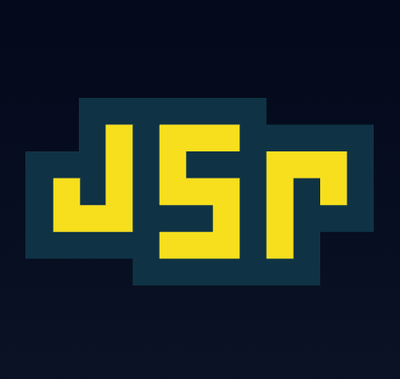
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
cloudant-promises
Advanced tools
A fork of nano-promises that also wraps the extra methods from cloudant
nano-promises
is a lightweight wrapper around the fabulous
nano
driver. It provides
the same features but uses promises instead of callbacks.
import nano from 'nano';
import prom from 'nano-promises';
var db = prom(nano('http://localhost:5984')).db.use('test');
db.insert({ 'crazy': true }, 'rabbit')
.then(function([body, headers]) {
console.log(body)
})
.catch(function(err) {
console.error(err);
});
nano-promises
promises always resolve to a value of the form [body, headers]
or reject to a value of the form error
. So it is important to
use desructuring in the then
handler of the promise.
async/await
The above example does not seem convincing to swith to a promise-based
approach. nano-promises
becomes very handy when used together
with the async/await
proposal for ES7:
var isRabbitCrazy = async function() {
try {
var [doc] = await db.get('rabbit');
return doc.crazy;
} catch(err) {
console.log('error fetching rabbit:', err);
throw err;
}
};
I don't know about you but I find this very expressive!
I love the nano
library. It has a very good api design and there's
a lot of work put into it!
The code in this repo is mostly adapted from co-nano
, but with
promises instead of thunks.
This code is licensed under the ISC license
FAQs
A fork of nano-promises that also wraps the extra methods from cloudant
We found that cloudant-promises demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.