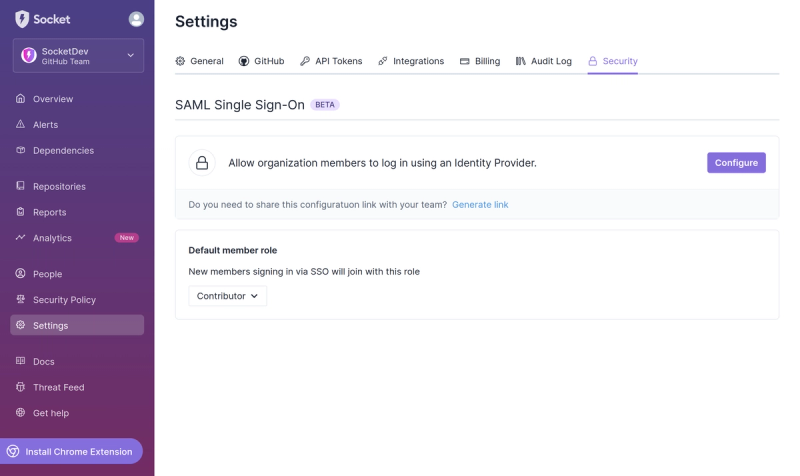
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
cjsbaseclass
Advanced tools
Readme
DRY Javascript Class with inheritance and embedded jQuery 2.2.4.
> bower install cjsbaseclass --save
> node bower_components/cjsbaseclass/dist/install-sublime-snippets.node.js
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<script type="text/javascript" src="cjsbaseclass.min.js" data-jquery-exclusive="false" data-silent-host="www.site-production.com"></script>
<script>
console.log($.fn.jquery);
</script>
</head>
<body>
</body>
</html>
data-jquery-exclusive
: If false, expose internal jQuery to global environment;data-silent-host
: All debug off on production environment. self.cookie.set('name', 'value', { expires: 7 });
self.cookie.get('name'); // => 'value'
self.cookie.get('nothing'); // => undefined
self.cookie.remove('name');
self.cookie.set('name', { foo: 'bar' });
self.cookie.getJSON('name'); // => { foo: 'bar' }
// Waits another class (CjsBaseClass based) on ready and call a callback
self.waitReady
(
'Tother',
function()
{
self.otherClassReady();
}
);
// Waits another class (CjsBaseClass based) on start and call a callback
self.waitStarted
(
'Tother',
function()
{
self.otherClassStarted();
}
);
// Fire a jQuery trigger
self.trigger('fire-trigger', { 'name': 'The Name', 'id': 123456 });
// Listen a jQuery trigger
self.onTrigger
(
'fire-trigger',
function(p_args)
{
console.log(p_args);
}
);
self.onTrigger
(
'fire-trigger-1',
function(p_args)
{
console.log(p_args);
},
'fire-trigger-2',
function(p_args)
{
console.log(p_args);
},
'fire-trigger-3',
function(p_args)
{
console.log(p_args);
}
);
// Waits a trigger fire with trigger_name param, if this already fired, callback is called immediately
self.waitTrigger
(
'fire-trigger',
function(p_args)
{
console.log(p_args);
}
);
// Returns browser name
self.log.info(self.browser); // Returns 'chrome' or 'firefox' or 'safari' or 'ie' or 'other'
// Returns all options on create class
self.options;
Object {debug: 1, highlighted: "purple", custom_option: "custom_value"}
// Returns in case browser (ex: chrome)
self.is.chrome; // returns true
self.is.firefox; // returns false
self.is.safari; // returns false
self.is.ie; // returns false
self.is.ie9; // returns false
// Returns is browser defined
// options: self.is.chrome, self.is.firefox, self.is.safari, self.is.ie, self.is.ie9
var myvar = (self.is.ie9) ? 'IS IE9 :(' : 'IS NOT IE9 :D';
// Log to console - only if a debug is true
self.log.print('my chinese variable :P');
// Log to console with alert icon
self.log.alert('Class TOther is ready!');
// Log to console with info icon
self.log.info(self.browser);
// Log to console with error icon
self.log.danger(self.browser);
// Add dynamic locker variable
self.lock('scroll');
self.lock('scroll,slide');
// Return if dynamic variable is locked
self.isLocked('scroll'); // true
self.islocked('scroll,slide'); // true
self.isLocked('another'); // false
self.islocked('scroll,another'); // false
// Remove dynamic locker variable
self.unlock('scroll');
self.unlock('scroll,slide');
// Enable/disble debug mode
self.setDebug(true); // Debug mode on: print logs on console
self.setDebug(false); // Debug mode off: self.log.print does not print console logs
// Set debug mode
self.setDebugMode(0); // debug is false, none on console log is printed
self.setDebugMode(1); // debug is true, console log is printed
self.setDebugMode(2); // debug is true, console log is printed (developer's log include)
// Debug modes
var CJS_DEBUG_MODE_0 = 0;
var CJS_DEBUG_MODE_1 = 1;
var CJS_DEBUG_MODE_2 = 2;
// Set/unset global debug mode
cjsbaseclass_ns.dev.setGlobalDebug(true);
cjsbaseclass_ns.dev.setGlobalDebug(false);
self.utils.loadScript
(
'https://cdnjs.cloudflare.com/ajax/libs/taffydb/2.7.2/taffy-min.js',
function()
{
var cities = TAFFY([{name:'New York',state:'WA'},{name:'Boston',state:'MA'}]);
cities.insert({name:'Portland',state:'OR'});
console.log(cities().order('name').first().name);
}
);
// TaffyDB: http://taffydb.com
MyNameSpace.myclass.utils.aloneOn(); // Only class intro has debug messages;
MyNameSpace.myclass.utils.aloneOff(); // All classes has debug messages;
self.utils.waitScrollTop
(
function()
{
console.log('Site is on top.');
}
);
// On first time fires a trigger: "site-scroll-on-top"
self.ui.animateScroll(250);
self.ui.animateScroll(250, 1000, 'easeInOutCubic', function(){ console.log('Callback'); });
self.ui.animateScroll($('#last_element'), 1000, 'easeInOutCubic', function(){ console.log('Callback'); });
self.ui.animateScroll
(
document.getElementById('last_element'),
1000,
'easeInOutCubic',
function()
{
console.log('Callback');
}
);
<form name="frmTest" id="frmTest" action="#">
<input type="text" name="frName" id="frName" value="Person Name" placeholder="">
<br/>
<input type="checkbox" name="frCheck" value="check-1">check-1
<input type="checkbox" name="frCheck" value="check-2" checked>check-2
<input type="checkbox" name="frCheck" value="check-3" checked>check-3
<input type="checkbox" name="frCheck" value="check-4">check-4
<br/>
<input type="radio" name="frRadio" value="radio-1">radio-1
<input type="radio" name="frRadio" value="radio-2" checked>radio-2
<input type="radio" name="frRadio" value="radio-3">radio-3
<input type="radio" name="frRadio" value="radio-4">radio-4
<br/>
<label for="frSelect">Select</label>
<br/>
<select name="frSelect" id="frSelect">
<option value="1">Option 1</option>}
<option value="2" selected>Option 2</option>}
<option value="3">Option 3</option>}
</select>
<br/>
<label for="frText">Text Area</label>
<br/>
<textarea name="frText" id="frText" cols="80" rows="8">
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam,
quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo
consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse
cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non
proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
</textarea>
</form>
self.form.toJson('#frmTest');
// returns:
{
"frName": "Person Name",
"frCheck": ["check-2", "check-3"],
"frRadio": "radio-2",
"frSelect": "2",
"frText": "Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod\r\ntempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam,\r\nquis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo\r\nconsequat. Duis aute irure dolor in reprehenderit in voluptate velit esse\r\ncillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non\r\nproident, sunt in culpa qui officia deserunt mollit anim id est laborum."
}
// COMPLETE
self.ajax
(
{
'options':
{
slug : 'slug-name',
exclusive: false,
url : 'test.json',
type : 'POST',
dataType : 'json'
},
'before': function()
{
self.log.info('Test ajax before');
},
'done': function(p_response)
{
self.log.info('Test on-ajax done');
},
'fail': function(p_response)
{
self.log.info('Test on-ajax fail', p_response);
},
'always': function()
{
self.log.info('Test on-ajax always');
},
'exception': function()
{
self.log.info('Test on-ajax exception');
}
}
);
// BASIC USE
// BASIC RESULT JSON STRUCTURE
{
"result": true,
"message": "Your request processed successfully.",
"id": 125316
}
self.ajax
(
{
'options':
{
slug : 'slug-name',
exclusive: false,
url : 'result_true.json',
type : 'GET',
dataType : 'json',
},
'success': function(p_result, p_message)
{
self.log.print('Success!!! :D', p_message);
},
'error': function(p_message, p_data)
{
self.log.danger(p_message || 'Ocorreu um erro na solicitação.');
}
}
);
// ONLY REQUEST ONDONE
self.ajax
(
{
'options':
{
slug : 'another-name',
url : 'test.json',
type : 'POST',
dataType : 'json'
},
'done': function(p_response)
{
self.log.info('Test on-ajax done');
}
}
);
// ONLY REQUEST
self.ajax
(
{
'options':
{
slug : 'only-json',
exclusive: true,
url : 'test.json',
type : 'POST',
dataType : 'json'
}
}
);
// WITH AUTO OVERLAY
self.ajax
(
{
'options':
{
slug : 'overlay',
autowait : 'auto',
url : 'test.json',
type : 'POST',
dataType : 'json'
}
}
);
// TRIGGERS
self.onAjaxTriggers
(
'slug-name',
{
'before': function()
{
},
'done': function(p_response)
{
},
'fail': function()
{
},
'always': function()
{
},
'exception': function()
{
}
}
);
/* global CjsBaseClass,CJS_DEBUG_MODE_0,CJS_DEBUG_MODE_1,CJS_DEBUG_MODE_2 */
var MyNameSpace = MyNameSpace || {};
MyNameSpace.Tmyclass = function($, objname, options)
{
'use strict';
var self = this;
this.create = function()
{
self.var_name = 'var_value';
self.events.onCreate();
};
this.onReady = function()
{
self.events.onReady();
};
this.start = function()
{
self.events.onStarted();
};
this.processTriggers = function()
{
};
this.onElementsEvents = function()
{
};
this.execute = function()
{
// AUTO STARTED CODE ON CLASS READY AND STARTED
};
CjsBaseClass.call(this, $, objname, options);
};
MyNameSpace.myclass = new MyNameSpace.Tmyclass
(
window.cjsbaseclass_jquery,
'myclass',
{
'debug' : CJS_DEBUG_MODE_1,
'highlighted' : 'auto',
'another_opt' : 'custom_value'
}
);
FAQs
Base Development Javascript Class
The npm package cjsbaseclass receives a total of 52 weekly downloads. As such, cjsbaseclass popularity was classified as not popular.
We found that cjsbaseclass demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.