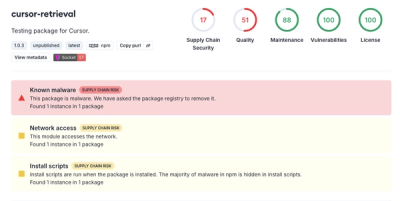
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
JavaScript inheritance implementation.
Thanks to my inspirations: JSFace by Tan Nhu, my.Class by Jie Meng-Gerard, simple inheritance by John Resig, and Underscore.js by Jeremy Ashkenas.
$ npm install clase
See test/clase_test.js
.
Create a Class with a constructor
var MyClass = Clase({
constructor: function (color) {
this.color = color;
}
});
var myclass = new MyClass('blue');
myclass.name; // 'blue'
Extend another class
var MyClass2 = Clase(MyClass, {
getColor: function () {
return this.color;
}
});
var myclass2 = new MyClass2('red');
myclass2.getColor(); // 'red'
// __super__ stores the parent prototype
MyClass2.__super__;
Extend the constructor or any method calling _super()
var MyClass2 = Clase(MyClass, {
constructor: function (color, number) {
this._super(color);
this.number = number;
}
});
var myclass2 = new MyClass2('red', 3);
myclass2.color; // 'red'
// __super__ stores the parent prototype
myclass2.number = 3;
Create static members with a second objecto when creating the class for the first time
var MyClass = Clase({
constructor: function (color) {
this.color = color;
}
}, {
isStatic: true
});
MyClass.isStatic; // true
Or create static members when extending from another class with the third argument
var MyClass2 = Clase(MyClass, {
constructor: function (color, number) {
this._super(color);
this.number = number;
},
getColor: function () {
return 'color ' + this.color + ' is #' + this.number;
}
}, {
isStatic: true
});
MyClass2.isStatic; // true
Or create create a class passing three objects:
var MyClass = Clase({
constructor: function (color) {
this.color = color;
}
}, {
constructor: function (color, number) {
this._super(color);
this.number = number;
},
getColor: function () {
return 'color ' + this.color + ' is #' + this.number;
}
}, {
isStatic: true
});
var myclass = new MyClass('red', 3);
myclass.getColor(); // 'color red is #3'
Extend two already created classes into a new one
var MyClass = Clase({
constructor: function (color) {
this.color = color;
}
});
var MyClass2 = Clase({
constructor: function (color, number) {
this._super(color);
this.number = number;
},
getColor: function () {
return 'color ' + this.color + ' is #' + this.number;
}
});
var MyClass3 = Clase(MyClass, MyClass2);
var myclass3 = new MyClass3('red', 3);
myclass3.getColor(); // 'color red is #3'
It also supports extending Backbone objects, for example a Backbone.Model with the nice _super()
method
var MyModel = Clase(Backbone.Model, {
initialize: function () {
this.set('color', 'red');
}
});
var MyModel2 = Clase(MyModel, {
initialize: function () {
this._super();
this.set('number', 3);
}
});
var mymodel2 = new MyModel2();
mymodel2.get('color'); // 'red'
mymodel2.get('number'); // 3
$ npm install
$ npm test
Open the test/clase_test.html
See LICENSE.txt
FAQs
JavaScript inheritance
We found that clase demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.