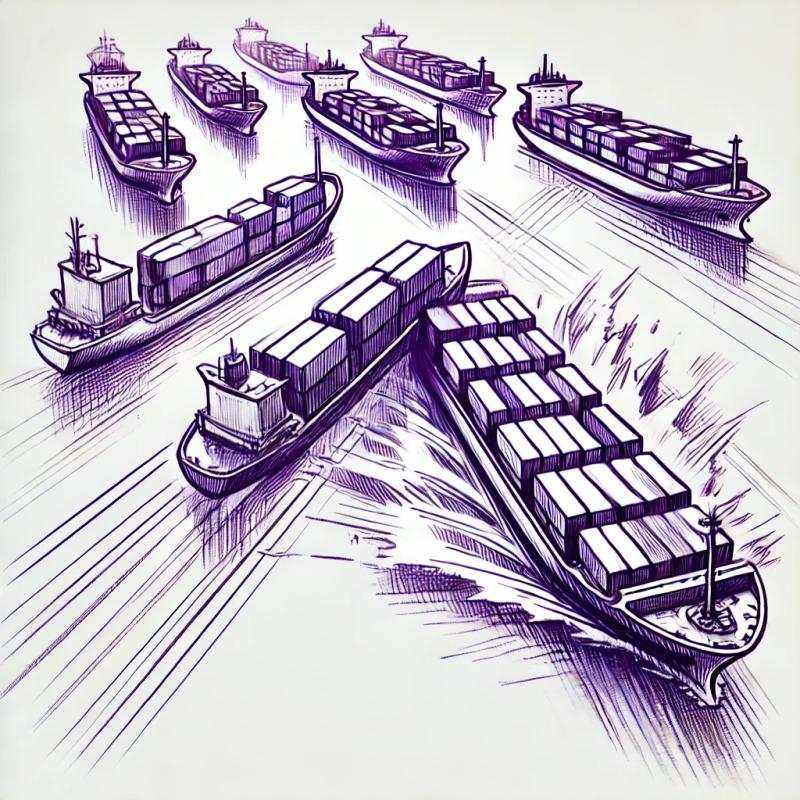
Security News
Namecheap Takes Down Polyfill.io Service Following Supply Chain Attack
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
circletype
Advanced tools
Readme
A JavaScript library that lets you curve type on the web.
Demo: http://circletype.labwire.ca
In a browser:
<script src="circletype.min.js"></script>
Using npm:
$ npm i circletype --save
Load ES6 module:
import CircleType from `circletype`;
A CircleType instance creates a circular text element.
Kind: global class
CircleType
number
CircleType
number
CircleType
CircleType
Param | Type | Description |
---|---|---|
elem | HTMLElement | A target HTML element. |
Example
// Instantiate `CircleType` with an HTML element.
const circleType = new CircleType(document.getElementById('myElement'));
// Set the text radius and direction. Note: setter methods are chainable.
circleType.radius(200).dir(-1);
CircleType
Sets the desired text radius. The minimum radius is the radius required
for the text to form a complete circle. If value
is less than the minimum
radius, the minimum radius is used.
Kind: instance method of CircleType
Returns: CircleType
- The current instance.
Param | Type | Description |
---|---|---|
value | number | A new text radius in pixels. |
Example
const circleType = new CircleType(document.getElementById('myElement'));
// Set the radius to 150 pixels.
circleType.radius(150);
number
Gets the text radius in pixels. The default radius is the radius required for the text to form a complete circle.
Kind: instance method of CircleType
Returns: number
- The current text radius.
Example
const circleType = new CircleType(document.getElementById('myElement'));
circleType.radius();
// > 150
CircleType
Sets the text direction. 1
is clockwise, -1
is counter-clockwise.
Kind: instance method of CircleType
Returns: CircleType
- The current instance.
Param | Type | Description |
---|---|---|
value | number | A new text direction. |
Example
const circleType = new CircleType(document.getElementById('myElement'));
// Set the direction to counter-clockwise.
circleType.dir(-1);
// Set the direction to clockwise.
circleType.dir(1);
number
Gets the text direction. 1
is clockwise, -1
is counter-clockwise.
Kind: instance method of CircleType
Returns: number
- The current text radius.
Example
const circleType = new CircleType(document.getElementById('myElement'));
circleType.dir();
// > 1 (clockwise)
CircleType
Schedules a task to recalculate the height of the element. This should be called if the font size is ever changed.
Kind: instance method of CircleType
Returns: CircleType
- The current instance.
Example
const circleType = new CircleType(document.getElementById('myElement'));
circleType.refresh();
CircleType
Removes the CircleType effect from the element, restoring it to its original state.
Kind: instance method of CircleType
Returns: CircleType
- This instance.
Example
const circleType = new CircleType(document.getElementById('myElement'));
// Restore `myElement` to its original state.
circleType.destroy();
FAQs
A JavaScript library that lets you curve type on the web.
The npm package circletype receives a total of 1,129 weekly downloads. As such, circletype popularity was classified as popular.
We found that circletype demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.
Security News
A JavaScript library maintainer is under fire after merging a controversial PR to support legacy versions of Node.js.