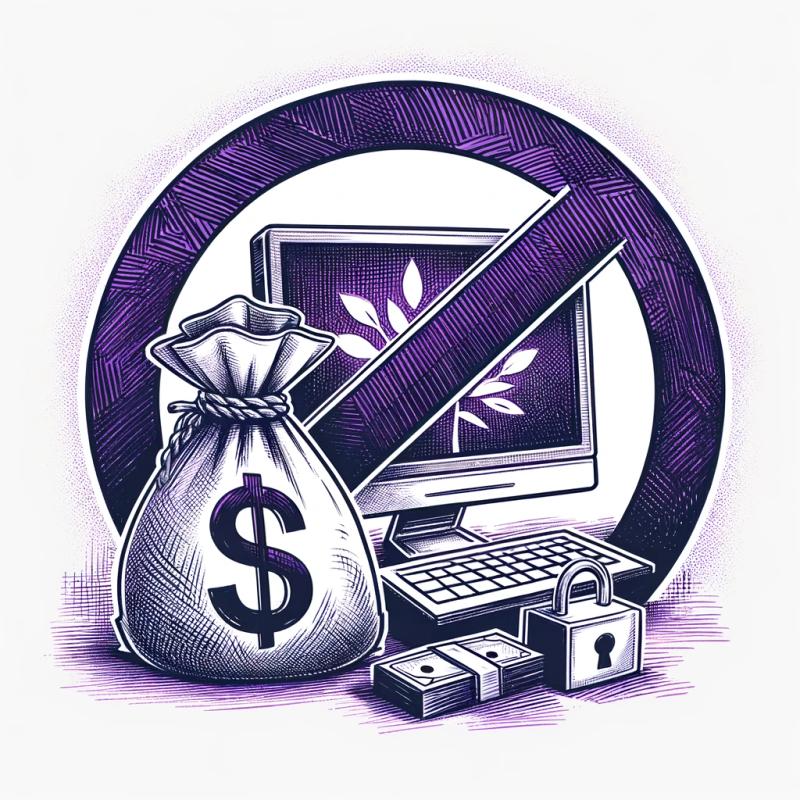
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
clamscan
Advanced tools
Changelog
0.4.0 (2014-11-19)
clamav
. Thank you @jshamley!scan_dir
method from working properlyReadme
Use Node JS to scan files on your server with ClamAV's clamscan binary. This is especially useful for scanning uploaded files provided by un-trusted sources.
This module has the ability to scan many files at once if you set the max_forks greater than 1. By default it is set to scan 2 at a time. Using this technique may not prove to be more efficient depending on your setup. Scans are called via child_process.exec
and, so, each execution (scan) is a new child process. The more CPU cores you have, the higher you can make this number. If you have 8 cores, I wouldn't go higher than 7. If you have 4, set this number to 3. If you have a 2-core machine, you can safely set this to 2, per my testing.
You will need to install ClamAV's clamscan binary on your server. On linux, it's quite simple.
Fedora-based distros:
sudo yum install clamav
Debian-based distros:
sudo apt-get install clamav
As for OSX, I've not tried it, but, here's a promising looking site: http://www.clamxav.com/index.php . I would stick with linux varieties, though...
This module is not intended to work on a Windows server. This would be a welcome addition if someone wants to add that feature (I may get around to it one day but have no urgent need for this).
npm install clamscan
Licensed under the MIT License:
All of the values listed in the example below represent the default values for their respective configuration item.
You can simply do this:
var clam = require('clamscan')();
And, you'll be good to go.
BUT: If you want more control, you can specify all sorts of options.
var clam = require('clamscan')({
max_forks: 2, // Num of files to scan at once (should be no more than # of CPU cores)
clam_path: '/usr/bin/clamscan', // Path to clamscan binary on your server
remove_infected: false, // If true, removes infected files
quarantine_infected: false, // False: Don't quarantine, Path: Moves files to this place.
scan_archives: true, // If true, scan archives (ex. zip, rar, tar, dmg, iso, etc...)
scan_recursively: true, // If true, deep scan folders recursively
scan_log: null, // Path to a writeable log file to write scan results into
db: null, // Path to a custom virus definition database
debug_mode: false // Whether or not to log info/debug/error msgs to the console
});
Here is a non-default values example (to help you get an idea of what the proper-looking values should be):
var clam = require('clamscan')({
max_forks: 1, // Do this if you only have one CPU core (12 for a monster machine)
clam_path: '/usr/bin/clam', // I dunno, maybe your clamscan is just call "clam"
remove_infected: true, // Removes files if they are infected
quarantine_path: '~/infected/', // Move file here. remove_infected must be FALSE, though.
scan_archives: false, // Choosing false here will save some CPU cycles
scan_recursively: true, // Choosing false here will save some CPU cycles
scan_log: '/var/log/node-clam', // You're a detail-oriented security professional.
db: '/usr/bin/better_clam_db', // Path to a custom virus definition database
debug_mode: true // This will put some debug info in your js console
});
This method allows you to scan a single file.
file_path
(string) Represents a path to the file to be scanned.callback
(function) Will be called when the scan is complete. It takes 3 parameters:err
(string or null) A standard error message string (null if no error)file
(string) The original file_path
passed into the is_infected
method.is_infected
(boolean) True: File is infected; False: File is clean.clam.is_infected('/a/picture/for_example.jpg', function(err, file, is_infected) {
if(err) {
console.log(err);
return false;
}
if(is_infected) {
res.send({msg: "File is infected!"});
} else {
res.send({msg: "File is clean!"});
}
});
Allows you to scan an entire directory for infected files.
dir_path
(string) Full path to the directory to scan.end_callback
(function) Will be called when the entire directory has been completely scanned. This callback takes 3 parameters:err
(string or null) A standard error message string (null if no error)good_files
(array) List of the full paths to all files that are clean.bad_files
(array) List of the full paths to all files that are infected.file_callback
(function) Will be called after each file in the directory has been scanned. This is useful for keeping track of the progress of the scan. This callback takes 3 parameters:err
(string or null) A standard error message string (null if no error)file
(string) Path to the file that just got scanned.is_infected
(boolean) True: File is infected; False: File is clean.clam.scan_dir('/some/path/to/scan', function(err, good_files, bad_files) {
if(!err) {
if(bad_files.length > 0) {
res.send({msg: "Your directory was infected. The offending files have been quarantined."});
} else {
res.send({msg: "Everything looks good! No problems here!."});
}
} else {
// Do some error handling
}
});
This allows you to scan many files that might be in different directories or maybe only certain files of a single directory. This is essentially a wrapper for is_infected
that simplifies the process of scanning many files but not a whole directory.
files
(array) A list of strings representing full paths to files you want scanned.end_callback
(function) Will be called when the entire directory has been completely scanned. This callback takes 3 parameters:err
A standard error message string (null if no error)good_files
(array) List of the full paths to all files that are clean.bad_files
(array) List of the full paths to all files that are infected.file_callback
(function) Will be called after each file in the directory has been scanned. This is useful for keeping track of the progress of the scan. This callback takes 3 parameters:err
(string or null) A standard error message string (null if no error)file
(string) Path to the file that just got scanned.is_infected
(boolean) True: File is infected; False: File is clean.var scan_status = {
good: 0,
bad: 0
};
var files = [
'/path/to/file/1.jpg',
'/path/to/file/2.mov',
'/path/to/file/3.rb'
];
clam.scan_files(files, function(err, good_files, bad_files) {
if(!err) {
if(bad_files.length > 0) {
res.send({
msg: good_files.length + ' files were OK. ' + bad_files.length + ' were infected!',
bad: bad_files,
good: good_files
});
} else {
res.send({msg: "Everything looks good! No problems here!."});
}
} else {
// Do some error handling
}
}, function(err, file, is_infected) {
if(is_infected) {
scan_status.bad++;
} else {
scan_status.good++;
}
console.log("Scan Status: " + (scan_status.bad + scan_status.good) + "/" + files.length);
});
Got a missing feature you'd like to use? Found a bug? Go ahead and fork this repo, build the feature and issue a pull request.
FAQs
Use Node JS to scan files on your server with ClamAV's clamscan/clamdscan binary or via TCP to a remote server or local UNIX Domain socket. This is especially useful for scanning uploaded files provided by un-trusted sources.
We found that clamscan demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).